Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Markup / ParserStack.cs / 1305600 / ParserStack.cs
/****************************************************************************\ * * File: ParserStack.cs * * Purpose: ParserStack...once was in XamlReaderHelper.cs * * History: * 11/21/08: [....] Moved from XamlReaderHelper.cs as we were replacing the parser. * * Copyright (C) 2008 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.Xml.Serialization; using System.IO; using System.Text; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Globalization; using MS.Utility; using System.Collections.Specialized; using Microsoft.Win32; using System.Runtime.InteropServices; using MS.Internal; using System.Windows.Markup; #if !PBTCOMPILER using System.Windows; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Shapes; #endif #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { ////// Parser Context stack /// The built-in Stack class doesn't let you do things like look at nodes beyond the top of the stack... /// so we'll use this instead. Note that this is not a complete stack implementation -- if you try to /// convert to array or some such, you'll be missing a couple elements. But this is plenty for our purposes. /// Main goal is to track current/parent context with a minimum of overhead. /// This class is internal so it can be shared with the BamlRecordReader /// internal class ParserStack : ArrayList { ////// Creates a default ParserStack /// internal ParserStack() : base() { } ////// Creates a clone. /// private ParserStack(ICollection collection) : base(collection) { } #region StackOverrides public void Push(object o) { Add(o); } public object Pop() { object o = this[Count - 1]; RemoveAt(Count - 1); return o; } #if !PBTCOMPILER public object Peek() { // Die if peeking on empty stack return this[Count - 1]; } #endif public override object Clone() { return new ParserStack(this); } #endregion // StackOverrides #region Properties ////// Returns the Current Context on the stack /// internal object CurrentContext { get { return Count > 0 ? this[Count - 1] : null; } } ////// Returns the Parent of the Current Context /// internal object ParentContext { get { return Count > 1 ? this[Count - 2] : null; } } ////// Returns the GrandParent of the Current Context /// internal object GrandParentContext { get { return Count > 2 ? this[Count - 3] : null; } } #if !PBTCOMPILER ////// Returns the GreatGrandParent of the Current Context /// internal object GreatGrandParentContext { get { return Count > 3 ? this[Count - 4] : null; } } #endif #endregion // Properties } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
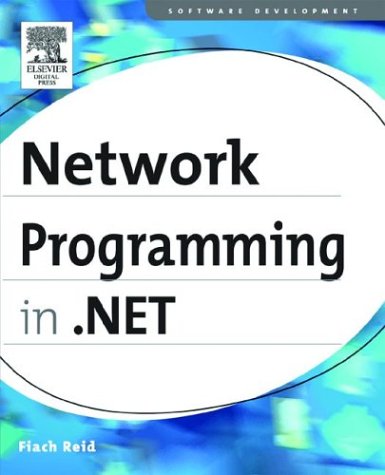
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ErrorRuntimeConfig.cs
- BulletDecorator.cs
- AspNetHostingPermission.cs
- ElasticEase.cs
- Certificate.cs
- SqlRewriteScalarSubqueries.cs
- ScriptRef.cs
- WindowsListViewItemCheckBox.cs
- TransformBlockRequest.cs
- IUnknownConstantAttribute.cs
- AdPostCacheSubstitution.cs
- CorrelationRequestContext.cs
- DataFormats.cs
- ToolBarButton.cs
- RequestSecurityToken.cs
- ToolZone.cs
- AesManaged.cs
- ParseHttpDate.cs
- FrameworkObject.cs
- CodeMemberProperty.cs
- LogReserveAndAppendState.cs
- XmlDocumentFragment.cs
- SubclassTypeValidatorAttribute.cs
- KeyConverter.cs
- CompositeCollectionView.cs
- SiteMap.cs
- ListViewGroupItemCollection.cs
- HyperLinkStyle.cs
- BevelBitmapEffect.cs
- SortKey.cs
- DataGridViewHitTestInfo.cs
- Int16KeyFrameCollection.cs
- ProcessThread.cs
- SecurityDescriptor.cs
- ProcessManager.cs
- DrawingAttributes.cs
- ItemCheckedEvent.cs
- LocatorManager.cs
- HttpDictionary.cs
- LocatorBase.cs
- IIS7UserPrincipal.cs
- ActivityCodeDomReferenceService.cs
- SamlDelegatingWriter.cs
- Compiler.cs
- SecurityTokenAttachmentMode.cs
- XmlNodeWriter.cs
- SimpleMailWebEventProvider.cs
- TextElementAutomationPeer.cs
- ParenthesizePropertyNameAttribute.cs
- EventLogEntryCollection.cs
- _TLSstream.cs
- DocumentGrid.cs
- TypeResolvingOptionsAttribute.cs
- InstalledFontCollection.cs
- ContentValidator.cs
- PeerService.cs
- CollectionEditor.cs
- Inflater.cs
- EntitySqlQueryCacheEntry.cs
- Win32Exception.cs
- DocumentViewerBaseAutomationPeer.cs
- Relationship.cs
- XMLDiffLoader.cs
- ConfigurationErrorsException.cs
- UmAlQuraCalendar.cs
- ControlsConfig.cs
- XmlElementCollection.cs
- DataTableReader.cs
- ButtonBase.cs
- Vector3DKeyFrameCollection.cs
- ISAPIApplicationHost.cs
- DefaultTextStoreTextComposition.cs
- TextEndOfSegment.cs
- ItemsPanelTemplate.cs
- ObjectContextServiceProvider.cs
- Variable.cs
- SchemaManager.cs
- AssemblyAttributes.cs
- XsltArgumentList.cs
- precedingsibling.cs
- UserControlBuildProvider.cs
- PropertyPathConverter.cs
- FileSystemEventArgs.cs
- Attributes.cs
- MatrixConverter.cs
- WebPartConnectionsConfigureVerb.cs
- StopStoryboard.cs
- ColorKeyFrameCollection.cs
- VisualStateGroup.cs
- Pair.cs
- IdentityHolder.cs
- RegexWriter.cs
- TextTreeObjectNode.cs
- WebPartZone.cs
- AudioException.cs
- TableLayoutSettingsTypeConverter.cs
- Listbox.cs
- sqlinternaltransaction.cs
- ClientSettingsProvider.cs
- ProfilePropertyNameValidator.cs