Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntityDesign / Design / System / Data / EntityModel / Emitters / TypeReference.cs / 1 / TypeReference.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.CodeDom; using System.Collections; using System.Collections.Generic; using System.Data.EntityModel.SchemaObjectModel; using System.Data.Common.Utils; using System.Reflection; namespace System.Data.EntityModel.Emitters { ////// Summary description for TypeReferences. /// internal class TypeReference { #region Fields internal static readonly Type ComplexTypeBaseClassType = typeof(System.Data.Objects.DataClasses.ComplexObject); internal static readonly Type EntityTypeBaseClassType = typeof(System.Data.Objects.DataClasses.EntityObject); private const string IEntityWithRelationshipsTypeBaseClassName = "IEntityWithRelationships"; private const string NewContextClassName = "ObjectContext"; private const string EntitySetClassName = "EntitySet"; private const string ObjectResultClassName = "ObjectResult"; public const string FQMetaDataWorkspaceTypeName = "System.Data.Metadata.Edm.MetadataWorkspace"; private static CodeTypeReference _byteArray; private static CodeTypeReference _dateTime; private static CodeTypeReference _guid; private static CodeTypeReference _objectContext; private static CodeTypeReference _string; private readonly Memoizer_forTypeMemoizer; private readonly Memoizer _nullableForTypeMemoizer; private readonly Memoizer , CodeTypeReference> _fromStringMemoizer; private readonly Memoizer , CodeTypeReference> _fromStringGenericMemoizer; #endregion #region Constructors internal TypeReference() { _forTypeMemoizer = new Memoizer (ComputeForType, null); _fromStringMemoizer = new Memoizer , CodeTypeReference>(ComputeFromString, null); _nullableForTypeMemoizer = new Memoizer (ComputeNullableForType, null); _fromStringGenericMemoizer = new Memoizer , CodeTypeReference>(ComputeFromStringGeneric, null); } #endregion #region Public Methods /// /// Get TypeReference for a type represented by a Type object /// /// the type object ///the associated TypeReference object public CodeTypeReference ForType(Type type) { return _forTypeMemoizer.Evaluate(type); } private CodeTypeReference ComputeForType(Type type) { // we know that we can safely global:: qualify this because it was already // compiled before we are emitting or else we wouldn't have a Type object CodeTypeReference value = new CodeTypeReference(type, CodeTypeReferenceOptions.GlobalReference); return value; } ////// Get TypeReference for a type represented by a Generic Type object. /// We don't cache the TypeReference for generic type object since the type would be the same /// irresepective of the generic arguments. We could potentially cache it using both the type name /// and generic type arguments. /// /// the generic type object ///the associated TypeReference object public CodeTypeReference ForType(Type generic, CodeTypeReference argument) { // we know that we can safely global:: qualify this because it was already // compiled before we are emitting or else we wouldn't have a Type object CodeTypeReference typeRef = new CodeTypeReference(generic, CodeTypeReferenceOptions.GlobalReference); typeRef.TypeArguments.Add(argument); return typeRef; } ////// Get TypeReference for a type represented by a namespace quailifed string /// /// namespace qualified string ///the TypeReference public CodeTypeReference FromString(string type) { return FromString(type, false); } ////// Get TypeReference for a type represented by a namespace quailifed string, /// with optional global qualifier /// /// namespace qualified string /// indicates whether the global qualifier should be added ///the TypeReference public CodeTypeReference FromString(string type, bool addGlobalQualifier) { return _fromStringMemoizer.Evaluate(new KeyValuePair(type, addGlobalQualifier)); } private CodeTypeReference ComputeFromString(KeyValuePair arguments) { string type = arguments.Key; bool addGlobalQualifier = arguments.Value; CodeTypeReference value; if (addGlobalQualifier) { value = new CodeTypeReference(type, CodeTypeReferenceOptions.GlobalReference); } else { value = new CodeTypeReference(type); } return