Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / PrintConfig / PrtCap_Public_Simple.cs / 1 / PrtCap_Public_Simple.cs
/*++ Copyright (C) 2003-2005 Microsoft Corporation All rights reserved. Module Name: PrtCap_Public_Simple.cs Abstract: Definition and implementation of simple public PT/PC classes. Author: [....] ([....]) 8/26/2005 --*/ using System; using System.Xml; using System.IO; using System.Collections; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Globalization; using System.Printing; using MS.Internal.Printing.Configuration; namespace System.Printing { ////// Represents page imageable area capability. /// public sealed class PageImageableArea { #region Constructors internal PageImageableArea(double originW, double originH, double extentW, double extentH) { _originW = originW; _originH = originH; _extentW = extentW; _extentH = extentH; } #endregion Constructors #region Public Properties ////// Gets the horizontal origin (in 1/96 inch unit) of the imageable area relative to the application media size. /// public double OriginWidth { get { return _originW; } } ////// Gets the vertical origin (in 1/96 inch unit) of the imageable area relative to the application media size. /// public double OriginHeight { get { return _originH; } } ////// Gets the horizontal distance (in 1/96 inch unit) between the origin and the bounding limit /// of the application media size. /// public double ExtentWidth { get { return _extentW; } } ////// Gets the vertical distance (in 1/96 inch unit) between the origin and the bounding limit /// of the application media size. /// public double ExtentHeight { get { return _extentH; } } #endregion Public Properties #region Public Methods ////// Converts the page imageable area capability to human-readable string. /// ///String that shows the page imageable area capability. public override string ToString() { return String.Format(CultureInfo.CurrentCulture, "({0}, {1}), ({2}, {3})", OriginWidth, OriginHeight, ExtentWidth, ExtentHeight); } #endregion Public Methods #region Private Fields private double _originW; private double _originH; private double _extentW; private double _extentH; #endregion private Fields } ////// Represents supported range for page scaling factor setting. /// public sealed class PageScalingFactorRange { #region Constructors internal PageScalingFactorRange(int scaleMin, int scaleMax) { _scaleMin = scaleMin; _scaleMax = scaleMax; } #endregion Constructors #region Public Properties ////// Gets the minimum page scaling factor (in percentage unit) supported. /// public int MinimumScale { get { return _scaleMin; } } ////// Gets the maximum page scaling factor (in percentage unit) supported. /// public int MaximumScale { get { return _scaleMax; } } #endregion Public Properties #region Public Methods ////// Converts the page scaling factor range to human-readable string. /// ///String that shows the page scaling factor range. public override string ToString() { return String.Format(CultureInfo.CurrentCulture, "({0}, {1})", MinimumScale, MaximumScale); } #endregion Public Methods #region Private Fields private int _scaleMin; private int _scaleMax; #endregion private Fields } ////// Represents a printer's printing capabilities. /// public sealed class PrintCapabilities { #region Constructors ////// Constructs a new instance of ///with printing capabilities /// based on the XML form of PrintCapabilities. /// object containing the XML form of PrintCapabilities. /// /// The ///parameter is null. /// /// The public PrintCapabilities(Stream xmlStream) { _printCap = new InternalPrintCapabilities(xmlStream); } #endregion Constructors #region Public Properties ///object specified by parameter doesn't contain a /// well-formed XML PrintCapabilities. The exception object's property describes /// why the XML is not