Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Recognition / SrgsGrammar / SrgsOneOf.cs / 1 / SrgsOneOf.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Globalization; using System.Speech.Internal; using System.Speech.Internal.SrgsParser; using System.Text; using System.Xml; namespace System.Speech.Recognition.SrgsGrammar { /// TODOC <_include file='doc\OneOf.uex' path='docs/doc[@for="OneOf"]/*' /> [Serializable] [DebuggerDisplay ("{DebuggerDisplayString ()}")] [DebuggerTypeProxy (typeof (OneOfDebugDisplay))] public class SrgsOneOf : SrgsElement, IOneOf { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors /// TODOC <_include file='doc\OneOf.uex' path='docs/doc[@for="OneOf.OneOf1"]/*' /> public SrgsOneOf () { } /// TODOC <_include file='doc\OneOf.uex' path='docs/doc[@for="OneOf.OneOf2"]/*' /> public SrgsOneOf (params string [] items) : this () { Helpers.ThrowIfNull (items, "items"); for (int i = 0; i < items.Length; i++) { if (items [i] == null) { throw new ArgumentNullException ("items", SR.Get (SRID.ParamsEntryNullIllegal)); } _items.Add (new SrgsItem (items [i])); } } /// TODOC <_include file='doc\OneOf.uex' path='docs/doc[@for="OneOf.OneOf3"]/*' /> public SrgsOneOf (params SrgsItem [] items) : this () { Helpers.ThrowIfNull (items, "items"); for (int i = 0; i < items.Length; i++) { SrgsItem item = items [i]; if (item == null) { throw new ArgumentNullException ("items", SR.Get (SRID.ParamsEntryNullIllegal)); } _items.Add (item); } } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region public Method ////// TODOC /// /// public void Add (SrgsItem item) { Helpers.ThrowIfNull (item, "item"); Items.Add (item); } #endregion //******************************************************************** // // Public Properties // //******************************************************************** #region public Properties // ISSUE: Do we need more construcors? Take a look at RuleElementCollection.AddOneOf methods. [Bug# 37115] /// TODOC <_include file='doc\OneOf.uex' path='docs/doc[@for="OneOf.Elements"]/*' /> public CollectionItems { get { return _items; } } #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region internal Methods internal override void WriteSrgs (XmlWriter writer) { // Write ... writer.WriteStartElement ("one-of"); foreach (SrgsItem element in _items) { element.WriteSrgs (writer); } writer.WriteEndElement (); } internal override string DebuggerDisplayString () { StringBuilder sb = new StringBuilder ("SrgsOneOf Count = "); sb.Append (_items.Count); return sb.ToString (); } #endregion //******************************************************************* // // Protected Properties // //******************************************************************* #region Protected Properties ////// Allows the Srgs Element base class to implement /// features requiring recursion in the elements tree. /// ///internal override SrgsElement [] Children { get { SrgsElement [] elements = new SrgsElement [_items.Count]; int i = 0; foreach (SrgsItem item in _items) { elements [i++] = item; } return elements; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private SrgsItemList _items = new SrgsItemList (); #endregion //******************************************************************* // // Private Types // //******************************************************************** #region Private Types // Used by the debbugger display attribute internal class OneOfDebugDisplay { public OneOfDebugDisplay (SrgsOneOf oneOf) { _items = oneOf._items; } [DebuggerBrowsable (DebuggerBrowsableState.RootHidden)] public SrgsItem [] AKeys { get { SrgsItem [] items = new SrgsItem [_items.Count]; for (int i = 0; i < _items.Count; i++) { items [i] = _items [i]; } return items; } } private Collection _items; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Globalization; using System.Speech.Internal; using System.Speech.Internal.SrgsParser; using System.Text; using System.Xml; namespace System.Speech.Recognition.SrgsGrammar { /// TODOC <_include file='doc\OneOf.uex' path='docs/doc[@for="OneOf"]/*' /> [Serializable] [DebuggerDisplay ("{DebuggerDisplayString ()}")] [DebuggerTypeProxy (typeof (OneOfDebugDisplay))] public class SrgsOneOf : SrgsElement, IOneOf { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors /// TODOC <_include file='doc\OneOf.uex' path='docs/doc[@for="OneOf.OneOf1"]/*' /> public SrgsOneOf () { } /// TODOC <_include file='doc\OneOf.uex' path='docs/doc[@for="OneOf.OneOf2"]/*' /> public SrgsOneOf (params string [] items) : this () { Helpers.ThrowIfNull (items, "items"); for (int i = 0; i < items.Length; i++) { if (items [i] == null) { throw new ArgumentNullException ("items", SR.Get (SRID.ParamsEntryNullIllegal)); } _items.Add (new SrgsItem (items [i])); } } /// TODOC <_include file='doc\OneOf.uex' path='docs/doc[@for="OneOf.OneOf3"]/*' /> public SrgsOneOf (params SrgsItem [] items) : this () { Helpers.ThrowIfNull (items, "items"); for (int i = 0; i < items.Length; i++) { SrgsItem item = items [i]; if (item == null) { throw