Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / ModelProviders / DLinqColumnProvider.cs / 1305376 / DLinqColumnProvider.cs
using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.DataAnnotations; using System.Data.Linq; using System.Data.Linq.Mapping; using System.Globalization; using System.Reflection; using System.Text.RegularExpressions; using System.Xml.Linq; namespace System.Web.DynamicData.ModelProviders { internal sealed class DLinqColumnProvider : ColumnProvider { private static Regex s_varCharRegEx = new Regex(@"N?(?:Var)?Char\(([0-9]+)\)", RegexOptions.IgnoreCase); // accepts char, nchar, varchar, and nvarchar private AttributeCollection _attributes; private AssociationProvider _association; private bool _isAssociation; public DLinqColumnProvider(DLinqTableProvider table, MetaDataMember member) : base(table) { Member = member; Name = member.Name; ColumnType = GetMemberType(member); IsPrimaryKey = member.IsPrimaryKey; IsGenerated = member.IsDbGenerated; _isAssociation = member.IsAssociation; IsCustomProperty = !member.IsAssociation && Member.DbType == null; Nullable = Member.IsAssociation ? Member.Association.IsNullable : Member.CanBeNull; MaxLength = ProcessMaxLength(ColumnType, Member.DbType); IsSortable = ProcessIsSortable(ColumnType, Member.DbType); } public override AttributeCollection Attributes { get { if (!Member.IsDiscriminator) return base.Attributes; if (_attributes == null) { ListnewAttributes = new List (); bool foundScaffoldAttribute = false; foreach (Attribute attr in base.Attributes) { if (attr is ScaffoldColumnAttribute) { foundScaffoldAttribute = true; break; } newAttributes.Add(attr); } if (foundScaffoldAttribute) _attributes = base.Attributes; else { newAttributes.Add(new ScaffoldColumnAttribute(false)); _attributes = new AttributeCollection(newAttributes.ToArray()); } } return _attributes; } } // internal to facilitate unit testing internal static int ProcessMaxLength(Type memberType, String dbType) { // Only strings and chars that come in from a database have max lengths if (dbType == null || (memberType != typeof(string) && Misc.RemoveNullableFromType(memberType) != typeof(char))) return 0; if (dbType.StartsWith("NText", StringComparison.OrdinalIgnoreCase)) { return Int32.MaxValue >> 1; // see sql server 2005 spec for ntext } if (dbType.StartsWith("Text", StringComparison.OrdinalIgnoreCase)) { return Int32.MaxValue; // see sql server 2005 spec for text } if (dbType.StartsWith("NVarChar(MAX)", StringComparison.OrdinalIgnoreCase)) { return (Int32.MaxValue >> 1) - 2; // see sql server 2005 spec for nvarchar } if (dbType.StartsWith("VarChar(MAX)", StringComparison.OrdinalIgnoreCase)) { return Int32.MaxValue - 2; // see sql server 2005 spec for varchar } Match m = s_varCharRegEx.Match(dbType); if (m.Success) { return Int32.Parse(m.Groups[1].Value, CultureInfo.InvariantCulture); } return 0; } internal static bool ProcessIsSortable(Type memberType, String dbType) { if (dbType == null) return false; if (memberType == typeof(string) && (dbType.StartsWith("Text", StringComparison.OrdinalIgnoreCase) || dbType.StartsWith("NText", StringComparison.OrdinalIgnoreCase))) { return false; } if (memberType == typeof(Binary) && dbType.StartsWith("Image", StringComparison.OrdinalIgnoreCase)) { return false; } if (memberType == typeof(XElement)) { return false; } return true; } internal MetaDataMember