well-formed PrintCapabilities. If not null, the exception object's /// property provides more details. /// /// Gets a read-only collection of ///that represents the printer's document collate capability. /// /// If the printer has no document collate capability, the returned collection will be empty. /// public ReadOnlyCollectionCollationCapability { get { if (_collationCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.DocumentCollate)) { foreach (CollateOption option in _printCap.DocumentCollateCapability.CollateOptions) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _collationCap = valueSet.AsReadOnly(); } return _collationCap; } } /// /// Gets a read-only collection of ///that represents the printer's /// device font substitution capability. /// /// If the printer has no device font substitution capability, the returned collection will be empty. /// public ReadOnlyCollectionDeviceFontSubstitutionCapability { get { if (_deviceFontCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.PageDeviceFontSubstitution)) { foreach (DeviceFontSubstitutionOption option in _printCap.PageDeviceFontSubstitutionCapability.DeviceFontSubstitutionOptions) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _deviceFontCap = valueSet.AsReadOnly(); } return _deviceFontCap; } } /// /// Gets a read-only collection of ///that represents the printer's job duplex capability. /// /// If the printer has no job duplex capability, the returned collection will be empty. /// public ReadOnlyCollectionDuplexingCapability { get { if (_duplexingCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.JobDuplex)) { foreach (DuplexOption option in _printCap.JobDuplexCapability.DuplexOptions) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _duplexingCap = valueSet.AsReadOnly(); } return _duplexingCap; } } /// /// Gets a read-only collection of ///that represents the printer's input bin capability. /// /// Print Schema defines three input bin capabilities: JobInputBin, DocumentInputBin and PageInputBin. /// /// If none of the JobInputBin, DocumentInputBin or PageInputBin capability is supported by the printer, /// then the returned collection will be empty. Otherwise, the returned collection will represent the printer's /// input bin capability for JobInputBin if JobInputBin is supported, or for DocumentInputBin if DocumentInputBin /// is supported, or for PageInputBin. /// public ReadOnlyCollectionInputBinCapability { get { if (_inputBinCap == null) { List valueSet = new List (); Collection inputBinOptions = null; if (_printCap.SupportsCapability(CapabilityName.JobInputBin)) { // read the JobInputBin capability inputBinOptions = _printCap.JobInputBinCapability.InputBins; } else if (_printCap.SupportsCapability(CapabilityName.DocumentInputBin)) { // read the DocumentInputBin capability if JobInputBin is not supported inputBinOptions = _printCap.DocumentInputBinCapability.InputBins; } else if (_printCap.SupportsCapability(CapabilityName.PageInputBin)) { // read the PageInputBin capability if neither JobInputBin nor DocumentInputBin is supported inputBinOptions = _printCap.PageInputBinCapability.InputBins; } if (inputBinOptions != null) { foreach (InputBinOption option in inputBinOptions) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _inputBinCap = valueSet.AsReadOnly(); } return _inputBinCap; } } /// /// Gets the maximum job copy count the printer can support. /// ////// If the printer doesn't report maximum job copy count supported, this property will return null. /// public NullableMaxCopyCount { get { if (_maxCopyCount == null) { if (_printCap.SupportsCapability(CapabilityName.JobCopyCount) && (_printCap.JobCopyCountCapability.MaxValue != PrintSchema.UnspecifiedIntValue)) { _maxCopyCount = _printCap.JobCopyCountCapability.MaxValue; } } return _maxCopyCount; } } /// /// Gets