Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / Condition.cs / 1 / Condition.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 10/14/2003 : BrendanM - Created // //--------------------------------------------------------------------------- // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 using System; using MS.Internal.Automation; using System.Windows.Automation; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; namespace System.Windows.Automation { // Internal Class that wraps the IntPtr to the Node internal sealed class SafeConditionMemoryHandle : SafeHandle { // Called by P/Invoke when returning SafeHandles // (Also used by UiaCoreApi to create invalid handles.) internal SafeConditionMemoryHandle() : base(IntPtr.Zero, true) { } // No need to provide a finalizer - SafeHandle's critical finalizer will // call ReleaseHandle for you. public override bool IsInvalid { get { return handle == IntPtr.Zero; } } override protected bool ReleaseHandle() { Marshal.FreeCoTaskMem(handle); return true; } // uiaCondition is one of the Uia condition structs - eg UiaCoreApi.UiaAndOrCondition internal static SafeConditionMemoryHandle AllocateConditionHandle(object uiaCondition) { // Allocate SafeHandle first to avoid failure later. SafeConditionMemoryHandle sh = new SafeConditionMemoryHandle(); int size = Marshal.SizeOf(uiaCondition); RuntimeHelpers.PrepareConstrainedRegions(); // ensures that the following finally block is atomic try { } finally { IntPtr mem = Marshal.AllocCoTaskMem(size); sh.SetHandle(mem); } Marshal.StructureToPtr(uiaCondition, sh.handle, false); return sh; } // used by And/Or conditions to allocate an array of pointers to other conditions internal static SafeConditionMemoryHandle AllocateConditionArrayHandle(Condition [] conditions) { // Allocate SafeHandle first to avoid failure later. SafeConditionMemoryHandle sh = new SafeConditionMemoryHandle(); int intPtrSize = Marshal.SizeOf(typeof(IntPtr)); RuntimeHelpers.PrepareConstrainedRegions(); // ensures that the following finally block is atomic try { } finally { IntPtr mem = Marshal.AllocCoTaskMem(conditions.Length * intPtrSize); sh.SetHandle(mem); } unsafe // Suppress "Exposing unsafe code thru public interface" UiaCoreApi is trusted #pragma warning suppress 56505 { IntPtr* pdata = (IntPtr*)sh.handle; for (int i = 0; i < conditions.Length; i++) { *pdata++ = conditions[i]._safeHandle.handle; } } return sh; } // Can't pass null into an API that takes a SafeHandle - so using this instead... internal static SafeConditionMemoryHandle NullHandle = new SafeConditionMemoryHandle(); } ////// Base type for conditions used by LogicalElementSearcher. /// #if (INTERNAL_COMPILE) internal abstract class Condition #else public abstract class Condition #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Internal ctor to prevent others from deriving from this class internal Condition() { } #endregion Constructors //------------------------------------------------------ // // Public readonly fields & constants // //----------------------------------------------------- #region Public readonly fields & constants ///Condition object that always evaluates to true public static readonly Condition TrueCondition = new BoolCondition(true); ///Condition object that always evaluates to false public static readonly Condition FalseCondition = new BoolCondition(false); #endregion Public readonly fields & constants //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal void SetMarshalData(object uiaCondition) { // Takes one of the interop UiaCondition classes (from UiaCoreApi.cs), and allocs // a SafeHandle with associated unmanaged memory - can then pass that