Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Input / Command / KeyBinding.cs / 1 / KeyBinding.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The KeyBinding class is used by the developer to create Keyboard Input Bindings // // See spec at : [....]/coreui/Specs/Commanding(new).mht // //* KeyBinding class serves the purpose of Input Bindings for Keyboard Device. // // History: // 06/01/2003 : [....] - Created // 05/01/2004 : chandra - changed to accommodate new design // ( [....]/coreui/Specs/Commanding(new).mht ) //--------------------------------------------------------------------------- using System; using System.Windows.Input; using System.Windows; using System.ComponentModel; using System.Windows.Markup; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// KeyBinding - Implements InputBinding (generic InputGesture-Command map) /// KeyBinding acts like a map for KeyGesture and Commands. /// Most of the logic is in InputBinding and KeyGesture, this only /// facilitates user to add Key/Modifiers directly without going in /// KeyGesture path. Also it provides the KeyGestureTypeConverter /// on the Gesture property to have KeyGesture, like Ctrl+X, Alt+V /// defined in Markup as Gesture="Ctrl+X" working /// public class KeyBinding : InputBinding { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructor ////// Constructor /// public KeyBinding() : base() { } ////// Constructor /// /// Command associated /// KeyGesture associated public KeyBinding(ICommand command, KeyGesture gesture) : base(command, gesture) { } ////// Constructor /// /// /// modifiers /// key public KeyBinding(ICommand command, Key key, ModifierKeys modifiers) : base(command, new KeyGesture(key, modifiers)) { } #endregion Constructor //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// KeyGesture Override, to ensure type-safety and provide a /// TypeConverter for KeyGesture /// [TypeConverter(typeof(KeyGestureConverter))] [ValueSerializer(typeof(KeyGestureValueSerializer))] public override InputGesture Gesture { get { return base.Gesture as KeyGesture; } set { if (value is KeyGesture) { base.Gesture = value; } else { throw new ArgumentException(SR.Get(SRID.InputBinding_ExpectedInputGesture, typeof(KeyGesture))); } } } ////// Modifier /// public ModifierKeys Modifiers { get { lock (_dataLock) { if (null != Gesture) { return ((KeyGesture)Gesture).Modifiers; } return ModifierKeys.None; } } set { lock (_dataLock) { if (null == Gesture) { Gesture = new KeyGesture(Key.None, (ModifierKeys)value, /*validateGesture = */ false); } else { Gesture = new KeyGesture(((KeyGesture)Gesture).Key, value, /*validateGesture = */ false); } } } } ////// Key /// public Key Key { get { lock (_dataLock) { if (null != Gesture) { return ((KeyGesture)Gesture).Key; } return Key.None; } } set { lock (_dataLock) { if (null == Gesture) { Gesture = new KeyGesture((Key)value, ModifierKeys.None, /*validateGesture = */ false); } else { Gesture = new KeyGesture(value, ((KeyGesture)Gesture).Modifiers, /*validateGesture = */ false); } } } } #endregion Public Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
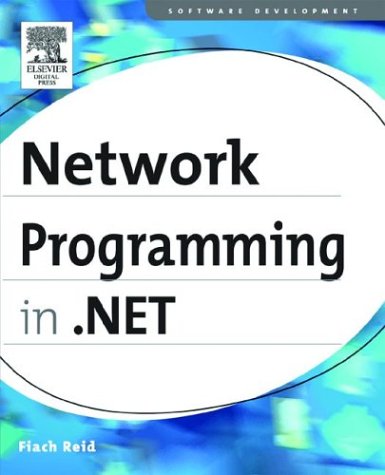
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbConnectionOptions.cs
- UIElement3D.cs
- DesignerDataRelationship.cs
- HierarchicalDataTemplate.cs
- XmlUTF8TextReader.cs
- ChangePasswordAutoFormat.cs
- DiscoveryDocument.cs
- ProtocolsConfiguration.cs
- TreeViewCancelEvent.cs
- StructuralType.cs
- InputManager.cs
- BitmapVisualManager.cs
- AssociationSet.cs
- XPathException.cs
- RequiredAttributeAttribute.cs
- KoreanCalendar.cs
- panel.cs
- ObjectViewFactory.cs
- TreeNodeClickEventArgs.cs
- ModuleBuilder.cs
- login.cs
- AnnotationAdorner.cs
- HelloMessage11.cs
- MessagePropertyVariants.cs
- ParserContext.cs
- FileLevelControlBuilderAttribute.cs
- WebPartDisplayModeEventArgs.cs
- MethodAccessException.cs
- HttpWebRequestElement.cs
- DataGridAutomationPeer.cs
- VBCodeProvider.cs
- MonthChangedEventArgs.cs
- WasHttpModulesInstallComponent.cs
- GuidConverter.cs
- GenerateHelper.cs
- ProviderSettingsCollection.cs
- DataBoundControl.cs
- Comparer.cs
- SqlReferenceCollection.cs
- COSERVERINFO.cs
- XmlLangPropertyAttribute.cs
- BindingMemberInfo.cs
- HttpConfigurationSystem.cs
- MemberAccessException.cs
- ActiveXHost.cs
- Win32Exception.cs
- StylusDownEventArgs.cs
- InputReferenceExpression.cs
- MultiDataTrigger.cs
- WebSysDefaultValueAttribute.cs
- RouteParameter.cs
- TCEAdapterGenerator.cs
- ComEventsInfo.cs
- RenderingEventArgs.cs
- TableLayout.cs
- ExportOptions.cs
- HtmlString.cs
- ValueTable.cs
- WebBrowser.cs
- SqlInternalConnection.cs
- SqlUtil.cs
- WebAdminConfigurationHelper.cs
- XmlWriterDelegator.cs
- DataGridViewUtilities.cs
- HTTPNotFoundHandler.cs
- LocalClientSecuritySettings.cs
- DbProviderManifest.cs
- XmlLinkedNode.cs
- VirtualPath.cs
- BaseComponentEditor.cs
- NeutralResourcesLanguageAttribute.cs
- PropertiesTab.cs
- DetailsViewCommandEventArgs.cs
- PageParser.cs
- TableAdapterManagerGenerator.cs
- SAPICategories.cs
- DocobjHost.cs
- SharedPersonalizationStateInfo.cs
- TextChange.cs
- WebBrowserNavigatingEventHandler.cs
- DynamicResourceExtensionConverter.cs
- DomNameTable.cs
- TextEffectResolver.cs
- MailAddressCollection.cs
- X509ServiceCertificateAuthentication.cs
- EntityDataSourceEntitySetNameItem.cs
- PocoEntityKeyStrategy.cs
- ResizeGrip.cs
- GraphicsPath.cs
- IDispatchConstantAttribute.cs
- GenericEnumerator.cs
- ToolTipAutomationPeer.cs
- ReaderWriterLockWrapper.cs
- PaintValueEventArgs.cs
- SqlUtils.cs
- AnnotationHighlightLayer.cs
- TaskHelper.cs
- UrlAuthFailedErrorFormatter.cs
- VariantWrapper.cs
- Point3DCollection.cs