Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / PtsHost / UIElementParaClient.cs / 1 / UIElementParaClient.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: UIElementParaClient class is responsible for handling display // related data of BlockUIContainers. // //--------------------------------------------------------------------------- using System; // IntPtr using System.Collections.Generic; // Listusing System.Collections.ObjectModel; // ReadOnlyCollection using System.Security; // SecurityCritical using System.Windows; // FrameworkElement using System.Windows.Media; // Visual using System.Windows.Documents; // BlockUIContainer using MS.Internal.Documents; // ParagraphResult, UIElementIsland using MS.Internal.Text; // TextDpi using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS namespace MS.Internal.PtsHost { /// /// FloaterParaClient class is responsible for handling display related /// data of paragraphs associated with nested floaters. /// internal sealed class UIElementParaClient : FloaterBaseParaClient { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Constructor. /// /// internal UIElementParaClient(FloaterBaseParagraph paragraph) : base(paragraph) { } #endregion Constructors //-------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods ////// Arrange paragraph. /// ////// Critical - as this calls Critical function PTS.FsQueryFloaterDetails /// Safe - The IntPtr parameters passed to PTS.FsQueryFloaterDetails are SecurityCriticalDataForSet /// which ensures that partial trust code won't be able to set it to a random value. /// The textdetails parameter passed to other methods is generated securely in this function. /// [SecurityCritical, SecurityTreatAsSafe] protected override void OnArrange() { base.OnArrange(); PTS.FSFLOATERDETAILS floaterDetails; PTS.Validate(PTS.FsQueryFloaterDetails(PtsContext.Context, _paraHandle.Value, out floaterDetails)); // Get paragraph's rectangle. _rect = floaterDetails.fsrcFloater; MbpInfo mbp = MbpInfo.FromElement(Paragraph.Element); if(ParentFlowDirection != PageFlowDirection) { mbp.MirrorMargin(); PTS.FSRECT pageRect = _pageContext.PageRect; PTS.Validate(PTS.FsTransformRectangle(PTS.FlowDirectionToFswdir(ParentFlowDirection), ref pageRect, ref _rect, PTS.FlowDirectionToFswdir(PageFlowDirection), out _rect)); } _rect.u += mbp.MarginLeft; _rect.du -= mbp.MarginLeft + mbp.MarginRight; _rect.du = Math.Max(TextDpi.ToTextDpi(TextDpi.MinWidth), _rect.du); _rect.dv = Math.Max(TextDpi.ToTextDpi(TextDpi.MinWidth), _rect.dv); } ////// Returns collection of rectangles for the BlockUIContainer element. /// If the element is not the paragraph's owner, empty collection is returned. /// internal override ListGetRectangles(ContentElement e, int start, int length) { List rectangles = new List (); if (Paragraph.Element == e) { // We have found the element. Return rectangles for this paragraph. GetRectanglesForParagraphElement(out rectangles); } return rectangles; } /// /// Validates visual node associated with paragraph. /// internal override void ValidateVisual(PTS.FSKUPDATE fskupdInherited) { // Obtain all mbd info MbpInfo mbp = MbpInfo.FromElement(Paragraph.Element); // MIRROR entire element to interface with underlying layout tree. // Border/Padding does not need to be mirrored, as it'll be mirrored with the content. PtsHelper.UpdateMirroringTransform(PageFlowDirection, ThisFlowDirection, _visual, TextDpi.FromTextDpi(2 * _rect.u + _rect.du)); // Add UIElementIsland to visual tree and set appropiate offset. UIElementIsland uiElementIsland = ((UIElementParagraph)Paragraph).UIElementIsland; if (uiElementIsland != null) { if (_visual.Children.Count != 1 || _visual.Children[0] != uiElementIsland) { // Disconnect UIElementIsland from its old parent. Visual currentParent = VisualTreeHelper.GetParent(uiElementIsland) as Visual; if (currentParent != null) { ContainerVisual parent = currentParent as ContainerVisual; Invariant.Assert(parent != null, "Parent should always derives from ContainerVisual."); parent.Children.Remove(uiElementIsland); } _visual.Children.Clear(); _visual.Children.Add(uiElementIsland); } uiElementIsland.Offset = new PTS.FSVECTOR(_rect.u + mbp.BPLeft, _rect.v + mbp.BPTop).FromTextDpi(); } else { _visual.Children.Clear(); } // Draw background and borders. Brush backgroundBrush = (Brush)Paragraph.Element.GetValue(TextElement.BackgroundProperty); _visual.DrawBackgroundAndBorder(backgroundBrush, mbp.BorderBrush, mbp.Border, _rect.FromTextDpi(), IsFirstChunk, IsLastChunk); } ////// Returns tight bounding path geometry. /// internal Geometry GetTightBoundingGeometryFromTextPositions(ITextPointer startPosition, ITextPointer endPosition) { if (startPosition.CompareTo(((BlockUIContainer)Paragraph.Element).ContentEnd) < 0 && endPosition.CompareTo(((BlockUIContainer)Paragraph.Element).ContentStart) > 0) { return new RectangleGeometry(_rect.FromTextDpi()); } return null; } ////// Creates paragraph result representing this paragraph. /// ///internal override ParagraphResult CreateParagraphResult() { return new UIElementParagraphResult(this); } /// /// Hit tests to the correct IInputElement within the paragraph that /// the mouse is over. /// internal override IInputElement InputHitTest(PTS.FSPOINT pt) { if (_rect.Contains(pt)) { return Paragraph.Element as IInputElement; } return null; } ////// Returns TextContentRange for the content of the paragraph. /// internal override TextContentRange GetTextContentRange() { BlockUIContainer elementOwner = (BlockUIContainer)Paragraph.Element; return TextContainerHelper.GetTextContentRangeForTextElement(elementOwner); } #endregion Internal Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: UIElementParaClient class is responsible for handling display // related data of BlockUIContainers. // //--------------------------------------------------------------------------- using System; // IntPtr using System.Collections.Generic; // Listusing System.Collections.ObjectModel; // ReadOnlyCollection using System.Security; // SecurityCritical using System.Windows; // FrameworkElement using System.Windows.Media; // Visual using System.Windows.Documents; // BlockUIContainer using MS.Internal.Documents; // ParagraphResult, UIElementIsland using MS.Internal.Text; // TextDpi using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS namespace MS.Internal.PtsHost { /// /// FloaterParaClient class is responsible for handling display related /// data of paragraphs associated with nested floaters. /// internal sealed class UIElementParaClient : FloaterBaseParaClient { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Constructor. /// /// internal UIElementParaClient(FloaterBaseParagraph paragraph) : base(paragraph) { } #endregion Constructors //-------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods ////// Arrange paragraph. /// ////// Critical - as this