Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / tx / System / Transactions / EnlistmentState.cs / 1305376 / EnlistmentState.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Transactions { using System; using System.Diagnostics; using System.Globalization; using System.Threading; using System.Transactions.Diagnostics; // Base class for all enlistment states abstract class EnlistmentState { internal abstract void EnterState( InternalEnlistment enlistment ); internal static EnlistmentStatePromoted _enlistmentStatePromoted; // Object for synchronizing access to the entire class( avoiding lock( typeof( ... )) ) private static object classSyncObject; // Helper object for static synchronization private static object ClassSyncObject { get { if( classSyncObject == null ) { object o = new object(); Interlocked.CompareExchange( ref classSyncObject, o, null ); } return classSyncObject; } } internal static EnlistmentStatePromoted _EnlistmentStatePromoted { get { if (_enlistmentStatePromoted == null) { lock (ClassSyncObject) { if (_enlistmentStatePromoted == null) { EnlistmentStatePromoted temp = new EnlistmentStatePromoted(); Thread.MemoryBarrier(); _enlistmentStatePromoted = temp; } } } return _enlistmentStatePromoted; } } internal virtual void EnlistmentDone( InternalEnlistment enlistment ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void Prepared( InternalEnlistment enlistment ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ForceRollback( InternalEnlistment enlistment, Exception e ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void Committed( InternalEnlistment enlistment ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void Aborted( InternalEnlistment enlistment, Exception e ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void InDoubt( InternalEnlistment enlistment, Exception e ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual byte[] RecoveryInformation( InternalEnlistment enlistment ) { throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void InternalAborted( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void InternalCommitted( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void InternalIndoubt( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStateCommitting( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStatePromoted( InternalEnlistment enlistment, IPromotedEnlistment promotedEnlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStateDelegated( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStatePreparing( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } internal virtual void ChangeStateSinglePhaseCommit( InternalEnlistment enlistment ) { Debug.Assert( false, string.Format( null, "Invalid Event for InternalEnlistment State; Current State: {0}", this.GetType() )); throw TransactionException.CreateEnlistmentStateException( SR.GetString( SR.TraceSourceLtm ), null ); } } internal class EnlistmentStatePromoted : EnlistmentState { internal override void EnterState( InternalEnlistment enlistment ) { enlistment.State = this; } internal override void EnlistmentDone( InternalEnlistment enlistment ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.EnlistmentDone(); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void Prepared( InternalEnlistment enlistment ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.Prepared(); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void ForceRollback( InternalEnlistment enlistment, Exception e ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.ForceRollback( e ); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void Committed( InternalEnlistment enlistment ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.Committed(); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void Aborted( InternalEnlistment enlistment, Exception e ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.Aborted( e ); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override void InDoubt( InternalEnlistment enlistment, Exception e ) { Monitor.Exit( enlistment.SyncRoot ); try { enlistment.PromotedEnlistment.InDoubt( e ); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } internal override byte[] RecoveryInformation( InternalEnlistment enlistment ) { Monitor.Exit( enlistment.SyncRoot ); try { return enlistment.PromotedEnlistment.GetRecoveryInformation(); } finally { #pragma warning disable 0618 //@ Monitor.Enter(enlistment.SyncRoot); #pragma warning restore 0618 } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
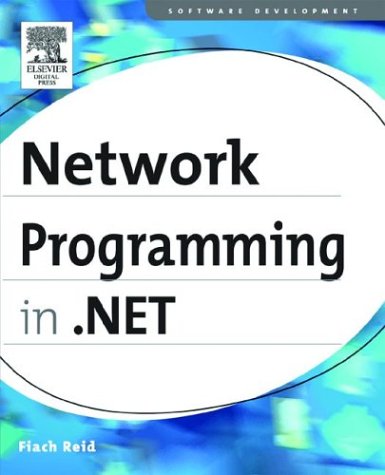
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StyleCollection.cs
- FixedNode.cs
- DataMemberAttribute.cs
- DrawingGroup.cs
- Separator.cs
- StrongTypingException.cs
- Directory.cs
- EntityDesignPluralizationHandler.cs
- IPEndPointCollection.cs
- SmtpNegotiateAuthenticationModule.cs
- WebPartDisplayModeEventArgs.cs
- SystemGatewayIPAddressInformation.cs
- CompositionTarget.cs
- DbConnectionInternal.cs
- MD5.cs
- BitConverter.cs
- XmlArrayItemAttribute.cs
- XPathCompileException.cs
- DuplexChannelFactory.cs
- KeyConstraint.cs
- OletxDependentTransaction.cs
- StrokeNodeOperations2.cs
- ModelTreeEnumerator.cs
- WindowsListViewItem.cs
- HttpCachePolicyWrapper.cs
- UpdateProgress.cs
- SystemBrushes.cs
- WpfKnownTypeInvoker.cs
- DataGridViewRowsAddedEventArgs.cs
- SqlServer2KCompatibilityCheck.cs
- ListViewInsertionMark.cs
- IdnMapping.cs
- DependencyPropertyChangedEventArgs.cs
- ApplicationId.cs
- AddInPipelineAttributes.cs
- NumericPagerField.cs
- TypeValidationEventArgs.cs
- WebControlAdapter.cs
- TailCallAnalyzer.cs
- CellIdBoolean.cs
- HandlerBase.cs
- UIElement3D.cs
- RegexStringValidator.cs
- LiteralTextParser.cs
- Validator.cs
- PrimitiveXmlSerializers.cs
- WsiProfilesElement.cs
- AppSettingsExpressionBuilder.cs
- SqlParameterCollection.cs
- MiniCustomAttributeInfo.cs
- BitmapEffectInputData.cs
- AdornerLayer.cs
- DataGridViewColumn.cs
- Int64Storage.cs
- EdmType.cs
- XmlImplementation.cs
- MemberRelationshipService.cs
- DocumentSequenceHighlightLayer.cs
- EventBuilder.cs
- ToolStripDropDownClosedEventArgs.cs
- ITextView.cs
- TypographyProperties.cs
- FixedDocumentSequencePaginator.cs
- ControlParser.cs
- SizeConverter.cs
- DBCommand.cs
- APCustomTypeDescriptor.cs
- RTLAwareMessageBox.cs
- InterleavedZipPartStream.cs
- KeysConverter.cs
- SqlDataSourceSelectingEventArgs.cs
- TypedMessageConverter.cs
- DecoratedNameAttribute.cs
- SafeProcessHandle.cs
- FilterQuery.cs
- XmlHierarchicalDataSourceView.cs
- Brush.cs
- ErrorWebPart.cs
- PopupControlService.cs
- ToolboxItemFilterAttribute.cs
- CheckedPointers.cs
- RefreshEventArgs.cs
- XamlTreeBuilder.cs
- CustomCategoryAttribute.cs
- RuleConditionDialog.cs
- SRGSCompiler.cs
- AuthenticationSection.cs
- HMACSHA384.cs
- QuaternionAnimation.cs
- AssemblyBuilderData.cs
- NumericExpr.cs
- BinaryParser.cs
- HttpHandlerActionCollection.cs
- Select.cs
- PerformanceCounter.cs
- OdbcCommand.cs
- TransformerConfigurationWizardBase.cs
- SpecularMaterial.cs
- IgnoreFileBuildProvider.cs
- TabItemWrapperAutomationPeer.cs