Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / DataOracleClient / System / Data / Common / System.Data.OracleClient_BID.cs / 1 / System.Data.OracleClient_BID.cs
//------------------------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Reflection; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Text; [module: BidIdentity("System.Data.OracleClient.1")] [module: BidMetaText(":FormatControl: InstanceID='' ")] [module: BidMetaText("Trace=600; Scope=30;")] [module: BidMetaText(" ora = System.Data.OracleClient;")] internal static partial class Bid { private const string dllName = "System.Data.OracleClient.dll"; // // Manually added wrappers // [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2); } internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3); } internal static void PoolerScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1); } else { hScp = NoData; } } // // ScopeEnter overloads // internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2); } else { hScp = NoData; } } // // Trace overloads // internal static void Trace(string fmtPrintfW, System.UInt32 a1, System.Int32 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2 ); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2 ); } internal static void Trace(string fmtPrintfW, System.String a1, System.Int32 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2 ); } #if DEBUG internal static void Trace(string fmtPrintfW, System.String a1, System.String a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } #endif internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2 ); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.Int32 a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3 ); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3 ); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.UInt32 a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3, System.IntPtr a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.Int32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.UInt32 a4, System.UInt32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5 ); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3, System.String a4, System.Int32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.Int32 a2, System.IntPtr a3, System.IntPtr a4, System.Int32 a5, System.Int32 a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4, System.UInt32 a5, System.UInt32 a6, System.UInt32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.String a4, System.Int32 a5, System.String a6, System.Int32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.Int32 a5, System.Int32 a6, System.Int32 a7, System.Int32 a8) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7,a8 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3, System.IntPtr a4, System.IntPtr a5, System.IntPtr a6, System.IntPtr a7, System.IntPtr a8) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7,a8 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.UInt32 a5, System.IntPtr a6, System.Int32 a7, System.IntPtr a8, System.IntPtr a9, System.Int32 a10, System.Int32 a11) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.UInt32 a5, System.IntPtr a6, System.Int32 a7, System.Int32 a8, System.IntPtr a9, System.IntPtr a10, System.Int32 a11, System.Int32 a12) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.String a3, System.Int32 a4, System.IntPtr a5, System.Int32 a6, System.Int32 a7, System.IntPtr a8, System.Int32 a9, System.IntPtr a10, System.Int32 a11, System.IntPtr a12, System.UInt32 a13, System.IntPtr a14, System.Int32 a15) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15 ); } // // Manually edited Trace overloads // [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.Int32 a1) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.IntPtr a1) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.Int32 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2 ); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3 ); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Int32 a2, System.Int32 a3, System.IntPtr a4, System.IntPtr a5, System.Int32 a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), a2, a3, a4, a5, a6); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4, System.IntPtr a5, System.Int32 a6, System.IntPtr a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7 ); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4, System.IntPtr a5, System.Int32 a6, System.IntPtr a7, System.Int32 a8, System.Int32 a9) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7,a8,a9 ); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.Data.OracleClient.OCI.ATTR a3, System.Data.OracleClient.OciHandle a4, System.String a5, System.UInt32 a6, System.Int32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1),a2.ToString(),System.Data.OracleClient.OciHandle.GetAttributeName(a1,a3), System.Data.OracleClient.OciHandle.HandleValueToTrace(a4), a5, a6, a7); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.Data.OracleClient.OCI.ATTR a3, System.Data.OracleClient.OciHandle a4, System.IntPtr a5, System.UInt32 