to the UIA APIs. _safeHandle = SafeConditionMemoryHandle.AllocateConditionHandle(uiaCondition); } #endregion Internal Methods //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal SafeConditionMemoryHandle _safeHandle; #endregion Internal Fields //----------------------------------------------------- // // Nested Classes // //----------------------------------------------------- private class BoolCondition: Condition { internal BoolCondition(bool b) { SetMarshalData(new UiaCoreApi.UiaCondition(b ? UiaCoreApi.ConditionType.True : UiaCoreApi.ConditionType.False)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 10/14/2003 : BrendanM - Created // //--------------------------------------------------------------------------- // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 using System; using MS.Internal.Automation; using System.Windows.Automation; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; namespace System.Windows.Automation { // Internal Class that wraps the IntPtr to the Node internal sealed class SafeConditionMemoryHandle : SafeHandle { // Called by P/Invoke when returning SafeHandles // (Also used by UiaCoreApi to create invalid handles.) internal SafeConditionMemoryHandle() : base(IntPtr.Zero, true) { } // No need to provide a finalizer - SafeHandle's critical finalizer will // call ReleaseHandle for you. public override bool IsInvalid { get { return handle == IntPtr.Zero; } } override protected bool ReleaseHandle() { Marshal.FreeCoTaskMem(handle); return true; } // uiaCondition is one of the Uia condition structs - eg UiaCoreApi.UiaAndOrCondition internal static SafeConditionMemoryHandle AllocateConditionHandle(object uiaCondition) { // Allocate SafeHandle first to avoid failure later. SafeConditionMemoryHandle sh = new SafeConditionMemoryHandle(); int size = Marshal.SizeOf(uiaCondition); RuntimeHelpers.PrepareConstrainedRegions(); // ensures that the following finally block is atomic try { } finally { IntPtr mem = Marshal.AllocCoTaskMem(size); sh.SetHandle(mem); } Marshal.StructureToPtr(uiaCondition, sh.handle, false); return sh; } // used by And/Or conditions to allocate an array of pointers to other conditions internal static SafeConditionMemoryHandle AllocateConditionArrayHandle(Condition [] conditions) { // Allocate SafeHandle first to avoid failure later. SafeConditionMemoryHandle sh = new SafeConditionMemoryHandle(); int intPtrSize = Marshal.SizeOf(typeof(IntPtr)); RuntimeHelpers.PrepareConstrainedRegions(); // ensures that the following finally block is atomic try { } finally { IntPtr mem = Marshal.AllocCoTaskMem(conditions.Length * intPtrSize); sh.SetHandle(mem); } unsafe // Suppress "Exposing unsafe code thru public interface" UiaCoreApi is trusted #pragma warning suppress 56505 { IntPtr* pdata = (IntPtr*)sh.handle; for (int i = 0; i < conditions.Length; i++) { *pdata++ = conditions[i]._safeHandle.handle; } } return sh; } // Can't pass null into an API that takes a SafeHandle - so using this instead... internal static SafeConditionMemoryHandle NullHandle = new SafeConditionMemoryHandle(); } ////// Base type for conditions used by LogicalElementSearcher. /// #if (INTERNAL_COMPILE) internal abstract class Condition #else public abstract class Condition #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Internal ctor to prevent others from deriving from this class internal Condition() { } #endregion Constructors //------------------------------------------------------ // // Public readonly fields & constants // //----------------------------------------------------- #region Public readonly fields & constants ///Condition object that always evaluates to true public