Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / DataSourceControl.cs / 1 / DataSourceControl.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Collections; using System.ComponentModel; using System.Security.Permissions; ////// A DataSourceControl represents a data source that can be used to /// data-bind a DataBoundControl. /// DataSourceControl is an abstract base class that defines the /// interface between a DataBoundControl and its data source. /// The design of DataSourceControl enables creation of a variety of /// data controls with different underlying data sources such /// as SqlDataControl, WebServiceDataControl, XmlDataControl etc. /// The data source is implemented as a control even though it /// has no visual rendering, to allow it to be persisted /// declaratively, and to allow it to participate in state /// management should it choose to. /// In abstract terms a DataSourceControl has an underlying data source. /// This data source may contain one or more lists of data within it. /// Each list is associated with a name and at the bare minimum /// supports enumeration via the IEnumerable interface. A DataBoundControl /// is typically bound to a single list within the DataControl. /// [ Bindable(false), ControlBuilder(typeof(DataSourceControlBuilder)), Designer("System.Web.UI.Design.DataSourceDesigner, " + AssemblyRef.SystemDesign), NonVisualControl() ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class DataSourceControl : Control, IDataSource, IListSource { private static readonly object EventDataSourceChanged = new object(); private static readonly object EventDataSourceChangedInternal = new object(); [ EditorBrowsable(EditorBrowsableState.Never), ] public override string ClientID { get { return base.ClientID; } } [ EditorBrowsable(EditorBrowsableState.Never), ] public override ControlCollection Controls { get { return base.Controls; } } [ Browsable(false), DefaultValue(false), EditorBrowsable(EditorBrowsableState.Never), ] public override bool EnableTheming { get { return false; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } [ Browsable(false), DefaultValue(""), EditorBrowsable(EditorBrowsableState.Never), ] public override string SkinID { get { return String.Empty; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } ////// Gets or sets a value that indicates whether a control should be rendered on /// the page. /// [ Browsable(false), DefaultValue(false), EditorBrowsable(EditorBrowsableState.Never), ] public override bool Visible { get { return false; } set { throw new NotSupportedException(SR.GetString(SR.ControlNonVisual, this.GetType().Name)); } } ////// Raised internally by a DataSource when data-related state is /// changed. DataSourceViews attach to this event so that they can /// be notified when the parent data source changes. /// This event is separate from DataSourceChanged because the DataSourceView /// attaches to it and fires its DataSourceViewChanged event. We want that /// to fire before the DataSourceChanged event. /// internal event EventHandler DataSourceChangedInternal { add { Events.AddHandler(EventDataSourceChangedInternal, value); } remove { Events.RemoveHandler(EventDataSourceChangedInternal, value); } } [ EditorBrowsable(EditorBrowsableState.Never), ] public override void ApplyStyleSheetSkin(Page page) { base.ApplyStyleSheetSkin(page); } ////// Overidden to prevent child controls from being added to this control. /// protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } [ EditorBrowsable(EditorBrowsableState.Never), ] public override Control FindControl(string id) { return base.FindControl(id); } ////// [ EditorBrowsable(EditorBrowsableState.Never), ] public override void Focus() { throw new NotSupportedException(SR.GetString(SR.NoFocusSupport, this.GetType().Name)); } protected abstract DataSourceView GetView(string viewName); protected virtual ICollection GetViewNames() { return null; } [ EditorBrowsable(EditorBrowsableState.Never), ] public override bool HasControls() { return base.HasControls(); } private void OnDataSourceChanged(EventArgs e) { EventHandler handler = (EventHandler)Events[EventDataSourceChanged]; if (handler != null) { handler(this, e); } } private void OnDataSourceChangedInternal(EventArgs e) { EventHandler handler = (EventHandler)Events[EventDataSourceChangedInternal]; if (handler != null) { handler(this, e); } } protected virtual void RaiseDataSourceChangedEvent(EventArgs e) { OnDataSourceChangedInternal(e); OnDataSourceChanged(e); } [ EditorBrowsable(EditorBrowsableState.Never), ] public override void RenderControl(HtmlTextWriter writer) { base.RenderControl(writer); } #region Implementation of IDataSource ////// Raised when the underlying data source has changed. The /// change may be due to a change in the control's properties, /// or a change in the data due to an edit action performed by /// the DataSourceControl. /// event EventHandler IDataSource.DataSourceChanged { add { Events.AddHandler(EventDataSourceChanged, value); } remove { Events.RemoveHandler(EventDataSourceChanged, value); } } ///DataSourceView IDataSource.GetView(string viewName) { return GetView(viewName); } /// ICollection IDataSource.GetViewNames() { return GetViewNames(); } #endregion #region Implementation of IListSource /// bool IListSource.ContainsListCollection { get { if (DesignMode) { return false; } return ListSourceHelper.ContainsListCollection(this); } } /// IList IListSource.GetList() { if (DesignMode) { return null; } return ListSourceHelper.GetList(this); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Collections; using System.ComponentModel; using System.Security.Permissions; ////// A DataSourceControl represents a data source that can be used to /// data-bind a DataBoundControl. /// DataSourceControl is an abstract base class that defines the /// interface between a DataBoundControl and its data source. /// The design of DataSourceControl enables creation of a variety of /// data controls with different underlying data sources such /// as SqlDataControl, WebServiceDataControl, XmlDataControl etc. /// The data source is implemented as a control even though it /// has no visual rendering, to allow it to be persisted /// declaratively, and to allow it to participate in state /// management should it choose to. /// In abstract terms a DataSourceControl has an underlying data source. /// This data source may contain one or more lists of data within it. /// Each list is associated with a name and at the bare minimum /// supports enumeration via the IEnumerable interface. A DataBoundControl /// is typically bound to a single list within the DataControl. /// [ Bindable(false), ControlBuilder(typeof(DataSourceControlBuilder)), Designer("System.Web.UI.Design.DataSourceDesigner, " + AssemblyRef.SystemDesign), NonVisualControl() ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class DataSourceControl : Control, IDataSource, IListSource { private static readonly object EventDataSourceChanged = new object(); private static readonly object EventDataSourceChangedInternal = new object(); [ EditorBrowsable(EditorBrowsableState.Never), ] public override string ClientID { get { return base.ClientID; } } [ EditorBrowsable(EditorBrowsableState.Never), ] public override ControlCollection Controls { get { return base.Controls; } } [ Browsable(false), DefaultValue(false), EditorBrowsable(EditorBrowsableState.Never), ] public override bool EnableTheming { get { return false; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } [ Browsable(false), DefaultValue(""), EditorBrowsable(EditorBrowsableState.Never), ] public override string SkinID { get { return String.Empty; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } ////// Gets or sets a value that indicates whether a control should be rendered on /// the page. /// [ Browsable(false), DefaultValue(false), EditorBrowsable(EditorBrowsableState.Never), ] public override bool Visible { get { return false; } set { throw new NotSupportedException(SR.GetString(SR.ControlNonVisual, this.GetType().Name)); } } ////// Raised internally by a DataSource when data-related state is /// changed. DataSourceViews attach to this event so that they can /// be notified when the parent data source changes. /// This event is separate from DataSourceChanged because the DataSourceView /// attaches to it and fires its DataSourceViewChanged event. We want that /// to fire before the DataSourceChanged event. /// internal event EventHandler DataSourceChangedInternal { add { Events.AddHandler(EventDataSourceChangedInternal, value); } remove { Events.RemoveHandler(EventDataSourceChangedInternal, value); } } [ EditorBrowsable(EditorBrowsableState.Never), ] public override void ApplyStyleSheetSkin(Page page) { base.ApplyStyleSheetSkin(page); } ////// Overidden to prevent child controls from being added to this control. /// protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } [ EditorBrowsable(EditorBrowsableState.Never), ] public