Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Security / Cryptography / DESCryptoServiceProvider.cs / 1 / DESCryptoServiceProvider.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // DESCryptoServiceProvider.cs // namespace System.Security.Cryptography { [System.Runtime.InteropServices.ComVisible(true)] public sealed class DESCryptoServiceProvider : DES { // // public constructors // public DESCryptoServiceProvider () { if (!Utils.HasAlgorithm(Constants.CALG_DES, 0)) throw new CryptographicException(Environment.GetResourceString("Cryptography_CSP_AlgorithmNotAvailable")); // Since the CSP only supports a CFB feedback of 8, make that the default FeedbackSizeValue = 8; } // // public methods // public override ICryptoTransform CreateEncryptor (byte[] rgbKey, byte[] rgbIV) { if (IsWeakKey(rgbKey)) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidKey_Weak"),"DES"); if (IsSemiWeakKey(rgbKey)) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidKey_SemiWeak"),"DES"); return _NewEncryptor(rgbKey, ModeValue, rgbIV, FeedbackSizeValue, CryptoAPITransformMode.Encrypt); } public override ICryptoTransform CreateDecryptor (byte[] rgbKey, byte[] rgbIV) { if (IsWeakKey(rgbKey)) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidKey_Weak"),"DES"); if (IsSemiWeakKey(rgbKey)) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidKey_SemiWeak"),"DES"); return _NewEncryptor(rgbKey, ModeValue, rgbIV, FeedbackSizeValue, CryptoAPITransformMode.Decrypt); } public override void GenerateKey () { KeyValue = new byte[8]; Utils.StaticRandomNumberGenerator.GetBytes(KeyValue); // Never hand back a weak or semi-weak key while (DES.IsWeakKey(KeyValue) || DES.IsSemiWeakKey(KeyValue)) { Utils.StaticRandomNumberGenerator.GetBytes(KeyValue); } } public override void GenerateIV () { IVValue = new byte[8]; Utils.StaticRandomNumberGenerator.GetBytes(IVValue); } // // private methods // private ICryptoTransform _NewEncryptor (byte[] rgbKey, CipherMode mode, byte[] rgbIV, int feedbackSize, CryptoAPITransformMode encryptMode) { int cArgs = 0; int[] rgArgIds = new int[10]; Object[] rgArgValues = new Object[10]; // Check for bad values // 1) we don't support OFB mode in DESCryptoServiceProvider if (mode == CipherMode.OFB) throw new CryptographicException(Environment.GetResourceString("Cryptography_CSP_OFBNotSupported")); // 2) we only support CFB with a feedback size of 8 bits if ((mode == CipherMode.CFB) && (feedbackSize != 8)) throw new CryptographicException(Environment.GetResourceString("Cryptography_CSP_CFBSizeNotSupported")); // Build the key if one does not already exist if (rgbKey == null) { rgbKey = new byte[8]; Utils.StaticRandomNumberGenerator.GetBytes(rgbKey); } // Set the mode for the encryptor (defaults to CBC) if (mode != CipherMode.CBC) { rgArgIds[cArgs] = Constants.KP_MODE; rgArgValues[cArgs] = mode; cArgs += 1; } // If not ECB mode -- pass in an IV if (mode != CipherMode.ECB) { if (rgbIV == null) { rgbIV = new byte[8]; Utils.StaticRandomNumberGenerator.GetBytes(rgbIV); } // // We truncate IV's that are longer than the block size to 8 bytes : this is // done to maintain backward compatibility with the behavior shipped in V1.x. // The call to set the IV in CryptoAPI will