Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / PrintConfig / PrtCap_Base.cs / 1 / PrtCap_Base.cs
/*++ Copyright (C) 2003 Microsoft Corporation All rights reserved. Module Name: PrtCap_Base.cs Abstract: Definition and implementation of PrintCapabilities base types. Author: [....] ([....]) 6/30/2003 --*/ using System; using System.IO; using System.Xml; using System.Collections; using System.Diagnostics; using System.Runtime.InteropServices; using System.Printing; using MS.Internal.Printing.Configuration; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages namespace MS.Internal.Printing.Configuration { ////// /// Do not use. /// Abstract base class of abstract internal class PrintCapabilityFeature { #region Constructors ///feature. /// /// Constructs a new instance of the PrintCapabilityFeature class. /// /// Theobject this feature belongs to. protected PrintCapabilityFeature(InternalPrintCapabilities ownerPrintCap) { this.OwnerPrintCap = ownerPrintCap; } #endregion Constructors #region Internal Methods // derived types must implement following abstract methods /// /// Standard PrintCapabilities feature add-new-option callback. /// internal abstract bool AddOptionCallback(PrintCapabilityOption option); ////// Standard PrintCapabilities feature add-sub-feature callback. /// internal abstract void AddSubFeatureCallback(PrintCapabilityFeature subFeature); ////// Standard PrintCapabilities feature feature-property calllback. /// internal abstract bool FeaturePropCallback(PrintCapabilityFeature feature, XmlPrintCapReader reader); ////// Standard PrintCapabilities feature new-option callback. /// internal abstract PrintCapabilityOption NewOptionCallback(PrintCapabilityFeature baseFeature); ////// Standard PrintCapabilities feature option-attribute callback. /// internal abstract void OptionAttrCallback(PrintCapabilityOption option, XmlPrintCapReader reader); ////// Standard PrintCapabilities feature option-property callback. /// internal abstract bool OptionPropCallback(PrintCapabilityOption option, XmlPrintCapReader reader); #endregion Internal Methods #region Internal Properties internal InternalPrintCapabilities OwnerPrintCap { get { return _ownerPrintCap; } set { _ownerPrintCap = value; } } // derived types must implement these abstract properties internal abstract bool IsValid { get; } internal abstract string FeatureName { get; } internal abstract bool HasSubFeature { get; } #endregion Internal Properties #region Internal/Private Fields private InternalPrintCapabilities _ownerPrintCap; #endregion Internal/Private Fields } ////// /// Do not use. /// Abstract base class of abstract internal class PrintCapabilityOption { #region Constructors ///option. /// /// Constructs a new instance of the PrintCapabilityOption class. /// /// Theobject this option belongs to. protected PrintCapabilityOption(PrintCapabilityFeature ownerFeature) { this.OwnerFeature = ownerFeature; } #endregion Constructors #region Internal Properties internal PrintCapabilityFeature OwnerFeature { get { return _ownerFeature; } set { _ownerFeature = value; } } #endregion Internal Properties #region Internal/Private Fields internal string _optionName; private PrintCapabilityFeature _ownerFeature; #endregion Internal/Private Fields } /// /// /// Do not use. /// Abstract base class of abstract internal class ParameterDefinition { #region Constructors ///parameter definition. /// /// Constructs a new instance of the ParameterDefinition class. /// protected ParameterDefinition() { } #endregion Constructors #region Internal Methods // derived types must implement following abstract methods ////// Standard PrintCapabilities parameter-def property callback. /// internal abstract bool ParamDefPropCallback(ParameterDefinition baseParam, XmlPrintCapReader reader); #endregion Internal Methods #region Internal Properties internal