Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / TextSegment.cs / 1 / TextSegment.cs
//---------------------------------------------------------------------------- // // File: TextSegment.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: A pair of TextPositions used to denote a run of TextContainer content. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; using System.Collections; ////// A pair of TextPositions used to denote a run of TextContainer content. /// // internal struct TextSegment { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor. /// /// /// Position preceeding the TextSegment's content. /// /// /// Position following the TextSegment's content. /// ////// If startPosition or endPosition are TextNavigators (derived from /// TextPointer), the TextSegment constructor will store new TextPointer /// instances internally. The values returned by the Start and End /// properties are always immutable TextPositions. /// internal TextSegment(ITextPointer startPosition, ITextPointer endPosition) : this(startPosition, endPosition, false) { } ////// Constructor. /// /// /// Position preceeding the TextSegment's content. /// /// /// Position following the TextSegment's content. /// /// /// Whether preserves LogicalDirection of start and end positions. /// internal TextSegment(ITextPointer startPosition, ITextPointer endPosition, bool preserveLogicalDirection) { ValidationHelper.VerifyPositionPair(startPosition, endPosition); if (startPosition.CompareTo(endPosition) == 0) { // To preserve segment emptiness // we use the same instance of a pointer // for both segment ends. _start = startPosition.GetFrozenPointer(startPosition.LogicalDirection); _end = _start; } else { Invariant.Assert(startPosition.CompareTo(endPosition) < 0); _start = startPosition.GetFrozenPointer(preserveLogicalDirection ? startPosition.LogicalDirection : LogicalDirection.Backward); _end = endPosition.GetFrozenPointer(preserveLogicalDirection ? endPosition.LogicalDirection : LogicalDirection.Forward); } } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// returns true if the segment contains a given position /// // internal bool Contains(ITextPointer position) { return (!this.IsNull && this._start.CompareTo(position) <= 0 && position.CompareTo(this._end) <= 0); } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Position preceeding the TextSegment's content. /// internal ITextPointer Start { get { return _start; } } ////// Position following the TextSegment's content. /// internal ITextPointer End { get { return _end; } } internal bool IsNull { get { return _start == null || _end == null; } } #endregion Internal Properties ////// The "TextSegment.Null" value. /// ////// TextSegtemt.Null is used in contexts where text segment is missing. /// internal static readonly TextSegment Null = new TextSegment(); //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // Position preceeding the TextSegment's content. private readonly ITextPointer _start; // Position following the TextSegment's content. private readonly ITextPointer _end; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: TextSegment.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: A pair of TextPositions used to denote a run of TextContainer content. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; using System.Collections; ////// A pair of TextPositions used to denote a run of TextContainer content. /// // internal struct TextSegment { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor. /// /// /// Position preceeding the TextSegment's content. /// /// /// Position following the TextSegment's content. /// ////// If startPosition or endPosition are TextNavigators (derived from /// TextPointer), the TextSegment constructor will store new TextPointer /// instances internally. The values returned by the Start and End /// properties are always immutable TextPositions. /// internal TextSegment(ITextPointer startPosition, ITextPointer endPosition) : this(startPosition, endPosition, false) { } ////// Constructor. /// /// /// Position preceeding the TextSegment's content. /// /// /// Position following the TextSegment's content. /// /// /// Whether preserves LogicalDirection of start and end positions. /// internal TextSegment(ITextPointer startPosition, ITextPointer endPosition, bool