Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Imaging / ColorConvertedBitmap.cs / 1305600 / ColorConvertedBitmap.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation. All Rights Reserved. // // File: ColorConvertedBitmap.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { #region ColorConvertedBitmap ////// ColorConvertedBitmap provides caching functionality for a BitmapSource. /// public sealed partial class ColorConvertedBitmap : Imaging.BitmapSource, ISupportInitialize { ////// Constructor /// public ColorConvertedBitmap() : base(true) { } ////// Construct a ColorConvertedBitmap /// /// Input BitmapSource to color convert /// Source Color Context /// Destination Color Context /// Destination Pixel format public ColorConvertedBitmap(BitmapSource source, ColorContext sourceColorContext, ColorContext destinationColorContext, PixelFormat format) : base(true) // Use base class virtuals { if (source == null) { throw new ArgumentNullException("source"); } if (sourceColorContext == null) { throw new ArgumentNullException("sourceColorContext"); } if (destinationColorContext == null) { throw new ArgumentNullException("destinationColorContext"); } _bitmapInit.BeginInit(); Source = source; SourceColorContext = sourceColorContext; DestinationColorContext = destinationColorContext; DestinationFormat = format; _bitmapInit.EndInit(); FinalizeCreation(); } // ISupportInitialize ////// Prepare the bitmap to accept initialize paramters. /// public void BeginInit() { WritePreamble(); _bitmapInit.BeginInit(); } ////// Prepare the bitmap to accept initialize paramters. /// ////// Critical - access critical resources /// PublicOK - All inputs verified /// [SecurityCritical ] public void EndInit() { WritePreamble(); _bitmapInit.EndInit(); IsValidForFinalizeCreation(/* throwIfInvalid = */ true); FinalizeCreation(); } private void ClonePrequel(ColorConvertedBitmap otherColorConvertedBitmap) { BeginInit(); } private void ClonePostscript(ColorConvertedBitmap otherColorConvertedBitmap) { EndInit(); } /// /// Create the unmanaged resources /// ////// Critical - access critical resource /// [SecurityCritical] internal override void FinalizeCreation() { _bitmapInit.EnsureInitializedComplete(); BitmapSourceSafeMILHandle wicConverter = null; HRESULT.Check(UnsafeNativeMethods.WICCodec.CreateColorTransform( out wicConverter)); lock (_syncObject) { Guid fmtDestFmt = DestinationFormat.Guid; HRESULT.Check(UnsafeNativeMethods.WICColorTransform.Initialize( wicConverter, Source.WicSourceHandle, SourceColorContext.ColorContextHandle, DestinationColorContext.ColorContextHandle, ref fmtDestFmt)); } // // This is just a link in a BitmapSource chain. The memory is being used by // the BitmapSource at the end of the chain, so no memory pressure needs // to be added here. // WicSourceHandle = wicConverter; _isSourceCached = Source.IsSourceCached; CreationCompleted = true; UpdateCachedSettings(); } ////// Notification on source changing. /// private void SourcePropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { BitmapSource newSource = e.NewValue as BitmapSource; _source = newSource; RegisterDownloadEventSource(_source); _syncObject = (newSource != null) ? newSource.SyncObject : _bitmapInit; } } internal override bool IsValidForFinalizeCreation(bool throwIfInvalid) { if (Source == null) { if (throwIfInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_NoArgument, "Source")); } return false; } if (SourceColorContext == null) { if (throwIfInvalid) { throw new InvalidOperationException(SR.Get(SRID.Color_NullColorContext)); } return false; } if (DestinationColorContext == null) { if (throwIfInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_NoArgument, "DestinationColorContext")); } return false; } return true; } ////// Notification on source colorcontext changing. /// private void SourceColorContextPropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { _sourceColorContext = e.NewValue as ColorContext; } } ////// Notification on destination colorcontext changing. /// private void DestinationColorContextPropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { _destinationColorContext = e.NewValue as ColorContext; } } ////// Notification on destination format changing. /// private void DestinationFormatPropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { _destinationFormat = (PixelFormat)e.NewValue; } } ////// Coerce Source /// private static object CoerceSource(DependencyObject d, object value) { ColorConvertedBitmap bitmap = (ColorConvertedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._source; } else { return value; } } ////// Coerce SourceColorContext /// private static object CoerceSourceColorContext(DependencyObject d, object value) { ColorConvertedBitmap bitmap = (ColorConvertedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._sourceColorContext; } else { return value; } } ////// Coerce DestinationColorContext /// private static object CoerceDestinationColorContext(DependencyObject d, object value) { ColorConvertedBitmap bitmap = (ColorConvertedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._destinationColorContext; } else { return value; } } ////// Coerce DestinationFormat /// private static object CoerceDestinationFormat(DependencyObject d, object value) { ColorConvertedBitmap bitmap = (ColorConvertedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._destinationFormat; } else { return value; } } #region Data members private BitmapSource _source; private ColorContext _sourceColorContext; private ColorContext _destinationColorContext; private PixelFormat _destinationFormat; #endregion } #endregion // ColorConvertedBitmap } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
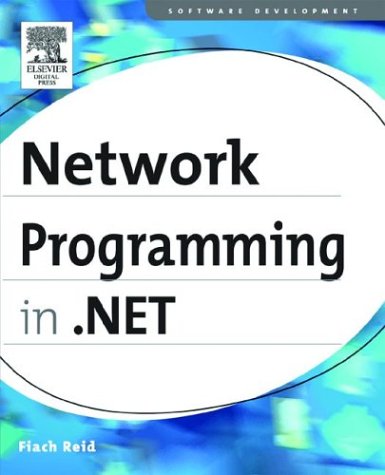
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OdbcConnectionFactory.cs
- MeshGeometry3D.cs
- XamlToRtfParser.cs
- WebEventTraceProvider.cs
- LambdaCompiler.cs
- ActiveXHost.cs
- ConfigurationStrings.cs
- PackageRelationshipCollection.cs
- SimpleWorkerRequest.cs
- TypeUtil.cs
- MSAAEventDispatcher.cs
- ImageListStreamer.cs
- XamlBrushSerializer.cs
- RegexNode.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- ServicePoint.cs
- Transactions.cs
- AggregateNode.cs
- Keyboard.cs
- PersonalizationStateInfoCollection.cs
- NamespaceCollection.cs
- WpfKnownTypeInvoker.cs
- FragmentNavigationEventArgs.cs
- PhysicalOps.cs
- LongValidatorAttribute.cs
- ToolStripLocationCancelEventArgs.cs
- FrameworkElement.cs
- TaskHelper.cs
- COM2ColorConverter.cs
- DashStyle.cs
- ContentFileHelper.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- SafeLibraryHandle.cs
- EditorZoneBase.cs
- DbReferenceCollection.cs
- DeclaredTypeValidatorAttribute.cs
- AutomationIdentifier.cs
- AdPostCacheSubstitution.cs
- LongPath.cs
- MouseEventArgs.cs
- _SingleItemRequestCache.cs
- ProcessProtocolHandler.cs
- ThumbAutomationPeer.cs
- EntitySqlQueryCacheKey.cs
- QuadraticEase.cs
- SamlAuthorizationDecisionClaimResource.cs
- BamlTreeNode.cs
- ConfigurationValidatorAttribute.cs
- SecurityChannel.cs
- URLIdentityPermission.cs
- DictionarySectionHandler.cs
- figurelength.cs
- AddInContractAttribute.cs
- TextLineBreak.cs
- OperatingSystem.cs
- ScriptIgnoreAttribute.cs
- AvtEvent.cs
- NativeMethods.cs
- ContainerSelectorActiveEvent.cs
- CodeGotoStatement.cs
- ReferencedType.cs
- BinaryNode.cs
- Button.cs
- TextComposition.cs
- CacheOutputQuery.cs
- CacheChildrenQuery.cs
- ListViewCancelEventArgs.cs
- InstanceKeyCompleteException.cs
- BindingsSection.cs
- KeyedCollection.cs
- EmptyEnumerator.cs
- TypeSchema.cs
- DispatchWrapper.cs
- ComEventsMethod.cs
- LeafCellTreeNode.cs
- WebPartConnectionsCancelEventArgs.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- mactripleDES.cs
- ObjectDataSourceMethodEventArgs.cs
- CompiledIdentityConstraint.cs
- PrintingPermissionAttribute.cs
- ProfessionalColors.cs
- XmlValidatingReader.cs
- RequestQueue.cs
- BidOverLoads.cs
- TreeNodeCollection.cs
- TraceContextEventArgs.cs
- ConfigurationManagerInternalFactory.cs
- ToolboxBitmapAttribute.cs
- WizardPanel.cs
- ComEventsMethod.cs
- AbstractDataSvcMapFileLoader.cs
- SqlClientMetaDataCollectionNames.cs
- MouseActionValueSerializer.cs
- IgnoreFileBuildProvider.cs
- Zone.cs
- CodeCatchClauseCollection.cs
- OracleInfoMessageEventArgs.cs
- CheckedPointers.cs
- List.cs