Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / RoutedEvent.cs / 1 / RoutedEvent.cs
using System; using System.ComponentModel; using System.Windows.Markup; using System.Globalization; namespace System.Windows { ////// RoutedEvent is a unique identifier for /// any registered RoutedEvent /// ////// RoutedEvent constitutes the ////// , /// , /// and /// /// /// /// NOTE: None of the members can be null /// [TypeConverter("System.Windows.Markup.RoutedEventConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [ValueSerializer("System.Windows.Markup.RoutedEventValueSerializer, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] public sealed class RoutedEvent { #region External API /// /// Associate another owner type with this event. /// ////// The owner type is used when resolving an event by name. /// /// Additional owner type ///This event. public RoutedEvent AddOwner(Type ownerType) { GlobalEventManager.AddOwner(this, ownerType); return this; } ////// Returns the Name of the RoutedEvent /// ////// RoutedEvent Name is unique within the /// OwnerType (super class types not considered /// when talking about uniqueness) /// ///public string Name { get {return _name;} } /// /// Returns the ////// of the RoutedEvent /// public RoutingStrategy RoutingStrategy { get {return _routingStrategy;} } /// /// Returns Type of Handler for the RoutedEvent /// ////// HandlerType is a type of delegate /// ///public Type HandlerType { get {return _handlerType;} } // Check to see if the given delegate is a legal handler for this type. // It either needs to be a type that the registering class knows how to // handle, or a RoutedEventHandler which we can handle without the help // of the registering class. internal bool IsLegalHandler( Delegate handler ) { Type handlerType = handler.GetType(); return ( (handlerType == HandlerType) || (handlerType == typeof(RoutedEventHandler) ) ); } /// /// Returns Type of Owner for the RoutedEvent /// ////// OwnerType is any object type /// ///public Type OwnerType { get {return _ownerType;} } /// /// String representation /// public override string ToString() { return string.Format(CultureInfo.InvariantCulture, "{0}.{1}", _ownerType.Name, _name ); } #endregion External API #region Construction // Constructor for a RoutedEvent (is internal // to the EventManager and is onvoked when a new // RoutedEvent is registered) internal RoutedEvent( string name, RoutingStrategy routingStrategy, Type handlerType, Type ownerType) { _name = name; _routingStrategy = routingStrategy; _handlerType = handlerType; _ownerType = ownerType; _globalIndex = GlobalEventManager.GetNextAvailableGlobalIndex(this); } ////// Index in GlobalEventManager /// internal int GlobalIndex { get { return _globalIndex; } } #endregion Construction #region Data private string _name; private RoutingStrategy _routingStrategy; private Type _handlerType; private Type _ownerType; private int _globalIndex; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.ComponentModel; using System.Windows.Markup; using System.Globalization; namespace System.Windows { ////// RoutedEvent is a unique identifier for /// any registered RoutedEvent /// ////// RoutedEvent constitutes the ////// , /// , /// and /// /// /// /// NOTE: None of the members can be null /// [TypeConverter("System.Windows.Markup.RoutedEventConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [ValueSerializer("System.Windows.Markup.RoutedEventValueSerializer, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] public sealed class RoutedEvent { #region External API /// /// Associate another owner type with this event. /// ////// The owner type is used when resolving an event by name. /// /// Additional owner type ///This event. public RoutedEvent AddOwner(Type ownerType) { GlobalEventManager.AddOwner(this, ownerType); return this; } ////// Returns the Name of the RoutedEvent /// ////// RoutedEvent Name is unique within the /// OwnerType (super class types not considered /// when talking about uniqueness) /// ///public string Name { get {return _name;} } /// /// Returns the ////// of the RoutedEvent /// public RoutingStrategy RoutingStrategy { get {return _routingStrategy;} } /// /// Returns Type of Handler for the RoutedEvent /// ////// HandlerType is a type of delegate /// ///public Type HandlerType { get {return _handlerType;} } // Check to see if the given delegate is a legal handler for this type. // It either needs to be a type that the registering class knows how to // handle, or a RoutedEventHandler which we can handle without the help // of the registering class. internal bool IsLegalHandler( Delegate handler ) { Type handlerType = handler.GetType(); return ( (handlerType == HandlerType) || (handlerType == typeof(RoutedEventHandler) ) ); } /// /// Returns Type of Owner for the RoutedEvent /// ////// OwnerType is any object type /// ///public Type OwnerType { get {return _ownerType;} } /// /// String representation /// public override string ToString() { return string.Format(CultureInfo.InvariantCulture, "{0}.{1}", _ownerType.Name, _name ); } #endregion External API #region Construction // Constructor for a RoutedEvent (is internal // to the EventManager and is onvoked when a new // RoutedEvent is registered) internal RoutedEvent( string name, RoutingStrategy routingStrategy, Type handlerType, Type ownerType) { _name = name; _routingStrategy = routingStrategy; _handlerType = handlerType; _ownerType = ownerType; _globalIndex = GlobalEventManager.GetNextAvailableGlobalIndex(this); } ////// Index in GlobalEventManager /// internal int GlobalIndex { get { return _globalIndex; } } #endregion Construction #region Data private string _name; private RoutingStrategy _routingStrategy; private Type _handlerType; private Type _ownerType; private int _globalIndex; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
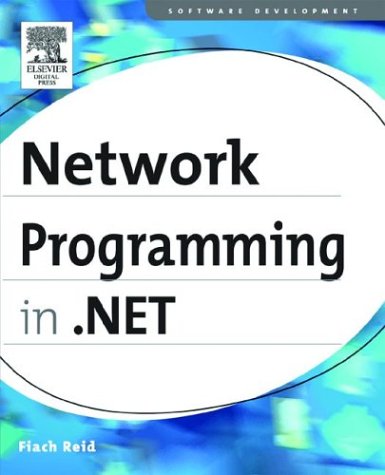
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MappingException.cs
- FlowDocumentFormatter.cs
- CollectionBuilder.cs
- XmlSchemaDatatype.cs
- CodeAttributeArgument.cs
- PolicyStatement.cs
- BinaryWriter.cs
- grammarelement.cs
- SQlBooleanStorage.cs
- EntityDataSourceStatementEditorForm.cs
- BuildProvider.cs
- RequestTimeoutManager.cs
- Freezable.cs
- TextProperties.cs
- _FixedSizeReader.cs
- CompressEmulationStream.cs
- EventlogProvider.cs
- MemberInfoSerializationHolder.cs
- XmlWriterSettings.cs
- Span.cs
- CursorInteropHelper.cs
- PrtCap_Public.cs
- DataServiceQuery.cs
- DefaultTypeArgumentAttribute.cs
- TypeSystemProvider.cs
- HtmlFormParameterReader.cs
- NetMsmqBindingCollectionElement.cs
- TypedElement.cs
- QueryStringParameter.cs
- GridViewRowPresenter.cs
- DataGridViewColumnConverter.cs
- WebConfigurationHost.cs
- MenuCommands.cs
- GeometryGroup.cs
- ServiceBuildProvider.cs
- AppSettings.cs
- DllHostedComPlusServiceHost.cs
- CommandHelper.cs
- View.cs
- DataStreams.cs
- Range.cs
- ISAPIWorkerRequest.cs
- WorkflowOperationAsyncResult.cs
- AppSettingsSection.cs
- XmlIgnoreAttribute.cs
- ThrowHelper.cs
- ToolStripStatusLabel.cs
- CrossAppDomainChannel.cs
- RoutedCommand.cs
- RequestCachePolicy.cs
- CaseStatement.cs
- SecurityKeyType.cs
- RowCache.cs
- SmtpFailedRecipientsException.cs
- AsnEncodedData.cs
- QilLoop.cs
- SoapHttpTransportImporter.cs
- BaseInfoTable.cs
- GroupAggregateExpr.cs
- ConfigXmlText.cs
- Hyperlink.cs
- NavigatorOutput.cs
- AppliesToBehaviorDecisionTable.cs
- DataGridViewUtilities.cs
- XmlArrayItemAttributes.cs
- TextHidden.cs
- DesignerCommandSet.cs
- SizeAnimationBase.cs
- ArrayWithOffset.cs
- ProtectedConfiguration.cs
- recordstate.cs
- Message.cs
- MouseGesture.cs
- Control.cs
- SchemaTypeEmitter.cs
- SqlInternalConnectionSmi.cs
- SmtpAuthenticationManager.cs
- ArgumentOutOfRangeException.cs
- DBBindings.cs
- RefExpr.cs
- IisTraceListener.cs
- Literal.cs
- MessageQueueKey.cs
- XmlUtil.cs
- DiscoveryProxy.cs
- EventLogPermissionEntry.cs
- MatrixCamera.cs
- PropertyDescriptorGridEntry.cs
- DependencyProperty.cs
- UntypedNullExpression.cs
- SafeMarshalContext.cs
- CapiHashAlgorithm.cs
- AnnotationAdorner.cs
- Pair.cs
- ObjectNavigationPropertyMapping.cs
- ToolStrip.cs
- NumericExpr.cs
- GenerateHelper.cs
- HtmlInputReset.cs
- CodeSubDirectoriesCollection.cs