preserveLogicalDirection) { ValidationHelper.VerifyPositionPair(startPosition, endPosition); if (startPosition.CompareTo(endPosition) == 0) { // To preserve segment emptiness // we use the same instance of a pointer // for both segment ends. _start = startPosition.GetFrozenPointer(startPosition.LogicalDirection); _end = _start; } else { Invariant.Assert(startPosition.CompareTo(endPosition) < 0); _start = startPosition.GetFrozenPointer(preserveLogicalDirection ? startPosition.LogicalDirection : LogicalDirection.Backward); _end = endPosition.GetFrozenPointer(preserveLogicalDirection ? endPosition.LogicalDirection : LogicalDirection.Forward); } } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// returns true if the segment contains a given position /// // internal bool Contains(ITextPointer position) { return (!this.IsNull && this._start.CompareTo(position) <= 0 && position.CompareTo(this._end) <= 0); } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Position preceeding the TextSegment's content. /// internal ITextPointer Start { get { return _start; } } ////// Position following the TextSegment's content. /// internal ITextPointer End { get { return _end; } } internal bool IsNull { get { return _start == null || _end == null; } } #endregion Internal Properties ////// The "TextSegment.Null" value. /// ////// TextSegtemt.Null is used in contexts where text segment is missing. /// internal static readonly TextSegment Null = new TextSegment(); //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // Position preceeding the TextSegment's content. private readonly ITextPointer _start; // Position following the TextSegment's content. private readonly ITextPointer _end; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
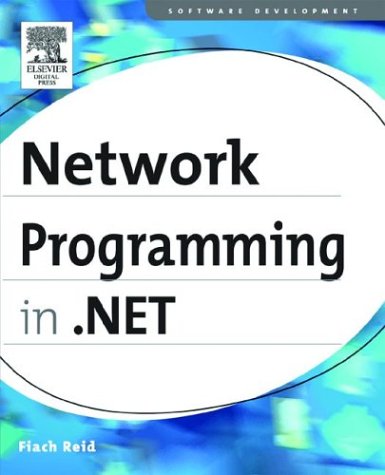
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConstructorBuilder.cs
- XmlCharCheckingReader.cs
- Attributes.cs
- FixedSOMTextRun.cs
- LeftCellWrapper.cs
- AuthenticationModuleElementCollection.cs
- EntityCommandCompilationException.cs
- Exceptions.cs
- ConfigurationStrings.cs
- diagnosticsswitches.cs
- MimeParameter.cs
- DockPattern.cs
- PersianCalendar.cs
- Message.cs
- HttpDictionary.cs
- SendKeys.cs
- CTreeGenerator.cs
- DeclaredTypeValidatorAttribute.cs
- EmptyQuery.cs
- DateTimeFormatInfoScanner.cs
- SiteIdentityPermission.cs
- FileDialog_Vista.cs
- SqlDataSourceTableQuery.cs
- Vector3DAnimationBase.cs
- ReadOnlyPermissionSet.cs
- TemplateLookupAction.cs
- TextEditorContextMenu.cs
- RawStylusInputReport.cs
- AssemblyBuilder.cs
- BitVector32.cs
- ContentTextAutomationPeer.cs
- RadioButton.cs
- BindUriHelper.cs
- BinaryObjectInfo.cs
- ObjectStateEntry.cs
- RenderCapability.cs
- PropertyManager.cs
- DefaultEventAttribute.cs
- BaseResourcesBuildProvider.cs
- DesignerOptionService.cs
- AuditLog.cs
- MimeAnyImporter.cs
- PrimaryKeyTypeConverter.cs
- MarshalDirectiveException.cs
- AnnotationResourceCollection.cs
- ApplicationSecurityInfo.cs
- StringAttributeCollection.cs
- LazyTextWriterCreator.cs
- PointAnimationUsingKeyFrames.cs
- BmpBitmapDecoder.cs
- DelegatingTypeDescriptionProvider.cs
- BamlReader.cs
- LogRestartAreaEnumerator.cs
- BaseUriHelper.cs
- DropShadowBitmapEffect.cs
- SafeCertificateStore.cs
- WS2007FederationHttpBindingCollectionElement.cs
- Properties.cs
- CompilerCollection.cs
- AffineTransform3D.cs
- HttpDictionary.cs
- Triangle.cs
- StateDesigner.LayoutSelectionGlyph.cs
- MenuRendererStandards.cs
- IImplicitResourceProvider.cs
- AuthStoreRoleProvider.cs
- InternalDuplexChannelListener.cs
- UnauthorizedAccessException.cs
- PackageDigitalSignatureManager.cs
- MenuItemAutomationPeer.cs
- HttpStreamMessage.cs
- Exception.cs
- Border.cs
- ManualWorkflowSchedulerService.cs
- input.cs
- RegionIterator.cs
- ReceiveDesigner.xaml.cs
- LocatorPartList.cs
- RequestCacheEntry.cs
- Setter.cs
- FontFamilyValueSerializer.cs
- DesignerSerializationOptionsAttribute.cs
- TransformerConfigurationWizardBase.cs
- WebEvents.cs
- ValidatingPropertiesEventArgs.cs
- IndividualDeviceConfig.cs
- ForeignKeyConstraint.cs
- BoolExpression.cs
- DetailsViewPagerRow.cs
- DispatcherExceptionFilterEventArgs.cs
- AuthenticationService.cs
- RequiredAttributeAttribute.cs
- MenuItemBindingCollection.cs
- Native.cs
- SettingsSection.cs
- X509CertificateValidationMode.cs
- CodeChecksumPragma.cs
- SerialReceived.cs
- DataBindingCollectionEditor.cs
- DocumentReference.cs