override Control FindControl(string id) { return base.FindControl(id); } ////// [ EditorBrowsable(EditorBrowsableState.Never), ] public override void Focus() { throw new NotSupportedException(SR.GetString(SR.NoFocusSupport, this.GetType().Name)); } protected abstract DataSourceView GetView(string viewName); protected virtual ICollection GetViewNames() { return null; } [ EditorBrowsable(EditorBrowsableState.Never), ] public override bool HasControls() { return base.HasControls(); } private void OnDataSourceChanged(EventArgs e) { EventHandler handler = (EventHandler)Events[EventDataSourceChanged]; if (handler != null) { handler(this, e); } } private void OnDataSourceChangedInternal(EventArgs e) { EventHandler handler = (EventHandler)Events[EventDataSourceChangedInternal]; if (handler != null) { handler(this, e); } } protected virtual void RaiseDataSourceChangedEvent(EventArgs e) { OnDataSourceChangedInternal(e); OnDataSourceChanged(e); } [ EditorBrowsable(EditorBrowsableState.Never), ] public override void RenderControl(HtmlTextWriter writer) { base.RenderControl(writer); } #region Implementation of IDataSource ////// Raised when the underlying data source has changed. The /// change may be due to a change in the control's properties, /// or a change in the data due to an edit action performed by /// the DataSourceControl. /// event EventHandler IDataSource.DataSourceChanged { add { Events.AddHandler(EventDataSourceChanged, value); } remove { Events.RemoveHandler(EventDataSourceChanged, value); } } ///DataSourceView IDataSource.GetView(string viewName) { return GetView(viewName); } /// ICollection IDataSource.GetViewNames() { return GetViewNames(); } #endregion #region Implementation of IListSource /// bool IListSource.ContainsListCollection { get { if (DesignMode) { return false; } return ListSourceHelper.ContainsListCollection(this); } } /// IList IListSource.GetList() { if (DesignMode) { return null; } return ListSourceHelper.GetList(this); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
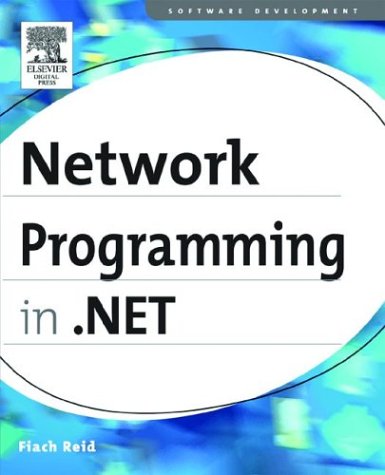
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MiniLockedBorderGlyph.cs
- PathParser.cs
- DataColumnMappingCollection.cs
- ValidationError.cs
- panel.cs
- RelationshipDetailsCollection.cs
- SecureEnvironment.cs
- ResourcePool.cs
- AspCompat.cs
- JsonWriter.cs
- File.cs
- SerializationSectionGroup.cs
- HyperLinkStyle.cs
- QuotedStringFormatReader.cs
- DataColumnPropertyDescriptor.cs
- Attribute.cs
- PriorityRange.cs
- GridViewAutoFormat.cs
- EventHandlers.cs
- EnterpriseServicesHelper.cs
- DesignerCategoryAttribute.cs
- RSAPKCS1SignatureFormatter.cs
- DocumentViewer.cs
- MessageAction.cs
- odbcmetadatacolumnnames.cs
- MarginsConverter.cs
- EventManager.cs
- DataTablePropertyDescriptor.cs
- UIHelper.cs
- StateWorkerRequest.cs
- IntSecurity.cs
- DefaultAssemblyResolver.cs
- ProfileManager.cs
- BrushValueSerializer.cs
- DrawListViewSubItemEventArgs.cs
- _SslStream.cs
- HtmlTextArea.cs
- WsdlParser.cs
- AmbientValueAttribute.cs
- CodeSnippetStatement.cs
- DbParameterHelper.cs
- SqlError.cs
- InvokeWebServiceDesigner.cs
- DbConnectionPool.cs
- QilFactory.cs
- AdPostCacheSubstitution.cs
- SettingsAttributes.cs
- Int32RectConverter.cs
- EventLogPermissionEntryCollection.cs
- MenuItemCollectionEditor.cs
- WebPartAuthorizationEventArgs.cs
- ImageMetadata.cs
- ColumnMapProcessor.cs
- TypeDescriptionProvider.cs
- _OSSOCK.cs
- DrawingImage.cs
- SystemTcpStatistics.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- _KerberosClient.cs
- SoundPlayer.cs
- CapabilitiesState.cs
- Debug.cs
- DriveNotFoundException.cs
- CollectionDataContractAttribute.cs
- BuildResultCache.cs
- LinqMaximalSubtreeNominator.cs
- InternalTransaction.cs
- SubqueryTrackingVisitor.cs
- Calendar.cs
- WebPartsSection.cs
- LeaseManager.cs
- Visitors.cs
- StorageRoot.cs
- WebBrowser.cs
- ButtonAutomationPeer.cs
- HtmlEmptyTagControlBuilder.cs
- TracingConnectionListener.cs
- BezierSegment.cs
- RepeatInfo.cs
- ActivationArguments.cs
- CodeEventReferenceExpression.cs
- DataGridViewCheckBoxCell.cs
- ResizeGrip.cs
- CompareInfo.cs
- SmtpFailedRecipientException.cs
- NoneExcludedImageIndexConverter.cs
- Permission.cs
- ComponentCollection.cs
- GradientSpreadMethodValidation.cs
- Annotation.cs
- Polyline.cs
- Recipient.cs
- DataServicePagingProviderWrapper.cs
- DesignerOptionService.cs
- XmlReflectionImporter.cs
- Connection.cs
- GlyphingCache.cs
- PartialClassGenerationTaskInternal.cs
- PropertyGridView.cs
- ClientConvert.cs