ignore any bytes after the first 8 // bytes. We'll still reject IV's that are shorter than the block size though. // if (rgbIV.Length < 8) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidIVSize")); rgArgIds[cArgs] = Constants.KP_IV; rgArgValues[cArgs] = rgbIV; cArgs += 1; } // If doing OFB or CFB, then we need to set the feed back loop size if ((mode == CipherMode.OFB) || (mode == CipherMode.CFB)) { rgArgIds[cArgs] = Constants.KP_MODE_BITS; rgArgValues[cArgs] = feedbackSize; cArgs += 1; } // Create the encryptpr/decryptor object return new CryptoAPITransform(Constants.CALG_DES, cArgs, rgArgIds, rgArgValues, rgbKey, PaddingValue, mode, BlockSizeValue, feedbackSize, false, encryptMode); } } }
Link Menu
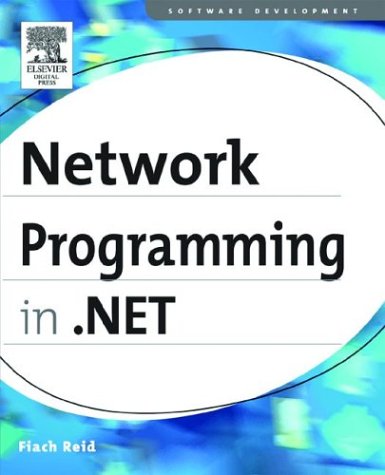
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GenericRootAutomationPeer.cs
- PassportAuthenticationEventArgs.cs
- RuntimeHelpers.cs
- TextSerializer.cs
- TrustSection.cs
- odbcmetadatacolumnnames.cs
- SelectionEditingBehavior.cs
- OleDbConnectionInternal.cs
- ConfigurationValue.cs
- LineServicesCallbacks.cs
- StateManagedCollection.cs
- String.cs
- WeakReferenceKey.cs
- ProfessionalColors.cs
- FillRuleValidation.cs
- BeginEvent.cs
- DataGridViewBand.cs
- MemoryFailPoint.cs
- ToolStripItemTextRenderEventArgs.cs
- ButtonField.cs
- DrawingCollection.cs
- CodeMemberEvent.cs
- SymLanguageType.cs
- PrintDialog.cs
- Serializer.cs
- BufferedGraphicsManager.cs
- XmlWrappingReader.cs
- SecuritySessionSecurityTokenProvider.cs
- HttpRuntimeSection.cs
- EdmEntityTypeAttribute.cs
- SByteStorage.cs
- TabItemWrapperAutomationPeer.cs
- WebPartVerbsEventArgs.cs
- MobileListItem.cs
- ExtenderProvidedPropertyAttribute.cs
- BamlBinaryWriter.cs
- _SingleItemRequestCache.cs
- ContractValidationHelper.cs
- DelegateSerializationHolder.cs
- ObjRef.cs
- RawMouseInputReport.cs
- CssClassPropertyAttribute.cs
- SynchronizationValidator.cs
- Camera.cs
- JoinGraph.cs
- XomlCompiler.cs
- UriSection.cs
- RelOps.cs
- DataGridViewTextBoxCell.cs
- IDQuery.cs
- BaseCAMarshaler.cs
- ListViewSelectEventArgs.cs
- ReliabilityContractAttribute.cs
- BinaryOperationBinder.cs
- XPathException.cs
- SharedPerformanceCounter.cs
- SqlUtils.cs
- Journal.cs
- SupportsEventValidationAttribute.cs
- LabelInfo.cs
- ChangesetResponse.cs
- ProviderConnectionPointCollection.cs
- MergeEnumerator.cs
- Compilation.cs
- DynamicRendererThreadManager.cs
- SchemaLookupTable.cs
- MaskedTextBoxTextEditor.cs
- MenuDesigner.cs
- GraphicsContext.cs
- DataSource.cs
- Bitmap.cs
- Viewport3DVisual.cs
- DockPattern.cs
- NotSupportedException.cs
- coordinator.cs
- ContractValidationHelper.cs
- SortDescriptionCollection.cs
- PathStreamGeometryContext.cs
- SqlBinder.cs
- BitConverter.cs
- ResolvedKeyFrameEntry.cs
- SimpleExpression.cs
- DataGridViewRowConverter.cs
- TypedDataSourceCodeGenerator.cs
- RecognizeCompletedEventArgs.cs
- COM2IProvidePropertyBuilderHandler.cs
- XPathNavigatorReader.cs
- Window.cs
- PointConverter.cs
- XPathDescendantIterator.cs
- DataPagerFieldCollection.cs
- WebEvents.cs
- SafeArrayTypeMismatchException.cs
- UIElementCollection.cs
- TextDecorationUnitValidation.cs
- DoubleConverter.cs
- TableAutomationPeer.cs
- RtfToXamlReader.cs
- Globals.cs
- InfoCardClaim.cs