Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SapiInterop / SapiRecoContext.cs / 1 / SapiRecoContext.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // All the calls to SAPI interfaces are wraped into the class 'SapiRecognizer', // 'SapiContext' and 'SapiGrammar'. // // The SAPI call are executed in the context of a proxy that is either a // pass-through or forward the request to an MTA proxy for SAPI 5.1 // // History: // 4/1/2006 jeanfp //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Speech.Recognition; using System.Speech.Internal.ObjectTokens; using System.Runtime.InteropServices; using System.Text; namespace System.Speech.Internal.SapiInterop { internal class SapiRecoContext : IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors // This constuctor must be called in the context of the backgroung proxy if any internal SapiRecoContext (ISpRecoContext recoContext, SapiProxy proxy) { _recoContext = recoContext; _proxy = proxy; } public void Dispose () { if (!_disposed) { // Called from the client proxy _proxy.Invoke2 (delegate { Marshal.ReleaseComObject (_recoContext); }); _disposed = true; } GC.SuppressFinalize (this); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods internal void SetInterest (UInt64 eventInterest, UInt64 queuedInterest) { _proxy.Invoke2 (delegate { _recoContext.SetInterest (eventInterest, queuedInterest); }); } internal SapiGrammar CreateGrammar (UInt64 id) { ISpRecoGrammar sapiGrammar; return (SapiGrammar) _proxy.Invoke (delegate { _recoContext.CreateGrammar (id, out sapiGrammar); return new SapiGrammar (sapiGrammar, _proxy); }); } internal void SetMaxAlternates (UInt32 count) { _proxy.Invoke2 (delegate { _recoContext.SetMaxAlternates (count); }); } internal void SetAudioOptions (SPAUDIOOPTIONS options, IntPtr audioFormatId, IntPtr waveFormatEx) { _proxy.Invoke2 (delegate { _recoContext.SetAudioOptions (options, audioFormatId, waveFormatEx); }); } internal void Bookmark (SPBOOKMARKOPTIONS options, UInt64 position, IntPtr lparam) { _proxy.Invoke2 (delegate { _recoContext.Bookmark (options, position, lparam); }); } internal void Resume () { _proxy.Invoke2 (delegate { _recoContext.Resume (0); }); } internal void SetContextState (SPCONTEXTSTATE state) { _proxy.Invoke2 (delegate { _recoContext.SetContextState (state); }); } internal EventNotify CreateEventNotify (AsyncSerializedWorker asyncWorker, bool supportsSapi53) { return (EventNotify) _proxy.Invoke (delegate { return new EventNotify ((ISpEventSource) _recoContext, asyncWorker, supportsSapi53); }); } internal void DisposeEventNotify (EventNotify eventNotify) { _proxy.Invoke2 (delegate { eventNotify.Dispose (); }); } internal void SetGrammarOptions (SPGRAMMAROPTIONS options) { _proxy.Invoke2 (delegate { ((ISpRecoContext2) _recoContext).SetGrammarOptions (options); }); } #endregion //******************************************************************** // // Private Fields // //******************************************************************** #region Private Fields private ISpRecoContext _recoContext; private SapiProxy _proxy; private bool _disposed; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // All the calls to SAPI interfaces are wraped into the class 'SapiRecognizer', // 'SapiContext' and 'SapiGrammar'. // // The SAPI call are executed in the context of a proxy that is either a // pass-through or forward the request to an MTA proxy for SAPI 5.1 // // History: // 4/1/2006 jeanfp //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Speech.Recognition; using System.Speech.Internal.ObjectTokens; using System.Runtime.InteropServices; using System.Text; namespace System.Speech.Internal.SapiInterop { internal class SapiRecoContext : IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors // This constuctor must be called in the context of the backgroung proxy if any internal SapiRecoContext (ISpRecoContext recoContext, SapiProxy proxy) { _recoContext = recoContext; _proxy = proxy; } public void Dispose () { if (!