Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Automation / Peers / TextAutomationPeer.cs / 1 / TextAutomationPeer.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ContentTextElementAutomationPeer.cs // // Description: Base class for AutomationPeers associated with TextPattern. // //--------------------------------------------------------------------------- using System.Collections.Generic; // Listusing System.Windows.Automation.Provider; // IRawElementProviderSimple using System.Windows.Documents; // ITextPointer namespace System.Windows.Automation.Peers { /// /// Base class for AutomationPeers associated with TextPattern. /// public abstract class TextAutomationPeer : FrameworkElementAutomationPeer { ////// Constructor. /// protected TextAutomationPeer(FrameworkElement owner) : base(owner) {} ////// GetNameCore will return a value matching (in priority order) /// /// 1. Automation.Name /// 2. GetLabeledBy.Name /// 3. String.Empty /// /// This differs from the base implementation in that we must /// never return GetPlainText() . /// override protected string GetNameCore() { string result = AutomationProperties.GetName(this.Owner); if (string.IsNullOrEmpty(result)) { AutomationPeer labelAutomationPeer = GetLabeledByCore(); if (labelAutomationPeer != null) { result = labelAutomationPeer.GetName(); } } return result ?? string.Empty; } ////// Maps AutomationPeer to provider object. /// internal new IRawElementProviderSimple ProviderFromPeer(AutomationPeer peer) { return base.ProviderFromPeer(peer); } ////// Maps automation provider to DependencyObject. /// internal DependencyObject ElementFromProvider(IRawElementProviderSimple provider) { DependencyObject element = null; AutomationPeer peer = PeerFromProvider(provider); if (peer is UIElementAutomationPeer) { element = ((UIElementAutomationPeer)peer).Owner; } else if (peer is ContentElementAutomationPeer) { element = ((ContentElementAutomationPeer)peer).Owner; } return element; } ////// Gets collection of AutomationPeers for given text range. /// internal abstract ListGetAutomationPeersFromRange(ITextPointer start, ITextPointer end); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ContentTextElementAutomationPeer.cs // // Description: Base class for AutomationPeers associated with TextPattern. // //--------------------------------------------------------------------------- using System.Collections.Generic; // List using System.Windows.Automation.Provider; // IRawElementProviderSimple using System.Windows.Documents; // ITextPointer namespace System.Windows.Automation.Peers { /// /// Base class for AutomationPeers associated with TextPattern. /// public abstract class TextAutomationPeer : FrameworkElementAutomationPeer { ////// Constructor. /// protected TextAutomationPeer(FrameworkElement owner) : base(owner) {} ////// GetNameCore will return a value matching (in priority order) /// /// 1. Automation.Name /// 2. GetLabeledBy.Name /// 3. String.Empty /// /// This differs from the base implementation in that we must /// never return GetPlainText() . /// override protected string GetNameCore() { string result = AutomationProperties.GetName(this.Owner); if (string.IsNullOrEmpty(result)) { AutomationPeer labelAutomationPeer = GetLabeledByCore(); if (labelAutomationPeer != null) { result = labelAutomationPeer.GetName(); } } return result ?? string.Empty; } ////// Maps