value; } /// /// Get TypeReference for a framework type /// /// unqualified name of the framework type ///the TypeReference public CodeTypeReference AdoFrameworkType(string name) { return FromString(Utils.FQAdoFrameworkName(name), true); } ////// Get TypeReference for a framework DataClasses type /// /// unqualified name of the framework DataClass type ///the TypeReference public CodeTypeReference AdoFrameworkDataClassesType(string name) { return FromString(Utils.FQAdoFrameworkDataClassesName(name), true); } ////// Get TypeReference for a framework Metadata Edm type /// /// unqualified name of the framework metadata edm type ///the TypeReference public CodeTypeReference AdoFrameworkMetadataEdmType(string name) { return FromString(Utils.FQAdoFrameworkMetadataEdmName(name), true); } ////// Get TypeReference for a framework Entity Client type /// /// unqualified name of the framework type ///the TypeReference public CodeTypeReference AdoEntityClientType(string name) { return FromString(Utils.FQAdoEntityClientName(name), true); } ////// Get TypeReference for a bound generic framework class /// /// the name of the generic framework class /// the type parameter for the framework class ///TypeReference for the bound framework class public CodeTypeReference AdoFrameworkGenericClass(string name, CodeTypeReference typeParameter) { return FrameworkGenericClass(Utils.AdoFrameworkNamespace, name, typeParameter); } ////// Get TypeReference for a bound generic framework data class /// /// the name of the generic framework data class /// the type parameter for the framework data class ///TypeReference for the bound framework data class public CodeTypeReference AdoFrameworkGenericDataClass(string name, CodeTypeReference typeParameter) { return FrameworkGenericClass(Utils.AdoFrameworkDataClassesNamespace, name, typeParameter); } ////// Get TypeReference for a bound generic framework class /// /// the namespace of the generic framework class /// the name of the generic framework class /// the type parameter for the framework class ///TypeReference for the bound framework class private CodeTypeReference FrameworkGenericClass(string namespaceName, string name, CodeTypeReference typeParameter) { return _fromStringGenericMemoizer.Evaluate(new KeyValuePair(namespaceName + "." + name, typeParameter)); } private CodeTypeReference ComputeFromStringGeneric(KeyValuePair arguments) { string name = arguments.Key; CodeTypeReference typeParameter = arguments.Value; CodeTypeReference typeRef = ComputeFromString(new KeyValuePair (name, true)); typeRef.TypeArguments.Add(typeParameter); return typeRef; } /// /// Get TypeReference for a bound Nullable<T> /// /// Type of the Nullable<T> type parameter ///TypeReference for a bound Nullable<T> public CodeTypeReference NullableForType(Type innerType) { return _nullableForTypeMemoizer.Evaluate(innerType); } private CodeTypeReference ComputeNullableForType(Type innerType) { // can't use FromString because it will return the same Generic type reference // but it will already have a previous type parameter (because of caching) CodeTypeReference typeRef = new CodeTypeReference(typeof(System.Nullable<>), CodeTypeReferenceOptions.GlobalReference); typeRef.TypeArguments.Add(ForType(innerType)); return typeRef; } ////// Gets an ObjectResult of elementType CodeTypeReference. /// public CodeTypeReference ObjectResult(CodeTypeReference elementType) { return AdoFrameworkGenericClass(ObjectResultClassName, elementType); } #endregion #region Public Properties ////// Gets a CodeTypeReference to the System.Byte[] type. /// ///public CodeTypeReference ByteArray { get { if (_byteArray == null) _byteArray = ForType(typeof(byte[])); return _byteArray; } } /// /// Gets a CodeTypeReference object for the System.DateTime type. /// public CodeTypeReference DateTime { get { if (_dateTime == null) _dateTime = ForType(typeof(System.DateTime)); return _dateTime; } } ////// Gets a CodeTypeReference object for the System.Guid type. /// public CodeTypeReference Guid { get { if (_guid == null) _guid = ForType(typeof(System.Guid)); return _guid; } } ////// TypeReference for the Framework's ObjectContext class /// public CodeTypeReference ObjectContext { get { if (_objectContext == null) _objectContext = AdoFrameworkType(NewContextClassName); return _objectContext; } } ////// TypeReference for the Framework base class to types that can used in InlineObjectCollection /// public CodeTypeReference ComplexTypeBaseClass { get { return ForType(ComplexTypeBaseClassType); } } ////// TypeReference for the Framework base class for EntityTypes /// public CodeTypeReference EntityTypeBaseClass { get { return ForType(EntityTypeBaseClassType); } } ////// TypeReference for the Framework base class for IEntityWithRelationships /// public CodeTypeReference IEntityWithRelationshipsTypeBaseClass { get { return AdoFrameworkDataClassesType(IEntityWithRelationshipsTypeBaseClassName); } } ////// Gets a CodeTypeReference object for the System.String type. /// public CodeTypeReference String { get { if (_string == null) _string = ForType(typeof(string)); return _string; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.CodeDom; using System.Collections; using System.Collections.Generic; using System.Data.EntityModel.SchemaObjectModel; using System.Data.Common.Utils; using System.Reflection; namespace System.Data.EntityModel.Emitters { ////// Summary description for TypeReferences. /// internal class TypeReference { #region Fields internal static readonly Type ComplexTypeBaseClassType = typeof(System.Data.Objects.DataClasses.ComplexObject); internal static readonly Type EntityTypeBaseClassType = typeof(System.Data.Objects.DataClasses.EntityObject); private const string IEntityWithRelationshipsTypeBaseClassName = "IEntityWithRelationships"; private const string NewContextClassName = "ObjectContext"; private const string EntitySetClassName = "EntitySet"; private const string ObjectResultClassName = "ObjectResult"; public const string FQMetaDataWorkspaceTypeName = "System.Data.Metadata.Edm.MetadataWorkspace"; private static CodeTypeReference _byteArray; private static CodeTypeReference _dateTime; private static CodeTypeReference _guid; private static CodeTypeReference _objectContext; private static CodeTypeReference _string; private readonly Memoizer_forTypeMemoizer; private readonly Memoizer _nullableForTypeMemoizer; private readonly Memoizer , CodeTypeReference> _fromStringMemoizer; private readonly Memoizer , CodeTypeReference> _fromStringGenericMemoizer; #endregion #region Constructors internal TypeReference() { _forTypeMemoizer = new Memoizer (ComputeForType, null); _fromStringMemoizer = new Memoizer , CodeTypeReference>(ComputeFromString, null); _nullableForTypeMemoizer = new Memoizer (ComputeNullableForType, null); _fromStringGenericMemoizer = new Memoizer , CodeTypeReference>(ComputeFromStringGeneric, null); } #endregion #region Public Methods /// /// Get TypeReference for a type represented by a Type object /// /// the type object ///the associated TypeReference object public CodeTypeReference ForType(Type type) { return _forTypeMemoizer.Evaluate(type); } private CodeTypeReference ComputeForType(Type type) { // we know that we can safely global:: qualify this because it was already // compiled before we are emitting or else we wouldn't have a Type object CodeTypeReference value = new CodeTypeReference(type, CodeTypeReferenceOptions.GlobalReference); return value; } ////// Get TypeReference for a type represented by a Generic Type object. /// We don't cache the TypeReference for generic type object since the type would be the same /// irresepective of the generic arguments. We could potentially cache it using both the type name /// and generic type arguments. /// /// the generic type object ///the associated TypeReference object public CodeTypeReference ForType(Type generic, CodeTypeReference argument) { // we know that we can safely global:: qualify this because it was already // compiled before we are emitting or else we wouldn't have a Type object CodeTypeReference typeRef = new CodeTypeReference(generic, CodeTypeReferenceOptions.GlobalReference); typeRef.TypeArguments.Add(argument); return typeRef; } ////// Get TypeReference for a type represented by a namespace quailifed string /// /// namespace qualified string ///the TypeReference public CodeTypeReference FromString(string type) { return