Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / Core / XmlReaderSettings.cs / 1305376 / XmlReaderSettings.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.IO; using System.Diagnostics; using System.Security.Permissions; #if !SILVERLIGHT using System.Xml.Schema; #endif using System.Runtime.Versioning; namespace System.Xml { // XmlReaderSettings class specifies basic features of an XmlReader. #if !SILVERLIGHT [PermissionSetAttribute(SecurityAction.InheritanceDemand, Name = "FullTrust")] #endif public sealed class XmlReaderSettings { // // Fields // // Nametable XmlNameTable nameTable; // XmlResolver XmlResolver xmlResolver; // Text settings int lineNumberOffset; int linePositionOffset; // Conformance settings ConformanceLevel conformanceLevel; bool checkCharacters; long maxCharactersInDocument; long maxCharactersFromEntities; // Filtering settings bool ignoreWhitespace; bool ignorePIs; bool ignoreComments; // security settings DtdProcessing dtdProcessing; #if !SILVERLIGHT //Validation settings ValidationType validationType; XmlSchemaValidationFlags validationFlags; XmlSchemaSet schemas; ValidationEventHandler valEventHandler; #endif // other settings bool closeInput; // read-only flag bool isReadOnly; // // Constructor // public XmlReaderSettings() { Initialize(); } // // Properties // // Nametable public XmlNameTable NameTable { get { return nameTable; } set { CheckReadOnly("NameTable"); nameTable = value; } } // XmlResolver public XmlResolver XmlResolver { set { CheckReadOnly("XmlResolver"); xmlResolver = value; } } internal XmlResolver GetXmlResolver() { return xmlResolver; } // Text settings public int LineNumberOffset { get { return lineNumberOffset; } set { CheckReadOnly("LineNumberOffset"); lineNumberOffset = value; } } public int LinePositionOffset { get { return linePositionOffset; } set { CheckReadOnly("LinePositionOffset"); linePositionOffset = value; } } // Conformance settings public ConformanceLevel ConformanceLevel { get { return conformanceLevel; } set { CheckReadOnly("ConformanceLevel"); if ((uint)value > (uint)ConformanceLevel.Document) { throw new ArgumentOutOfRangeException("value"); } conformanceLevel = value; } } public bool CheckCharacters { get { return checkCharacters; } set { CheckReadOnly("CheckCharacters"); checkCharacters = value; } } public long MaxCharactersInDocument { get { return maxCharactersInDocument; } set { CheckReadOnly("MaxCharactersInDocument"); if (value < 0) { throw new ArgumentOutOfRangeException("value"); } maxCharactersInDocument = value; } } public long MaxCharactersFromEntities { get { return maxCharactersFromEntities; } set { CheckReadOnly("MaxCharactersFromEntities"); if (value < 0) { throw new ArgumentOutOfRangeException("value"); } maxCharactersFromEntities = value; } } // Filtering settings public bool IgnoreWhitespace { get { return ignoreWhitespace; } set { CheckReadOnly("IgnoreWhitespace"); ignoreWhitespace = value; } } public bool IgnoreProcessingInstructions { get { return ignorePIs; } set { CheckReadOnly("IgnoreProcessingInstructions"); ignorePIs = value; } } public bool IgnoreComments { get { return ignoreComments; } set { CheckReadOnly("IgnoreComments"); ignoreComments = value; } } #if !SILVERLIGHT [Obsolete("Use XmlReaderSettings.DtdProcessing property instead.")] public bool ProhibitDtd { get { return dtdProcessing == DtdProcessing.Prohibit; } set { CheckReadOnly("ProhibitDtd"); dtdProcessing = value ? DtdProcessing.Prohibit : DtdProcessing.Parse; } } #endif public DtdProcessing DtdProcessing { get { return dtdProcessing; } set { CheckReadOnly("DtdProcessing"); if ((uint)value > (uint)DtdProcessing.Parse) { throw new ArgumentOutOfRangeException("value"); } dtdProcessing = value; } } public bool CloseInput { get { return closeInput; } set { CheckReadOnly("CloseInput"); closeInput = value; } } #if !SILVERLIGHT public ValidationType ValidationType { get { return validationType; } set { CheckReadOnly("ValidationType"); if ((uint)value > (uint)ValidationType.Schema) { throw new