a6, System.Int32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1),a2.ToString(),System.Data.OracleClient.OciHandle.GetAttributeName(a1,a3), System.Data.OracleClient.OciHandle.HandleValueToTrace(a4), a5, a6, a7); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.Data.OracleClient.OCI.ATTR a3, System.Data.OracleClient.OciHandle a4, System.Int32 a5, System.UInt32 a6, System.Int32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1),a2.ToString(),System.Data.OracleClient.OciHandle.GetAttributeName(a1,a3), System.Data.OracleClient.OciHandle.HandleValueToTrace(a4), a5, a6, a7); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.Data.OracleClient.OciHandle a3, System.Int32 a4, System.IntPtr a5, System.Int32 a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1),a2.ToString(),System.Data.OracleClient.OciHandle.HandleValueToTrace(a3), a4, a5, a6); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.Int32 a3, System.UInt32 a4, System.Data.OracleClient.OCI.ATTR a5, System.Data.OracleClient.OciHandle a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), a2.ToString(), a3, a4, System.Data.OracleClient.OciHandle.GetAttributeName(a1,a5), System.Data.OracleClient.OciHandle.HandleValueToTrace(a6)); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.Data.OracleClient.OciHandle a3, System.UInt32 a4, System.Data.OracleClient.OCI.ATTR a5, System.Data.OracleClient.OciHandle a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), a2.ToString(), System.Data.OracleClient.OciHandle.HandleValueToTrace(a3), a4, System.Data.OracleClient.OciHandle.GetAttributeName(a1,a5), System.Data.OracleClient.OciHandle.HandleValueToTrace(a6)); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.UInt32 a3, System.IntPtr a4, System.Int32 a5, System.Int32 a6, System.Data.OracleClient.OCI.DATATYPE a7, System.IntPtr a8, System.IntPtr a9, System.IntPtr a10, System.Int32 a11, System.IntPtr a12, System.Int32 a13) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), a3, a4, a5, a6, a7.ToString(), a8, a9, a10, a11, a12, a13); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.UInt32 a3, System.UInt32 a4, System.UInt32 a5, System.UInt32 a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), a3, a4, a5, a6); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.String a3, System.UInt32 a4, System.Data.OracleClient.OCI.ATTR a5, System.Data.OracleClient.OciHandle a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), a2.ToString(), a3, a4, System.Data.OracleClient.OciHandle.GetAttributeName(a1,a5), System.Data.OracleClient.OciHandle.HandleValueToTrace(a6)); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.String a3, System.Int32 a4, System.Int32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), a3, a4, a5); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.Data.OracleClient.OciHandle a3, System.Data.OracleClient.OCI.CRED a4, System.Int32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), System.Data.OracleClient.OciHandle.HandleValueToTrace(a3), a4.ToString(), a5); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.Data.OracleClient.OciHandle a3, System.Int32 a4, System.Int32 a5, System.IntPtr a6, System.IntPtr a7, System.Int32 a8 ) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), System.Data.OracleClient.OciHandle.HandleValueToTrace(a3), a4, a5, a6, a7, a8); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.Int32 a3, System.Int32 a4, System.Int32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), a3,a4,a5); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.Int32 a3, System.Int32 a4, System.Int32 a5, System.String a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), a3,a4,a5,a6); } // // Interop calls to pluggable hooks [SuppressUnmanagedCodeSecurity] applied // private static partial class NativeMethods { // // ScopeEnter // [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2); // // Trace // [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.UInt32 a1, System.Int32 a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.String a1, System.Int32 a2); #if DEBUG [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.String a1, System.String a2); #endif [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.UInt32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3, System.IntPtr a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.Int32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.UInt32 a4, System.UInt32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3, System.String a4, System.Int32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.String a3, System.Int32 a4, System.Int32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3, System.Int32 a4, System.Int32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.UInt32 a3, System.UInt32 a4, System.UInt32 a5, System.UInt32 a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.Int32 a2, System.IntPtr a3, System.IntPtr a4, System.Int32 a5, System.Int32 a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4, System.UInt32 a5, System.UInt32 a6, System.UInt32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.String