Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / TypeConstant.cs / 2 / TypeConstant.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Text; using System.Collections.Generic; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Data.Common; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.Structures { // A constant for storing type values, e.g., a type constant is used to // denotes (say) a Person type, Address type, etc. It essentially // encapsulates a CDM Type internal class TypeConstant : CellConstant { #region Constructor // effects: Creates a type constant corresponding to "type" internal TypeConstant(EdmType type) { m_cdmType = type; } #endregion #region Fields // The CDM type denoted by this type constant private EdmType m_cdmType; #endregion #region Properties // effects: Returns the CDM type corresponding to this internal EdmType CdmType { get { return m_cdmType; } } #endregion #region Methods // effects: Given a projectedSlot that contributes to outputMember in // the output extent view, generates a constructor expression for // outputMember's type, i.e, an expression of the form "Type(....)" // If refscope is non-null, instead of constructing an Entity or // Complex type, constructs a reference internal override StringBuilder AsCql(StringBuilder builder, MemberPath outputMember, string blockAlias) { // Gather the fields for a constructor or a Ref expression Listfields = new List (); EntitySet refScope = outputMember.GetScopeOfRelationEnd(); EntityType refEntityType = null; if (refScope != null) { // Construct a scoped reference: CreateRef(CPerson1, row(pid1, pid2)) EntityType entityType = refScope.ElementType; refEntityType = (EntityType)(((RefType)outputMember.EdmType).ElementType); builder.Append("CreateRef("); CqlWriter.AppendEscapedQualifiedName(builder, refScope.EntityContainer.Name, refScope.Name); builder.Append(", row("); foreach (EdmMember keyMember in entityType.KeyMembers) { // Given the member, we need its aliased name MemberPath memberPath = new MemberPath(outputMember, keyMember); string fullFieldAlias = CqlWriter.GetQualifiedName(blockAlias, memberPath.CqlFieldAlias); fields.Add(fullFieldAlias); } } else { // Construct an entity/complex/Association type in the Members order // for fields: CPerson(CPerson1_Pid, CPerson1_Name) // It better be a structural type StructuralType structuralType = (StructuralType)m_cdmType; foreach (EdmMember member in Helper.GetAllStructuralMembers(structuralType)) { // Given the member, we need its aliased name: CPerson1_Pid MemberPath memberPath = new MemberPath(outputMember, member); string fullFieldAlias = CqlWriter.GetQualifiedName(blockAlias, memberPath.CqlFieldAlias); fields.Add(fullFieldAlias); } // Constructor call for the constructedType CqlWriter.AppendEscapedTypeName(builder, m_cdmType); builder.Append('('); } StringUtil.ToSeparatedString(builder, fields, ", ", null); builder.Append(')'); if (refScope != null) { Debug.Assert(refEntityType != null); builder.Append(","); CqlWriter.AppendEscapedTypeName(builder, refEntityType); builder.Append(')'); } return builder; } #endregion #region Public Override Methods for object and String methods protected override bool IsEqualTo(CellConstant right) { TypeConstant rightTypeConstant = right as TypeConstant; if (rightTypeConstant == null) { return false; } return m_cdmType == rightTypeConstant.m_cdmType; } protected override int GetHash() { if (m_cdmType == null) { // null type constant return 0; } else { return m_cdmType.GetHashCode(); } } private void