Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Imaging / PngBitmapEncoder.cs / 1305600 / PngBitmapEncoder.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All Rights Reserved // // File: PngBitmapEncoder.cs // //----------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; namespace System.Windows.Media.Imaging { #region PngInterlaceOption ////// Possibile options for the interlaced setting. /// public enum PngInterlaceOption : int { ////// Let the encoder decide what is best. /// Default = 0, ////// Save as an interlaced bitmap. /// On = 1, ////// Do not save as an interlaced bitmap. /// Off = 2, } #endregion #region PngBitmapEncoder ////// Built-in Encoder for Png files. /// public sealed class PngBitmapEncoder : BitmapEncoder { #region Constructors ////// Constructor for PngBitmapEncoder /// ////// Critical - will eventually create unmanaged resources /// PublicOK - all inputs are verified /// [SecurityCritical ] public PngBitmapEncoder() : base(true) { _supportsPreview = false; _supportsGlobalThumbnail = false; _supportsGlobalMetadata = false; _supportsFrameThumbnails = false; _supportsMultipleFrames = false; _supportsFrameMetadata = true; } #endregion #region Public Properties ////// Encode this bitmap as interlaced. /// public PngInterlaceOption Interlace { get { return _interlaceOption; } set { _interlaceOption = value; } } #endregion #region Internal Properties / Methods ////// Returns the container format for this encoder /// ////// Critical - uses guid to create unmanaged resources /// internal override Guid ContainerFormat { [SecurityCritical] get { return _containerFormat; } } ////// Setups the encoder and other properties before encoding each frame /// ////// Critical - calls Critical Initialize() /// [SecurityCritical] internal override void SetupFrame(SafeMILHandle frameEncodeHandle, SafeMILHandle encoderOptions) { PROPBAG2 propBag = new PROPBAG2(); PROPVARIANT propValue = new PROPVARIANT(); // There is only one encoder option supported here: if (_interlaceOption != c_defaultInterlaceOption) { try { propBag.Init("InterlaceOption"); propValue.Init(_interlaceOption == PngInterlaceOption.On); HRESULT.Check(UnsafeNativeMethods.IPropertyBag2.Write( encoderOptions, 1, ref propBag, ref propValue)); } finally { propBag.Clear(); propValue.Clear(); } } HRESULT.Check(UnsafeNativeMethods.WICBitmapFrameEncode.Initialize( frameEncodeHandle, encoderOptions )); } #endregion #region Internal Abstract /// Need to implement this to derive from the "sealed" object internal override void SealObject() { throw new NotImplementedException(); } #endregion #region Data Members ////// Critical - CLSID used for creation of critical resources /// [SecurityCritical] private Guid _containerFormat = MILGuidData.GUID_ContainerFormatPng; private const PngInterlaceOption c_defaultInterlaceOption = PngInterlaceOption.Default; private PngInterlaceOption _interlaceOption = c_defaultInterlaceOption; #endregion } #endregion // PngBitmapEncoder } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
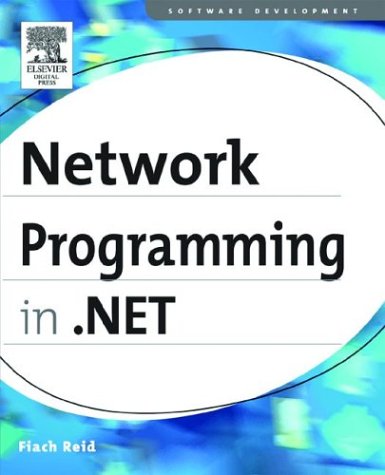
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextFormatterContext.cs
- BindingNavigator.cs
- MailAddressCollection.cs
- Transactions.cs
- XmlCompatibilityReader.cs
- WebHttpSecurity.cs
- EmbeddedMailObjectCollectionEditor.cs
- SimpleHandlerBuildProvider.cs
- ColumnWidthChangingEvent.cs
- LineSegment.cs
- If.cs
- PathFigure.cs
- DataGridItem.cs
- BitmapEffectState.cs
- JsonEnumDataContract.cs
- Parameter.cs
- ScrollEventArgs.cs
- TextFragmentEngine.cs
- WorkflowRuntimeServiceElementCollection.cs
- XmlBaseReader.cs
- TrackingProfile.cs
- ConnectionManager.cs
- ForwardPositionQuery.cs
- DrawingBrush.cs
- RoleGroup.cs
- NativeMethods.cs
- FileNotFoundException.cs
- AppSettingsExpressionBuilder.cs
- PromptEventArgs.cs
- Blend.cs
- MemoryStream.cs
- wmiprovider.cs
- AuthStoreRoleProvider.cs
- CursorConverter.cs
- XmlNamedNodeMap.cs
- ConnectionStringsExpressionEditor.cs
- sqlcontext.cs
- TextEditorSelection.cs
- XmlParserContext.cs
- Lasso.cs
- ProjectionCamera.cs
- LingerOption.cs
- ImpersonateTokenRef.cs
- DataGridViewHeaderCell.cs
- AccessKeyManager.cs
- XmlExceptionHelper.cs
- CollectionView.cs
- TrackingDataItemValue.cs
- ManualResetEvent.cs
- ToolTipAutomationPeer.cs
- FlowDocumentReaderAutomationPeer.cs
- SequentialOutput.cs
- Int32CollectionConverter.cs
- WeakReferenceEnumerator.cs
- SemaphoreFullException.cs
- DrawTreeNodeEventArgs.cs
- BCLDebug.cs
- ExpressionEditorSheet.cs
- UrlPath.cs
- SchemaImporterExtensionsSection.cs
- SudsParser.cs
- QueueProcessor.cs
- TransformCollection.cs
- OperationInvokerBehavior.cs
- UnsafeNativeMethods.cs
- ReadOnlyNameValueCollection.cs
- NamespaceQuery.cs
- ComplusTypeValidator.cs
- ItemsControlAutomationPeer.cs
- RegionIterator.cs
- RangeBase.cs
- StringFormat.cs
- NativeRightsManagementAPIsStructures.cs
- XmlWriterTraceListener.cs
- DelegateSerializationHolder.cs
- CacheAxisQuery.cs
- Point3DAnimationBase.cs
- QueryReaderSettings.cs
- ProtocolProfile.cs
- EventMappingSettingsCollection.cs
- MediaTimeline.cs
- VarRefManager.cs
- ZipIOFileItemStream.cs
- OdbcDataReader.cs
- KeyGesture.cs
- NavigationWindowAutomationPeer.cs
- Constraint.cs
- fixedPageContentExtractor.cs
- OpCodes.cs
- OneOf.cs
- VisualTreeHelper.cs
- XpsFilter.cs
- HtmlControlDesigner.cs
- MeshGeometry3D.cs
- TypedTableBase.cs
- QilChoice.cs
- InspectionWorker.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- PointHitTestParameters.cs
- CodeTypeReferenceCollection.cs