Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / FixedHyperLink.cs / 1305600 / FixedHyperLink.cs
//---------------------------------------------------------------------------- //// Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // // Description: // Implements the help class of FixedHyperLink. // // History: // 02/04/2005 - Ming Liu(MingLiu) - Created. // // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Windows.Threading; using System.Windows.Markup; using System.Windows.Navigation; using System.Windows.Media; ////// The IFixedNavigate interface will be implemented by FixedPage, FixedDocument, /// and FixedDocumentSequence to support fixed hyperlink. /// internal interface IFixedNavigate { ////// Find the element which given ID in this document context. /// /// The ID of UIElement to search for /// The fixedPage that contains returns UIElement ///UIElement FindElementByID(string elementID, out FixedPage rootFixedPage); /// /// Navigate to the element with ID= elementID /// /// void NavigateAsync (string elementID); } internal static class FixedHyperLink { ////// NavigationService property ChangedCallback. /// public static void OnNavigationServiceChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { FixedDocument fixedContent = d as FixedDocument; if (fixedContent != null) { NavigationService oldService = (NavigationService) e.OldValue; NavigationService newService = (NavigationService) e.NewValue; if (oldService != null) { oldService.FragmentNavigation -= new FragmentNavigationEventHandler(FragmentHandler); } if (newService != null) { newService.FragmentNavigation += new FragmentNavigationEventHandler(FragmentHandler); } } } ////// Called by NavigationService to let document content to handle the fragment first. /// /// /// internal static void FragmentHandler(object sender, FragmentNavigationEventArgs e) { NavigationService ns = sender as NavigationService; if (ns != null) { string fragment = e.Fragment; IFixedNavigate fixedNavigate = ns.Content as IFixedNavigate; if (fixedNavigate != null) { fixedNavigate.NavigateAsync(e.Fragment); e.Handled = true; } } } ////// Fire BringinToView event on the element ID. /// /// The host document of element ID, call any one implents IFixedNavigate /// internal static void NavigateToElement(object ElementHost, string elementID) { FixedPage rootFixedPage = null; FrameworkElement targetElement = null; targetElement = ((IFixedNavigate)ElementHost).FindElementByID(elementID, out rootFixedPage) as FrameworkElement; if (targetElement != null) { if (targetElement is FixedPage) { // // For fixedpage, we only need to scroll to page position. // targetElement.BringIntoView(); } else { //Just passing in raw rect of targetElement. Let DocumentViewer/Grid handle transforms targetElement.BringIntoView(targetElement.VisualContentBounds); } } return; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
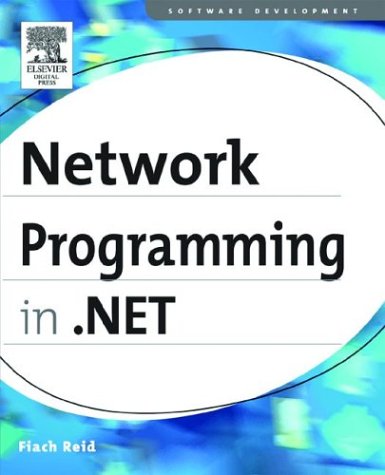
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SiteMapNodeItem.cs
- DataServiceOperationContext.cs
- oledbconnectionstring.cs
- UserInitiatedNavigationPermission.cs
- VectorAnimation.cs
- ColumnHeaderCollectionEditor.cs
- FontCollection.cs
- OracleDataReader.cs
- ByeMessageCD1.cs
- TableRow.cs
- DataObjectEventArgs.cs
- SettingsAttributeDictionary.cs
- Point3DKeyFrameCollection.cs
- FigureParaClient.cs
- Visual3DCollection.cs
- ConsoleTraceListener.cs
- ConfigurationElementProperty.cs
- StateManagedCollection.cs
- Messages.cs
- GeometryGroup.cs
- RuntimeHelpers.cs
- NullExtension.cs
- DesignOnlyAttribute.cs
- InputBinder.cs
- TextElementEditingBehaviorAttribute.cs
- DataPagerFieldCollection.cs
- ActivationServices.cs
- ListContractAdapter.cs
- ComponentDispatcherThread.cs
- CodeExporter.cs
- DecoratedNameAttribute.cs
- EncryptedXml.cs
- FileVersionInfo.cs
- MetaForeignKeyColumn.cs
- XomlCompilerError.cs
- _IPv6Address.cs
- DataSvcMapFile.cs
- UIElementPropertyUndoUnit.cs
- DispatcherEventArgs.cs
- Form.cs
- PerformanceCounterPermission.cs
- RequestValidator.cs
- TitleStyle.cs
- PenThreadWorker.cs
- OrderedEnumerableRowCollection.cs
- StrokeFIndices.cs
- BamlRecordWriter.cs
- CmsUtils.cs
- VirtualDirectoryMappingCollection.cs
- TransformConverter.cs
- XmlName.cs
- ReachFixedPageSerializerAsync.cs
- MemoryPressure.cs
- RequestSecurityTokenResponse.cs
- PeerService.cs
- __Filters.cs
- httpstaticobjectscollection.cs
- ByteConverter.cs
- InteropBitmapSource.cs
- BaseDataBoundControl.cs
- MemberHolder.cs
- JsonReaderWriterFactory.cs
- ClientSponsor.cs
- SQLDecimalStorage.cs
- HScrollProperties.cs
- ControlCollection.cs
- DrawingState.cs
- DispatchWrapper.cs
- TableLayoutCellPaintEventArgs.cs
- LocalizedNameDescriptionPair.cs
- IIS7WorkerRequest.cs
- KeyboardEventArgs.cs
- ContextProperty.cs
- DataBindingCollection.cs
- HttpHeaderCollection.cs
- WorkflowInlining.cs
- SystemKeyConverter.cs
- PerformanceCounterManager.cs
- JsonFormatGeneratorStatics.cs
- ExpressionEditorAttribute.cs
- OSEnvironmentHelper.cs
- ServiceNotStartedException.cs
- IQueryable.cs
- WebPartsSection.cs
- FormViewDeletedEventArgs.cs
- C14NUtil.cs
- StickyNoteContentControl.cs
- TdsValueSetter.cs
- FlowLayoutPanel.cs
- WindowsSolidBrush.cs
- ConsoleCancelEventArgs.cs
- ConfigXmlComment.cs
- StreamMarshaler.cs
- SurrogateSelector.cs
- MouseButton.cs
- EventLog.cs
- ResolveNameEventArgs.cs
- PolicyUnit.cs
- ServiceNotStartedException.cs
- WinFormsUtils.cs