Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / Configuration / StrongNameUtility.cs / 1 / StrongNameUtility.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Diagnostics; using System.IO; using System.Runtime.InteropServices; using System.Security.Permissions; internal class StrongNameUtility { // Help class shouldn't be instantiated. private StrongNameUtility() { } /// Free the buffer allocated by strong name functions /// /// address of memory to free [DllImport("mscoree.dll")] internal extern static void StrongNameFreeBuffer(IntPtr pbMemory); ////// Return the last error /// ///error information for the last strong name call [DllImport("mscoree.dll")] internal extern static int StrongNameErrorInfo(); ////// Generate a new key pair for strong name use /// /// desired key container name /// flags /// [out] generated public / private key blob /// [out] size of the generated blob ///true if the key was generated, false if there was an error [DllImport("mscoree.dll")] internal extern static bool StrongNameKeyGen([MarshalAs(UnmanagedType.LPWStr)]string wszKeyContainer, uint dwFlags, [Out]out IntPtr ppbKeyBlob, [Out]out long pcbKeyBlob); internal static bool GenerateStrongNameFile(string filename) { // variables that hold the unmanaged key IntPtr keyBlob = IntPtr.Zero; long generatedSize = 0; // create the key bool createdKey = StrongNameKeyGen(null, 0 /*No flags. 1 is to save the key in the key container */, out keyBlob, out generatedSize); // if there was a problem, translate it and report it if (!createdKey || keyBlob == IntPtr.Zero) { throw Marshal.GetExceptionForHR(StrongNameErrorInfo()); } try { Debug.Assert(keyBlob != IntPtr.Zero); // make sure the key size makes sense Debug.Assert(generatedSize > 0 && generatedSize <= Int32.MaxValue); if (generatedSize <= 0 || generatedSize > Int32.MaxValue) { throw new InvalidOperationException(SR.GetString(SR.Browser_InvalidStrongNameKey)); } // get the key into managed memory byte[] key = new byte[generatedSize]; Marshal.Copy(keyBlob, key, 0, (int)generatedSize); // write the key to the specified file using (FileStream snkStream = new FileStream(filename, FileMode.Create, FileAccess.Write)) { using (BinaryWriter snkWriter = new BinaryWriter(snkStream)) { snkWriter.Write(key); } } } finally { // release the unmanaged memory the key resides in if (keyBlob != IntPtr.Zero) { StrongNameFreeBuffer(keyBlob); } } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Diagnostics; using System.IO; using System.Runtime.InteropServices; using System.Security.Permissions; internal class StrongNameUtility { // Help class shouldn't be instantiated. private StrongNameUtility() { } /// Free the buffer allocated by strong name functions /// /// address of memory to free [DllImport("mscoree.dll")] internal extern static void StrongNameFreeBuffer(IntPtr pbMemory); ////// Return the last error /// ///error information for the last strong name call [DllImport("mscoree.dll")] internal extern static int StrongNameErrorInfo(); ////// Generate a new key pair for strong name use /// /// desired key container name /// flags /// [out] generated public / private key blob /// [out] size of the generated blob ///true if the key was generated, false if there was an error [DllImport("mscoree.dll")] internal extern static bool StrongNameKeyGen([MarshalAs(UnmanagedType.LPWStr)]string wszKeyContainer, uint dwFlags, [Out]out IntPtr ppbKeyBlob, [Out]out long pcbKeyBlob); internal static bool GenerateStrongNameFile(string filename) { // variables that hold the unmanaged key IntPtr keyBlob = IntPtr.Zero; long generatedSize = 0; // create the key bool createdKey = StrongNameKeyGen(null, 0 /*No flags. 