the horizontal dimension (in 1/96 inch unit) of the application media size relative to the PageOrientation. /// public NullableOrientedPageMediaWidth { get { if (_orientedPageMediaWidth == null) { if (_printCap.SupportsCapability(CapabilityName.PageImageableSize) && (_printCap.PageImageableSizeCapability.ImageableSizeWidth != PrintSchema.UnspecifiedDoubleValue)) { _orientedPageMediaWidth = _printCap.PageImageableSizeCapability.ImageableSizeWidth; } } return _orientedPageMediaWidth; } } /// /// Gets the vertical dimension (in 1/96 inch unit) of the application media size relative to the PageOrientation. /// public NullableOrientedPageMediaHeight { get { if (_orientedPageMediaHeight == null) { if (_printCap.SupportsCapability(CapabilityName.PageImageableSize) && (_printCap.PageImageableSizeCapability.ImageableSizeHeight != PrintSchema.UnspecifiedDoubleValue)) { _orientedPageMediaHeight = _printCap.PageImageableSizeCapability.ImageableSizeHeight; } } return _orientedPageMediaHeight; } } /// /// Gets a read-only collection of ///that represents the printer's /// page output color capability. /// /// If the printer has no page output color capability, the returned collection will be empty. /// public ReadOnlyCollectionOutputColorCapability { get { if (_outputColorCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.PageOutputColor)) { foreach (OutputColorOption option in _printCap.PageOutputColorCapability.OutputColors) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _outputColorCap = valueSet.AsReadOnly(); } return _outputColorCap; } } /// /// Gets a read-only collection of ///that represents the printer's /// page output quality capability. /// /// If the printer has no page output quality capability, the returned collection will be empty. /// public ReadOnlyCollectionOutputQualityCapability { get { if (_outputQualityCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.PageOutputQuality)) { foreach (OutputQualityOption option in _printCap.PageOutputQualityCapability.OutputQualityOptions) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _outputQualityCap = valueSet.AsReadOnly(); } return _outputQualityCap; } } /// /// Gets a read-only collection of ///that represents the printer's /// page borderless capability. /// /// If the printer has no page borderless capability, the returned collection will be empty. /// public ReadOnlyCollectionPageBorderlessCapability { get { if (_pageBorderlessCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.PageBorderless)) { foreach (BorderlessOption option in _printCap.PageBorderlessCapability.BorderlessOptions) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _pageBorderlessCap = valueSet.AsReadOnly(); } return _pageBorderlessCap; } } /// /// Gets a ///that represents the printer's page imageable area capability. /// /// If the printer has no page imageable area capability, this property will return null. /// public PageImageableArea PageImageableArea { get { if (_pageImageableArea == null) { if (_printCap.SupportsCapability(CapabilityName.PageImageableSize) && (_printCap.PageImageableSizeCapability.ImageableArea != null)) { CanvasImageableArea area = _printCap.PageImageableSizeCapability.ImageableArea; if ((area.OriginWidth != PrintSchema.UnspecifiedDoubleValue) && (area.OriginHeight != PrintSchema.UnspecifiedDoubleValue) && (area.ExtentWidth != PrintSchema.UnspecifiedDoubleValue) && (area.ExtentHeight != PrintSchema.UnspecifiedDoubleValue)) { _pageImageableArea = new PageImageableArea(area.OriginWidth, area.OriginHeight, area.ExtentWidth, area.ExtentHeight); } } } return _pageImageableArea; } } ////// Gets a read-only collection of ///that represents the printer's /// page media size capability. /// /// If the printer has no page media size capability, the returned collection will be empty. /// public