Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / manager / ReachSerializer.cs / 1 / ReachSerializer.cs
/*++ Copyright (C) 2004- 2005 Microsoft Corporation All rights reserved. Module Name: ReachSerializer.cs Abstract: This file contains the definition of a Base class that defines the common functionality required to serialie on type in a graph of types rooted by some object instance Author: [....] ([....]) 1-December-2004 Revision History: --*/ using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.ComponentModel.Design.Serialization; using System.Windows.Xps.Packaging; using System.Windows.Documents; using System.Windows.Media; namespace System.Windows.Xps.Serialization { ////// Base class defining common functionalities required to /// serialize one type. /// internal abstract class ReachSerializer : IDisposable { #region Constructor ////// Constructor for class ReachSerializer /// /// /// The serializtion manager, the services of which are /// used later for the serialization process of the type. /// public ReachSerializer( PackageSerializationManager manager ) { if(manager == null) { throw new ArgumentNullException("manager"); } _serializationManager = manager; _xmlWriter = null; } ////// Constructor for class ReachSerializer /// internal ReachSerializer( ) { _serializationManager = null; _xmlWriter = null; } #endregion Constructor #region Public Methods ////// The main method that is called to serialize the object of /// that given type. /// /// /// Instance of object to be serialized. /// ////// Critical - Access the SerializationManager GraphContextStack which is a /// ContextStack which is link critical /// /// TreatAsSafe - The context stack is used local to the function. /// All access to the GraphContextStack is marked security critical /// [SecurityCritical, SecurityTreatAsSafe] public virtual void SerializeObject( Object serializedObject ) { if(serializedObject == null) { throw new ArgumentNullException("serializedObject"); } if(SerializationManager == null) { throw new XpsSerializationException(ReachSR.Get(ReachSRID.ReachSerialization_MustHaveSerializationManager)); } // // At this stage discover the graph of properties of the object that // need to be serialized // SerializableObjectContext serializableObjectContext = DiscoverObjectData(serializedObject, null); if(serializableObjectContext!=null) { // // Push the object at hand on the context stack // SerializationManager.GraphContextStack.Push(serializableObjectContext); // // At this stage we should start streaming the markup representing the // object graph to the corresponding destination // PersistObjectData(serializableObjectContext); // // Pop the object from the context stack // SerializationManager.GraphContextStack.Pop(); // // Recycle the used SerializableObjectContext // SerializableObjectContext.RecycleContext(serializableObjectContext); } } #endregion Public Methods #region Internal Methods ////// The main method that is called to serialize the object of /// that given type and that is usually called from within the /// serialization manager when a node in the graph of objects is /// at a turn where it should be serialized. /// /// /// The context of the property being serialized at this time and /// it points internally to the object encapsulated by that node. /// ////// Critical - Access the SerializationManager GraphContextStack which is a /// ContextStack which is link critical /// /// TreatAsSafe - The context stack is used local to the function. /// All access to the GraphContextStack is marked security critical /// [SecurityCritical, SecurityTreatAsSafe] internal virtual void SerializeObject( SerializablePropertyContext serializedProperty ) { if(serializedProperty == null) { throw new ArgumentNullException("serializedProperty"); } if(SerializationManager == null) { throw new XpsSerializationException(ReachSR.Get(ReachSRID.ReachSerialization_MustHaveSerializationManager)); } // // At this stage discover the graph of properties of the object that // need to be serialized // SerializableObjectContext serializableObjectContext = DiscoverObjectData(serializedProperty.Value, serializedProperty); if(serializableObjectContext!