Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / ArgIterator.cs / 1 / ArgIterator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System { using System; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; // This class will not be marked serializable [StructLayout(LayoutKind.Auto)] public struct ArgIterator { [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern ArgIterator(IntPtr arglist); // create an arg iterator that points at the first argument that // is not statically declared (that is the first ... arg) // 'arglist' is the value returned by the ARGLIST instruction public ArgIterator(RuntimeArgumentHandle arglist) : this(arglist.Value) { } [MethodImplAttribute(MethodImplOptions.InternalCall)] private unsafe extern ArgIterator(IntPtr arglist, void *ptr); // create an arg iterator that points just past 'firstArg'. // 'arglist' is the value returned by the ARGLIST instruction // This is much like the C va_start macro [CLSCompliant(false)] public unsafe ArgIterator(RuntimeArgumentHandle arglist, void* ptr) : this(arglist.Value, ptr) { } // Fetch an argument as a typed referece, advance the iterator. // Throws an exception if past end of argument list [CLSCompliant(false)] public TypedReference GetNextArg() { TypedReference result = new TypedReference (); // reference to TypedReference is banned, so have to pass result as pointer unsafe { FCallGetNextArg (&result); } return result; } [MethodImplAttribute(MethodImplOptions.InternalCall)] // reference to TypedReference is banned, so have to pass result as void pointer private unsafe extern void FCallGetNextArg(void * result); // Alternate version of GetNextArg() intended primarily for IJW code // generated by VC's "va_arg()" construct. [CLSCompliant(false)] public TypedReference GetNextArg(RuntimeTypeHandle rth) { if (sigPtr != IntPtr.Zero) { // This is an ordinary ArgIterator capable of determining // types from a signature. Just do a regular GetNextArg. return GetNextArg(); } else { // Prevent abuse of this API with a default ArgIterator (it // doesn't require permission to create a zero-inited value // type). Check that ArgPtr isn't zero or this API will allow a // malicious caller to increment the pointer to an arbitrary // location in memory and read the contents. if (ArgPtr == IntPtr.Zero) throw new ArgumentNullException(); TypedReference result = new TypedReference (); // reference to TypedReference is banned, so have to pass result as pointer unsafe { InternalGetNextArg(&result, rth); } return result; } } [MethodImplAttribute(MethodImplOptions.InternalCall)] // reference to TypedReference is banned, so have to pass result as void pointer private unsafe extern void InternalGetNextArg(void * result, RuntimeTypeHandle rth); // This method should invalidate the iterator (va_end). It is not supported yet. public void End() { } // How many arguments are left in the list [MethodImplAttribute(MethodImplOptions.InternalCall)] public extern int GetRemainingCount(); // Gets the type of the current arg, does NOT advance the iterator [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern unsafe void* _GetNextArgType(); public unsafe RuntimeTypeHandle GetNextArgType() { return new RuntimeTypeHandle(_GetNextArgType()); } public override int GetHashCode() { return