1 is to save the key in the key container */, out keyBlob, out generatedSize); // if there was a problem, translate it and report it if (!createdKey || keyBlob == IntPtr.Zero) { throw Marshal.GetExceptionForHR(StrongNameErrorInfo()); } try { Debug.Assert(keyBlob != IntPtr.Zero); // make sure the key size makes sense Debug.Assert(generatedSize > 0 && generatedSize <= Int32.MaxValue); if (generatedSize <= 0 || generatedSize > Int32.MaxValue) { throw new InvalidOperationException(SR.GetString(SR.Browser_InvalidStrongNameKey)); } // get the key into managed memory byte[] key = new byte[generatedSize]; Marshal.Copy(keyBlob, key, 0, (int)generatedSize); // write the key to the specified file using (FileStream snkStream = new FileStream(filename, FileMode.Create, FileAccess.Write)) { using (BinaryWriter snkWriter = new BinaryWriter(snkStream)) { snkWriter.Write(key); } } } finally { // release the unmanaged memory the key resides in if (keyBlob != IntPtr.Zero) { StrongNameFreeBuffer(keyBlob); } } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
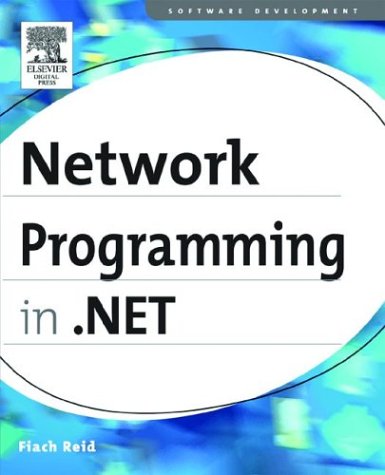
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PermissionListSet.cs
- StatusBarPanelClickEvent.cs
- JpegBitmapEncoder.cs
- IIS7UserPrincipal.cs
- SelectionRangeConverter.cs
- RelationshipManager.cs
- MobileCapabilities.cs
- FrameworkContentElement.cs
- ADMembershipProvider.cs
- EventLogInformation.cs
- ApplicationId.cs
- Crypto.cs
- XmlSchemaAppInfo.cs
- SponsorHelper.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- CommandBinding.cs
- ToolboxItemCollection.cs
- EqualityArray.cs
- adornercollection.cs
- SessionViewState.cs
- SqlGatherProducedAliases.cs
- ButtonFieldBase.cs
- IteratorFilter.cs
- JsonReader.cs
- OleDbCommandBuilder.cs
- MessageTraceRecord.cs
- DbModificationCommandTree.cs
- PermissionSetTriple.cs
- PageSetupDialog.cs
- ProcessStartInfo.cs
- ViewPort3D.cs
- FreeFormDesigner.cs
- SingleAnimationUsingKeyFrames.cs
- XmlSchemaAnyAttribute.cs
- ElementHostPropertyMap.cs
- TextParaLineResult.cs
- SafeRegistryHandle.cs
- GridViewActionList.cs
- CodeSnippetTypeMember.cs
- SaveFileDialog.cs
- SrgsDocumentParser.cs
- AdjustableArrowCap.cs
- XmlChoiceIdentifierAttribute.cs
- DateTimeConverter2.cs
- SelectedDatesCollection.cs
- HostSecurityManager.cs
- BookmarkEventArgs.cs
- CodeDelegateCreateExpression.cs
- MessageSmuggler.cs
- MetadataSerializer.cs
- IsolatedStoragePermission.cs
- HtmlEmptyTagControlBuilder.cs
- ClientSettingsProvider.cs
- XXXInfos.cs
- TableChangeProcessor.cs
- ImageAnimator.cs
- DataKeyArray.cs
- SByteConverter.cs
- DecimalConstantAttribute.cs
- SourceSwitch.cs
- XmlWrappingReader.cs
- TextModifier.cs
- ContextInformation.cs
- RoleService.cs
- SafeThreadHandle.cs
- Pts.cs
- CodeAttributeDeclaration.cs
- DetailsViewCommandEventArgs.cs
- PageCodeDomTreeGenerator.cs
- ScriptServiceAttribute.cs
- StorageSetMapping.cs
- Focus.cs
- _AuthenticationState.cs
- ListBoxItemWrapperAutomationPeer.cs
- CacheModeConverter.cs
- RefExpr.cs
- Debugger.cs
- ChangePassword.cs
- DecoderFallback.cs
- GridSplitter.cs
- HMACRIPEMD160.cs
- SynchronizingStream.cs
- shaperfactoryquerycacheentry.cs
- ConnectionPoint.cs
- ConcurrentDictionary.cs
- HitTestWithGeometryDrawingContextWalker.cs
- SolidColorBrush.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- DataServiceRequestOfT.cs
- ScrollEvent.cs
- ApplicationFileParser.cs
- DesignerProperties.cs
- InstanceOwnerQueryResult.cs
- BindingExpressionBase.cs
- RunWorkerCompletedEventArgs.cs
- FlagPanel.cs
- TagMapCollection.cs
- SqlClientFactory.cs
- HotSpotCollection.cs
- TemplateControlParser.cs