_disposed) { // Called from the client proxy _proxy.Invoke2 (delegate { Marshal.ReleaseComObject (_recoContext); }); _disposed = true; } GC.SuppressFinalize (this); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods internal void SetInterest (UInt64 eventInterest, UInt64 queuedInterest) { _proxy.Invoke2 (delegate { _recoContext.SetInterest (eventInterest, queuedInterest); }); } internal SapiGrammar CreateGrammar (UInt64 id) { ISpRecoGrammar sapiGrammar; return (SapiGrammar) _proxy.Invoke (delegate { _recoContext.CreateGrammar (id, out sapiGrammar); return new SapiGrammar (sapiGrammar, _proxy); }); } internal void SetMaxAlternates (UInt32 count) { _proxy.Invoke2 (delegate { _recoContext.SetMaxAlternates (count); }); } internal void SetAudioOptions (SPAUDIOOPTIONS options, IntPtr audioFormatId, IntPtr waveFormatEx) { _proxy.Invoke2 (delegate { _recoContext.SetAudioOptions (options, audioFormatId, waveFormatEx); }); } internal void Bookmark (SPBOOKMARKOPTIONS options, UInt64 position, IntPtr lparam) { _proxy.Invoke2 (delegate { _recoContext.Bookmark (options, position, lparam); }); } internal void Resume () { _proxy.Invoke2 (delegate { _recoContext.Resume (0); }); } internal void SetContextState (SPCONTEXTSTATE state) { _proxy.Invoke2 (delegate { _recoContext.SetContextState (state); }); } internal EventNotify CreateEventNotify (AsyncSerializedWorker asyncWorker, bool supportsSapi53) { return (EventNotify) _proxy.Invoke (delegate { return new EventNotify ((ISpEventSource) _recoContext, asyncWorker, supportsSapi53); }); } internal void DisposeEventNotify (EventNotify eventNotify) { _proxy.Invoke2 (delegate { eventNotify.Dispose (); }); } internal void SetGrammarOptions (SPGRAMMAROPTIONS options) { _proxy.Invoke2 (delegate { ((ISpRecoContext2) _recoContext).SetGrammarOptions (options); }); } #endregion //******************************************************************** // // Private Fields // //******************************************************************** #region Private Fields private ISpRecoContext _recoContext; private SapiProxy _proxy; private bool _disposed; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
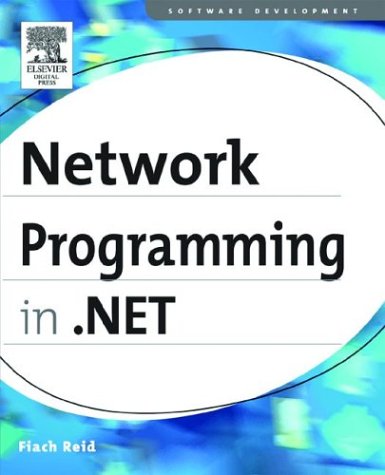
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AnonymousIdentificationModule.cs
- TransformPatternIdentifiers.cs
- JapaneseCalendar.cs
- SortedList.cs
- XMLDiffLoader.cs
- Action.cs
- ConnectionManagementElementCollection.cs
- PopupRoot.cs
- MulticastDelegate.cs
- TreeViewAutomationPeer.cs
- DesignConnectionCollection.cs
- TableFieldsEditor.cs
- RemoveStoryboard.cs
- ClientRoleProvider.cs
- ConnectionConsumerAttribute.cs
- ClonableStack.cs
- ManagementException.cs
- ObjectConverter.cs
- SafeNativeMethodsCLR.cs
- PropertyEntry.cs
- EntityDataSourceQueryBuilder.cs
- Debug.cs
- Point3DAnimationUsingKeyFrames.cs
- DiscreteKeyFrames.cs
- XmlHelper.cs
- MenuItem.cs
- SourceSwitch.cs
- ProviderException.cs
- LockedBorderGlyph.cs
- GenericTypeParameterBuilder.cs
- SslStream.cs
- TextRangeBase.cs
- ServicePointManager.cs
- SequentialOutput.cs
- ControlEvent.cs
- TemplateControlParser.cs
- TabPage.cs
- DataGridViewSelectedColumnCollection.cs
- ClosableStream.cs
- PageParser.cs
- UmAlQuraCalendar.cs
- Resources.Designer.cs
- TemplatedAdorner.cs
- DialogResultConverter.cs
- OdbcEnvironmentHandle.cs
- ParagraphVisual.cs
- SystemPens.cs
- MruCache.cs
- MultipartContentParser.cs
- LoginCancelEventArgs.cs
- SiteOfOriginContainer.cs
- ObjectDataSourceFilteringEventArgs.cs
- RichTextBoxAutomationPeer.cs
- WebPartUserCapability.cs
- ElapsedEventArgs.cs
- MetafileHeader.cs
- MultipartContentParser.cs
- CalendarSelectionChangedEventArgs.cs
- Themes.cs
- Root.cs
- ConfigurationErrorsException.cs
- KeyGestureConverter.cs
- VariantWrapper.cs
- LinqDataSource.cs
- TypeConverterHelper.cs
- WrappingXamlSchemaContext.cs
- MenuItemBindingCollection.cs
- ReversePositionQuery.cs
- PathFigureCollection.cs
- RTLAwareMessageBox.cs
- Membership.cs
- StatusBarAutomationPeer.cs
- EntityModelBuildProvider.cs
- PhysicalFontFamily.cs
- TableLayoutStyle.cs
- MeshGeometry3D.cs
- Tokenizer.cs
- WebEventCodes.cs
- MenuCommand.cs
- SqlErrorCollection.cs
- OledbConnectionStringbuilder.cs
- MissingSatelliteAssemblyException.cs
- SiteMapDataSourceView.cs
- TimeSpanOrInfiniteValidator.cs
- AttachmentCollection.cs
- XmlSchemaIdentityConstraint.cs
- RequestSecurityTokenSerializer.cs
- AnimationTimeline.cs
- PropertyKey.cs
- ScriptControl.cs
- recordstatescratchpad.cs
- AnonymousIdentificationModule.cs
- RightsManagementEncryptionTransform.cs
- KeyGestureValueSerializer.cs
- UserPreferenceChangedEventArgs.cs
- TextServicesProperty.cs
- ExtentCqlBlock.cs
- XPathEmptyIterator.cs
- Timer.cs
- FlowDocumentPaginator.cs