Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / Globalization / BamlResourceSerializer.cs / 1 / BamlResourceSerializer.cs
//-------------------------------------------------------- // Class that implements BamlResourceSerializer // // Created: [....] @ 12/1/2003 // //------------------------------------------------------- using System; using System.IO; using System.Globalization; using System.Runtime.InteropServices; using System.Collections; using System.Collections.Generic; using System.Windows.Markup; using System.Windows.Markup.Localizer; using System.Diagnostics; using System.Text; using System.Windows; namespace MS.Internal.Globalization { ////// BamlResourceSerializer /// internal sealed class BamlResourceSerializer { //------------------------------- // Internal static //------------------------------- internal static void Serialize(BamlLocalizer localizer, BamlTree tree, Stream output) { // Thread safe implementation (new BamlResourceSerializer()).SerializeImp(localizer, tree, output); } //---------------------------------- // constructor. //---------------------------------- ////// constructor /// private BamlResourceSerializer() { } //---------------------------------- // private method //---------------------------------- ////// Serialize the tree out to the stream. /// private void SerializeImp( BamlLocalizer localizer, BamlTree tree, Stream output ) { Debug.Assert(output != null, "The output stream given is null"); Debug.Assert(tree != null && tree.Root != null, "The tree to be serialized is null."); _writer = new BamlWriter(output); _bamlTreeStack = new Stack(); // intialize the stack. _bamlTreeStack.Push(tree.Root); while (_bamlTreeStack.Count > 0) { BamlTreeNode currentNode = _bamlTreeStack.Pop(); if (!currentNode.Visited) { // Mark this node so that it won't be serialized again. currentNode.Visited = true; currentNode.Serialize(_writer); PushChildrenToStack(currentNode.Children); } else { BamlStartElementNode elementNode = currentNode as BamlStartElementNode; Debug.Assert(elementNode != null); if (elementNode != null) { localizer.RaiseErrorNotifyEvent( new BamlLocalizerErrorNotifyEventArgs( BamlTreeMap.GetKey(elementNode), BamlLocalizerError.DuplicateElement ) ); } } } // do not close stream as we don't own it. } private void PushChildrenToStack(List children) { if (children == null) return; for (int i = children.Count - 1; i >= 0; i--) { _bamlTreeStack.Push(children[i]); } } //--------------------------------- // private //--------------------------------- private BamlWriter _writer; private Stack _bamlTreeStack; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
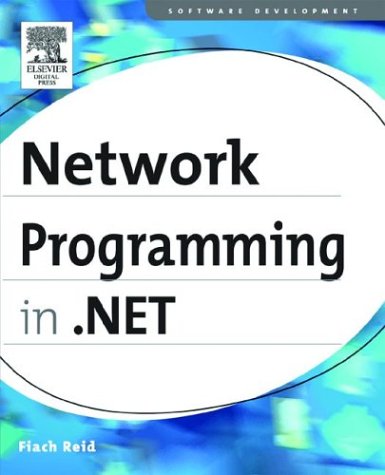
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlHelper.cs
- TableHeaderCell.cs
- FixedDocumentSequencePaginator.cs
- PreservationFileWriter.cs
- TextBoxDesigner.cs
- XmlException.cs
- DataObjectMethodAttribute.cs
- PackageProperties.cs
- ExpressionBinding.cs
- ExceptionUtil.cs
- DebuggerAttributes.cs
- X509Utils.cs
- InstalledFontCollection.cs
- ControlAdapter.cs
- FileSecurity.cs
- PublisherMembershipCondition.cs
- SqlConnectionString.cs
- EventSetter.cs
- Variable.cs
- QuadraticBezierSegment.cs
- BufferAllocator.cs
- XmlSchemaSubstitutionGroup.cs
- Calendar.cs
- CatalogZoneBase.cs
- SharedConnectionInfo.cs
- ReadOnlyDictionary.cs
- Renderer.cs
- OdbcConnectionString.cs
- TemplateControlParser.cs
- FilterableData.cs
- RulePatternOps.cs
- Misc.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- ToolStripContentPanelDesigner.cs
- AsymmetricAlgorithm.cs
- PropertyTab.cs
- LazyTextWriterCreator.cs
- EventWaitHandleSecurity.cs
- RuntimeConfigurationRecord.cs
- GraphicsState.cs
- ProfessionalColorTable.cs
- HttpPostProtocolReflector.cs
- MessageBox.cs
- EntityParameterCollection.cs
- AttributeXamlType.cs
- XmlNavigatorStack.cs
- RemoveStoryboard.cs
- CurrencyWrapper.cs
- TextMessageEncoder.cs
- SourceLineInfo.cs
- BitmapImage.cs
- DocumentEventArgs.cs
- UriParserTemplates.cs
- CompositeActivityTypeDescriptorProvider.cs
- CqlErrorHelper.cs
- HttpListenerContext.cs
- XPathMultyIterator.cs
- TextEndOfLine.cs
- ParameterModifier.cs
- ScriptResourceDefinition.cs
- OdbcHandle.cs
- ThumbAutomationPeer.cs
- ClientTargetCollection.cs
- StrokeIntersection.cs
- FormViewCommandEventArgs.cs
- ItemContainerGenerator.cs
- QuarticEase.cs
- DispatcherProcessingDisabled.cs
- Italic.cs
- HttpVersion.cs
- TextEffect.cs
- KeyedCollection.cs
- GradientBrush.cs
- Point4DConverter.cs
- CommandHelper.cs
- SchemaAttDef.cs
- AvTraceFormat.cs
- BuildResultCache.cs
- IndentedWriter.cs
- IteratorDescriptor.cs
- TypeConstant.cs
- LassoSelectionBehavior.cs
- SHA1CryptoServiceProvider.cs
- AutoResizedEvent.cs
- regiisutil.cs
- DllNotFoundException.cs
- GPRECTF.cs
- CompModSwitches.cs
- TrackingStringDictionary.cs
- CompositeDataBoundControl.cs
- BoundColumn.cs
- StrokeNodeOperations.cs
- XmlSchemaFacet.cs
- SortKey.cs
- SmtpClient.cs
- DeclarativeCatalogPartDesigner.cs
- XmlNodeChangedEventArgs.cs
- SimpleMailWebEventProvider.cs
- UpDownEvent.cs
- ComboBoxItem.cs