Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / MS / Internal / Threading / ExceptionWrapper.cs / 1305600 / ExceptionWrapper.cs
// //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // //---------------------------------------------------------------------------- using Microsoft.Win32; // Registry & ComponentDispatcher & MSG using System.Security; // CAS using System.Security.Permissions; // Registry permissions using System.Runtime.InteropServices; // SEHException using System.Diagnostics; // Debug & Debugger using System.Threading; using MS.Internal.Threading; // ExceptionFilterHelper using MS.Internal.WindowsBase; namespace System.Windows.Threading { ////// Class for Filtering and Catching Exceptions /// internal class ExceptionWrapper { internal ExceptionWrapper() { _exceptionFilterHelper = new ExceptionFilterHelper( new InternalRealCallDelegate(InternalRealCall), new FilterExceptionDelegate(FilterException), new CatchExceptionDelegate(CatchException) ); } // Helper for exception filtering: // The real impl is in Base\MS\Internal\Threading\ExceptionFilterHelper.vb public object TryCatchWhen(object source, Delegate callback, object args, int numArgs, Delegate catchHandler) { return _exceptionFilterHelper.TryCatchWhen(source, callback, args, numArgs, catchHandler); // object result = null; // try // { // result = InternalRealCall(callback, args, numArgs); // } // catch(Exception e) WHEN FilterException( Exception e ); // { // if (e is NullReferenceException || e is SEHException) // throw; // else // if( ! CatchException(source, e) ) // throw; // } // return result; } private object InternalRealCall(Delegate callback, object args, int numArgs) { object result = null; Debug.Assert(numArgs == 0 || // old API, no args numArgs == 1 || // old API, 1 arg, the args param is it numArgs == -1); // new API, any number of args, the args param is an array of them // Support the fast-path for certain 0-param and 1-param delegates, even // of an arbitrary "params object[]" is passed. int numArgsEx = numArgs; object singleArg = args; if(numArgs == -1) { object[] argsArr = (object[])args; if (argsArr == null || argsArr.Length == 0) { numArgsEx = 0; } else if(argsArr.Length == 1) { numArgsEx = 1; singleArg = argsArr[0]; } } // Special-case delegates that we know about to avoid the // expensive DynamicInvoke call. if(numArgsEx == 0) { Action action = callback as Action; if (action != null) { action(); } else { Dispatcher.ShutdownCallback shutdownCallback = callback as Dispatcher.ShutdownCallback; if(shutdownCallback != null) { shutdownCallback(); } else { // The delegate could return anything. result = callback.DynamicInvoke(); } } } else if(numArgsEx == 1) { DispatcherOperationCallback dispatcherOperationCallback = callback as DispatcherOperationCallback; if(dispatcherOperationCallback != null) { result = dispatcherOperationCallback(singleArg); } else { SendOrPostCallback sendOrPostCallback = callback as SendOrPostCallback; if(sendOrPostCallback != null) { sendOrPostCallback(singleArg); } else { if(numArgs == -1) { // Explicitly pass an object[] to DynamicInvoke so that // it will not try to wrap the arg in another object[]. result = callback.DynamicInvoke((object[])args); } else { // By pass the args parameter as a single object, // DynamicInvoke will wrap it in an object[] due to the // params keyword. result = callback.DynamicInvoke(args); } } } } else { // Explicitly pass an object[] to DynamicInvoke so that // it will not try to wrap the arg in another object[]. result = callback.DynamicInvoke((object[])args); } return result; } private bool FilterException(object source, Exception