static readonly Condition TrueCondition = new BoolCondition(true); ///Condition object that always evaluates to false public static readonly Condition FalseCondition = new BoolCondition(false); #endregion Public readonly fields & constants //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal void SetMarshalData(object uiaCondition) { // Takes one of the interop UiaCondition classes (from UiaCoreApi.cs), and allocs // a SafeHandle with associated unmanaged memory - can then pass that to the UIA APIs. _safeHandle = SafeConditionMemoryHandle.AllocateConditionHandle(uiaCondition); } #endregion Internal Methods //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal SafeConditionMemoryHandle _safeHandle; #endregion Internal Fields //----------------------------------------------------- // // Nested Classes // //----------------------------------------------------- private class BoolCondition: Condition { internal BoolCondition(bool b) { SetMarshalData(new UiaCoreApi.UiaCondition(b ? UiaCoreApi.ConditionType.True : UiaCoreApi.ConditionType.False)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
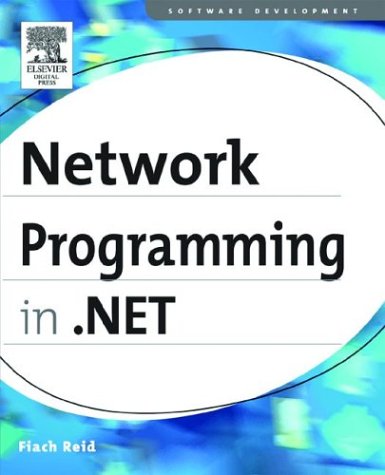
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlCDATASection.cs
- TextDecorationCollection.cs
- XmlSchemaComplexContentRestriction.cs
- ResourceWriter.cs
- LabelDesigner.cs
- SystemIcmpV6Statistics.cs
- ActivityWithResult.cs
- HtmlInputControl.cs
- OleServicesContext.cs
- BitmapEditor.cs
- CellParagraph.cs
- RepeatButton.cs
- ParameterInfo.cs
- ServicePoint.cs
- TextServicesContext.cs
- TextRunTypographyProperties.cs
- DocumentSchemaValidator.cs
- EntityContainerRelationshipSetEnd.cs
- ItemTypeToolStripMenuItem.cs
- ProcessStartInfo.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- PersonalizationStateQuery.cs
- UrlRoutingModule.cs
- DataGridViewAutoSizeModeEventArgs.cs
- TypeUsageBuilder.cs
- ReadOnlyDataSource.cs
- MdiWindowListStrip.cs
- WpfGeneratedKnownProperties.cs
- OutputScopeManager.cs
- NavigateEvent.cs
- HtmlInputSubmit.cs
- SequentialUshortCollection.cs
- CapabilitiesPattern.cs
- HttpContext.cs
- WsdlBuildProvider.cs
- DataTableReader.cs
- TagPrefixAttribute.cs
- EntityClientCacheEntry.cs
- SafeNativeMethods.cs
- XmlAttributeCollection.cs
- EmbeddedObject.cs
- XmlSecureResolver.cs
- SafeNativeMethods.cs
- XmlWriterSettings.cs
- RealizationDrawingContextWalker.cs
- StringOutput.cs
- MessageSecurityOverTcpElement.cs
- EntityTypeEmitter.cs
- ScriptMethodAttribute.cs
- Point3D.cs
- ChainedAsyncResult.cs
- UpdateManifestForBrowserApplication.cs
- returneventsaver.cs
- UnionCodeGroup.cs
- PolyLineSegment.cs
- TypeUtils.cs
- RIPEMD160Managed.cs
- DataContractSerializerSection.cs
- ParseHttpDate.cs
- CngAlgorithmGroup.cs
- EntityContainerEmitter.cs
- DiagnosticSection.cs
- Switch.cs
- CryptoApi.cs
- ToolbarAUtomationPeer.cs
- TranslateTransform.cs
- AttributeCollection.cs
- UnaryQueryOperator.cs
- XmlSchemaSubstitutionGroup.cs
- UserPreferenceChangingEventArgs.cs
- httpserverutility.cs
- WinEventQueueItem.cs
- ColorIndependentAnimationStorage.cs
- MSHTMLHostUtil.cs
- CaseInsensitiveComparer.cs
- SmtpFailedRecipientException.cs
- PartialCachingControl.cs
- NamedServiceModelExtensionCollectionElement.cs
- DefaultValueMapping.cs
- UxThemeWrapper.cs
- CopyNodeSetAction.cs
- DeleteBookmarkScope.cs
- DataGridViewHeaderCell.cs
- FlowNode.cs
- OracleFactory.cs
- ModelItem.cs
- TimeSpanConverter.cs
- MouseGesture.cs
- DropTarget.cs
- ProtectedConfigurationSection.cs
- HtmlLinkAdapter.cs
- newinstructionaction.cs
- Constants.cs
- DataPagerFieldItem.cs
- SByte.cs
- BitmapEffectDrawingContextWalker.cs
- TreeView.cs
- ContourSegment.cs
- PropertyItem.cs
- TraceUtility.cs