Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / xsp / System / Web / Extensions / ApplicationServices / RoleService.cs / 1 / RoleService.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.ApplicationServices { using System; using System.Diagnostics.CodeAnalysis; using System.ServiceModel; using System.ServiceModel.Activation; using System.ServiceModel.Configuration; using System.Runtime.Serialization; using System.Web; using System.Web.Security; using System.Web.Configuration; using System.Web.Management; using System.Web.Resources; using System.Security.Principal; using System.Web.Hosting; using System.Threading; using System.Configuration.Provider; using System.Security.Permissions; [ AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Required), AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal), ServiceContract(Namespace = "http://asp.net/ApplicationServices/v200"), ServiceBehavior(Namespace="http://asp.net/ApplicationServices/v200", InstanceContextMode = InstanceContextMode.Single, ConcurrencyMode = ConcurrencyMode.Multiple) ] public class RoleService { private static object _selectingProviderEventHandlerLock = new object(); private static EventHandler_selectingProvider; public static event EventHandler SelectingProvider { add { lock (_selectingProviderEventHandlerLock) { _selectingProvider += value; } } remove { lock (_selectingProviderEventHandlerLock) { _selectingProvider -= value; } } } private static void EnsureProviderEnabled() { if (!Roles.Enabled) { throw new ProviderException(AtlasWeb.RoleService_RolesFeatureNotEnabled); } } private RoleProvider GetRoleProvider(IPrincipal user) { string providerName = Roles.Provider.Name; SelectingProviderEventArgs args = new SelectingProviderEventArgs(user, providerName); OnSelectingProvider(args); providerName = args.ProviderName; RoleProvider provider = Roles.Providers[providerName]; if (provider == null) { throw new ProviderException(AtlasWeb.RoleService_RoleProviderNotFound); } return provider; } [OperationContract] public string[] GetRolesForCurrentUser() { try { ApplicationServiceHelper.EnsureRoleServiceEnabled(); EnsureProviderEnabled(); IPrincipal user = ApplicationServiceHelper.GetCurrentUser(HttpContext.Current); string username = ApplicationServiceHelper.GetUserName(user); RoleProvider provider = GetRoleProvider(user); return provider.GetRolesForUser(username); } catch (Exception e) { LogException(e); throw; } } [OperationContract] public bool IsCurrentUserInRole(string role) { if (role == null) { throw new ArgumentNullException("role"); } try { ApplicationServiceHelper.EnsureRoleServiceEnabled(); EnsureProviderEnabled(); IPrincipal user = ApplicationServiceHelper.GetCurrentUser(HttpContext.Current); string username = ApplicationServiceHelper.GetUserName(user); RoleProvider provider = GetRoleProvider(user); return provider.IsUserInRole(username, role); } catch (Exception e) { LogException(e); throw; } } private void LogException(Exception e) { WebServiceErrorEvent errorevent = new WebServiceErrorEvent(AtlasWeb.UnhandledExceptionEventLogMessage, this, e); errorevent.Raise(); } private void OnSelectingProvider(SelectingProviderEventArgs e) { EventHandler handler = _selectingProvider; if (handler != null) { handler(this, e); } } //hiding public constructor internal RoleService() { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.ApplicationServices { using System; using System.Diagnostics.CodeAnalysis; using System.ServiceModel; using System.ServiceModel.Activation; using System.ServiceModel.Configuration; using System.Runtime.Serialization; using System.Web; using System.Web.Security; using System.Web.Configuration; using System.Web.Management; using System.Web.Resources; using System.Security.Principal; using System.Web.Hosting; using System.Threading; using System.Configuration.Provider; using System.Security.Permissions; [ AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Required), AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal), ServiceContract(Namespace = "http://asp.net/ApplicationServices/v200"), ServiceBehavior(Namespace="http://asp.net/ApplicationServices/v200", InstanceContextMode = InstanceContextMode.Single, ConcurrencyMode = ConcurrencyMode.Multiple) ] public class RoleService { private static object _selectingProviderEventHandlerLock = new object(); private static EventHandler_selectingProvider; public static event EventHandler SelectingProvider { add { lock (_selectingProviderEventHandlerLock) { _selectingProvider += value; } } remove { lock (_selectingProviderEventHandlerLock) { _selectingProvider -= value; } } } private static void EnsureProviderEnabled() { if (!Roles.Enabled) { throw new ProviderException(AtlasWeb.RoleService_RolesFeatureNotEnabled); } } private RoleProvider GetRoleProvider(IPrincipal user) { string providerName = Roles.Provider.Name; SelectingProviderEventArgs args = new SelectingProviderEventArgs(user, providerName); OnSelectingProvider(args); providerName = args.ProviderName; RoleProvider provider = Roles.Providers[providerName]; if (provider == null) { throw new ProviderException(AtlasWeb.RoleService_RoleProviderNotFound); } return provider; } [OperationContract] public string[] GetRolesForCurrentUser() { try { ApplicationServiceHelper.EnsureRoleServiceEnabled(); EnsureProviderEnabled(); IPrincipal user = ApplicationServiceHelper.GetCurrentUser(HttpContext.Current); string username = ApplicationServiceHelper.GetUserName(user); RoleProvider provider = GetRoleProvider(user); return provider.GetRolesForUser(username); } catch (Exception e) { LogException(e); throw; } } [OperationContract] public bool IsCurrentUserInRole(string role) { if (role == null) { throw new ArgumentNullException("role"); } try { ApplicationServiceHelper.EnsureRoleServiceEnabled(); EnsureProviderEnabled(); IPrincipal user = ApplicationServiceHelper.GetCurrentUser(HttpContext.Current); string username = ApplicationServiceHelper.GetUserName(user); RoleProvider provider = GetRoleProvider(user); return provider.IsUserInRole(username, role); } catch (Exception e) { LogException(e); throw; } } private void LogException(Exception e) { WebServiceErrorEvent errorevent = new WebServiceErrorEvent(AtlasWeb.UnhandledExceptionEventLogMessage, this, e); errorevent.Raise(); } private void OnSelectingProvider(SelectingProviderEventArgs e) { EventHandler handler = _selectingProvider; if (handler != null) { handler(this, e); } } //hiding public constructor internal RoleService() { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
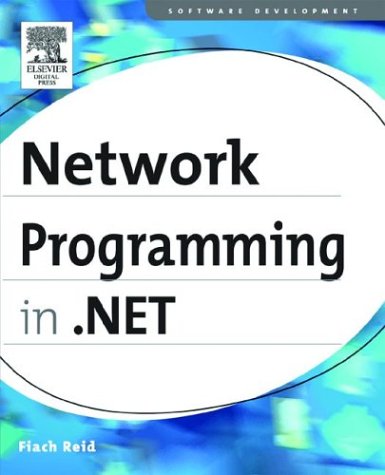
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LogExtentCollection.cs
- RuleElement.cs
- SettingsBindableAttribute.cs
- ReaderOutput.cs
- SQLMembershipProvider.cs
- SettingsPropertyNotFoundException.cs
- FontDriver.cs
- ComponentChangingEvent.cs
- UDPClient.cs
- Group.cs
- RefreshPropertiesAttribute.cs
- JsonFormatReaderGenerator.cs
- SemaphoreSecurity.cs
- DataControlPagerLinkButton.cs
- SqlRowUpdatedEvent.cs
- BitmapFrame.cs
- SystemException.cs
- ReferenceSchema.cs
- InfocardChannelParameter.cs
- TreeView.cs
- SmtpMail.cs
- TraceXPathNavigator.cs
- FolderLevelBuildProvider.cs
- ShaperBuffers.cs
- TransformValueSerializer.cs
- RepeatInfo.cs
- IteratorFilter.cs
- OSEnvironmentHelper.cs
- Attachment.cs
- DbMetaDataFactory.cs
- BasicExpressionVisitor.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- httpstaticobjectscollection.cs
- Span.cs
- MouseButton.cs
- RouteItem.cs
- SerializationFieldInfo.cs
- CacheRequest.cs
- ConvertersCollection.cs
- EdmFunctionAttribute.cs
- WebAdminConfigurationHelper.cs
- PageParserFilter.cs
- SchemaCollectionCompiler.cs
- RadioButtonBaseAdapter.cs
- CorrelationManager.cs
- ColumnMap.cs
- MenuItemBinding.cs
- CompilationUtil.cs
- SBCSCodePageEncoding.cs
- UserNamePasswordValidator.cs
- DbParameterHelper.cs
- ControlPersister.cs
- EntityParameter.cs
- ImageIndexConverter.cs
- followingquery.cs
- BaseServiceProvider.cs
- OperandQuery.cs
- NativeMethods.cs
- InvocationExpression.cs
- DataGrid.cs
- PageContentAsyncResult.cs
- XmlHierarchicalEnumerable.cs
- DateTimeFormatInfoScanner.cs
- NativeActivityAbortContext.cs
- AttributeCollection.cs
- SiteMapNodeItem.cs
- XmlBufferedByteStreamReader.cs
- SimpleRecyclingCache.cs
- ReferentialConstraint.cs
- MasterPage.cs
- Attributes.cs
- BindingSourceDesigner.cs
- ProvidersHelper.cs
- DockPatternIdentifiers.cs
- HwndTarget.cs
- HttpProcessUtility.cs
- XmlSchemaSet.cs
- ThreadBehavior.cs
- Executor.cs
- CommandLibraryHelper.cs
- arc.cs
- WebPartManager.cs
- brushes.cs
- FixedPageStructure.cs
- Events.cs
- ImportContext.cs
- DataSourceControl.cs
- WsatTransactionHeader.cs
- TabControlDesigner.cs
- DependencyPropertyConverter.cs
- ScopelessEnumAttribute.cs
- InvalidDataContractException.cs
- securitycriticaldataClass.cs
- MatrixConverter.cs
- IndentedWriter.cs
- Set.cs
- XmlReaderSettings.cs
- GridEntryCollection.cs
- ConfigurationPropertyAttribute.cs
- JavascriptCallbackResponseProperty.cs