Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Recognition / SrgsGrammar / SrgsToken.cs / 1 / SrgsToken.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 [....] Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Speech.Internal; using System.Speech.Internal.SrgsParser; using System.Text; using System.Xml; namespace System.Speech.Recognition.SrgsGrammar { /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token"]/*' /> // Note that currently if multiple words are stored in a Token they are treated internally // and in the result as multiple tokens. [Serializable] [DebuggerDisplay ("{DebuggerDisplayString ()}")] public class SrgsToken : SrgsElement, IToken { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token.Token2"]/*' /> public SrgsToken (string text) { Helpers.ThrowIfEmptyOrNull (text, "text"); Text = text; } #endregion //******************************************************************** // // Public Properties // //******************************************************************* #region public Properties /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token.Text"]/*' /> public string Text { get { return _text; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); // remove all spaces if any string text = value.Trim (Helpers._achTrimChars); if (string.IsNullOrEmpty (text) || text.IndexOf ('\"') >= 0) { throw new ArgumentException (SR.Get (SRID.InvalidTokenString), "value"); } _text = text; } } /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token.Pronunciation"]/*' /> public string Pronunciation { get { return _pronunciation; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); _pronunciation = value; } } /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token.Display"]/*' /> public string Display { get { return _display; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); _display = value; } } #endregion //******************************************************************** // // Internal methods // //******************************************************************** #region Internal methods internal override void WriteSrgs (XmlWriter writer) { // Write_text writer.WriteStartElement ("token"); if (_display != null) { writer.WriteAttributeString ("sapi", "display", XmlParser.sapiNamespace, _display); } if (_pronunciation != null) { writer.WriteAttributeString ("sapi", "pron", XmlParser.sapiNamespace, _pronunciation); } // If an empty string is provided, skip the WriteString // to have the XmlWrite to putrather than if (_text != null && _text.Length > 0) { writer.WriteString (_text); } writer.WriteEndElement (); } internal override void Validate (SrgsGrammar grammar) { if (_pronunciation != null || _display != null) { grammar.HasPronunciation = true; } // Validate the pronunciation if any if (_pronunciation != null) { for (int iCurPron = 0, iDeliminator = 0; iCurPron < _pronunciation.Length; iCurPron = iDeliminator + 1) { // Find semi-colon deliminator and replace with null iDeliminator = _pronunciation.IndexOf (';', iCurPron); if (iDeliminator == -1) { iDeliminator = _pronunciation.Length; } string subPronunciation = _pronunciation.Substring (iCurPron, iDeliminator - iCurPron); // Convert the pronunciation, will throw if error switch (grammar.PhoneticAlphabet) { case AlphabetType.Sapi: PhonemeConverter.ConvertPronToId (subPronunciation, grammar.Culture.LCID); break; case AlphabetType.Ups: PhonemeConverter.UpsConverter.ConvertPronToId (subPronunciation); break; case AlphabetType.Ipa: PhonemeConverter.ValidateUpsIds (subPronunciation.ToCharArray ()); break; } } } base.Validate (grammar); } internal override string DebuggerDisplayString () { StringBuilder sb = new StringBuilder ("Token '"); sb.Append (_text); sb.Append ("'"); if (_pronunciation != null) { sb.Append (" Pronunciation '"); sb.Append (_pronunciation); sb.Append ("'"); } return sb.ToString (); } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private string _text = string.Empty; private string _pronunciation; private string _display; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
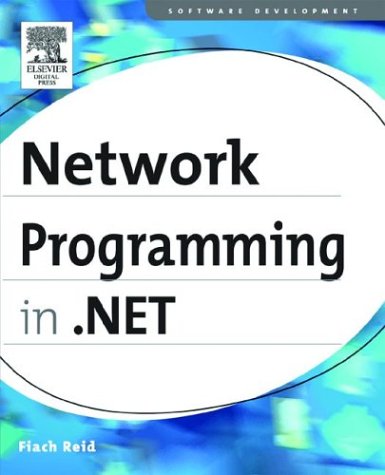
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridTable.cs
- NameScopePropertyAttribute.cs
- WebBrowser.cs
- ProviderSettingsCollection.cs
- BufferedGraphicsContext.cs
- DbConnectionClosed.cs
- TraceHandlerErrorFormatter.cs
- OperandQuery.cs
- DocumentOrderComparer.cs
- XsltException.cs
- HttpSysSettings.cs
- QilStrConcatenator.cs
- PolicyVersionConverter.cs
- MenuBindingsEditorForm.cs
- CodeTryCatchFinallyStatement.cs
- ProcessInputEventArgs.cs
- SafeProcessHandle.cs
- HostingEnvironmentException.cs
- SystemException.cs
- DoneReceivingAsyncResult.cs
- CharacterMetrics.cs
- DataViewManagerListItemTypeDescriptor.cs
- HeaderCollection.cs
- ItemDragEvent.cs
- NavigationPropertyEmitter.cs
- StylusButtonCollection.cs
- DbParameterCollectionHelper.cs
- MemberRelationshipService.cs
- ComAdminWrapper.cs
- MethodExpr.cs
- RowUpdatingEventArgs.cs
- VersionedStream.cs
- ControlBindingsCollection.cs
- VisualStyleRenderer.cs
- Hash.cs
- FrameworkContentElement.cs
- DbConnectionStringBuilder.cs
- KeyValuePair.cs
- IisTraceListener.cs
- ReceiveActivityValidator.cs
- AsymmetricSignatureFormatter.cs
- XmlWriterDelegator.cs
- OrderingInfo.cs
- SoapIgnoreAttribute.cs
- DataGridViewCellMouseEventArgs.cs
- SqlTopReducer.cs
- SchemaCollectionCompiler.cs
- IndexingContentUnit.cs
- SqlServer2KCompatibilityAnnotation.cs
- SecurityStandardsManager.cs
- HostVisual.cs
- SessionStateModule.cs
- InputScopeManager.cs
- Matrix.cs
- Imaging.cs
- Cursors.cs
- TextRunProperties.cs
- InputMethodStateChangeEventArgs.cs
- ServerProtocol.cs
- Scene3D.cs
- DataList.cs
- SmtpNtlmAuthenticationModule.cs
- Int16.cs
- IndentedWriter.cs
- BaseUriHelper.cs
- CodeCommentStatementCollection.cs
- ClientWindowsAuthenticationMembershipProvider.cs
- DataTableReaderListener.cs
- AttributeTableBuilder.cs
- HttpDebugHandler.cs
- CodeSnippetCompileUnit.cs
- HttpCapabilitiesBase.cs
- TcpClientSocketManager.cs
- TemplateControlBuildProvider.cs
- TagPrefixCollection.cs
- IISMapPath.cs
- _FtpControlStream.cs
- ClearTypeHintValidation.cs
- TableLayoutCellPaintEventArgs.cs
- TypeReference.cs
- EntityCommandDefinition.cs
- BookmarkScope.cs
- TextBoxAutomationPeer.cs
- CertificateElement.cs
- COM2FontConverter.cs
- DataGridCaption.cs
- ExceptionHandler.cs
- JournalEntryListConverter.cs
- StartFileNameEditor.cs
- GridEntry.cs
- PnrpPeerResolver.cs
- TableRowsCollectionEditor.cs
- PromptBuilder.cs
- ConfigurationPermission.cs
- CalendarDateRangeChangingEventArgs.cs
- XmlHierarchicalEnumerable.cs
- FactoryMaker.cs
- DataGridViewCellLinkedList.cs
- DataGridComboBoxColumn.cs
- ColorContextHelper.cs