e) { // If we have a Catch handler we should catch the exception // unless the Filter handler says we shouldn't. bool shouldCatch = (null != Catch); if(null != Filter) { shouldCatch = Filter(source, e); } return shouldCatch; } // This returns false when caller should rethrow the exception. // true means Exception is "handled" and things just continue on. private bool CatchException(object source, Exception e, Delegate catchHandler) { if (catchHandler != null) { if(catchHandler is DispatcherOperationCallback) { ((DispatcherOperationCallback)catchHandler)(null); } else { catchHandler.DynamicInvoke(null); } } if(null != Catch) return Catch(source, e); return false; } ////// Exception Catch Handler Delegate /// Returns true if the exception is "handled" /// Returns false if the caller should rethow the exception. /// public delegate bool CatchHandler(object source, Exception e); ////// Exception Catch Handler /// Returns true if the exception is "handled" /// Returns false if the caller should rethow the exception. /// public event CatchHandler Catch; public delegate bool FilterHandler(object source, Exception e); public event FilterHandler Filter; private ExceptionFilterHelper _exceptionFilterHelper; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
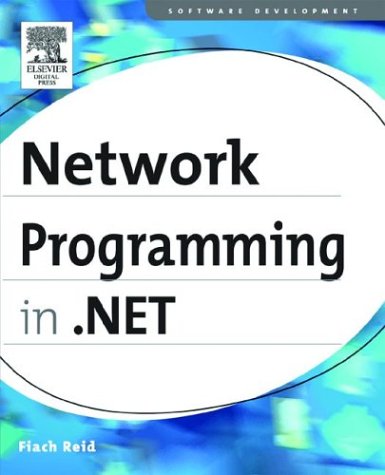
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XsdBuilder.cs
- CancellationHandlerDesigner.cs
- ADMembershipProvider.cs
- DataGridViewCellConverter.cs
- ObjRef.cs
- VectorAnimation.cs
- DocumentPageHost.cs
- SecurityTokenRequirement.cs
- Vector3DAnimationBase.cs
- COAUTHIDENTITY.cs
- VisualStateChangedEventArgs.cs
- RouteData.cs
- BasicViewGenerator.cs
- VersionPair.cs
- FacetChecker.cs
- SmtpClient.cs
- IndicFontClient.cs
- SectionInformation.cs
- RijndaelManagedTransform.cs
- TagPrefixCollection.cs
- NativeMsmqMessage.cs
- ParameterInfo.cs
- DrawingImage.cs
- ControlCommandSet.cs
- BuildTopDownAttribute.cs
- XmlDesignerDataSourceView.cs
- Guid.cs
- QilIterator.cs
- Win32Exception.cs
- ObjectStateEntryDbDataRecord.cs
- IPAddressCollection.cs
- TextBox.cs
- FilterElement.cs
- DataListItemCollection.cs
- ApplicationManager.cs
- InputProcessorProfilesLoader.cs
- OpenFileDialog.cs
- Metadata.cs
- ProviderCommandInfoUtils.cs
- TransformPatternIdentifiers.cs
- GridViewRowPresenterBase.cs
- HtmlInputFile.cs
- ConfigurationLocation.cs
- RelativeSource.cs
- ServicesUtilities.cs
- PropertyGeneratedEventArgs.cs
- ConfigurationCollectionAttribute.cs
- IIS7WorkerRequest.cs
- _UriSyntax.cs
- Number.cs
- PropertyStore.cs
- TypeElementCollection.cs
- RuntimeArgumentHandle.cs
- WasEndpointConfigContainer.cs
- XmlnsCompatibleWithAttribute.cs
- ReflectPropertyDescriptor.cs
- mactripleDES.cs
- TextParaLineResult.cs
- IImplicitResourceProvider.cs
- TraceContext.cs
- MaskedTextBoxDesignerActionList.cs
- CaseExpr.cs
- BasicExpressionVisitor.cs
- Operators.cs
- DataView.cs
- ProxyHwnd.cs
- MenuAdapter.cs
- DataContract.cs
- WindowsAuthenticationModule.cs
- SafeNativeMethods.cs
- ViewStateException.cs
- DataControlFieldHeaderCell.cs
- SystemColors.cs
- VolatileResourceManager.cs
- SmtpMail.cs
- IndexerNameAttribute.cs
- RadioButtonRenderer.cs
- XmlIlGenerator.cs
- TdsEnums.cs
- GridPattern.cs
- OutputCacheSettings.cs
- ContractComponent.cs
- ContentFilePart.cs
- DeviceFilterDictionary.cs
- SystemPens.cs
- OrthographicCamera.cs
- ArrayList.cs
- RadialGradientBrush.cs
- SourceSwitch.cs
- DetailsViewDeletedEventArgs.cs
- HitTestParameters.cs
- BindingNavigator.cs
- DataKeyArray.cs
- EraserBehavior.cs
- ClaimComparer.cs
- ProtocolsConfiguration.cs
- Camera.cs
- AnnotationAdorner.cs
- formatter.cs
- Root.cs