ReadOnlyCollectionPageMediaSizeCapability { get { if (_pageMediaSizeCap == null) { List mediaSet = new List (); if (_printCap.SupportsCapability(CapabilityName.PageMediaSize)) { foreach (FixedMediaSizeOption option in _printCap.PageMediaSizeCapability.FixedMediaSizes) { if ((option.MediaSizeWidth != PrintSchema.UnspecifiedDoubleValue) && (option.MediaSizeHeight != PrintSchema.UnspecifiedDoubleValue)) { mediaSet.Add(new PageMediaSize(option.Value, option.MediaSizeWidth, option.MediaSizeHeight)); } else { mediaSet.Add(new PageMediaSize(option.Value)); } } } _pageMediaSizeCap = mediaSet.AsReadOnly(); } return _pageMediaSizeCap; } } /// /// Gets a read-only collection of ///that represents the printer's /// page media type capability. /// /// If the printer has no page media type capability, the returned collection will be empty. /// public ReadOnlyCollectionPageMediaTypeCapability { get { if (_pageMediaTypeCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.PageMediaType)) { foreach (MediaTypeOption option in _printCap.PageMediaTypeCapability.MediaTypes) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _pageMediaTypeCap = valueSet.AsReadOnly(); } return _pageMediaTypeCap; } } /// /// Gets a read-only collection of ///that represents the printer's /// job page order capability. /// /// If the printer has no job page order capability, the returned collection will be empty. /// public ReadOnlyCollectionPageOrderCapability { get { if (_pageOrderCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.JobPageOrder)) { foreach (PageOrderOption option in _printCap.JobPageOrderCapability.PageOrderOptions) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _pageOrderCap = valueSet.AsReadOnly(); } return _pageOrderCap; } } /// /// Gets a read-only collection of ///that represents the printer's /// page orientation capability. /// /// If the printer has no page orientation capability, the returned collection will be empty. /// public ReadOnlyCollectionPageOrientationCapability { get { if (_pageOrientationCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.PageOrientation)) { foreach (OrientationOption option in _printCap.PageOrientationCapability.Orientations) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _pageOrientationCap = valueSet.AsReadOnly(); } return _pageOrientationCap; } } /// /// Gets a read-only collection of ///that represents the printer's /// page resolution capability. /// /// If the printer has no page resolution capability, the returned collection will be empty. /// public ReadOnlyCollectionPageResolutionCapability { get { if (_pageResolutionCap == null) { List resSet = new List (); if (_printCap.SupportsCapability(CapabilityName.PageResolution)) { foreach (ResolutionOption option in _printCap.PageResolutionCapability.Resolutions) { if ((int)option.QualitativeResolution != PrintSchema.EnumUnspecifiedValue) { resSet.Add(new PageResolution(option.ResolutionX, option.ResolutionY, option.QualitativeResolution)); } else { resSet.Add(new PageResolution(option.ResolutionX, option.ResolutionY)); } } } _pageResolutionCap = resSet.AsReadOnly(); } return _pageResolutionCap; } } /// /// Gets a ///that represents the printer's supported range /// for page scaling factor. /// /// If the printer has no page scaling capability, this property will return null. /// public PageScalingFactorRange PageScalingFactorRange { get { if (_pageScalingFactorRange == null) { if (_printCap.SupportsCapability(CapabilityName.PageScaling)) { int min = -1, max = -1; foreach (ScalingOption option in _printCap.PageScalingCapability.ScalingOptions) { // // Going through the scaling options and find out the smallest [min, max] range // if (option.Value == PageScaling.Custom) { if ((min == -1) || (option.CustomScaleWidth.MinValue > min)) { min = option.CustomScaleWidth.MinValue; } if ((max == -1) || (option.CustomScaleWidth.MaxValue < max)) { max = option.CustomScaleWidth.MaxValue; } if (option.CustomScaleHeight.MinValue > min) min = option.CustomScaleHeight.MinValue; if (option.CustomScaleHeight.MaxValue < max) max = option.CustomScaleHeight.MaxValue; } else if (option.Value == PageScaling.CustomSquare) { if ((min == -1) || (option.CustomSquareScale.MinValue > min)) { min = option.CustomSquareScale.MinValue; } if ((max == -1) || (option.CustomSquareScale.MaxValue < max)) { max = option.CustomSquareScale.MaxValue; } } } if ((min > 0) && (max > 0)) { _pageScalingFactorRange = new PageScalingFactorRange(min, max); } } } return _pageScalingFactorRange; } } ////// Gets a read-only collection of integers that represents the printer's job pages-per-sheet capability. /// ////// If the printer has no job pages-per-sheet capability, the returned collection will be empty. /// (Pages-per-sheet is to output multiple logical pages to a single physical sheet.) /// public ReadOnlyCollectionPagesPerSheetCapability { get { if (_pagesPerSheetCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.JobNUp)) { foreach (NUpOption option in _printCap.JobNUpCapability.NUps) { if (!valueSet.Contains(option.PagesPerSheet)) { valueSet.Add(option.PagesPerSheet); } } } _pagesPerSheetCap = valueSet.AsReadOnly(); } return _pagesPerSheetCap; } } /// /// Gets a read-only collection of ///that represents the printer's /// job pages-per-sheet direction capability. /// /// If the printer has no job pages-per-sheet direction capability, the returned collection will be empty. /// (Pages-per-sheet is to output multiple logical pages to a single physical sheet.) /// public ReadOnlyCollectionPagesPerSheetDirectionCapability { get { if (_pagesPerSheetDirectionCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.JobNUp) && _printCap.JobNUpCapability.SupportsPresentationDirection) { foreach (NUpPresentationDirectionOption option in _printCap.JobNUpCapability.PresentationDirectionCapability.PresentationDirections) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _pagesPerSheetDirectionCap = valueSet.AsReadOnly(); } return _pagesPerSheetDirectionCap; } } /// /// Gets a read-only collection of ///that represents the printer's /// page photo printing intent capability. /// /// If the printer has no page photo printing intent capability, the returned collection will be empty. /// public ReadOnlyCollectionPhotoPrintingIntentCapability { get { if (_photoIntentCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.PagePhotoPrintingIntent)) { foreach (PhotoPrintingIntentOption option in _printCap.PagePhotoPrintingIntentCapability.PhotoPrintingIntentOptions) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _photoIntentCap = valueSet.AsReadOnly(); } return _photoIntentCap; } } /// /// Gets a read-only collection of ///that represents the printer's job output stapling capability. /// /// If the printer has no job output stapling capability, the returned collection will be empty. /// public ReadOnlyCollectionStaplingCapability { get { if (_staplingCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.JobStaple)) { foreach (StaplingOption option in _printCap.JobStapleCapability.StaplingOptions) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _staplingCap = valueSet.AsReadOnly(); } return _staplingCap; } } /// /// Gets a read-only collection of ///that represents the printer's /// TrueType font handling mode capability. /// /// If the printer has no TrueType font handling mode capability, the returned collection will be empty. /// public ReadOnlyCollectionTrueTypeFontModeCapability { get { if (_ttFontCap == null) { List valueSet = new List (); if (_printCap.SupportsCapability(CapabilityName.PageTrueTypeFontMode)) { foreach (TrueTypeFontModeOption option in _printCap.PageTrueTypeFontModeCapability.TrueTypeFontModes) { if (!valueSet.Contains(option.Value)) { valueSet.Add(option.Value); } } } _ttFontCap = valueSet.AsReadOnly(); } return _ttFontCap; } } #endregion Public Properties #region Private Fields private InternalPrintCapabilities _printCap; private ReadOnlyCollection _collationCap; private ReadOnlyCollection _deviceFontCap; private ReadOnlyCollection _duplexingCap; private ReadOnlyCollection _inputBinCap; private Nullable _maxCopyCount; private Nullable _orientedPageMediaWidth; private Nullable _orientedPageMediaHeight; private ReadOnlyCollection _outputColorCap; private ReadOnlyCollection _outputQualityCap; private ReadOnlyCollection _pageBorderlessCap; private PageImageableArea _pageImageableArea; private ReadOnlyCollection _pageMediaSizeCap; private ReadOnlyCollection _pageMediaTypeCap; private ReadOnlyCollection _pageOrderCap; private ReadOnlyCollection _pageOrientationCap; private ReadOnlyCollection _pageResolutionCap; private PageScalingFactorRange _pageScalingFactorRange; private ReadOnlyCollection _pagesPerSheetCap; private ReadOnlyCollection _pagesPerSheetDirectionCap; private ReadOnlyCollection _photoIntentCap; private ReadOnlyCollection _staplingCap; private ReadOnlyCollection _ttFontCap; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
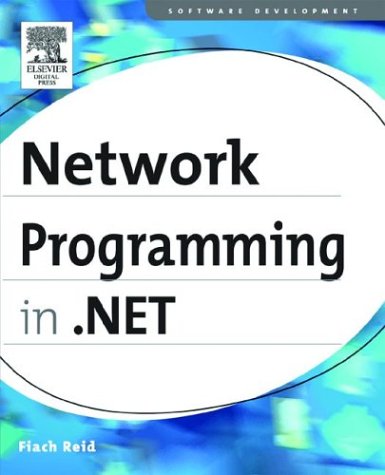
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Events.cs
- HttpGetProtocolImporter.cs
- PassportAuthenticationEventArgs.cs
- XPathQueryGenerator.cs
- DeferredElementTreeState.cs
- CapabilitiesSection.cs
- ComponentEditorPage.cs
- Separator.cs
- DynamicILGenerator.cs
- UnsafeCollabNativeMethods.cs
- InternalConfigRoot.cs
- AssemblySettingAttributes.cs
- SqlFacetAttribute.cs
- CodeCatchClause.cs
- Expressions.cs
- IndexedString.cs
- DynamicMethod.cs
- HttpResponse.cs
- SqlRowUpdatingEvent.cs
- LayoutTable.cs
- AccessibleObject.cs
- ObjectDataSourceFilteringEventArgs.cs
- DefaultBindingPropertyAttribute.cs
- CustomErrorCollection.cs
- SimpleHandlerBuildProvider.cs
- RegexBoyerMoore.cs
- Visual3D.cs
- CopyAttributesAction.cs
- WebPartVerbsEventArgs.cs
- ResourceReferenceExpression.cs
- Int64Animation.cs
- CmsInterop.cs
- MsmqElementBase.cs
- DesignerActionKeyboardBehavior.cs
- ServiceThrottlingBehavior.cs
- TextBlock.cs
- DownloadProgressEventArgs.cs
- TypographyProperties.cs
- ProcessRequestArgs.cs
- Binding.cs
- CodeIdentifiers.cs
- MembershipSection.cs
- ButtonFlatAdapter.cs
- DataContract.cs
- MenuItem.cs
- XpsThumbnail.cs
- WebDescriptionAttribute.cs
- ImageMap.cs
- CapabilitiesUse.cs
- Selection.cs
- MultiDataTrigger.cs
- SqlRemoveConstantOrderBy.cs
- RoleService.cs
- AdRotatorDesigner.cs
- RectAnimationUsingKeyFrames.cs
- TreeView.cs
- SelectionItemProviderWrapper.cs
- RichTextBoxContextMenu.cs
- unsafeIndexingFilterStream.cs
- ExpressionReplacer.cs
- TextDecoration.cs
- URLString.cs
- ExpressionPrefixAttribute.cs
- EntityStoreSchemaGenerator.cs
- EntryIndex.cs
- CrossSiteScriptingValidation.cs
- BroadcastEventHelper.cs
- CharKeyFrameCollection.cs
- DetailsViewUpdateEventArgs.cs
- HttpBrowserCapabilitiesWrapper.cs
- TreeViewCancelEvent.cs
- ResolveMatchesCD1.cs
- Metafile.cs
- RadioButtonPopupAdapter.cs
- EncodingInfo.cs
- ADMembershipProvider.cs
- RemoteWebConfigurationHostStream.cs
- VisualStyleElement.cs
- XLinq.cs
- BamlStream.cs
- WindowsFormsHostPropertyMap.cs
- DataReceivedEventArgs.cs
- PersonalizationAdministration.cs
- SecurityKeyType.cs
- SrgsDocumentParser.cs
- CanonicalXml.cs
- HwndSubclass.cs
- CompiledIdentityConstraint.cs
- ListControlConvertEventArgs.cs
- PrintDialogDesigner.cs
- NumberFormatInfo.cs
- SymLanguageVendor.cs
- AxisAngleRotation3D.cs
- ConfigXmlComment.cs
- AddIn.cs
- NetworkInterface.cs
- ConfigurationValidatorBase.cs
- EditorServiceContext.cs
- ObjectPropertyMapping.cs
- BinaryParser.cs