InternalToString(StringBuilder builder, bool isInvariant) { if (m_cdmType == null) { // NULL type constant builder.Append(isInvariant? "NULL": System.Data.Entity.Strings.ViewGen_Null); } else { builder.Append(m_cdmType.Name); } } internal override string ToUserString() { StringBuilder builder = new StringBuilder(); InternalToString(builder, false); return builder.ToString(); } internal override void ToCompactString(StringBuilder builder) { InternalToString(builder, true); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Text; using System.Collections.Generic; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Data.Common; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.Structures { // A constant for storing type values, e.g., a type constant is used to // denotes (say) a Person type, Address type, etc. It essentially // encapsulates a CDM Type internal class TypeConstant : CellConstant { #region Constructor // effects: Creates a type constant corresponding to "type" internal TypeConstant(EdmType type) { m_cdmType = type; } #endregion #region Fields // The CDM type denoted by this type constant private EdmType m_cdmType; #endregion #region Properties // effects: Returns the CDM type corresponding to this internal EdmType CdmType { get { return m_cdmType; } } #endregion #region Methods // effects: Given a projectedSlot that contributes to outputMember in // the output extent view, generates a constructor expression for // outputMember's type, i.e, an expression of the form "Type(....)" // If refscope is non-null, instead of constructing an Entity or // Complex type, constructs a reference internal override StringBuilder AsCql(StringBuilder builder, MemberPath outputMember, string blockAlias) { // Gather the fields for a constructor or a Ref expression Listfields = new List (); EntitySet refScope = outputMember.GetScopeOfRelationEnd(); EntityType refEntityType = null; if (refScope != null) { // Construct a scoped reference: CreateRef(CPerson1, row(pid1, pid2)) EntityType entityType = refScope.ElementType; refEntityType = (EntityType)(((RefType)outputMember.EdmType).ElementType); builder.Append("CreateRef("); CqlWriter.AppendEscapedQualifiedName(builder, refScope.EntityContainer.Name, refScope.Name); builder.Append(", row("); foreach (EdmMember keyMember in entityType.KeyMembers) { // Given the member, we need its aliased name MemberPath memberPath = new MemberPath(outputMember, keyMember); string fullFieldAlias = CqlWriter.GetQualifiedName(blockAlias, memberPath.CqlFieldAlias); fields.Add(fullFieldAlias); } } else { // Construct an entity/complex/Association type in the Members order // for fields: CPerson(CPerson1_Pid, CPerson1_Name) // It better be a structural type StructuralType structuralType = (StructuralType)m_cdmType; foreach (EdmMember member in Helper.GetAllStructuralMembers(structuralType)) { // Given the member, we need its aliased name: CPerson1_Pid MemberPath memberPath = new MemberPath(outputMember, member); string fullFieldAlias = CqlWriter.GetQualifiedName(blockAlias, memberPath.CqlFieldAlias); fields.Add(fullFieldAlias); } // Constructor call for the constructedType CqlWriter.AppendEscapedTypeName(builder, m_cdmType); builder.Append('('); } StringUtil.ToSeparatedString(builder, fields, ", ", null); builder.Append(')'); if (refScope != null) { Debug.Assert(refEntityType != null); builder.Append(","); CqlWriter.AppendEscapedTypeName(builder, refEntityType); builder.Append(')'); } return builder; } #endregion #region Public Override Methods for