FromString(type, false); } ////// Get TypeReference for a type represented by a namespace quailifed string, /// with optional global qualifier /// /// namespace qualified string /// indicates whether the global qualifier should be added ///the TypeReference public CodeTypeReference FromString(string type, bool addGlobalQualifier) { return _fromStringMemoizer.Evaluate(new KeyValuePair(type, addGlobalQualifier)); } private CodeTypeReference ComputeFromString(KeyValuePair arguments) { string type = arguments.Key; bool addGlobalQualifier = arguments.Value; CodeTypeReference value; if (addGlobalQualifier) { value = new CodeTypeReference(type, CodeTypeReferenceOptions.GlobalReference); } else { value = new CodeTypeReference(type); } return value; } /// /// Get TypeReference for a framework type /// /// unqualified name of the framework type ///the TypeReference public CodeTypeReference AdoFrameworkType(string name) { return FromString(Utils.FQAdoFrameworkName(name), true); } ////// Get TypeReference for a framework DataClasses type /// /// unqualified name of the framework DataClass type ///the TypeReference public CodeTypeReference AdoFrameworkDataClassesType(string name) { return FromString(Utils.FQAdoFrameworkDataClassesName(name), true); } ////// Get TypeReference for a framework Metadata Edm type /// /// unqualified name of the framework metadata edm type ///the TypeReference public CodeTypeReference AdoFrameworkMetadataEdmType(string name) { return FromString(Utils.FQAdoFrameworkMetadataEdmName(name), true); } ////// Get TypeReference for a framework Entity Client type /// /// unqualified name of the framework type ///the TypeReference public CodeTypeReference AdoEntityClientType(string name) { return FromString(Utils.FQAdoEntityClientName(name), true); } ////// Get TypeReference for a bound generic framework class /// /// the name of the generic framework class /// the type parameter for the framework class ///TypeReference for the bound framework class public CodeTypeReference AdoFrameworkGenericClass(string name, CodeTypeReference typeParameter) { return FrameworkGenericClass(Utils.AdoFrameworkNamespace, name, typeParameter); } ////// Get TypeReference for a bound generic framework data class /// /// the name of the generic framework data class /// the type parameter for the framework data class ///TypeReference for the bound framework data class public CodeTypeReference AdoFrameworkGenericDataClass(string name, CodeTypeReference typeParameter) { return FrameworkGenericClass(Utils.AdoFrameworkDataClassesNamespace, name, typeParameter); } ////// Get TypeReference for a bound generic framework class /// /// the namespace of the generic framework class /// the name of the generic framework class /// the type parameter for the framework class ///TypeReference for the bound framework class private CodeTypeReference FrameworkGenericClass(string namespaceName, string name, CodeTypeReference typeParameter) { return _fromStringGenericMemoizer.Evaluate(new KeyValuePair(namespaceName + "." + name, typeParameter)); } private CodeTypeReference ComputeFromStringGeneric(KeyValuePair arguments) { string name = arguments.Key; CodeTypeReference typeParameter = arguments.Value; CodeTypeReference typeRef = ComputeFromString(new KeyValuePair (name, true)); typeRef.TypeArguments.Add(typeParameter); return typeRef; } /// /// Get TypeReference for a bound Nullable<T> /// /// Type of the Nullable<T> type parameter ///TypeReference for a bound Nullable<T> public CodeTypeReference NullableForType(Type innerType) { return _nullableForTypeMemoizer.Evaluate(innerType); } private CodeTypeReference ComputeNullableForType(Type innerType) { // can't use FromString because it will return the same Generic type reference // but it will already have a previous type parameter (because of caching) CodeTypeReference typeRef = new CodeTypeReference(typeof(System.Nullable<>), CodeTypeReferenceOptions.GlobalReference); typeRef.TypeArguments.Add(ForType(innerType)); return typeRef; } ////// Gets an ObjectResult of elementType CodeTypeReference. /// public CodeTypeReference ObjectResult(CodeTypeReference elementType) { return AdoFrameworkGenericClass(ObjectResultClassName, elementType); } #endregion #region Public Properties ////// Gets a CodeTypeReference to the System.Byte[] type. /// ///public CodeTypeReference ByteArray { get { if (_byteArray == null) _byteArray = ForType(typeof(byte[])); return _byteArray; } } /// /// Gets a CodeTypeReference object for the System.DateTime type. /// public CodeTypeReference DateTime { get { if (_dateTime == null) _dateTime = ForType(typeof(System.DateTime)); return _dateTime; } } ////// Gets a CodeTypeReference object for the System.Guid type. /// public CodeTypeReference Guid { get { if (_guid == null) _guid = ForType(typeof(System.Guid)); return _guid; } } ////// TypeReference for the Framework's ObjectContext class /// public CodeTypeReference ObjectContext { get { if (_objectContext == null) _objectContext = AdoFrameworkType(NewContextClassName); return _objectContext; } } ////// TypeReference for the Framework base class to types that can used in InlineObjectCollection /// public CodeTypeReference ComplexTypeBaseClass { get { return ForType(ComplexTypeBaseClassType); } } ////// TypeReference for the Framework base class for EntityTypes /// public CodeTypeReference EntityTypeBaseClass { get { return ForType(EntityTypeBaseClassType); } } ////// TypeReference for the Framework base class for IEntityWithRelationships /// public CodeTypeReference IEntityWithRelationshipsTypeBaseClass { get { return AdoFrameworkDataClassesType(IEntityWithRelationshipsTypeBaseClassName); } } ////// Gets a CodeTypeReference object for the System.String type. /// public CodeTypeReference String { get { if (_string == null) _string = ForType(typeof(string)); return _string; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
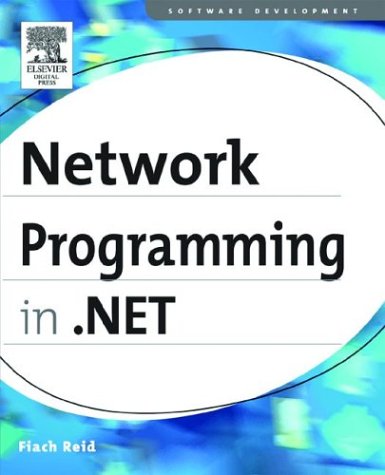
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DrawingContextWalker.cs
- ObjectConverter.cs
- UnknownMessageReceivedEventArgs.cs
- Marshal.cs
- NGCUIElementCollectionSerializerAsync.cs
- Lease.cs
- ProfileBuildProvider.cs
- HtmlContainerControl.cs
- securitycriticaldataClass.cs
- Timeline.cs
- UpDownBase.cs
- DataViewSettingCollection.cs
- BuildProviderUtils.cs
- ValidationEventArgs.cs
- EtwProvider.cs
- StackOverflowException.cs
- StylusShape.cs
- Int64.cs
- TransformProviderWrapper.cs
- VisualProxy.cs
- XsltArgumentList.cs
- DesignerAutoFormatCollection.cs
- PerformanceCounterLib.cs
- SuppressMergeCheckAttribute.cs
- SamlAssertion.cs
- HttpModule.cs
- InternalBufferOverflowException.cs
- MD5.cs
- SessionParameter.cs
- PrimitiveSchema.cs
- UserValidatedEventArgs.cs
- XmlLanguageConverter.cs
- DrawingImage.cs
- JavaScriptString.cs
- ExpressionParser.cs
- RowUpdatedEventArgs.cs
- DispatchChannelSink.cs
- XmlSchemaAll.cs
- WindowsListViewGroupHelper.cs
- Operand.cs
- WindowsPen.cs
- SelectionRange.cs
- BuildProvidersCompiler.cs
- UriPrefixTable.cs
- WebPartMenuStyle.cs
- ScopelessEnumAttribute.cs
- DesigntimeLicenseContextSerializer.cs
- WorkItem.cs
- serverconfig.cs
- BinaryMethodMessage.cs
- XmlAnyElementAttributes.cs
- ConfigXmlDocument.cs
- PartialCachingControl.cs
- WindowsToolbar.cs
- StrokeCollectionConverter.cs
- ProxyWebPartManager.cs
- DispatchChannelSink.cs
- WindowsFont.cs
- TouchesOverProperty.cs
- FormViewInsertEventArgs.cs
- CatalogZone.cs
- KnownTypeHelper.cs
- HierarchicalDataBoundControl.cs
- ApplicationTrust.cs
- WebPartsSection.cs
- ConsumerConnectionPointCollection.cs
- ConcurrentDictionary.cs
- LayoutTableCell.cs
- AddingNewEventArgs.cs
- PropertyGeneratedEventArgs.cs
- ObjectQuery_EntitySqlExtensions.cs
- ResourceExpressionBuilder.cs
- SerializerDescriptor.cs
- TimeSpanSecondsConverter.cs
- _LazyAsyncResult.cs
- SqlDelegatedTransaction.cs
- HtmlToClrEventProxy.cs
- designeractionlistschangedeventargs.cs
- CalendarAutoFormatDialog.cs
- XsltContext.cs
- MetadataCache.cs
- BooleanStorage.cs
- BoundPropertyEntry.cs
- HandlerWithFactory.cs
- MustUnderstandSoapException.cs
- ComponentFactoryHelpers.cs
- MessageSecurityOverMsmqElement.cs
- BoolExpr.cs
- ToolStripPanelDesigner.cs
- ButtonColumn.cs
- Pair.cs
- DataPointer.cs
- CqlParserHelpers.cs
- TableItemPattern.cs
- CommandConverter.cs
- And.cs
- CodeIdentifier.cs
- CryptoKeySecurity.cs
- DocumentPageViewAutomationPeer.cs
- WindowsIPAddress.cs