Member { get; private set; } internal void Initialize() { if (_isAssociation && _association == null) { _association = new DLinqAssociationProvider(this); } } internal bool ShouldRemove { get; set; } private static Type GetMemberType(MetaDataMember member) { Type type = member.Type; if (type.IsGenericType && type.GetGenericTypeDefinition() == typeof(EntitySet<>)) { return type.GetGenericArguments()[0]; } else { return type; } } #region IEntityMember Members public override PropertyInfo EntityTypeProperty { get { return (PropertyInfo)Member.Member; } } public override AssociationProvider Association { get { Initialize(); return _association; } } internal new bool IsForeignKeyComponent { set { base.IsForeignKeyComponent = value; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
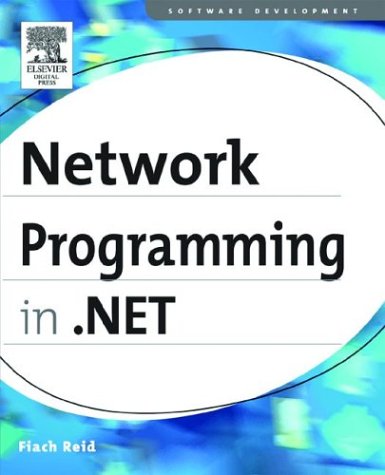
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Expression.DebuggerProxy.cs
- DoubleAnimation.cs
- WorkflowApplicationAbortedEventArgs.cs
- GlyphCache.cs
- Compress.cs
- PathGeometry.cs
- ObjectDataSourceChooseTypePanel.cs
- DateTimeFormatInfo.cs
- XPathPatternParser.cs
- DataServiceEntityAttribute.cs
- AssertFilter.cs
- DbDataRecord.cs
- ComEventsMethod.cs
- ParameterElement.cs
- ModifierKeysConverter.cs
- Method.cs
- HttpPostedFile.cs
- SiteMapDesignerDataSourceView.cs
- SamlConstants.cs
- ResXBuildProvider.cs
- DataGridTableCollection.cs
- ChannelOptions.cs
- RectValueSerializer.cs
- DigestTraceRecordHelper.cs
- ProxyGenerator.cs
- XmlSchemaComplexContentRestriction.cs
- CodeActivityContext.cs
- Int16.cs
- compensatingcollection.cs
- TerminatingOperationBehavior.cs
- SafeHandles.cs
- CapabilitiesAssignment.cs
- ContravarianceAdapter.cs
- TableRow.cs
- InputLangChangeRequestEvent.cs
- UnsafeNativeMethods.cs
- TemplatePropertyEntry.cs
- PartitionedStreamMerger.cs
- AppDomainProtocolHandler.cs
- WrapPanel.cs
- OSFeature.cs
- DrawingBrush.cs
- EventData.cs
- InvalidEnumArgumentException.cs
- DataGridViewButtonColumn.cs
- XmlSchemaType.cs
- QuaternionConverter.cs
- SystemIPAddressInformation.cs
- StrongNameHelpers.cs
- _DomainName.cs
- DataGridViewColumnHeaderCell.cs
- VirtualPathProvider.cs
- PenCursorManager.cs
- WebPartVerbsEventArgs.cs
- QueuedDeliveryRequirementsMode.cs
- DSACryptoServiceProvider.cs
- DocumentViewerBaseAutomationPeer.cs
- InvalidWorkflowException.cs
- LinqDataSourceInsertEventArgs.cs
- LinearGradientBrush.cs
- SessionPageStateSection.cs
- RC2CryptoServiceProvider.cs
- OperationResponse.cs
- SqlDependency.cs
- OleAutBinder.cs
- BooleanFunctions.cs
- WeakReferenceEnumerator.cs
- RefType.cs
- CodeMethodReturnStatement.cs
- File.cs
- TransformerConfigurationWizardBase.cs
- FilteredXmlReader.cs
- PersonalizationProviderHelper.cs
- CacheChildrenQuery.cs
- AutomationPatternInfo.cs
- ProcessHostMapPath.cs
- MimePart.cs
- DefaultPrintController.cs
- DurableInstanceContextProvider.cs
- Ops.cs
- SemanticResultKey.cs
- CodeAccessPermission.cs
- SQLBytesStorage.cs
- WebPartDesigner.cs
- CanExecuteRoutedEventArgs.cs
- PersianCalendar.cs
- OpacityConverter.cs
- CompiledXpathExpr.cs
- TransformProviderWrapper.cs
- XmlCharCheckingWriter.cs
- DoubleStorage.cs
- GraphicsContext.cs
- PopupRoot.cs
- DrawListViewSubItemEventArgs.cs
- APCustomTypeDescriptor.cs
- ConfigXmlAttribute.cs
- Int16AnimationBase.cs
- SmtpFailedRecipientException.cs
- SeparatorAutomationPeer.cs
- EnvironmentPermission.cs