calls Critical function PTS.FsQueryFloaterDetails /// Safe - The IntPtr parameters passed to PTS.FsQueryFloaterDetails are SecurityCriticalDataForSet /// which ensures that partial trust code won't be able to set it to a random value. /// The textdetails parameter passed to other methods is generated securely in this function. /// [SecurityCritical, SecurityTreatAsSafe] protected override void OnArrange() { base.OnArrange(); PTS.FSFLOATERDETAILS floaterDetails; PTS.Validate(PTS.FsQueryFloaterDetails(PtsContext.Context, _paraHandle.Value, out floaterDetails)); // Get paragraph's rectangle. _rect = floaterDetails.fsrcFloater; MbpInfo mbp = MbpInfo.FromElement(Paragraph.Element); if(ParentFlowDirection != PageFlowDirection) { mbp.MirrorMargin(); PTS.FSRECT pageRect = _pageContext.PageRect; PTS.Validate(PTS.FsTransformRectangle(PTS.FlowDirectionToFswdir(ParentFlowDirection), ref pageRect, ref _rect, PTS.FlowDirectionToFswdir(PageFlowDirection), out _rect)); } _rect.u += mbp.MarginLeft; _rect.du -= mbp.MarginLeft + mbp.MarginRight; _rect.du = Math.Max(TextDpi.ToTextDpi(TextDpi.MinWidth), _rect.du); _rect.dv = Math.Max(TextDpi.ToTextDpi(TextDpi.MinWidth), _rect.dv); } ////// Returns collection of rectangles for the BlockUIContainer element. /// If the element is not the paragraph's owner, empty collection is returned. /// internal override ListGetRectangles(ContentElement e, int start, int length) { List rectangles = new List (); if (Paragraph.Element == e) { // We have found the element. Return rectangles for this paragraph. GetRectanglesForParagraphElement(out rectangles); } return rectangles; } /// /// Validates visual node associated with paragraph. /// internal override void ValidateVisual(PTS.FSKUPDATE fskupdInherited) { // Obtain all mbd info MbpInfo mbp = MbpInfo.FromElement(Paragraph.Element); // MIRROR entire element to interface with underlying layout tree. // Border/Padding does not need to be mirrored, as it'll be mirrored with the content. PtsHelper.UpdateMirroringTransform(PageFlowDirection, ThisFlowDirection, _visual, TextDpi.FromTextDpi(2 * _rect.u + _rect.du)); // Add UIElementIsland to visual tree and set appropiate offset. UIElementIsland uiElementIsland = ((UIElementParagraph)Paragraph).UIElementIsland; if (uiElementIsland != null) { if (_visual.Children.Count != 1 || _visual.Children[0] != uiElementIsland) { // Disconnect UIElementIsland from its old parent. Visual currentParent = VisualTreeHelper.GetParent(uiElementIsland) as Visual; if (currentParent != null) { ContainerVisual parent = currentParent as ContainerVisual; Invariant.Assert(parent != null, "Parent should always derives from ContainerVisual."); parent.Children.Remove(uiElementIsland); } _visual.Children.Clear(); _visual.Children.Add(uiElementIsland); } uiElementIsland.Offset = new PTS.FSVECTOR(_rect.u + mbp.BPLeft, _rect.v + mbp.BPTop).FromTextDpi(); } else { _visual.Children.Clear(); } // Draw background and borders. Brush backgroundBrush = (Brush)Paragraph.Element.GetValue(TextElement.BackgroundProperty); _visual.DrawBackgroundAndBorder(backgroundBrush, mbp.BorderBrush, mbp.Border, _rect.FromTextDpi(), IsFirstChunk, IsLastChunk); } ////// Returns tight bounding path geometry. /// internal Geometry GetTightBoundingGeometryFromTextPositions(ITextPointer startPosition, ITextPointer endPosition) { if (startPosition.CompareTo(((BlockUIContainer)Paragraph.Element).ContentEnd) < 0 && endPosition.CompareTo(((BlockUIContainer)Paragraph.Element).ContentStart) > 0) { return new RectangleGeometry(_rect.FromTextDpi()); } return null; } ////// Creates paragraph result representing this paragraph. /// ///internal override ParagraphResult CreateParagraphResult() { return new UIElementParagraphResult(this); } /// /// Hit tests to the correct IInputElement within the paragraph that /// the mouse is over. /// internal override IInputElement InputHitTest(PTS.FSPOINT pt) { if (_rect.Contains(pt)) { return Paragraph.Element as IInputElement; } return null; } ////// Returns TextContentRange for the content of the paragraph. /// internal override TextContentRange GetTextContentRange() { BlockUIContainer elementOwner = (BlockUIContainer)Paragraph.Element; return TextContainerHelper.GetTextContentRangeForTextElement(elementOwner); } #endregion Internal Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
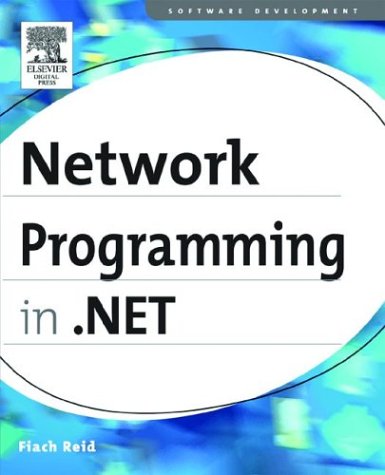
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TableItemPattern.cs
- ClusterRegistryConfigurationProvider.cs
- ISSmlParser.cs
- MsmqIntegrationProcessProtocolHandler.cs
- ExternalException.cs
- CallSite.cs
- XsdBuildProvider.cs
- BindStream.cs
- BaseValidator.cs
- GetWinFXPath.cs
- HatchBrush.cs
- Int32Storage.cs
- RIPEMD160Managed.cs
- CodeGotoStatement.cs
- DataGridViewRowPrePaintEventArgs.cs
- DataTableReader.cs
- SystemGatewayIPAddressInformation.cs
- HyperLinkField.cs
- AnnotationObservableCollection.cs
- ItemDragEvent.cs
- WebConfigManager.cs
- NullReferenceException.cs
- CellTreeNodeVisitors.cs
- DrawingContextDrawingContextWalker.cs
- TableLayoutPanelCodeDomSerializer.cs
- PointUtil.cs
- PickDesigner.xaml.cs
- DesignerTransaction.cs
- ActiveXContainer.cs
- LogStore.cs
- XhtmlTextWriter.cs
- DesignerTransaction.cs
- PcmConverter.cs
- TransformCryptoHandle.cs
- HorizontalAlignConverter.cs
- SequenceDesignerAccessibleObject.cs
- PageSettings.cs
- Query.cs
- FormatConvertedBitmap.cs
- Vector3DIndependentAnimationStorage.cs
- DurableEnlistmentState.cs
- StringReader.cs
- ExpressionBuilderContext.cs
- ControlCachePolicy.cs
- CommonXSendMessage.cs
- Fx.cs
- OracleRowUpdatingEventArgs.cs
- HScrollProperties.cs
- DataGridSortCommandEventArgs.cs
- XmlDigitalSignatureProcessor.cs
- SqlException.cs
- WindowsSidIdentity.cs
- CheckoutException.cs
- ConnectionManagementElementCollection.cs
- RepeatInfo.cs
- DBConcurrencyException.cs
- CustomAttribute.cs
- basecomparevalidator.cs
- VerificationException.cs
- TextElementCollectionHelper.cs
- XmlJsonReader.cs
- GroupBox.cs
- UriTemplateVariablePathSegment.cs
- CompilationUtil.cs
- UnsafeCollabNativeMethods.cs
- BindingSource.cs
- EventlogProvider.cs
- WindowsRichEdit.cs
- DriveNotFoundException.cs
- ScrollBar.cs
- TcpConnectionPool.cs
- SuppressMessageAttribute.cs
- Funcletizer.cs
- DataViewSettingCollection.cs
- EditorPartCollection.cs
- QuinticEase.cs
- ComponentRenameEvent.cs
- SecurityDocument.cs
- ByteFacetDescriptionElement.cs
- UserPersonalizationStateInfo.cs
- ClientTargetSection.cs
- CompiledQueryCacheKey.cs
- IndexedString.cs
- PointValueSerializer.cs
- MessageBox.cs
- FastEncoderWindow.cs
- EngineSiteSapi.cs
- ReadOnlyDictionary.cs
- XamlSerializerUtil.cs
- CodeArrayIndexerExpression.cs
- TimeoutValidationAttribute.cs
- ProxyDataContractResolver.cs
- Model3D.cs
- BinHexEncoding.cs
- BaseCAMarshaler.cs
- BitmapImage.cs
- Hashtable.cs
- SiteMap.cs
- CacheSection.cs
- EventLogRecord.cs