a4, System.Int32 a5, System.String a6, System.Int32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.Int32 a5, System.Int32 a6, System.Int32 a7, System.Int32 a8); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.Int32 a5, System.IntPtr a6, System.IntPtr a7, System.Int32 a8); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3, System.IntPtr a4, System.IntPtr a5, System.IntPtr a6, System.IntPtr a7, System.IntPtr a8); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.UInt32 a5, System.IntPtr a6, System.Int32 a7, System.IntPtr a8, System.IntPtr a9, System.Int32 a10, System.Int32 a11); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.UInt32 a5, System.IntPtr a6, System.Int32 a7, System.Int32 a8, System.IntPtr a9, System.IntPtr a10, System.Int32 a11, System.Int32 a12); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.String a3, System.Int32 a4, System.IntPtr a5, System.Int32 a6, System.Int32 a7, System.IntPtr a8, System.Int32 a9, System.IntPtr a10, System.Int32 a11, System.IntPtr a12, System.UInt32 a13, System.IntPtr a14 , System.Int32 a15); // // Interop for manually edited Trace overloads // [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.Int32 a2); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4, System.IntPtr a5, System.Int32 a6, System.IntPtr a7); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.Int32 a2, System.Int32 a3, System.IntPtr a4, System.IntPtr a5, System.Int32 a6); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4, System.IntPtr a5, System.Int32 a6, System.IntPtr a7, System.Int32 a8, System.Int32 a9); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.String a3, System.IntPtr a4, System.String a5, System.UInt32 a6, System.Int32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.String a3, System.IntPtr a4, System.IntPtr a5, System.UInt32 a6, System.Int32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.String a3, System.IntPtr a4, System.Int32 a5, System.UInt32 a6, System.Int32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.IntPtr a3, System.Int32 a4, System.IntPtr a5, System.Int32 a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.Int32 a3, System.UInt32 a4, System.String a5, System.IntPtr a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.IntPtr a3, System.UInt32 a4, System.String a5, System.IntPtr a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.UInt32 a3, System.IntPtr a4, System.Int32 a5, System.Int32 a6, System.String a7, System.IntPtr a8, System.IntPtr a9, System.IntPtr a10, System.Int32 a11, System.IntPtr a12, System.Int32 a13); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.String a3, System.UInt32 a4, System.String a5, System.IntPtr a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.String a4, System.Int32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1,System.IntPtr a2,System.Int32 a3,System.Int32 a4,System.Int32 a5,System.String a6); } // NativeMethods //=////////////////////////////////////////////////////////////////////////////////////////////// /* Do not remove! very useful to create new overloads internal static void Trace(string fmtPrintfW, ) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, ); } internal static void TraceEx(uint flags, string fmtPrintfW, ) { if (modID != NoData) NativeMethods.Trace(modID, UIntPtr.Zero, (UIntPtr)flags, fmtPrintfW, ); } [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, ); System.IntPtr a1, System.IntPtr a2, System.Int32 a3, System.IntPtr a4, System.Int32 a5, System.Int32 a6, System.IntPtr a7, System.IntPtr a8, System.IntPtr a9, System.Int32 a10, System.Int32 a11, System.IntPtr a12, System.Int32 a13, System.IntPtr a14, System.Int32 a15, a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15); */ } // Bid // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Reflection; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Text; [module: BidIdentity("System.Data.OracleClient.1")] [module: BidMetaText(":FormatControl: InstanceID='' ")] [module: BidMetaText("Trace=600; Scope=30;")] [module: BidMetaText(" ora = System.Data.OracleClient;")] internal static partial class Bid { private const string dllName = "System.Data.OracleClient.dll"; // // Manually added wrappers // [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2); } internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3); } internal static void PoolerScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1); } else { hScp = NoData; } } // // ScopeEnter overloads // internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2); } else { hScp = NoData; } } // // Trace overloads // internal static void Trace(string fmtPrintfW, System.UInt32 a1, System.Int32 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2 ); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2 ); } internal static void Trace(string fmtPrintfW, System.String a1, System.Int32 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2 ); } #if DEBUG internal static void Trace(string fmtPrintfW, System.String a1, System.String a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } #endif internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2 ); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.Int32 a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3 ); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3 ); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.UInt32 a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3, System.IntPtr a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.Int32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.UInt32 a4, System.UInt32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5 ); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3, System.String a4, System.Int32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.Int32 a2, System.IntPtr a3, System.IntPtr a4, System.Int32 a5, System.Int32 a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4, System.UInt32 a5, System.UInt32 a6, System.UInt32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.String a4, System.Int32 a5, System.String a6, System.Int32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.Int32 a5, System.Int32 a6, System.Int32 a7, System.Int32 a8) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7,a8 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3, System.IntPtr a4, System.IntPtr a5, System.IntPtr a6, System.IntPtr a7, System.IntPtr a8) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7,a8 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.UInt32 a5, System.IntPtr a6, System.Int32 a7, System.IntPtr a8, System.IntPtr a9, System.Int32 a10, System.Int32 a11) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.UInt32 a5, System.IntPtr a6, System.Int32 a7, System.Int32 a8, System.IntPtr a9, System.IntPtr a10, System.Int32 a11, System.Int32 a12) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12 ); } internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.String a3, System.Int32 a4, System.IntPtr a5, System.Int32 a6, System.Int32 a7, System.IntPtr a8, System.Int32 a9, System.IntPtr a10, System.Int32 a11, System.IntPtr a12, System.UInt32 a13, System.IntPtr a14, System.Int32 a15) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15 ); } // // Manually edited Trace overloads // [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.Int32 a1) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.IntPtr a1) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.Int32 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2 ); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3 ); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Int32 a2, System.Int32 a3, System.IntPtr a4, System.IntPtr a5, System.Int32 a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), a2, a3, a4, a5, a6); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4, System.IntPtr a5, System.Int32 a6, System.IntPtr a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7 ); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4, System.IntPtr a5, System.Int32 a6, System.IntPtr a7, System.Int32 a8, System.Int32 a9) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7,a8,a9 ); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.Data.OracleClient.OCI.ATTR a3, System.Data.OracleClient.OciHandle a4, System.String a5, System.UInt32 a6, System.Int32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1),a2.ToString(),System.Data.OracleClient.OciHandle.GetAttributeName(a1,a3), System.Data.OracleClient.OciHandle.HandleValueToTrace(a4), a5, a6, a7); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.Data.OracleClient.OCI.ATTR a3, System.Data.OracleClient.OciHandle a4, System.IntPtr a5, System.UInt32 a6, System.Int32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1),a2.ToString(),System.Data.OracleClient.OciHandle.GetAttributeName(a1,a3), System.Data.OracleClient.OciHandle.HandleValueToTrace(a4), a5, a6, a7); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.Data.OracleClient.OCI.ATTR a3, System.Data.OracleClient.OciHandle a4, System.Int32 a5, System.UInt32 a6, System.Int32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1),a2.ToString(),System.Data.OracleClient.OciHandle.GetAttributeName(a1,a3), System.Data.OracleClient.OciHandle.HandleValueToTrace(a4), a5, a6, a7); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.Data.OracleClient.OciHandle a3, System.Int32 a4, System.IntPtr a5, System.Int32 a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1),a2.ToString(),System.Data.OracleClient.OciHandle.HandleValueToTrace(a3), a4, a5, a6); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.Int32 a3, System.UInt32 a4, System.Data.OracleClient.OCI.ATTR a5, System.Data.OracleClient.OciHandle a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), a2.ToString(), a3, a4, System.Data.OracleClient.OciHandle.GetAttributeName(a1,a5), System.Data.OracleClient.OciHandle.HandleValueToTrace(a6)); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.Data.OracleClient.OciHandle a3, System.UInt32 a4, System.Data.OracleClient.OCI.ATTR a5, System.Data.OracleClient.OciHandle