Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Imaging / CroppedBitmap.cs / 1 / CroppedBitmap.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation. All Rights Reserved. // // File: CroppedBitmap.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { #region CroppedBitmap ////// CroppedBitmap provides caching functionality for a BitmapSource. /// public sealed partial class CroppedBitmap : Imaging.BitmapSource, ISupportInitialize { ////// Constructor /// public CroppedBitmap() : base(true) { } ////// Construct a CroppedBitmap /// /// BitmapSource to apply to the crop to /// Source rect of the bitmap to use public CroppedBitmap(BitmapSource source, Int32Rect sourceRect) : base(true) // Use base class virtuals { if (source == null) { throw new ArgumentNullException("source"); } _bitmapInit.BeginInit(); Source = source; SourceRect = sourceRect; _bitmapInit.EndInit(); FinalizeCreation(); } // ISupportInitialize ////// Prepare the bitmap to accept initialize paramters. /// public void BeginInit() { WritePreamble(); _bitmapInit.BeginInit(); } ////// Prepare the bitmap to accept initialize paramters. /// ////// Critical - access critical resources /// PublicOK - All inputs verified /// [SecurityCritical ] public void EndInit() { WritePreamble(); _bitmapInit.EndInit(); if (Source == null) { throw new InvalidOperationException(SR.Get(SRID.Image_NoArgument, "Source")); } FinalizeCreation(); } private void ClonePrequel(CroppedBitmap otherCroppedBitmap) { BeginInit(); } private void ClonePostscript(CroppedBitmap otherCroppedBitmap) { EndInit(); } /// /// Create the unmanaged resources /// ////// Critical - access critical resource /// [SecurityCritical] internal override void FinalizeCreation() { _bitmapInit.EnsureInitializedComplete(); BitmapSourceSafeMILHandle wicClipper = null; Int32Rect rect = SourceRect; BitmapSource source = Source; if (rect.IsEmpty) { rect.Width = source.PixelWidth; rect.Height = source.PixelHeight; } using (FactoryMaker factoryMaker = new FactoryMaker()) { try { IntPtr wicFactory = factoryMaker.ImagingFactoryPtr; HRESULT.Check(UnsafeNativeMethods.WICImagingFactory.CreateBitmapClipper( wicFactory, out wicClipper)); lock (_syncObject) { HRESULT.Check(UnsafeNativeMethods.WICBitmapClipper.Initialize( wicClipper, source.WicSourceHandle, ref rect)); } WicSourceHandle = wicClipper; _isSourceCached = source.IsSourceCached; wicClipper = null; } catch { _bitmapInit.Reset(); throw; } finally { if (wicClipper != null) wicClipper.Close(); } } CreationCompleted = true; UpdateCachedSettings(); } ////// Notification on source changing. /// private void SourcePropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { BitmapSource newSource = e.NewValue as BitmapSource; _source = newSource; _syncObject = (newSource != null) ? newSource.SyncObject : _bitmapInit; } } ////// Notification on source rect changing. /// private void SourceRectPropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { _sourceRect = (Int32Rect)e.NewValue; } } ////// Coerce Source /// private static object CoerceSource(DependencyObject d, object value) { CroppedBitmap bitmap = (CroppedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._source; } else { return value; } } ////// Coerce SourceRect /// private static object CoerceSourceRect(DependencyObject d, object value) { CroppedBitmap bitmap = (CroppedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._sourceRect; } else { return value; } } #region Data members private BitmapSource _source; private Int32Rect _sourceRect; #endregion } #endregion // CroppedBitmap } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
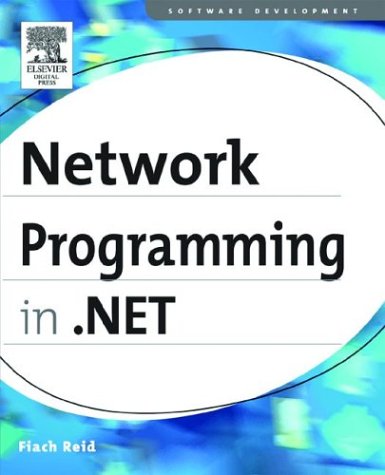
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsGraphics2.cs
- SslStream.cs
- categoryentry.cs
- MetabaseServerConfig.cs
- TreeNodeStyleCollection.cs
- ScrollItemPattern.cs
- UserControl.cs
- BitmapSizeOptions.cs
- SourceItem.cs
- x509store.cs
- IncrementalCompileAnalyzer.cs
- MissingMemberException.cs
- ColumnMapProcessor.cs
- ConfigurationPropertyAttribute.cs
- XmlSubtreeReader.cs
- XPathScanner.cs
- StandardOleMarshalObject.cs
- SafeFileMappingHandle.cs
- Nullable.cs
- RegistryPermission.cs
- TextMarkerSource.cs
- CollectionBuilder.cs
- UnauthorizedWebPart.cs
- WebFormsRootDesigner.cs
- DataSourceConverter.cs
- FlowDocumentScrollViewer.cs
- NextPreviousPagerField.cs
- ServiceOperationParameter.cs
- DbParameterCollectionHelper.cs
- InspectionWorker.cs
- IPPacketInformation.cs
- BooleanAnimationBase.cs
- ResetableIterator.cs
- BufferModeSettings.cs
- InkCanvas.cs
- KeyedHashAlgorithm.cs
- TextBox.cs
- Calendar.cs
- FilterableData.cs
- SchemaObjectWriter.cs
- ClientUIRequest.cs
- VisualTreeUtils.cs
- CodeDefaultValueExpression.cs
- ThreadExceptionDialog.cs
- SelectionGlyph.cs
- HTMLTagNameToTypeMapper.cs
- ResourceExpressionBuilder.cs
- DataGridClipboardCellContent.cs
- WebPartUtil.cs
- MobileControlDesigner.cs
- MenuDesigner.cs
- ListViewUpdatedEventArgs.cs
- ShapingEngine.cs
- BufferManager.cs
- CopyOfAction.cs
- ConstructorExpr.cs
- Line.cs
- DataGridViewCellPaintingEventArgs.cs
- TabControlAutomationPeer.cs
- StylusPointProperty.cs
- Transform3DGroup.cs
- ZipIOExtraField.cs
- LeaseManager.cs
- AffineTransform3D.cs
- WebScriptServiceHost.cs
- ListItemCollection.cs
- EmptyImpersonationContext.cs
- ExceptionDetail.cs
- HttpNamespaceReservationInstallComponent.cs
- CompilationLock.cs
- MDIWindowDialog.cs
- OdbcStatementHandle.cs
- MessageDispatch.cs
- MetadataArtifactLoaderCompositeFile.cs
- AssemblyAttributes.cs
- BaseValidator.cs
- RegisteredArrayDeclaration.cs
- QueryFunctions.cs
- CopyAttributesAction.cs
- BindingList.cs
- PolyLineSegmentFigureLogic.cs
- EntitySet.cs
- ResizeGrip.cs
- CatalogZoneBase.cs
- XmlnsDefinitionAttribute.cs
- PersistenceProviderFactory.cs
- Baml2006Reader.cs
- DataGridState.cs
- PhonemeEventArgs.cs
- AggregateNode.cs
- Rectangle.cs
- WebCodeGenerator.cs
- RegisteredHiddenField.cs
- WindowsFormsHostAutomationPeer.cs
- DispatcherHookEventArgs.cs
- EmbeddedObject.cs
- RuleElement.cs
- Int32EqualityComparer.cs
- ListViewItemSelectionChangedEvent.cs
- AutoCompleteStringCollection.cs