ArgumentOutOfRangeException("value"); } validationType = value; } } public XmlSchemaValidationFlags ValidationFlags { get { return validationFlags; } set { CheckReadOnly("ValidationFlags"); if ((uint)value > (uint)(XmlSchemaValidationFlags.ProcessInlineSchema | XmlSchemaValidationFlags.ProcessSchemaLocation | XmlSchemaValidationFlags.ReportValidationWarnings | XmlSchemaValidationFlags.ProcessIdentityConstraints | XmlSchemaValidationFlags.AllowXmlAttributes)) { throw new ArgumentOutOfRangeException("value"); } validationFlags = value; } } public XmlSchemaSet Schemas { get { if (schemas == null) { schemas = new XmlSchemaSet(); } return schemas; } set { CheckReadOnly("Schemas"); schemas = value; } } public event ValidationEventHandler ValidationEventHandler { add { CheckReadOnly("ValidationEventHandler"); valEventHandler += value; } remove { CheckReadOnly("ValidationEventHandler"); valEventHandler -= value; } } #endif // // Public methods // public void Reset() { CheckReadOnly("Reset"); Initialize(); } public XmlReaderSettings Clone() { XmlReaderSettings clonedSettings = this.MemberwiseClone() as XmlReaderSettings; clonedSettings.ReadOnly = false; return clonedSettings; } // // Internal methods // #if !SILVERLIGHT internal ValidationEventHandler GetEventHandler() { return valEventHandler; } #endif #if !SILVERLIGHT [ResourceConsumption(ResourceScope.Machine)] [ResourceExposure(ResourceScope.Machine)] #endif internal XmlReader CreateReader(String inputUri, XmlParserContext inputContext) { if (inputUri == null) { throw new ArgumentNullException("inputUri"); } if (inputUri.Length == 0) { throw new ArgumentException(Res.GetString(Res.XmlConvert_BadUri), "inputUri"); } // resolve and open the url XmlResolver tmpResolver = this.GetXmlResolver(); if (tmpResolver == null) { tmpResolver = CreateDefaultResolver(); } Uri baseUri = tmpResolver.ResolveUri(null, inputUri); Stream stream = (Stream)tmpResolver.GetEntity(baseUri, string.Empty, typeof(Stream)); if (stream == null) { throw new XmlException(Res.Xml_CannotResolveUrl, inputUri); } // need to clone the settigns so that we can set CloseInput to true to make sure the stream gets closed in the end XmlReaderSettings newSettings = this; if (!newSettings.CloseInput) { newSettings = newSettings.Clone(); newSettings.CloseInput = true; } try { // create reader return newSettings.CreateReader(stream, baseUri, null, inputContext); } catch { stream.Close(); throw; } } internal XmlReader CreateReader(Stream input, Uri baseUri, string baseUriString, XmlParserContext inputContext) { if (input == null) { throw new ArgumentNullException("input"); } if (baseUriString == null) { if (baseUri == null) { baseUriString = string.Empty; } else { baseUriString = baseUri.ToString(); } } // create text XML reader XmlReader reader = new XmlTextReaderImpl(input, null, 0, this, baseUri, baseUriString, inputContext, closeInput); #if !SILVERLIGHT // wrap with validating reader if (this.ValidationType != ValidationType.None) { reader = AddValidation(reader); } #endif return reader; } internal XmlReader CreateReader(TextReader input, string baseUriString, XmlParserContext inputContext) { if (input == null) { throw new ArgumentNullException("input"); } if (baseUriString == null) { baseUriString = string.Empty; } // create xml text reader XmlReader reader = new XmlTextReaderImpl(input, this, baseUriString, inputContext); #if !SILVERLIGHT // wrap with validating reader if (this.ValidationType != ValidationType.None) { reader = AddValidation(reader); } #endif return reader; } internal XmlReader CreateReader(XmlReader reader) { if (reader == null) { throw new ArgumentNullException("reader"); } #if SILVERLIGHT // wrap with conformance layer (if needed) return AddConformanceWrapper(reader); #else // wrap with validating reader and a conformance layer (if needed) return AddValidationAndConformanceWrapper(reader); #endif } internal bool ReadOnly { get { return isReadOnly; } set { isReadOnly = value; } } void CheckReadOnly(string propertyName) { if (isReadOnly) { throw new