a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), a2.ToString(), System.Data.OracleClient.OciHandle.HandleValueToTrace(a3), a4, System.Data.OracleClient.OciHandle.GetAttributeName(a1,a5), System.Data.OracleClient.OciHandle.HandleValueToTrace(a6)); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.UInt32 a3, System.IntPtr a4, System.Int32 a5, System.Int32 a6, System.Data.OracleClient.OCI.DATATYPE a7, System.IntPtr a8, System.IntPtr a9, System.IntPtr a10, System.Int32 a11, System.IntPtr a12, System.Int32 a13) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), a3, a4, a5, a6, a7.ToString(), a8, a9, a10, a11, a12, a13); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.UInt32 a3, System.UInt32 a4, System.UInt32 a5, System.UInt32 a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), a3, a4, a5, a6); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OCI.HTYPE a2, System.String a3, System.UInt32 a4, System.Data.OracleClient.OCI.ATTR a5, System.Data.OracleClient.OciHandle a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), a2.ToString(), a3, a4, System.Data.OracleClient.OciHandle.GetAttributeName(a1,a5), System.Data.OracleClient.OciHandle.HandleValueToTrace(a6)); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.String a3, System.Int32 a4, System.Int32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), a3, a4, a5); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.Data.OracleClient.OciHandle a3, System.Data.OracleClient.OCI.CRED a4, System.Int32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), System.Data.OracleClient.OciHandle.HandleValueToTrace(a3), a4.ToString(), a5); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.Data.OracleClient.OciHandle a3, System.Int32 a4, System.Int32 a5, System.IntPtr a6, System.IntPtr a7, System.Int32 a8 ) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), System.Data.OracleClient.OciHandle.HandleValueToTrace(a3), a4, a5, a6, a7, a8); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.Int32 a3, System.Int32 a4, System.Int32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), a3,a4,a5); } internal static void Trace(string fmtPrintfW, System.Data.OracleClient.OciHandle a1, System.Data.OracleClient.OciHandle a2, System.Int32 a3, System.Int32 a4, System.Int32 a5, System.String a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,System.Data.OracleClient.OciHandle.HandleValueToTrace(a1), System.Data.OracleClient.OciHandle.HandleValueToTrace(a2), a3,a4,a5,a6); } // // Interop calls to pluggable hooks [SuppressUnmanagedCodeSecurity] applied // private static partial class NativeMethods { // // ScopeEnter // [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2); // // Trace // [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.UInt32 a1, System.Int32 a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.String a1, System.Int32 a2); #if DEBUG [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.String a1, System.String a2); #endif [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.UInt32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3, System.IntPtr a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.Int32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.UInt32 a4, System.UInt32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3, System.String a4, System.Int32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.String a3, System.Int32 a4, System.Int32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3, System.Int32 a4, System.Int32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.UInt32 a3, System.UInt32 a4, System.UInt32 a5, System.UInt32 a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.Int32 a2, System.IntPtr a3, System.IntPtr a4, System.Int32 a5, System.Int32 a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4, System.UInt32 a5, System.UInt32 a6, System.UInt32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.String a4, System.Int32 a5, System.String a6, System.Int32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.Int32 a5, System.Int32 a6, System.Int32 a7, System.Int32 a8); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.Int32 a5, System.IntPtr a6, System.IntPtr a7, System.Int32 a8); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3, System.IntPtr a4, System.IntPtr a5, System.IntPtr a6, System.IntPtr a7, System.IntPtr a8); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.UInt32 a5, System.IntPtr a6, System.Int32 a7, System.IntPtr a8, System.IntPtr a9, System.Int32 a10, System.Int32 a11); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.Int32 a4, System.UInt32 a5, System.IntPtr a6, System.Int32 a7, System.Int32 a8, System.IntPtr a9, System.IntPtr a10, System.Int32 a11, System.Int32 a12); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.String a3, System.Int32 a4, System.IntPtr a5, System.Int32 a6, System.Int32 a7, System.IntPtr a8, System.Int32 a9, System.IntPtr a10, System.Int32 a11, System.IntPtr a12, System.UInt32 a13, System.IntPtr a14 , System.Int32 a15); // // Interop for manually edited Trace overloads // [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.Int32 a2); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.Int32 