=null) { // // Push the object at hand on the context stack // SerializationManager.GraphContextStack.Push(serializableObjectContext); // // At this stage we should start streaming the markup representing the // object graph to the corresponding destination // PersistObjectData(serializableObjectContext); // // Pop the object from the context stack // SerializationManager.GraphContextStack.Pop(); // // Recycle the used SerializableObjectContext // SerializableObjectContext.RecycleContext(serializableObjectContext); } } ////// The method is called once the object data is discovered at that /// point of the serialization process. /// /// /// The context of the object to be serialized at this time. /// internal abstract void PersistObjectData( SerializableObjectContext serializableObjectContext ); ////// Serialize the properties within the object /// context into METRO /// ////// Method follows these steps /// 1. Serializes the instance as string content /// if is not meant to be a complex value. Else ... /// 2. Serialize Properties as attributes /// 3. Serialize Complex Properties as separate parts /// through calling separate serializers /// Also this is the virtual to override custom attributes or /// contents need to be serialized /// /// /// The context of the object to be serialized at this time. /// internal virtual void SerializeObjectCore( SerializableObjectContext serializableObjectContext ) { if (serializableObjectContext == null) { throw new ArgumentNullException("serializableObjectContext"); } if (!serializableObjectContext.IsReadOnlyValue && serializableObjectContext.IsComplexValue) { SerializeProperties(serializableObjectContext); } } ////// This method is the one that writes out the attribute within /// the xml stream when serializing simple properites. /// /// /// The property that is to be serialized as an attribute at this time. /// internal virtual void WriteSerializedAttribute( SerializablePropertyContext serializablePropertyContext ) { if(serializablePropertyContext == null) { throw new ArgumentNullException("serializablePropertyContext"); } } #endregion Internal Methods #region Private Methods ////// This method is the one that parses down the object at hand /// to discover all the properties that are expected to be serialized /// at that object level. /// the xml stream when serializing simple properties. /// /// /// The instance of the object being serialized. /// /// /// The instance of property on the parent object from which this /// object stemmed. This could be null if this is the node object /// or the object has no parent. /// ////// Critical - Access the SerializationManager GraphContextStack which is a /// ContextStack which is link critical /// /// TreatAsSafe - The context stack is used local to the function. /// All access to the GraphContextStack is marked security critical /// [SecurityCritical, SecurityTreatAsSafe] private SerializableObjectContext DiscoverObjectData( Object serializedObject, SerializablePropertyContext serializedProperty ) { // // Trying to figure out the parent of this node, which is at this stage // the same node previously pushed on the stack or in other words it is // the node that is currently on the top of the stack // SerializableObjectContext serializableObjectParentContext = (SerializableObjectContext)SerializationManager. GraphContextStack[typeof(SerializableObjectContext)]; // // Create the context for the current object // SerializableObjectContext serializableObjectContext = SerializableObjectContext.CreateContext(SerializationManager, serializedObject, serializableObjectParentContext, serializedProperty); // // Set the root object to be serialized at the level of the SerializationManager // if(SerializationManager.RootSerializableObjectContext == null) { SerializationManager.RootSerializableObjectContext = serializableObjectContext; } return serializableObjectContext; } ////// Trigger all properties serialization /// private void SerializeProperties( SerializableObjectContext serializableObjectContext ) { if (serializableObjectContext == null) { throw new ArgumentNullException("serializableObjectContext"); } SerializablePropertyCollection propertyCollection = serializableObjectContext.PropertiesCollection; if(propertyCollection!