Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / DigitalSignature.cs / 1 / DigitalSignature.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // The DigitalSignature class represents a digital signature or signature // request. // // History: // 05/11/05 - [....] created // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Security.Cryptography.X509Certificates; using System.IO.Packaging; using System.Security; // for SecurityCritical attributes using System.Windows.Xps.Packaging; // for XpsDigitalSignature namespace MS.Internal.Documents { ////// The DigitalSignature class represents a digital signature or signature /// request. /// internal sealed class DigitalSignature { #region Constructor ////// Critical /// - We are marking this critical so that we are guaranteed that all /// callers that construct an instance of this class are in a /// trusted code path. /// [SecurityCritical] internal DigitalSignature() { } #endregion Constructor #region Internal properties ////// Gets or sets the status of signature (Valid, Invalid, NotSigned ...) /// internal SignatureStatus SignatureState { get { return _signatureState.Value; } ////// Critical /// - Writing a critical for set piece of data /// [SecurityCritical] set { _signatureState.Value = value; } } ////// Gets or sets the friendly name of the signer (obtained from the cert. /// internal string SubjectName { get { return _subjectName.Value; } ////// Critical /// - Writing a critical for set piece of data /// [SecurityCritical] set { _subjectName.Value = value; } } ////// Gets or sets the intent (from the Signature Definition) /// internal string Reason { get { return _reason.Value; } ////// Critical /// - Writing a critical for set piece of data /// [SecurityCritical] set { _reason.Value = value; } } ////// Gets or sets when this signature was applied (not a trusted time) /// internal DateTime? SignedOn { get { return _signedOn.Value; } ////// Critical /// - Writing a critical for set piece of data /// [SecurityCritical] set { _signedOn.Value = value; } } ////// Gets or sets the location field (what signer type into signature definition) /// internal string Location { get { return _location.Value; } ////// Critical /// - Writing a critical for set piece of data /// [SecurityCritical] set { _location.Value = value; } } ////// Gets or sets the restriction on document properties /// internal bool IsDocumentPropertiesRestricted { get { return _isDocumentPropertiesRestricted.Value; } ////// Critical /// - Writing a critical for set piece of data /// [SecurityCritical] set { _isDocumentPropertiesRestricted.Value = value; } } ////// Gets or sets whether adding signatures will invalidate this signature /// internal bool IsAddingSignaturesRestricted { get { return _isAddingSignaturesRestricted.Value; } ////// Critical /// - Writing a critical for set piece of data /// [SecurityCritical] set { _isAddingSignaturesRestricted.Value = value; } } ////// Gets or sets the Signature ID /// internal Guid? GuidID { get { return _guidID.Value; } ////// Critical /// - Writing a critical for set piece of data /// [SecurityCritical] set { _guidID.Value = value; } } ////// Gets or sets the X.509 Certificate /// internal X509Certificate2 Certificate { get { return _x509Certificate2.Value; } ////// Critical /// - Writing a critical for set piece of data /// [SecurityCritical] set { _x509Certificate2.Value = value; } } ////// Gets or sets the XpsDigitalSignature associated with this signature. /// This value is expected to be left null for signature requests. /// internal XpsDigitalSignature XpsDigitalSignature { get { return _xpsDigitalSignature.Value; } ////// Critical /// - Writing a critical for set piece of data /// [SecurityCritical] set { _xpsDigitalSignature.Value = value; } } #endregion Internal properties #region Private data ////// Status of signature (Valid, Invalid, NotSigned ...) /// private SecurityCriticalDataForSet_signatureState; /// /// Friendly name of the signer (obtained from the certificate) /// private SecurityCriticalDataForSet_subjectName; /// /// Intent: From the Signature Definition /// private SecurityCriticalDataForSet_reason; /// /// When this signature was applied (not a trusted time) /// private SecurityCriticalDataForSet_signedOn; /// /// Location field (what signer type into signature definition) /// private SecurityCriticalDataForSet_location; /// /// Whether or not document properties changes are restricted by this signature /// private SecurityCriticalDataForSet_isDocumentPropertiesRestricted; /// /// Whether or not adding signatures will invalidate this signature /// private SecurityCriticalDataForSet_isAddingSignaturesRestricted; /// /// SignatureID /// private SecurityCriticalDataForSet_guidID; private SecurityCriticalDataForSet _x509Certificate2; /// /// The XpsDigitalSignature associated with this signature /// private SecurityCriticalDataForSet_xpsDigitalSignature; #endregion Private data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
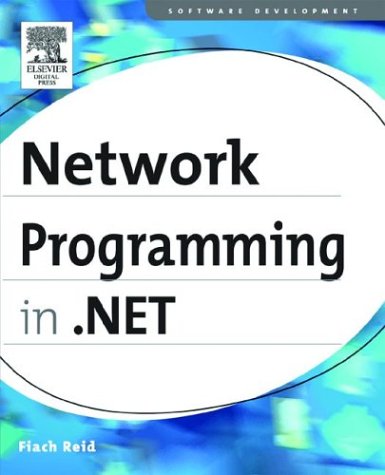
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StructuralObject.cs
- CurrencyWrapper.cs
- DesignerVerbCollection.cs
- WindowsToolbarItemAsMenuItem.cs
- AliasedSlot.cs
- BasePattern.cs
- NotifyInputEventArgs.cs
- NamedPermissionSet.cs
- StateBag.cs
- InternalException.cs
- CssTextWriter.cs
- webeventbuffer.cs
- StaticResourceExtension.cs
- DelegateHelpers.Generated.cs
- RequestQueue.cs
- isolationinterop.cs
- _DisconnectOverlappedAsyncResult.cs
- AsymmetricSecurityProtocol.cs
- CombinedGeometry.cs
- OleDbConnectionInternal.cs
- DragDropHelper.cs
- UserPersonalizationStateInfo.cs
- SystemEvents.cs
- ArgIterator.cs
- NonSerializedAttribute.cs
- Solver.cs
- RIPEMD160.cs
- DrawingGroupDrawingContext.cs
- HtmlEncodedRawTextWriter.cs
- GradientSpreadMethodValidation.cs
- Vars.cs
- XmlTextReaderImplHelpers.cs
- FileCodeGroup.cs
- Focus.cs
- IsolatedStorageFileStream.cs
- FacetChecker.cs
- AccessibleObject.cs
- Util.cs
- AppSecurityManager.cs
- DataGridViewImageCell.cs
- SimpleTextLine.cs
- BooleanConverter.cs
- EmissiveMaterial.cs
- MenuCommand.cs
- StateMachineExecutionState.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- ButtonBaseAdapter.cs
- AppearanceEditorPart.cs
- ToolStripCollectionEditor.cs
- ButtonField.cs
- DataSourceCacheDurationConverter.cs
- ConfigXmlWhitespace.cs
- TableRowCollection.cs
- TraceSwitch.cs
- PropertyStore.cs
- CodeGenerator.cs
- MimeTextImporter.cs
- DataSourceHelper.cs
- PersonalizationStateInfoCollection.cs
- ChangePasswordAutoFormat.cs
- BoundField.cs
- ContextMenuService.cs
- SqlFacetAttribute.cs
- ConfigurationSection.cs
- XmlMemberMapping.cs
- ChangeConflicts.cs
- ActivityCompletionCallbackWrapper.cs
- BmpBitmapDecoder.cs
- WebPartUserCapability.cs
- SqlTypesSchemaImporter.cs
- XsdDuration.cs
- StringSorter.cs
- File.cs
- EmbeddedMailObject.cs
- RelativeSource.cs
- DbBuffer.cs
- RIPEMD160Managed.cs
- SSmlParser.cs
- IisTraceWebEventProvider.cs
- SafeNativeMethodsCLR.cs
- LayoutExceptionEventArgs.cs
- TextEditorParagraphs.cs
- FieldAccessException.cs
- HasCopySemanticsAttribute.cs
- AppDomainUnloadedException.cs
- DynamicPropertyHolder.cs
- followingquery.cs
- ProgressPage.cs
- XmlStreamNodeWriter.cs
- Symbol.cs
- CustomErrorsSection.cs
- XmlSerializerSection.cs
- XmlAnyAttributeAttribute.cs
- FrameDimension.cs
- EntityDataSourceContainerNameConverter.cs
- ListCollectionView.cs
- ConstructorNeedsTagAttribute.cs
- BaseProcessProtocolHandler.cs
- FileNotFoundException.cs
- KeyEvent.cs