AutomationPeer to provider object. /// internal new IRawElementProviderSimple ProviderFromPeer(AutomationPeer peer) { return base.ProviderFromPeer(peer); } ////// Maps automation provider to DependencyObject. /// internal DependencyObject ElementFromProvider(IRawElementProviderSimple provider) { DependencyObject element = null; AutomationPeer peer = PeerFromProvider(provider); if (peer is UIElementAutomationPeer) { element = ((UIElementAutomationPeer)peer).Owner; } else if (peer is ContentElementAutomationPeer) { element = ((ContentElementAutomationPeer)peer).Owner; } return element; } ////// Gets collection of AutomationPeers for given text range. /// internal abstract ListGetAutomationPeersFromRange(ITextPointer start, ITextPointer end); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
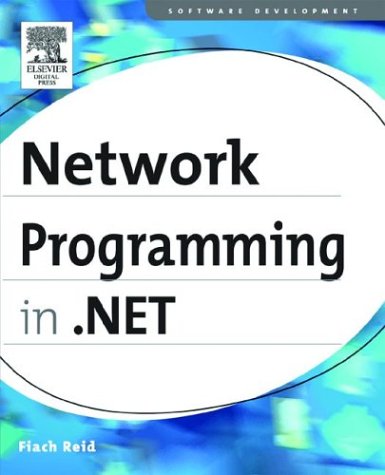
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlLanguage.cs
- OdbcHandle.cs
- SkewTransform.cs
- ListView.cs
- PerformanceCountersElement.cs
- DataGridViewCellCancelEventArgs.cs
- CodeTypeParameter.cs
- TextCompositionEventArgs.cs
- ProfileGroupSettingsCollection.cs
- FormsAuthenticationCredentials.cs
- MailMessageEventArgs.cs
- AdRotatorDesigner.cs
- DependencyPropertyConverter.cs
- MorphHelper.cs
- SchemaElementDecl.cs
- CodeBinaryOperatorExpression.cs
- DragEvent.cs
- BooleanKeyFrameCollection.cs
- DiscoveryCallbackBehavior.cs
- Interlocked.cs
- PenLineJoinValidation.cs
- RowToParametersTransformer.cs
- XmlSchemaInclude.cs
- ReadOnlyKeyedCollection.cs
- QueryCacheManager.cs
- DataBindingExpressionBuilder.cs
- CodeGeneratorOptions.cs
- BitmapData.cs
- ListViewPagedDataSource.cs
- ButtonField.cs
- HttpListenerPrefixCollection.cs
- TraceUtility.cs
- TableParagraph.cs
- AlternateView.cs
- XPathArrayIterator.cs
- AmbiguousMatchException.cs
- LogPolicy.cs
- ThreadStaticAttribute.cs
- WebPartMovingEventArgs.cs
- SQLInt64Storage.cs
- CurrencyManager.cs
- PathFigureCollection.cs
- XmlSchema.cs
- TypeSystem.cs
- LicenseException.cs
- ReturnEventArgs.cs
- CultureSpecificStringDictionary.cs
- SuppressIldasmAttribute.cs
- MatrixAnimationUsingPath.cs
- CoreChannel.cs
- SecurityRuntime.cs
- HighContrastHelper.cs
- TreeViewEvent.cs
- EntityContainerAssociationSet.cs
- ExecutedRoutedEventArgs.cs
- DocumentXPathNavigator.cs
- InheritanceService.cs
- SiteMapNodeItem.cs
- ModelItemImpl.cs
- NullEntityWrapper.cs
- DataGridView.cs
- KerberosReceiverSecurityToken.cs
- DoubleLink.cs
- CreateUserWizardStep.cs
- OleDbReferenceCollection.cs
- DispatcherOperation.cs
- XmlComment.cs
- XhtmlConformanceSection.cs
- EventLogger.cs
- NullReferenceException.cs
- GenericXmlSecurityTokenAuthenticator.cs
- SubstitutionList.cs
- CombinedGeometry.cs
- CodeMemberEvent.cs
- DeobfuscatingStream.cs
- WebBodyFormatMessageProperty.cs
- File.cs
- DefaultClaimSet.cs
- ZipIOFileItemStream.cs
- SQLDecimal.cs
- ValueTable.cs
- RightsManagementEncryptedStream.cs
- UserInitiatedRoutedEventPermissionAttribute.cs
- ConnectionStringSettingsCollection.cs
- RuleSetDialog.Designer.cs
- DropSource.cs
- Button.cs
- SafeNativeMemoryHandle.cs
- SqlWorkflowInstanceStore.cs
- ReachFixedDocumentSerializerAsync.cs
- QuestionEventArgs.cs
- EasingFunctionBase.cs
- SessionMode.cs
- InputMethodStateTypeInfo.cs
- ParagraphVisual.cs
- x509store.cs
- LocalizabilityAttribute.cs
- RSAPKCS1SignatureFormatter.cs
- XslCompiledTransform.cs
- TreeWalkHelper.cs