Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SrgsCompiler / Rule.cs / 1 / Rule.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Speech.Internal.SrgsParser; using System.Text; namespace System.Speech.Internal.SrgsCompiler { ////// Summary description for Rule. /// #if VSCOMPILE [DebuggerDisplay ("{Name}")] #endif internal sealed class Rule : ParseElementCollection, IRule, IComparable{ //******************************************************************* // // Constructors // //******************************************************************* #region Constructors // Only used for the special transition internal Rule (int iSerialize) : base (null, null) { _iSerialize = iSerialize; } internal Rule (Backend backend, string name, CfgRule cfgRule, int iSerialize, GrammarOptions SemanticFormat, ref int cImportedRules) : base (backend, null) { _rule = this; Init (name, cfgRule, iSerialize, SemanticFormat, ref cImportedRules); } internal Rule (Backend backend, string name, int offsetName, SPCFGRULEATTRIBUTES attributes, int id, int iSerialize, GrammarOptions SemanticFormat, ref int cImportedRules) : base (backend, null) { _rule = this; Init (name, new CfgRule (id, offsetName, attributes), iSerialize, SemanticFormat, ref cImportedRules); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region internal Methods #region IComparable Interface implementation int IComparable .CompareTo (Rule rule2) { Rule rule1 = this; if (rule1._cfgRule.Import) { return (rule2._cfgRule.Import) ? rule1._cfgRule._nameOffset - rule2._cfgRule._nameOffset : -1; } else if (rule1._cfgRule.Dynamic) { return (rule2._cfgRule.Dynamic) ? rule1._cfgRule._nameOffset - rule2._cfgRule._nameOffset : 1; } else { return (rule2._cfgRule.Import) ? 1 : (rule2._cfgRule.Dynamic) ? -1 : rule1._cfgRule._nameOffset - rule2._cfgRule._nameOffset; } } #endregion #if DEBUG /// /// /// /// ///internal void CheckForExitPath (ref int iRecursiveDepth) { //CfgGrammar.TraceInformation ("Rule::CheckForExitPath"); if (!_fHasExitPath) { // This check allows empty rules. if (_firstState != null && _firstState.NumArcs != 0) { _firstState.CheckExitPath (ref iRecursiveDepth); } } } #endif /// /// /// ///internal void Validate () { //CfgGrammar.TraceInformation ("Rule::Validate"); if ((!_cfgRule.Dynamic) && (!_cfgRule.Import) && _id != "VOID" && _firstState.NumArcs == 0) { XmlParser.ThrowSrgsException (SRID.EmptyRule); } else { #if false // if (!(fImport || fDynamic)) { return m_pFirstState->CheckEpsilonRule(); } #endif _fHasDynamicRef = _cfgRule.Dynamic; } } /// /// /// /// internal void PopulateDynamicRef (ref int iRecursiveDepth) { if (iRecursiveDepth > CfgGrammar.MAX_TRANSITIONS_COUNT) { XmlParser.ThrowSrgsException ((SRID.MaxTransitionsCount)); } foreach (Rule rule in _listRules) { if (!rule._fHasDynamicRef) { rule._fHasDynamicRef = true; rule.PopulateDynamicRef (ref iRecursiveDepth); } } } internal Rule Clone (StringBlob symbol, string ruleName) { Rule rule = new Rule (_iSerialize); int idWord; int offsetName = symbol.Add (ruleName, out idWord); rule._id = ruleName; rule._cfgRule = new CfgRule (idWord, offsetName, (uint) _cfgRule._flag); rule._cfgRule.DirtyRule = true; rule._cfgRule.FirstArcIndex = 0; return rule; } internal void Serialize (StreamMarshaler streamBuffer) { //CfgGrammar.TraceInformation ("CRule::Serialize"); // Dynamic rules and imports have no arcs _cfgRule.FirstArcIndex = _firstState != null && !_firstState.OutArcs.IsEmpty ? (uint) _firstState.SerializeId : 0; _cfgRule.DirtyRule = true; streamBuffer.WriteStream (_cfgRule); } void IElement.PostParse (IElement grammar) { // Empty rule if (_endArc == null) { System.Diagnostics.Debug.Assert (_startArc == null); _firstState = _backend.CreateNewState (this); } else { // The last arc may contain an epsilon value. Remove it. TrimEndEpsilons (_endArc, _backend); // If the first arc was an epsilon value then there is no need to create a new state if (_startArc.IsEpsilonTransition && _startArc.End != null && Graph.MoveSemanticTagRight (_startArc)) { // Discard the arc and replace it with the startArc _firstState = _startArc.End; System.Diagnostics.Debug.Assert (_startArc.End == _startArc.End); _startArc.End = null; } else { // if _first has not be set, create it _firstState = _backend.CreateNewState (this); // Attach the start and end arc to the rule _startArc.Start = _firstState; } } } #if !NO_STG void IRule.CreateScript (IGrammar grammar, string rule, string method, RuleMethodScript type) { ((GrammarElement) grammar).CustomGrammar._scriptRefs.Add (new ScriptRef (rule, method, type)); } #endif #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties internal string Name { get { return _id; } } #if !NO_STG string IRule.BaseClass { get { return _baseclass; } set { _baseclass = value; } } internal