string ParameterName { get { return _parameterName; } set { _parameterName = value; } } // derived types must implement these abstract properties internal abstract bool IsValid { get; } #endregion Internal Properties #region Internal/Private Fields private string _parameterName; #endregion Internal/Private Fields } ////// /// Do not use. /// Derived class from internal class NonNegativeIntParameterDefinition : ParameterDefinition { #region Constructors ///for parameter definition with non-negative integer value. /// /// Constructs a new instance of the NonNegativeIntParameterDefinition class. /// internal NonNegativeIntParameterDefinition() : base() { _minValue = _maxValue = _defaultValue = PrintSchema.UnspecifiedIntValue; } #endregion Constructors #region Public Properties ////// Gets the parameter's maximum integer value. /// public int MaxValue { get { return _maxValue; } } ////// Gets the parameter's minimum integer value. /// public int MinValue { get { return _minValue; } } ////// Gets the parameter's default integer value. /// public int DefaultValue { get { return _defaultValue; } } #endregion Public Properties #region Public Methods ////// Converts this ///object to a human-readable string. /// A string that represents this public override string ToString() { return ParameterName + ": min=" + MinValue + ", max=" + MaxValue + ", default=" + DefaultValue; } #endregion Public Methods #region Internal Methods // With current design, all parameter-def properties are generic to any specific parameters, // so we just need to implement the prop-callback at the base class level and all derived // classes will inherit the implementation. ///object. XML is not well-formed. internal override sealed bool ParamDefPropCallback(ParameterDefinition baseParam, XmlPrintCapReader reader) { NonNegativeIntParameterDefinition param = baseParam as NonNegativeIntParameterDefinition; bool handled = true; #if _DEBUG Trace.Assert(reader.CurrentElementNodeType == PrintSchemaNodeTypes.Property, "THIS SHOULD NOT HAPPEN: NonNegativeIntParamDefPropCallback() gets non-Property node"); #endif if (reader.CurrentElementPSFNameAttrValue == PrintSchemaTags.Keywords.ParameterProps.DefaultValue) { try { param._defaultValue = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine("-Error- " + e.Message); #endif } } else if (reader.CurrentElementPSFNameAttrValue == PrintSchemaTags.Keywords.ParameterProps.MaxValue) { try { param._maxValue = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine("-Error- " + e.Message); #endif } } else if (reader.CurrentElementPSFNameAttrValue == PrintSchemaTags.Keywords.ParameterProps.MinValue) { try { param._minValue = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine("-Error- " + e.Message); #endif } } else { handled = false; #if _DEBUG Trace.WriteLine("-Warning- skip unknown Property '" + reader.CurrentElementNameAttrValue + "' at line " + reader._xmlReader.LineNumber + ", position " + reader._xmlReader.LinePosition); #endif } return handled; } #endregion Internal Methods #region Internal Properties internal override sealed bool IsValid { get { if ((MinValue >= 0) && (MaxValue >= 0) && (DefaultValue >= 0)) { return true; } else { #if _DEBUG Trace.WriteLine("-Warning- negative integer is invalid in " + this.ToString()); #endif return false; } } } #endregion Internal Properties #region Internal Fields internal int _maxValue; internal int _minValue; internal int _defaultValue; #endregion Internal Fields } ////// /// Do not use. /// Derived class from internal class LengthParameterDefinition : NonNegativeIntParameterDefinition { #region Constructors ///for parameter definition with non-negative double value. /// /// Constructs a new instance of the LengthParameterDefinition class. /// internal LengthParameterDefinition() : base() { } #endregion Constructors #region Public