Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Animation / ParallelTimeline.cs / 1 / ParallelTimeline.cs
//---------------------------------------------------------------------------- //// Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.ComponentModel; namespace System.Windows.Media.Animation { ////// This class represents a group of Timelines where the children /// become active according to the value of their Begin property rather /// than their specific order in the Children collection. Children /// are also able to overlap and run in parallel with each other. /// public partial class ParallelTimeline : TimelineGroup { #region Constructors ////// Creates a ParallelTimeline with default properties. /// public ParallelTimeline() : base() { } ////// Creates a ParallelTimeline with the specified BeginTime. /// /// /// The scheduled BeginTime for this ParallelTimeline. /// public ParallelTimeline(TimeSpan? beginTime) : base(beginTime) { } ////// Creates a ParallelTimeline with the specified begin time and duration. /// /// /// The scheduled BeginTime for this ParallelTimeline. /// /// /// The simple Duration of this ParallelTimeline. /// public ParallelTimeline(TimeSpan? beginTime, Duration duration) : base(beginTime, duration) { } ////// Creates a ParallelTimeline with the specified BeginTime, Duration and RepeatBehavior. /// /// /// The scheduled BeginTime for this ParallelTimeline. /// /// /// The simple Duration of this ParallelTimeline. /// /// /// The RepeatBehavior for this ParallelTimeline. /// public ParallelTimeline(TimeSpan? beginTime, Duration duration, RepeatBehavior repeatBehavior) : base(beginTime, duration, repeatBehavior) { } #endregion #region Methods ////// Return the duration from a specific clock /// /// /// The Clock whose natural duration is desired. /// ////// A Duration quantity representing the natural duration. /// protected override Duration GetNaturalDurationCore(Clock clock) { Duration simpleDuration = TimeSpan.Zero; ClockGroup clockGroup = clock as ClockGroup; if (clockGroup != null) { Listchildren = clockGroup.InternalChildren; // The container ends when all of its children have ended at least // one of their active periods. if (children != null) { bool hasChildWithUnresolvedDuration = false; for (int childIndex = 0; childIndex < children.Count; childIndex++) { Duration childEndOfActivePeriod = children[childIndex].EndOfActivePeriod; if (childEndOfActivePeriod == Duration.Forever) { // If we have even one child with a duration of forever // our resolved duration will also be forever. It doesn't // matter if other children have unresolved durations. return Duration.Forever; } else if (childEndOfActivePeriod == Duration.Automatic) { hasChildWithUnresolvedDuration = true; } else if (childEndOfActivePeriod > simpleDuration) { simpleDuration = childEndOfActivePeriod; } } // We've iterated through all our children. We know that at this // point none of them have a duration of Forever or we would have // returned already. If any of them still have unresolved // durations then our duration is also still unresolved and we // will return automatic. Otherwise, we'll fall out of the 'if' // block and return the simpleDuration as our final resolved // duration. if (hasChildWithUnresolvedDuration) { return Duration.Automatic; } } } return simpleDuration; } #endregion #region SlipBehavior Property /// /// SlipBehavior Property /// public static readonly