new ArgumentNullException ("items", SR.Get (SRID.ParamsEntryNullIllegal)); } _items.Add (item); } } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region public Method ////// TODOC /// /// public void Add (SrgsItem item) { Helpers.ThrowIfNull (item, "item"); Items.Add (item); } #endregion //******************************************************************** // // Public Properties // //******************************************************************** #region public Properties // ISSUE: Do we need more construcors? Take a look at RuleElementCollection.AddOneOf methods. [Bug# 37115] /// TODOC <_include file='doc\OneOf.uex' path='docs/doc[@for="OneOf.Elements"]/*' /> public CollectionItems { get { return _items; } } #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region internal Methods internal override void WriteSrgs (XmlWriter writer) { // Write ... writer.WriteStartElement ("one-of"); foreach (SrgsItem element in _items) { element.WriteSrgs (writer); } writer.WriteEndElement (); } internal override string DebuggerDisplayString () { StringBuilder sb = new StringBuilder ("SrgsOneOf Count = "); sb.Append (_items.Count); return sb.ToString (); } #endregion //******************************************************************* // // Protected Properties // //******************************************************************* #region Protected Properties ////// Allows the Srgs Element base class to implement /// features requiring recursion in the elements tree. /// ///internal override SrgsElement [] Children { get { SrgsElement [] elements = new SrgsElement [_items.Count]; int i = 0; foreach (SrgsItem item in _items) { elements [i++] = item; } return elements; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private SrgsItemList _items = new SrgsItemList (); #endregion //******************************************************************* // // Private Types // //******************************************************************** #region Private Types // Used by the debbugger display attribute internal class OneOfDebugDisplay { public OneOfDebugDisplay (SrgsOneOf oneOf) { _items = oneOf._items; } [DebuggerBrowsable (DebuggerBrowsableState.RootHidden)] public SrgsItem [] AKeys { get { SrgsItem [] items = new SrgsItem [_items.Count]; for (int i = 0; i < _items.Count; i++) { items [i] = _items [i]; } return items; } } private Collection _items; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
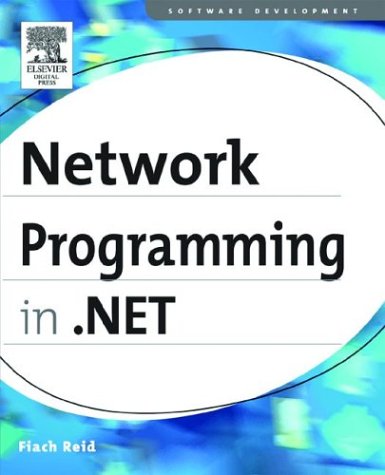
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServiceException.cs
- PublisherIdentityPermission.cs
- AudienceUriMode.cs
- Int32KeyFrameCollection.cs
- InstallerTypeAttribute.cs
- OpenTypeLayoutCache.cs
- columnmapfactory.cs
- QilGenerator.cs
- ComponentSerializationService.cs
- BindingCollection.cs
- _MultipleConnectAsync.cs
- Reference.cs
- RectIndependentAnimationStorage.cs
- AsyncStreamReader.cs
- PersonalizationProviderHelper.cs
- ScaleTransform.cs
- PropertyGridCommands.cs
- SourceElementsCollection.cs
- ExtendedPropertyDescriptor.cs
- EntityContainerEntitySetDefiningQuery.cs
- TrustLevel.cs
- ZipPackagePart.cs
- StyleHelper.cs
- Vector3DCollectionConverter.cs
- ExtendedProtectionPolicy.cs
- UIElementHelper.cs
- FileUpload.cs
- InputDevice.cs
- DataGridViewTextBoxCell.cs
- WebEventTraceProvider.cs
- ButtonFlatAdapter.cs
- CultureInfoConverter.cs
- SamlSecurityToken.cs
- SwitchElementsCollection.cs
- UndoManager.cs
- XDeferredAxisSource.cs
- GetCryptoTransformRequest.cs
- DomNameTable.cs
- ContravarianceAdapter.cs
- OletxTransactionFormatter.cs
- CustomError.cs
- InternalPolicyElement.cs
- LayoutInformation.cs
- SpinLock.cs
- CssClassPropertyAttribute.cs
- RecordManager.cs
- TabItemWrapperAutomationPeer.cs
- UnsafeNativeMethods.cs
- SqlExpander.cs
- DictionarySectionHandler.cs
- TransformPatternIdentifiers.cs
- MetadataArtifactLoaderCompositeResource.cs
- CatalogZone.cs
- PolygonHotSpot.cs
- WorkflowDataContext.cs
- IChannel.cs
- XpsDocument.cs
- ArrayTypeMismatchException.cs
- QueryCacheKey.cs
- ItemsPanelTemplate.cs
- XmlSecureResolver.cs
- CacheOutputQuery.cs
- EncoderExceptionFallback.cs
- Form.cs
- ZipIOExtraField.cs
- Graphics.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- EventHandlersStore.cs
- PersistNameAttribute.cs
- ScriptServiceAttribute.cs
- SizeAnimation.cs
- XmlHierarchyData.cs
- ParserExtension.cs
- LayoutInformation.cs
- CommandPlan.cs
- DescendantOverDescendantQuery.cs
- XmlValueConverter.cs
- TraceEventCache.cs
- TextEvent.cs
- SubMenuStyleCollection.cs
- WebDescriptionAttribute.cs
- EventLogEntryCollection.cs
- DBDataPermissionAttribute.cs
- SecurityToken.cs
- Pointer.cs
- WorkflowControlEndpoint.cs
- TypeDescriptor.cs
- PowerModeChangedEventArgs.cs
- XamlParser.cs
- AnnotationHelper.cs
- CustomWebEventKey.cs
- UrlAuthFailedErrorFormatter.cs
- XmlSchemaInclude.cs
- CheckoutException.cs
- BypassElementCollection.cs
- SamlSecurityTokenAuthenticator.cs
- ParameterModifier.cs
- InsufficientMemoryException.cs
- MenuBindingsEditor.cs
- XPathDocumentIterator.cs