unchecked((int)((long)ArgCookie)); } // Inherited from object public override bool Equals(Object o) { throw new NotSupportedException(Environment.GetResourceString("NotSupported_NYI")); } private IntPtr ArgCookie; // Cookie from the EE. // The SigPtr structure consists of the following members private IntPtr sigPtr; // Pointer to remaining signature. private IntPtr sigPtrLen; // Remaining length of the pointer // Note, sigPtrLen is actually a DWORD, but on 64bit systems this structure becomes // 8-byte aligned, which requires us to pad it. private IntPtr ArgPtr; // Pointer to remaining args. private int RemainingArgs; // # of remaining args. } }
Link Menu
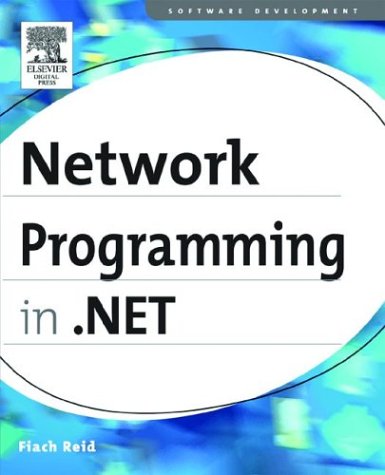
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Vector3DConverter.cs
- ProviderConnectionPointCollection.cs
- storagemappingitemcollection.viewdictionary.cs
- Base64Stream.cs
- WebPartConnectionsConfigureVerb.cs
- Int32CollectionConverter.cs
- KernelTypeValidation.cs
- PropertyChangedEventArgs.cs
- Path.cs
- EDesignUtil.cs
- IsolatedStoragePermission.cs
- FusionWrap.cs
- ConfigurationElement.cs
- ConvertEvent.cs
- ChangeProcessor.cs
- PersistenceException.cs
- SessionEndedEventArgs.cs
- SystemColors.cs
- WSDualHttpBindingElement.cs
- TableColumn.cs
- ConstraintConverter.cs
- WinInet.cs
- ThreadInterruptedException.cs
- TableDetailsRow.cs
- RemoteWebConfigurationHostStream.cs
- AnnotationComponentManager.cs
- SystemIPInterfaceProperties.cs
- CircleHotSpot.cs
- GB18030Encoding.cs
- RootDesignerSerializerAttribute.cs
- ISAPIApplicationHost.cs
- ToolStripDropDownMenu.cs
- ToolStripRendererSwitcher.cs
- PropertyGridDesigner.cs
- FamilyMapCollection.cs
- TableCellAutomationPeer.cs
- SqlCacheDependencyDatabaseCollection.cs
- BasicHttpBindingCollectionElement.cs
- TextModifierScope.cs
- BoundsDrawingContextWalker.cs
- EventWaitHandleSecurity.cs
- InstancePersistenceException.cs
- MsmqActivation.cs
- DataRecordInternal.cs
- EmptyEnumerator.cs
- HostSecurityManager.cs
- WebBrowserPermission.cs
- FeatureSupport.cs
- WindowsImpersonationContext.cs
- Validator.cs
- SizeAnimationClockResource.cs
- TraceListener.cs
- Transform3DGroup.cs
- NativeMethods.cs
- XmlSchemaObject.cs
- Psha1DerivedKeyGenerator.cs
- _CacheStreams.cs
- InvalidComObjectException.cs
- ProcessStartInfo.cs
- BitmapEffectState.cs
- DataQuery.cs
- ApplicationSettingsBase.cs
- DBSchemaRow.cs
- WebConfigurationHost.cs
- DataKeyCollection.cs
- DataGridViewCellStyleChangedEventArgs.cs
- WorkerRequest.cs
- DirtyTextRange.cs
- _UriTypeConverter.cs
- DynamicArgumentDesigner.xaml.cs
- NotSupportedException.cs
- ClassicBorderDecorator.cs
- XPathItem.cs
- DecimalConstantAttribute.cs
- SchemaElementDecl.cs
- NavigationWindowAutomationPeer.cs
- PagesChangedEventArgs.cs
- regiisutil.cs
- ChildTable.cs
- SequenceDesignerAccessibleObject.cs
- WebChannelFactory.cs
- UpdatableWrapper.cs
- TerminatorSinks.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- KeyGestureValueSerializer.cs
- Track.cs
- DragCompletedEventArgs.cs
- PerspectiveCamera.cs
- XPathSingletonIterator.cs
- IDQuery.cs
- DBCommand.cs
- SafeNativeMethods.cs
- ConfigXmlText.cs
- MonitorWrapper.cs
- DrawingVisualDrawingContext.cs
- CultureInfoConverter.cs
- InputReferenceExpression.cs
- InertiaTranslationBehavior.cs
- ValidationPropertyAttribute.cs
- CompiledXpathExpr.cs