Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / GeneralTransformGroup.cs / 1 / GeneralTransformGroup.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: GeneralTransformGroup.cs //----------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Collections.Generic; using MS.Internal; using System.Windows.Media.Animation; using System.Globalization; using System.Text; using System.Runtime.InteropServices; using System.Windows.Markup; using System.Windows.Media.Composition; using System.Diagnostics; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// GeneralTrasnform group /// [ContentProperty("Children")] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public sealed partial class GeneralTransformGroup : GeneralTransform { #region Constructors ////// Default Constructor /// public GeneralTransformGroup() { } #endregion ////// Transform a point /// /// input point /// output point ///True if the point is transformed successfully public override bool TryTransform(Point inPoint, out Point result) { result = inPoint; if ((Children == null) || (Children.Count == 0)) { return false; } Point inP = inPoint; bool fPointTransformed = true; // transform the point through each of the transforms for (int i = 0; i < Children.Count; i++) { if (Children.Internal_GetItem(i).TryTransform(inPoint, out result) == false) { fPointTransformed = false; } inPoint = result; } return fPointTransformed; } ////// Transforms the bounding box to the smallest axis aligned bounding box /// that contains all the points in the original bounding box /// /// Input bounding rect ///Transformed bounding rect public override Rect TransformBounds(Rect rect) { if ((Children == null) || (Children.Count == 0)) { return rect; } Rect result = rect; for (int i = 0; i < Children.Count; i++) { result = Children.Internal_GetItem(i).TransformBounds(result); } return result; } ////// Returns the inverse transform if it has an inverse, null otherwise /// public override GeneralTransform Inverse { get { ReadPreamble(); if ((Children == null) || (Children.Count == 0)) { return null; } GeneralTransformGroup group = new GeneralTransformGroup(); for (int i = Children.Count - 1; i >= 0; i--) { GeneralTransform g = Children.Internal_GetItem(i).Inverse; // if any of the transforms does not have an inverse, // then the entire group does not have one if (g == null) return null; group.Children.Add(g); } return group; } } ////// Returns a best effort affine transform /// internal override Transform AffineTransform { [FriendAccessAllowed] // Built into Core, also used by Framework. get { if ((Children == null) || (Children.Count == 0)) { return null; } Matrix matrix = Matrix.Identity; foreach (GeneralTransform gt in Children) { Transform t = gt.AffineTransform; if (t != null) { matrix *= t.Value; } } return new MatrixTransform(matrix); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: GeneralTransformGroup.cs //----------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Collections.Generic; using MS.Internal; using System.Windows.Media.Animation; using System.Globalization; using System.Text; using System.Runtime.InteropServices; using System.Windows.Markup; using System.Windows.Media.Composition; using System.Diagnostics; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// GeneralTrasnform group /// [ContentProperty("Children")] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public sealed partial class GeneralTransformGroup : GeneralTransform { #region Constructors ////// Default Constructor /// public GeneralTransformGroup() { } #endregion ////// Transform a point /// /// input point /// output point ///True if the point is transformed successfully public override bool TryTransform(Point inPoint, out Point result) { result = inPoint; if ((Children == null) || (Children.Count == 0)) { return false; } Point inP = inPoint; bool fPointTransformed = true; // transform the point through each of the transforms for (int i = 0; i < Children.Count; i++) { if (Children.Internal_GetItem(i).TryTransform(inPoint, out result) == false) { fPointTransformed = false; } inPoint = result; } return fPointTransformed; } ////// Transforms the bounding box to the smallest axis aligned bounding box /// that contains all the points in the original bounding box /// /// Input bounding rect ///Transformed bounding rect public override Rect TransformBounds(Rect rect) { if ((Children == null) || (Children.Count == 0)) { return rect; } Rect result = rect; for (int i = 0; i < Children.Count; i++) { result = Children.Internal_GetItem(i).TransformBounds(result); } return result; } ////// Returns the inverse transform if it has an inverse, null otherwise /// public override GeneralTransform Inverse { get { ReadPreamble(); if ((Children == null) || (Children.Count == 0)) { return null; } GeneralTransformGroup group = new GeneralTransformGroup(); for (int i = Children.Count - 1; i >= 0; i--) { GeneralTransform g = Children.Internal_GetItem(i).Inverse; // if any of the transforms does not have an inverse, // then the entire group does not have one if (g == null) return null; group.Children.Add(g); } return group; } } ////// Returns a best effort affine transform /// internal override Transform AffineTransform { [FriendAccessAllowed] // Built into Core, also used by Framework. get { if ((Children == null) || (Children.Count == 0)) { return null; } Matrix matrix = Matrix.Identity; foreach (GeneralTransform gt in Children) { Transform t = gt.AffineTransform; if (t != null) { matrix *= t.Value; } } return new MatrixTransform(matrix); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
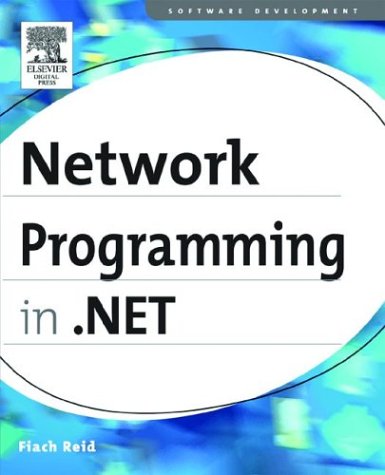
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnsafeNativeMethods.cs
- SystemEvents.cs
- ReadContentAsBinaryHelper.cs
- XmlCharType.cs
- RightsManagementPermission.cs
- EntityWrapperFactory.cs
- InputLanguageManager.cs
- BitmapEffectDrawingContent.cs
- DesignTimeParseData.cs
- DotExpr.cs
- SecurityAppliedMessage.cs
- FactoryMaker.cs
- WebPartsSection.cs
- UnmanagedMemoryStreamWrapper.cs
- MemoryFailPoint.cs
- InternalTypeHelper.cs
- PropertyMapper.cs
- XsdCachingReader.cs
- ToolStripPanelRenderEventArgs.cs
- AnnotationHelper.cs
- AutomationEvent.cs
- TrackingDataItem.cs
- WindowsEditBoxRange.cs
- RectAnimationClockResource.cs
- ApplicationCommands.cs
- GridViewRowPresenter.cs
- SqlProviderServices.cs
- PersistenceTypeAttribute.cs
- SerializationSectionGroup.cs
- KoreanLunisolarCalendar.cs
- AuthorizationRule.cs
- SatelliteContractVersionAttribute.cs
- ConfigurationStrings.cs
- _Win32.cs
- StorageScalarPropertyMapping.cs
- indexingfiltermarshaler.cs
- DBCommand.cs
- XmlNodeList.cs
- log.cs
- DecoderExceptionFallback.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- Semaphore.cs
- SqlCommandSet.cs
- Suspend.cs
- FormViewModeEventArgs.cs
- AudioLevelUpdatedEventArgs.cs
- XmlRootAttribute.cs
- SqlBooleanizer.cs
- RoleGroupCollection.cs
- ObfuscationAttribute.cs
- IteratorDescriptor.cs
- ConfigurationStrings.cs
- WebPartZoneCollection.cs
- XD.cs
- CellParaClient.cs
- ArcSegment.cs
- PeerNameRecordCollection.cs
- SpStreamWrapper.cs
- OutOfMemoryException.cs
- XmlSchemaInfo.cs
- SizeF.cs
- Translator.cs
- StreamInfo.cs
- DeleteHelper.cs
- DesignerDataTable.cs
- DataFormats.cs
- WebPartEventArgs.cs
- SiteMap.cs
- InvalidTimeZoneException.cs
- AndMessageFilter.cs
- OleAutBinder.cs
- PeerNameRegistration.cs
- ConnectionPoint.cs
- UTF7Encoding.cs
- DeriveBytes.cs
- TraceUtils.cs
- ContentPropertyAttribute.cs
- MetadataCollection.cs
- BasicHttpMessageSecurityElement.cs
- DocumentApplication.cs
- Point3DAnimation.cs
- EditorPartChrome.cs
- _NegoState.cs
- TextEditorTables.cs
- SectionInput.cs
- LicenseProviderAttribute.cs
- UndoEngine.cs
- MethodBody.cs
- Misc.cs
- SmiEventStream.cs
- MouseActionConverter.cs
- BasicViewGenerator.cs
- SecurityToken.cs
- NewExpression.cs
- EventLogPermissionEntry.cs
- ValidationResult.cs
- RowBinding.cs
- ColorConvertedBitmapExtension.cs
- RTLAwareMessageBox.cs
- DataGridAutomationPeer.cs