Properties ////// Gets the parameter's maximum value, in 1/96 inch unit. /// public new double MaxValue { get { return UnitConverter.LengthValueFromMicronToDIP(_maxValue); } } ////// Gets the parameter's minimum value, in 1/96 inch unit. /// public new double MinValue { get { return UnitConverter.LengthValueFromMicronToDIP(_minValue); } } ////// Gets the parameter's default value, in 1/96 inch unit. /// public new double DefaultValue { get { return UnitConverter.LengthValueFromMicronToDIP(_defaultValue); } } #endregion Public Properties #region Public Methods ////// Converts this ///object to a human-readable string. /// A string that represents this public override string ToString() { return ParameterName + ": min=" + MinValue + ", max=" + MaxValue + ", default=" + DefaultValue; } #endregion Public Methods } ///object. /// /// Do not use. /// Abstract base class of abstract internal class PrintCapabilityRootProperty { #region Constructors ///root-level property. /// /// Constructs a new instance of the PrintCapabilityRootProperty class. /// protected PrintCapabilityRootProperty() { } #endregion Constructors #region Internal Methods // derived types must implement following abstract method ////// Standard PrintCapabilities root-level property's callback to parse and build itself. /// internal abstract bool BuildProperty(XmlPrintCapReader reader); #endregion Internal Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
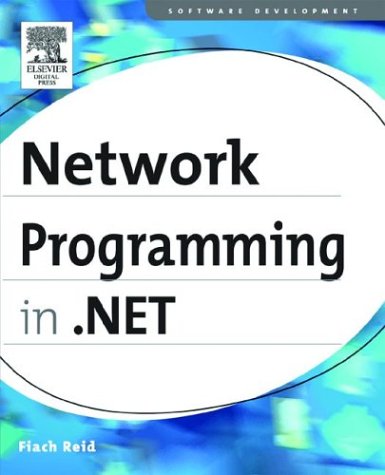
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClrProviderManifest.cs
- AuthenticationModuleElementCollection.cs
- SafeProcessHandle.cs
- TokenBasedSet.cs
- FloaterParaClient.cs
- Label.cs
- DataKey.cs
- LoginCancelEventArgs.cs
- VersionedStream.cs
- TextBox.cs
- ConfigXmlReader.cs
- ConfigurationStrings.cs
- ListViewGroupItemCollection.cs
- EntityStoreSchemaFilterEntry.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- StateInitializationDesigner.cs
- PerformanceCounterLib.cs
- FileDialogCustomPlace.cs
- smtpconnection.cs
- QueryCacheEntry.cs
- LinkedResourceCollection.cs
- ObjectStateFormatter.cs
- PathSegmentCollection.cs
- SkinIDTypeConverter.cs
- ListViewHitTestInfo.cs
- DoubleSumAggregationOperator.cs
- DrawingBrush.cs
- CaretElement.cs
- GridViewEditEventArgs.cs
- ClientTargetCollection.cs
- SerializationInfo.cs
- SkipStoryboardToFill.cs
- DataSourceXmlElementAttribute.cs
- JavaScriptObjectDeserializer.cs
- BitmapCacheBrush.cs
- XmlEncoding.cs
- SqlFlattener.cs
- LayoutTable.cs
- NumberEdit.cs
- MatrixAnimationBase.cs
- DBSchemaTable.cs
- DataListComponentEditor.cs
- BasePattern.cs
- ActivationServices.cs
- SizeConverter.cs
- TaiwanCalendar.cs
- HttpModuleActionCollection.cs
- EndpointAddress.cs
- EncodingTable.cs
- KeyedCollection.cs
- IntSecurity.cs
- DataGridColumnCollection.cs
- BoundColumn.cs
- XMLSyntaxException.cs
- ListSortDescriptionCollection.cs
- ModelPerspective.cs
- WindowsFormsHost.cs
- ToolboxSnapDragDropEventArgs.cs
- NetworkInterface.cs
- UriSection.cs
- IconConverter.cs
- XmlEnumAttribute.cs
- CompositeActivityCodeGenerator.cs
- FixedHighlight.cs
- XmlSchemaDatatype.cs
- CharStorage.cs
- PhysicalOps.cs
- ResourcePool.cs
- DataFormat.cs
- DataControlLinkButton.cs
- ColumnTypeConverter.cs
- formatter.cs
- HMACSHA512.cs
- CompoundFileStreamReference.cs
- Policy.cs
- FontSizeConverter.cs
- HtmlTable.cs
- LinkGrep.cs
- _Events.cs
- XPathBuilder.cs
- PagesChangedEventArgs.cs
- OutOfProcStateClientManager.cs
- WebPermission.cs
- SqlError.cs
- MatrixStack.cs
- ObjectSpanRewriter.cs
- Misc.cs
- ClientBase.cs
- SymbolType.cs
- HashSetEqualityComparer.cs
- WinFormsSpinner.cs
- SafeTokenHandle.cs
- SingleBodyParameterMessageFormatter.cs
- FrameworkReadOnlyPropertyMetadata.cs
- HttpProfileBase.cs
- CursorInteropHelper.cs
- Annotation.cs
- StrokeNodeOperations.cs
- PersistenceProviderFactory.cs
- AddInController.cs