Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Data / System / Data / ConstraintEnumerator.cs / 1 / ConstraintEnumerator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Diagnostics; using System.Collections; using System.ComponentModel; ////// ConstraintEnumerator is an object for enumerating all constraints in a DataSet /// internal class ConstraintEnumerator { System.Collections.IEnumerator tables; System.Collections.IEnumerator constraints; Constraint currentObject; public ConstraintEnumerator(DataSet dataSet) { tables = (dataSet != null) ? dataSet.Tables.GetEnumerator() : null; currentObject = null; } public bool GetNext() { Constraint candidate; currentObject = null; while (tables != null) { if (constraints == null) { if (!tables.MoveNext()) { tables = null; return false; } constraints = ((DataTable)tables.Current).Constraints.GetEnumerator(); } if (!constraints.MoveNext()) { constraints = null; continue; } Debug.Assert(constraints.Current is Constraint, "ConstraintEnumerator, contains object which is not constraint"); candidate = (Constraint)constraints.Current; if (IsValidCandidate(candidate)) { currentObject = candidate; return true; } } return false; } public Constraint GetConstraint() { // If currentObject is null we are before first GetNext or after last GetNext--consumer is bad Debug.Assert (currentObject != null, "GetObject should never be called w/ null currentObject."); return currentObject; } protected virtual bool IsValidCandidate(Constraint constraint) { return true; } protected Constraint CurrentObject { get { return currentObject; } } } internal class ForeignKeyConstraintEnumerator : ConstraintEnumerator { public ForeignKeyConstraintEnumerator(DataSet dataSet) : base(dataSet) { } protected override bool IsValidCandidate(Constraint constraint) { return(constraint is ForeignKeyConstraint); } public ForeignKeyConstraint GetForeignKeyConstraint() { // If CurrentObject is null we are before first GetNext or after last GetNext--consumer is bad Debug.Assert (CurrentObject != null, "GetObject should never be called w/ null currentObject."); return(ForeignKeyConstraint)CurrentObject; } } internal sealed class ChildForeignKeyConstraintEnumerator : ForeignKeyConstraintEnumerator { // this is the table to do comparisons against DataTable table; public ChildForeignKeyConstraintEnumerator(DataSet dataSet, DataTable inTable) : base(dataSet) { this.table = inTable; } protected override bool IsValidCandidate(Constraint constraint) { return((constraint is ForeignKeyConstraint) && (((ForeignKeyConstraint)constraint).Table == table)); } } internal sealed class ParentForeignKeyConstraintEnumerator : ForeignKeyConstraintEnumerator { // this is the table to do comparisons against DataTable table; public ParentForeignKeyConstraintEnumerator(DataSet dataSet, DataTable inTable) : base(dataSet) { this.table = inTable; } protected override bool IsValidCandidate(Constraint constraint) { return((constraint is ForeignKeyConstraint) && (((ForeignKeyConstraint)constraint).RelatedTable == table)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Diagnostics; using System.Collections; using System.ComponentModel; ////// ConstraintEnumerator is an object for enumerating all constraints in a DataSet /// internal class ConstraintEnumerator { System.Collections.IEnumerator tables; System.Collections.IEnumerator constraints; Constraint currentObject; public ConstraintEnumerator(DataSet dataSet) { tables = (dataSet != null) ? dataSet.Tables.GetEnumerator() : null; currentObject = null; } public bool GetNext() { Constraint candidate; currentObject = null; while (tables != null) { if (constraints == null) { if (!tables.MoveNext()) { tables = null; return false; } constraints = ((DataTable)tables.Current).Constraints.GetEnumerator(); } if (!constraints.MoveNext()) { constraints = null; continue; } Debug.Assert(constraints.Current is Constraint, "ConstraintEnumerator, contains object which is not constraint"); candidate = (Constraint)constraints.Current; if (IsValidCandidate(candidate)) { currentObject = candidate; return true; } } return false; } public Constraint GetConstraint() { // If currentObject is null we are before first GetNext or after last GetNext--consumer is bad Debug.Assert (currentObject != null, "GetObject should never be called w/ null currentObject."); return currentObject; } protected virtual bool IsValidCandidate(Constraint constraint) { return true; } protected Constraint CurrentObject { get { return currentObject; } } } internal class ForeignKeyConstraintEnumerator : ConstraintEnumerator { public ForeignKeyConstraintEnumerator(DataSet dataSet) : base(dataSet) { } protected override bool IsValidCandidate(Constraint constraint) { return(constraint is ForeignKeyConstraint); } public ForeignKeyConstraint GetForeignKeyConstraint() { // If CurrentObject is null we are before first GetNext or after last GetNext--consumer is bad Debug.Assert (CurrentObject != null, "GetObject should never be called w/ null currentObject."); return(ForeignKeyConstraint)CurrentObject; } } internal sealed class ChildForeignKeyConstraintEnumerator : ForeignKeyConstraintEnumerator { // this is the table to do comparisons against DataTable table; public ChildForeignKeyConstraintEnumerator(DataSet dataSet, DataTable inTable) : base(dataSet) { this.table = inTable; } protected override bool IsValidCandidate(Constraint constraint) { return((constraint is ForeignKeyConstraint) && (((ForeignKeyConstraint)constraint).Table == table)); } } internal sealed class ParentForeignKeyConstraintEnumerator : ForeignKeyConstraintEnumerator { // this is the table to do comparisons against DataTable table; public ParentForeignKeyConstraintEnumerator(DataSet dataSet, DataTable inTable) : base(dataSet) { this.table = inTable; } protected override bool IsValidCandidate(Constraint constraint) { return((constraint is ForeignKeyConstraint) && (((ForeignKeyConstraint)constraint).RelatedTable == table)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
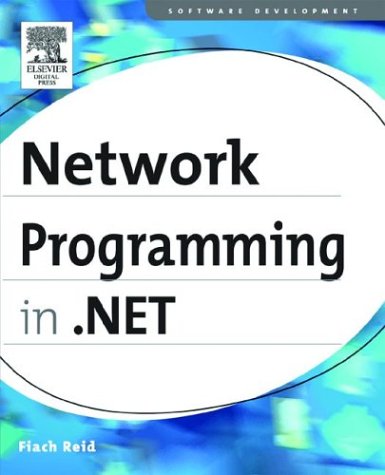
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormsAuthenticationTicket.cs
- OperationDescriptionCollection.cs
- dataSvcMapFileLoader.cs
- XXXOnTypeBuilderInstantiation.cs
- PropertySegmentSerializer.cs
- WebControl.cs
- FormViewDeletedEventArgs.cs
- TextAdaptor.cs
- JsonStringDataContract.cs
- UntrustedRecipientException.cs
- ToggleButtonAutomationPeer.cs
- StatusBarItem.cs
- CardSpacePolicyElement.cs
- Span.cs
- ToolStripContextMenu.cs
- XmlComplianceUtil.cs
- CorrelationKey.cs
- DependencyPropertyChangedEventArgs.cs
- HtmlInputFile.cs
- ImageConverter.cs
- BackgroundFormatInfo.cs
- ViewStateModeByIdAttribute.cs
- safesecurityhelperavalon.cs
- AttributeProviderAttribute.cs
- DeviceSpecific.cs
- InvokeHandlers.cs
- PerformanceCounterLib.cs
- SafePEFileHandle.cs
- EpmSyndicationContentDeSerializer.cs
- FileEnumerator.cs
- basecomparevalidator.cs
- ResolveCriteria.cs
- InvariantComparer.cs
- ControlHelper.cs
- PerformanceCounter.cs
- DependencyObject.cs
- FilteredDataSetHelper.cs
- RenderOptions.cs
- KerberosRequestorSecurityToken.cs
- Win32.cs
- DynamicMethod.cs
- ExtenderProviderService.cs
- EntityCommandExecutionException.cs
- NonDualMessageSecurityOverHttpElement.cs
- RSAOAEPKeyExchangeFormatter.cs
- DtrList.cs
- HttpApplicationFactory.cs
- XmlWrappingReader.cs
- XmlText.cs
- TransportReplyChannelAcceptor.cs
- TextEditorThreadLocalStore.cs
- WebControlParameterProxy.cs
- ColumnResult.cs
- MulticastDelegate.cs
- DataServiceQueryOfT.cs
- UnsafeNativeMethodsCLR.cs
- ToolZone.cs
- PermissionSet.cs
- FontCacheLogic.cs
- ArrangedElement.cs
- PriorityBinding.cs
- BaseTreeIterator.cs
- TreeIterator.cs
- XmlUrlEditor.cs
- MulticastOption.cs
- ScrollableControl.cs
- HashHelpers.cs
- SSmlParser.cs
- ProfileManager.cs
- RelationshipConverter.cs
- HttpResponseBase.cs
- CacheChildrenQuery.cs
- HWStack.cs
- OrCondition.cs
- NumericExpr.cs
- EntityUtil.cs
- PrinterSettings.cs
- StreamGeometry.cs
- LicenseProviderAttribute.cs
- TextTreeNode.cs
- ReflectionPermission.cs
- DataBinder.cs
- EdgeProfileValidation.cs
- OdbcPermission.cs
- AsyncOperationManager.cs
- BuildManagerHost.cs
- base64Transforms.cs
- HtmlWindow.cs
- SchemaAttDef.cs
- StyleCollection.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- UpdatePanel.cs
- WebReferencesBuildProvider.cs
- AVElementHelper.cs
- MenuItemCollection.cs
- XmlDomTextWriter.cs
- ScriptResourceMapping.cs
- RelationshipConverter.cs
- ResXDataNode.cs
- SettingsPropertyCollection.cs