DependencyProperty SlipBehaviorProperty = DependencyProperty.Register( "SlipBehavior", typeof(SlipBehavior), typeof(ParallelTimeline), new PropertyMetadata( SlipBehavior.Grow, new PropertyChangedCallback(ParallelTimeline_PropertyChangedFunction)), new ValidateValueCallback(ValidateSlipBehavior)); private static bool ValidateSlipBehavior(object value) { return TimeEnumHelper.IsValidSlipBehavior((SlipBehavior)value); } ////// Returns the SlipBehavior for this ClockGroup /// [DefaultValue(SlipBehavior.Grow)] public SlipBehavior SlipBehavior { get { return (SlipBehavior)GetValue(SlipBehaviorProperty); } set { SetValue(SlipBehaviorProperty, value); } } internal static void ParallelTimeline_PropertyChangedFunction(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((ParallelTimeline)d).PropertyChanged(e.Property); } #endregion // SlipBehavior Property } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
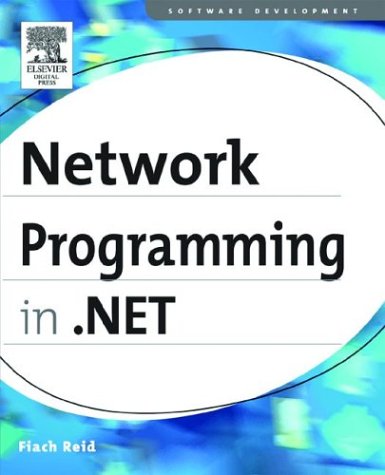
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HTTP_SERVICE_CONFIG_URLACL_KEY.cs
- Track.cs
- TimeSpanSecondsConverter.cs
- SchemaTypeEmitter.cs
- ControlBuilderAttribute.cs
- JavaScriptObjectDeserializer.cs
- GlyphTypeface.cs
- ZoomPercentageConverter.cs
- ControlBuilder.cs
- Overlapped.cs
- LinqDataSourceValidationException.cs
- SingleConverter.cs
- AttributeUsageAttribute.cs
- XmlAttributeHolder.cs
- Enumerable.cs
- Switch.cs
- EnumConverter.cs
- ChannelManager.cs
- MetadataArtifactLoaderResource.cs
- SessionEndingCancelEventArgs.cs
- GeometryModel3D.cs
- PolicyStatement.cs
- _ListenerResponseStream.cs
- XPathItem.cs
- AttachmentService.cs
- RSAPKCS1KeyExchangeFormatter.cs
- ConnectionsZone.cs
- SqlBulkCopyColumnMappingCollection.cs
- arc.cs
- TextServicesDisplayAttribute.cs
- ServiceObjectContainer.cs
- CompiledRegexRunnerFactory.cs
- QueryableDataSource.cs
- CompilationUnit.cs
- DataContractSerializer.cs
- BuildProvidersCompiler.cs
- ToolBarButtonDesigner.cs
- MemberProjectionIndex.cs
- SinglePageViewer.cs
- EntityDataSourceWizardForm.cs
- PassportAuthenticationModule.cs
- JsonStringDataContract.cs
- TimeSpan.cs
- DataGridViewRowStateChangedEventArgs.cs
- DesignerActionUIService.cs
- ZipIOExtraFieldZip64Element.cs
- QilXmlWriter.cs
- LocalizabilityAttribute.cs
- _BufferOffsetSize.cs
- WebBodyFormatMessageProperty.cs
- PropertyPushdownHelper.cs
- HtmlAnchor.cs
- EventLogInformation.cs
- ZipIOExtraFieldPaddingElement.cs
- Bidi.cs
- PathNode.cs
- DataTable.cs
- PropertyGrid.cs
- BatchParser.cs
- PathSegment.cs
- RealProxy.cs
- ManagementOperationWatcher.cs
- _NegoStream.cs
- ScrollableControl.cs
- VerticalAlignConverter.cs
- UIPermission.cs
- EventLogQuery.cs
- FlowNode.cs
- PerspectiveCamera.cs
- TemplateControl.cs
- ElementAction.cs
- AbsoluteQuery.cs
- CacheDependency.cs
- XmlHierarchicalEnumerable.cs
- CallContext.cs
- ConstraintConverter.cs
- DropDownList.cs
- BitmapEffectGroup.cs
- ThreadAttributes.cs
- LinkUtilities.cs
- SqlInternalConnection.cs
- AccessibleObject.cs
- ItemAutomationPeer.cs
- GrammarBuilderDictation.cs
- SamlNameIdentifierClaimResource.cs
- UnauthorizedWebPart.cs
- ShaderEffect.cs
- DataGridViewSortCompareEventArgs.cs
- ControlTemplate.cs
- OutputCacheSettingsSection.cs
- RunWorkerCompletedEventArgs.cs
- Thumb.cs
- IndentedTextWriter.cs
- TagPrefixAttribute.cs
- StorageComplexPropertyMapping.cs
- FrameworkContentElementAutomationPeer.cs
- FeatureSupport.cs
- EntityWithKeyStrategy.cs
- AutomationPatternInfo.cs
- Stack.cs