string BaseClass { get { return _baseclass; } } internal StringBuilder Script { get { return _script; } } internal StringBuilder Constructors { get { return _constructors; } } #endif #endregion //******************************************************************* // // Private Methods // //******************************************************************** #region Private Methods private void Init (string id, CfgRule cfgRule, int iSerialize, GrammarOptions SemanticFormat, ref int cImportedRules) { _id = id; _cfgRule = cfgRule; _firstState = null; _cfgRule.DirtyRule = true; _iSerialize = iSerialize; _fHasExitPath = false; _fHasDynamicRef = false; _fIsEpsilonRule = false; _fStaticRule = false; if (_cfgRule.Import) { cImportedRules++; } } private static void TrimEndEpsilons (Arc end, Backend backend) { Arc endArc = end; State endState = endArc.Start; if (endState != null) { // Remove the end arc if possible, check done by MoveSemanticTagRight if (endArc.IsEpsilonTransition && endState.OutArcs.CountIsOne && Graph.MoveSemanticTagLeft (endArc)) { // State has a single input epsilon transition // Delete the input epsilon transition and delete state. endArc.Start = null; // Remove all the in arcs duplicate the arcs first foreach (Arc inArc in endState.InArcs.ToList ()) { inArc.End = null; TrimEndEpsilons (inArc, backend); } // Delete the input epsilon transition and delete state if appropriate. backend.DeleteState (endState); } } } #endregion //******************************************************************* // // Internal Fields // //******************************************************************* #region Internal Fields internal CfgRule _cfgRule; internal State _firstState; internal bool _fHasExitPath; internal bool _fHasDynamicRef; internal bool _fIsEpsilonRule; internal int _iSerialize; // internal int _iSerialize2; #if DEBUG internal int _cStates; #endif internal List_listRules = new List (); // this is used to refer to a static rule from a dynamic rule internal bool _fStaticRule; #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private string _id; #if !NO_STG // STG fields private string _baseclass; private StringBuilder _script = new StringBuilder (); private StringBuilder _constructors = new StringBuilder (); #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
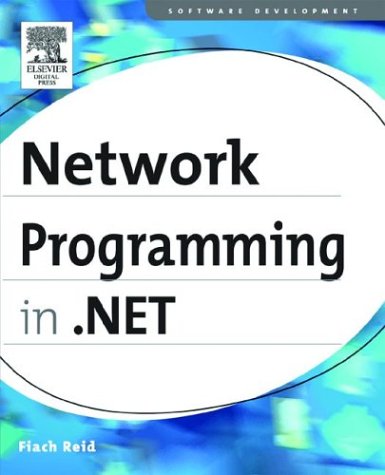
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UpDownEvent.cs
- ObjectDataProvider.cs
- ConstraintCollection.cs
- ZipPackage.cs
- SeekableReadStream.cs
- AdditionalEntityFunctions.cs
- Matrix3D.cs
- CellPartitioner.cs
- LocalizabilityAttribute.cs
- LinqExpressionNormalizer.cs
- ConfigXmlDocument.cs
- DbFunctionCommandTree.cs
- CustomCategoryAttribute.cs
- Size3D.cs
- EntitySet.cs
- XmlSubtreeReader.cs
- BamlLocalizer.cs
- SqlFlattener.cs
- StateMachine.cs
- RelationshipSet.cs
- InvokeProviderWrapper.cs
- CodeDirectiveCollection.cs
- XmlSerializationReader.cs
- Binding.cs
- ListViewItemEventArgs.cs
- StrokeNodeData.cs
- DoubleAnimationClockResource.cs
- DbConnectionPoolGroup.cs
- WebPartDeleteVerb.cs
- SafeNativeMethods.cs
- StylusButtonEventArgs.cs
- EdmFunctions.cs
- SearchExpression.cs
- BuildProviderCollection.cs
- DefaultValueTypeConverter.cs
- GraphicsPathIterator.cs
- DispatcherProcessingDisabled.cs
- TextRangeEditLists.cs
- _LocalDataStoreMgr.cs
- ScriptResourceInfo.cs
- DbConnectionClosed.cs
- GenerateScriptTypeAttribute.cs
- ActivityInstanceMap.cs
- EmptyControlCollection.cs
- TabItemWrapperAutomationPeer.cs
- GenerateHelper.cs
- CountdownEvent.cs
- LogSwitch.cs
- HtmlElementCollection.cs
- UserControlBuildProvider.cs
- counter.cs
- ConsoleCancelEventArgs.cs
- ValidatedControlConverter.cs
- DataGridViewCellCancelEventArgs.cs
- InstallerTypeAttribute.cs
- NotConverter.cs
- DecoderReplacementFallback.cs
- Queue.cs
- Stylesheet.cs
- IncrementalReadDecoders.cs
- ListItemCollection.cs
- CellRelation.cs
- EntitySqlQueryBuilder.cs
- XmlSchemaSet.cs
- AttributeQuery.cs
- TreeView.cs
- UnsafeNativeMethods.cs
- TemplatedWizardStep.cs
- XmlIlTypeHelper.cs
- StreamInfo.cs
- MemberCollection.cs
- BaseCodePageEncoding.cs
- AsyncCompletedEventArgs.cs
- X509Chain.cs
- TextTreeExtractElementUndoUnit.cs
- DecimalAverageAggregationOperator.cs
- Inline.cs
- _KerberosClient.cs
- PropertyGrid.cs
- SafeCryptoKeyHandle.cs
- IndicShape.cs
- propertyentry.cs
- LinqDataSourceDeleteEventArgs.cs
- DelegateBodyWriter.cs
- HasActivatableWorkflowEvent.cs
- ScriptBehaviorDescriptor.cs
- StructuralType.cs
- Timeline.cs
- PersonalizationStateInfoCollection.cs
- ProfileSettings.cs
- LineProperties.cs
- _Rfc2616CacheValidators.cs
- SubstitutionDesigner.cs
- MonthChangedEventArgs.cs
- DbExpressionBuilder.cs
- ITextView.cs
- DbInsertCommandTree.cs
- CodeCatchClause.cs
- GACIdentityPermission.cs
- LocationUpdates.cs