XmlException(Res.Xml_ReadOnlyProperty, this.GetType().Name + '.' + propertyName); } } // // Private methods // void Initialize() { nameTable = null; xmlResolver = CreateDefaultResolver(); lineNumberOffset = 0; linePositionOffset = 0; checkCharacters = true; conformanceLevel = ConformanceLevel.Document; ignoreWhitespace = false; ignorePIs = false; ignoreComments = false; dtdProcessing = DtdProcessing.Prohibit; closeInput = false; maxCharactersFromEntities = 0; maxCharactersInDocument = 0; #if !SILVERLIGHT schemas = null; validationType = ValidationType.None; validationFlags = XmlSchemaValidationFlags.ProcessIdentityConstraints; validationFlags |= XmlSchemaValidationFlags.AllowXmlAttributes; #endif isReadOnly = false; } XmlResolver CreateDefaultResolver() { #if SILVERLIGHT return new XmlXapResolver(); #else return new XmlUrlResolver(); #endif } #if !SILVERLIGHT internal XmlReader AddValidation(XmlReader reader) { if (this.validationType == ValidationType.Schema) { reader = new XsdValidatingReader(reader, this.GetXmlResolver(), this); } else if (this.validationType == ValidationType.DTD) { reader = CreateDtdValidatingReader(reader); } return reader; } private XmlReader AddValidationAndConformanceWrapper(XmlReader reader) { // wrap with DTD validating reader if (this.validationType == ValidationType.DTD) { reader = CreateDtdValidatingReader(reader); } // add conformance checking (must go after DTD validation because XmlValidatingReader works only on XmlTextReader), // but before XSD validation because of typed value access reader = AddConformanceWrapper(reader); if (this.validationType == ValidationType.Schema) { reader = new XsdValidatingReader(reader, GetXmlResolver(), this); } return reader; } private XmlValidatingReaderImpl CreateDtdValidatingReader(XmlReader baseReader) { return new XmlValidatingReaderImpl(baseReader, this.GetEventHandler(), (this.ValidationFlags & XmlSchemaValidationFlags.ProcessIdentityConstraints) != 0); } #endif internal XmlReader AddConformanceWrapper(XmlReader baseReader) { XmlReaderSettings baseReaderSettings = baseReader.Settings; bool checkChars = false; bool noWhitespace = false; bool noComments = false; bool noPIs = false; DtdProcessing dtdProc = (DtdProcessing)(-1); bool needWrap = false; if (baseReaderSettings == null) { #pragma warning disable 618 #if SILVERLIGHT if (this.conformanceLevel != ConformanceLevel.Auto) { throw new InvalidOperationException(Res.GetString(Res.Xml_IncompatibleConformanceLevel, this.conformanceLevel.ToString())); } #else if (this.conformanceLevel != ConformanceLevel.Auto && this.conformanceLevel != XmlReader.GetV1ConformanceLevel(baseReader)) { throw new InvalidOperationException(Res.GetString(Res.Xml_IncompatibleConformanceLevel, this.conformanceLevel.ToString())); } #endif #if !SILVERLIGHT // get the V1 XmlTextReader ref XmlTextReader v1XmlTextReader = baseReader as XmlTextReader; if (v1XmlTextReader == null) { XmlValidatingReader vr = baseReader as XmlValidatingReader; if (vr != null) { v1XmlTextReader = (XmlTextReader)vr.Reader; } } #endif // assume the V1 readers already do all conformance checking; // wrap only if IgnoreWhitespace, IgnoreComments, IgnoreProcessingInstructions or ProhibitDtd is true; if (this.ignoreWhitespace) { WhitespaceHandling wh = WhitespaceHandling.All; #if !SILVERLIGHT // special-case our V1 readers to see if whey already filter whitespaces if (v1XmlTextReader != null) { wh = v1XmlTextReader.WhitespaceHandling; } #endif if (wh == WhitespaceHandling.All) { noWhitespace = true; needWrap = true; } } if (this.ignoreComments) { noComments = true; needWrap = true; } if (this.ignorePIs) { noPIs = true; needWrap = true; } // DTD processing DtdProcessing baseDtdProcessing = DtdProcessing.Parse; #if !SILVERLIGHT if (v1XmlTextReader != null) { baseDtdProcessing = v1XmlTextReader.DtdProcessing; } #endif if ((this.dtdProcessing == DtdProcessing.Prohibit && baseDtdProcessing != DtdProcessing.Prohibit) || (this.dtdProcessing == DtdProcessing.Ignore && baseDtdProcessing == DtdProcessing.Parse)) { dtdProc = this.dtdProcessing; needWrap = true; } #pragma warning restore 618 } else { if (this.conformanceLevel != baseReaderSettings.ConformanceLevel && this.conformanceLevel != ConformanceLevel.Auto) { throw new InvalidOperationException(Res.GetString(Res.Xml_IncompatibleConformanceLevel, this.conformanceLevel.ToString())); } if (this.checkCharacters && !baseReaderSettings.CheckCharacters) { checkChars = true; needWrap = true; } if (this.ignoreWhitespace && !baseReaderSettings.IgnoreWhitespace) { noWhitespace = true; needWrap = true; } if (this.ignoreComments && !baseReaderSettings.IgnoreComments) { noComments = true; needWrap = true; } if (this.ignorePIs && !baseReaderSettings.IgnoreProcessingInstructions) { noPIs = true; needWrap = true; } if ((this.dtdProcessing == DtdProcessing.Prohibit && baseReaderSettings.DtdProcessing != DtdProcessing.Prohibit) || (this.dtdProcessing == DtdProcessing.Ignore && baseReaderSettings.DtdProcessing == DtdProcessing.Parse)) { dtdProc = this.dtdProcessing; needWrap = true; } } if (needWrap) { IXmlNamespaceResolver readerAsNSResolver = baseReader as IXmlNamespaceResolver; if (readerAsNSResolver != null) { return new XmlCharCheckingReaderWithNS(baseReader, readerAsNSResolver, checkChars, noWhitespace, noComments, noPIs, dtdProc); } else { return new XmlCharCheckingReader(baseReader, checkChars, noWhitespace, noComments, noPIs, dtdProc); } } else { return baseReader; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
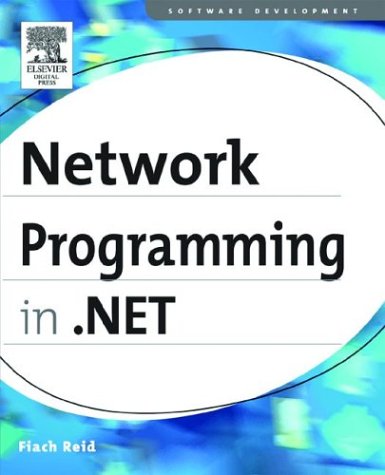
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OutputCacheProfileCollection.cs
- NotCondition.cs
- EmbeddedMailObjectsCollection.cs
- ListComponentEditor.cs
- Preprocessor.cs
- BitmapInitialize.cs
- columnmapfactory.cs
- StringWriter.cs
- TextSelectionProcessor.cs
- CatalogUtil.cs
- FixedSOMContainer.cs
- Aggregates.cs
- FunctionDetailsReader.cs
- Int64AnimationBase.cs
- Interlocked.cs
- UIElement3DAutomationPeer.cs
- HTMLTagNameToTypeMapper.cs
- NotImplementedException.cs
- SystemIPAddressInformation.cs
- PropertyValueEditor.cs
- TimeSpanStorage.cs
- QueryExtender.cs
- ToolStripOverflow.cs
- Control.cs
- SymLanguageType.cs
- HandlerBase.cs
- BuiltInPermissionSets.cs
- SendActivityDesignerTheme.cs
- StrongTypingException.cs
- ProcessThread.cs
- CatalogPartChrome.cs
- RelationshipEntry.cs
- ObjectParameter.cs
- dbenumerator.cs
- SqlTriggerAttribute.cs
- SqlDataSourceCommandEventArgs.cs
- ResourceDescriptionAttribute.cs
- NamespaceTable.cs
- AdapterUtil.cs
- XamlSerializerUtil.cs
- ApplicationException.cs
- TopClause.cs
- TextContainerChangeEventArgs.cs
- HttpClientCertificate.cs
- sapiproxy.cs
- Drawing.cs
- ObjectDataSourceMethodEditor.cs
- WebPartConnectionsConfigureVerb.cs
- SineEase.cs
- DetailsViewRowCollection.cs
- UnmanagedMemoryStream.cs
- MemoryMappedFileSecurity.cs
- GradientStop.cs
- KeyValueConfigurationElement.cs
- _Connection.cs
- DataGridViewCellStyleConverter.cs
- TraceHandler.cs
- ScopelessEnumAttribute.cs
- WebBrowserHelper.cs
- SiteMapSection.cs
- OleServicesContext.cs
- DataGridViewHitTestInfo.cs
- ThreadAttributes.cs
- CodeGotoStatement.cs
- DbDataRecord.cs
- EncodingNLS.cs
- TimeIntervalCollection.cs
- ButtonChrome.cs
- QueryExecutionOption.cs
- ListViewSortEventArgs.cs
- String.cs
- QilParameter.cs
- Schema.cs
- ListViewGroupConverter.cs
- Int32EqualityComparer.cs
- PrintControllerWithStatusDialog.cs
- DisplayNameAttribute.cs
- AuthenticationSection.cs
- TextElementEnumerator.cs
- CaseInsensitiveHashCodeProvider.cs
- NaturalLanguageHyphenator.cs
- AppDomainEvidenceFactory.cs
- RegistryExceptionHelper.cs
- ContainsSearchOperator.cs
- QuaternionAnimation.cs
- ToolBarPanel.cs
- OrderByBuilder.cs
- X509ClientCertificateCredentialsElement.cs
- StringResourceManager.cs
- ItemCollection.cs
- SmtpDigestAuthenticationModule.cs
- SystemSounds.cs
- WebBrowserEvent.cs
- AppDomainManager.cs
- sqlstateclientmanager.cs
- DocumentStream.cs
- CancellationTokenSource.cs
- SemaphoreFullException.cs
- DataGridViewRowCancelEventArgs.cs
- BezierSegment.cs