=null) { for(propertyCollection.Reset(); propertyCollection.MoveNext();) { SerializablePropertyContext serializablePropertyContext = (SerializablePropertyContext)propertyCollection.Current; if(serializablePropertyContext!=null) { SerializeProperty(serializablePropertyContext); } } } } ////// Trigger serializing one property at a time. /// private void SerializeProperty( SerializablePropertyContext serializablePropertyContext ) { if(serializablePropertyContext == null) { throw new ArgumentNullException("serializablePropertyContext"); } if(!serializablePropertyContext.IsComplex) { // // Non-Complex Properties are serialized as attributes // WriteSerializedAttribute(serializablePropertyContext); } else { // // Complex properties could be treated in different ways // based on their type. Examples of that are: // // // ReachSerializer serializer = SerializationManager.GetSerializer(serializablePropertyContext.Value); // If there is no serializer for this type, we won't serialize this property if(serializer!=null) { serializer.SerializeObject(serializablePropertyContext); } } } #endregion Private Methods #region Public Properties ////// Query / Set Xml Writer for the equivelan part /// public virtual XmlWriter XmlWriter { get { return _xmlWriter; } set { _xmlWriter = value; } } ////// Query the SerializationManager used by this serializer. /// public virtual PackageSerializationManager SerializationManager { get { return _serializationManager; } } #endregion Public Properties #region IDisposable implementation void IDisposable.Dispose() { } #endregion IDisposable implementation #region Private Data members private PackageSerializationManager _serializationManager; private XmlWriter _xmlWriter; #endregion Private Data members }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
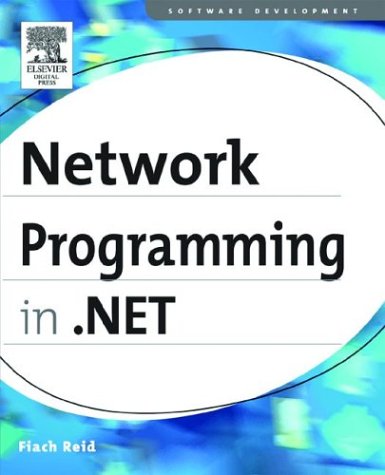
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DecimalConverter.cs
- ToolBarButton.cs
- FileDialogCustomPlacesCollection.cs
- MultiPropertyDescriptorGridEntry.cs
- DataBindingCollection.cs
- MasterPageCodeDomTreeGenerator.cs
- CodeSubDirectory.cs
- EditableRegion.cs
- Point3DConverter.cs
- BamlCollectionHolder.cs
- LogFlushAsyncResult.cs
- DragCompletedEventArgs.cs
- VirtualPathProvider.cs
- UriScheme.cs
- CqlParser.cs
- WebErrorHandler.cs
- ObjectReferenceStack.cs
- OperatorExpressions.cs
- Mutex.cs
- SiblingIterators.cs
- ListViewDeleteEventArgs.cs
- PlanCompiler.cs
- AttributeQuery.cs
- InvalidFilterCriteriaException.cs
- WebUtil.cs
- KeyValuePair.cs
- HttpModuleAction.cs
- SqlExpressionNullability.cs
- ScriptResourceHandler.cs
- ButtonColumn.cs
- NonClientArea.cs
- TextElementEnumerator.cs
- OciEnlistContext.cs
- FilterElement.cs
- HtmlValidatorAdapter.cs
- Container.cs
- LineInfo.cs
- RunInstallerAttribute.cs
- ContentElement.cs
- RootContext.cs
- ResourceContainer.cs
- MessageContractAttribute.cs
- ConstraintConverter.cs
- BuildProviderUtils.cs
- TextEditorParagraphs.cs
- ExpressionCopier.cs
- QueryOutputWriter.cs
- ExtentJoinTreeNode.cs
- BezierSegment.cs
- StylusDownEventArgs.cs
- SafeMILHandleMemoryPressure.cs
- MultiTrigger.cs
- ProcessManager.cs
- ToolStripContainerDesigner.cs
- SmiMetaDataProperty.cs
- XmlDocumentType.cs
- OuterGlowBitmapEffect.cs
- SqlInternalConnectionTds.cs
- HtmlForm.cs
- TypeBuilder.cs
- NativeMethodsOther.cs
- GridViewAutomationPeer.cs
- Main.cs
- MultipartContentParser.cs
- CustomValidator.cs
- SimpleHandlerFactory.cs
- ActivityExecutorDelegateInfo.cs
- UnknownBitmapEncoder.cs
- XsltException.cs
- AnyReturnReader.cs
- ThousandthOfEmRealDoubles.cs
- AttachmentService.cs
- GridViewPageEventArgs.cs
- RequestCacheValidator.cs
- BaseDataBoundControl.cs
- Transform3DCollection.cs
- ListView.cs
- ProxyManager.cs
- PackWebResponse.cs
- DataGridColumnReorderingEventArgs.cs
- FormsAuthenticationEventArgs.cs
- StringFreezingAttribute.cs
- EntitySetDataBindingList.cs
- MaskPropertyEditor.cs
- ExtensionQuery.cs
- DriveNotFoundException.cs
- ConfigXmlElement.cs
- Visual3D.cs
- IBuiltInEvidence.cs
- _HeaderInfo.cs
- DeclaredTypeElement.cs
- SqlReferenceCollection.cs
- GroupStyle.cs
- TimelineGroup.cs
- DataRowExtensions.cs
- BmpBitmapEncoder.cs
- CompilerGeneratedAttribute.cs
- SiteOfOriginContainer.cs
- ConvertersCollection.cs
- SafeNativeMethodsMilCoreApi.cs