object and String methods protected override bool IsEqualTo(CellConstant right) { TypeConstant rightTypeConstant = right as TypeConstant; if (rightTypeConstant == null) { return false; } return m_cdmType == rightTypeConstant.m_cdmType; } protected override int GetHash() { if (m_cdmType == null) { // null type constant return 0; } else { return m_cdmType.GetHashCode(); } } private void InternalToString(StringBuilder builder, bool isInvariant) { if (m_cdmType == null) { // NULL type constant builder.Append(isInvariant? "NULL": System.Data.Entity.Strings.ViewGen_Null); } else { builder.Append(m_cdmType.Name); } } internal override string ToUserString() { StringBuilder builder = new StringBuilder(); InternalToString(builder, false); return builder.ToString(); } internal override void ToCompactString(StringBuilder builder) { InternalToString(builder, true); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
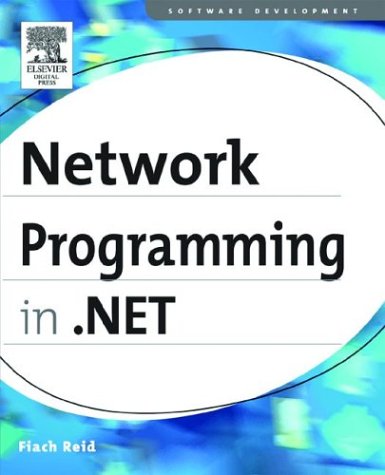
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyMetadata.cs
- SecurityKeyUsage.cs
- PermissionRequestEvidence.cs
- OdbcParameterCollection.cs
- SizeValueSerializer.cs
- DataGridAddNewRow.cs
- DataExpression.cs
- SafeFileMappingHandle.cs
- EventHandlerList.cs
- FutureFactory.cs
- Speller.cs
- PropertyAccessVisitor.cs
- MobileSysDescriptionAttribute.cs
- DocumentPage.cs
- SpanIndex.cs
- DependencyPropertyAttribute.cs
- ClientRuntimeConfig.cs
- CustomTypeDescriptor.cs
- BooleanAnimationUsingKeyFrames.cs
- FixedSOMTableRow.cs
- StorageEntityTypeMapping.cs
- COM2EnumConverter.cs
- MultipleViewPatternIdentifiers.cs
- PartialCachingAttribute.cs
- AttributeSetAction.cs
- X509InitiatorCertificateServiceElement.cs
- ExecutionContext.cs
- SharedPerformanceCounter.cs
- HtmlMeta.cs
- ClientFormsIdentity.cs
- DetailsViewModeEventArgs.cs
- BaseTemplateBuildProvider.cs
- Int32RectValueSerializer.cs
- WebConfigurationManager.cs
- XamlBrushSerializer.cs
- FormParameter.cs
- sqlnorm.cs
- Duration.cs
- UrlMappingsSection.cs
- DefaultPropertyAttribute.cs
- PriorityItem.cs
- HostedHttpRequestAsyncResult.cs
- TimeSpanValidator.cs
- SqlCacheDependencyDatabaseCollection.cs
- HttpCapabilitiesEvaluator.cs
- CompressedStack.cs
- TextDecorationCollection.cs
- AttributeQuery.cs
- DoubleLinkList.cs
- SharedPersonalizationStateInfo.cs
- XmlChoiceIdentifierAttribute.cs
- SinglePhaseEnlistment.cs
- RSAOAEPKeyExchangeDeformatter.cs
- Variant.cs
- PermissionToken.cs
- x509store.cs
- RequestCacheManager.cs
- ProfileSection.cs
- CodeValidator.cs
- WorkItem.cs
- CustomValidator.cs
- PersonalizationProvider.cs
- HtmlInputControl.cs
- UpdateCommand.cs
- XamlStream.cs
- FlowDocumentReaderAutomationPeer.cs
- RepeaterItemEventArgs.cs
- UnsafeNativeMethodsMilCoreApi.cs
- SinglePageViewer.cs
- PeerNameRecordCollection.cs
- CollectionConverter.cs
- XPathItem.cs
- FileDialog.cs
- XD.cs
- DiscoveryDocument.cs
- ConstNode.cs
- DataStreamFromComStream.cs
- ReadContentAsBinaryHelper.cs
- HashCoreRequest.cs
- ResumeStoryboard.cs
- EditorZoneBase.cs
- _DomainName.cs
- Speller.cs
- FixedDocument.cs
- WebPartEventArgs.cs
- SurrogateSelector.cs
- RightsManagementEncryptionTransform.cs
- SQLInt32Storage.cs
- XomlDesignerLoader.cs
- DbConnectionPoolGroupProviderInfo.cs
- RowToFieldTransformer.cs
- ArrangedElementCollection.cs
- CompoundFileStorageReference.cs
- XhtmlConformanceSection.cs
- ReachDocumentReferenceCollectionSerializerAsync.cs
- ConcurrentStack.cs
- VisualBrush.cs
- DefaultBindingPropertyAttribute.cs
- SchemaCollectionPreprocessor.cs
- PropertyInformationCollection.cs