a3); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4, System.IntPtr a5, System.Int32 a6, System.IntPtr a7); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.Int32 a2, System.Int32 a3, System.IntPtr a4, System.IntPtr a5, System.Int32 a6); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.IntPtr a3, System.IntPtr a4, System.IntPtr a5, System.Int32 a6, System.IntPtr a7, System.Int32 a8, System.Int32 a9); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.String a3, System.IntPtr a4, System.String a5, System.UInt32 a6, System.Int32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.String a3, System.IntPtr a4, System.IntPtr a5, System.UInt32 a6, System.Int32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.String a3, System.IntPtr a4, System.Int32 a5, System.UInt32 a6, System.Int32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.IntPtr a3, System.Int32 a4, System.IntPtr a5, System.Int32 a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.Int32 a3, System.UInt32 a4, System.String a5, System.IntPtr a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.IntPtr a3, System.UInt32 a4, System.String a5, System.IntPtr a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.UInt32 a3, System.IntPtr a4, System.Int32 a5, System.Int32 a6, System.String a7, System.IntPtr a8, System.IntPtr a9, System.IntPtr a10, System.Int32 a11, System.IntPtr a12, System.Int32 a13); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.String a2, System.String a3, System.UInt32 a4, System.String a5, System.IntPtr a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1, System.IntPtr a2, System.IntPtr a3, System.String a4, System.Int32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1,System.IntPtr a2,System.Int32 a3,System.Int32 a4,System.Int32 a5,System.String a6); } // NativeMethods //=////////////////////////////////////////////////////////////////////////////////////////////// /* Do not remove! very useful to create new overloads internal static void Trace(string fmtPrintfW, ) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, ); } internal static void TraceEx(uint flags, string fmtPrintfW, ) { if (modID != NoData) NativeMethods.Trace(modID, UIntPtr.Zero, (UIntPtr)flags, fmtPrintfW, ); } [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, ); System.IntPtr a1, System.IntPtr a2, System.Int32 a3, System.IntPtr a4, System.Int32 a5, System.Int32 a6, System.IntPtr a7, System.IntPtr a8, System.IntPtr a9, System.Int32 a10, System.Int32 a11, System.IntPtr a12, System.Int32 a13, System.IntPtr a14, System.Int32 a15, a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15); */ } // Bid // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
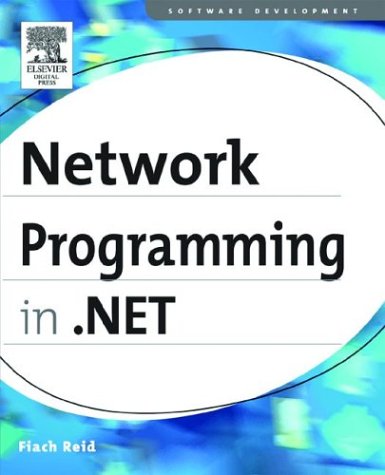
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GraphicsContext.cs
- TargetControlTypeCache.cs
- HttpWriter.cs
- CodeDomConfigurationHandler.cs
- CLSCompliantAttribute.cs
- SqlTopReducer.cs
- TextRangeProviderWrapper.cs
- DataSourceBooleanViewSchemaConverter.cs
- DelegatingStream.cs
- FileInfo.cs
- AnchoredBlock.cs
- UIElement3D.cs
- ContextProperty.cs
- Buffer.cs
- PresentationAppDomainManager.cs
- GlobalizationSection.cs
- login.cs
- PrimitiveXmlSerializers.cs
- EventItfInfo.cs
- CompositeScriptReference.cs
- XmlEncoding.cs
- LoginCancelEventArgs.cs
- OLEDB_Enum.cs
- RootContext.cs
- ConfigurationConverterBase.cs
- LocalServiceSecuritySettings.cs
- WmlTextBoxAdapter.cs
- AxisAngleRotation3D.cs
- StringPropertyBuilder.cs
- StringFreezingAttribute.cs
- AssertSection.cs
- StyleXamlParser.cs
- OracleTransaction.cs
- MediaCommands.cs
- DataServiceQuery.cs
- MessageHeaderDescription.cs
- ScriptDescriptor.cs
- DataGridItemCollection.cs
- SplineKeyFrames.cs
- DrawingDrawingContext.cs
- RectangleGeometry.cs
- TreeNodeEventArgs.cs
- UnsafeNativeMethods.cs
- XmlILIndex.cs
- ISAPIWorkerRequest.cs
- DataError.cs
- IProducerConsumerCollection.cs
- ConfigurationSectionGroupCollection.cs
- CustomCategoryAttribute.cs
- XmlCharCheckingReader.cs
- SimpleExpression.cs
- IIS7WorkerRequest.cs
- WebPartEditorOkVerb.cs
- XPathNodeInfoAtom.cs
- HttpDictionary.cs
- WebPartEditVerb.cs
- NullRuntimeConfig.cs
- AccessorTable.cs
- ReadOnlyDataSourceView.cs
- ListItemCollection.cs
- TypedColumnHandler.cs
- HandlerFactoryCache.cs
- XamlTypeWithExplicitNamespace.cs
- PassportAuthenticationModule.cs
- HelpEvent.cs
- MenuItem.cs
- HashStream.cs
- TypeElementCollection.cs
- RuntimeConfigurationRecord.cs
- PagePropertiesChangingEventArgs.cs
- DocumentAutomationPeer.cs
- ConditionalAttribute.cs
- BinHexDecoder.cs
- ResourceManager.cs
- ImageListImageEditor.cs
- RegexCode.cs
- RangeBase.cs
- WebBrowserUriTypeConverter.cs
- OutputCacheSettings.cs
- Control.cs
- SqlDependency.cs
- ActivatedMessageQueue.cs
- ValidationEventArgs.cs
- TraceHandlerErrorFormatter.cs
- FtpWebRequest.cs
- FontNameConverter.cs
- BitmapEffectCollection.cs
- XsltOutput.cs
- SiteMapNodeItem.cs
- WebPartUserCapability.cs
- MediaEntryAttribute.cs
- ImageBrush.cs
- ProviderConnectionPointCollection.cs
- XmlDataSource.cs
- UnknownWrapper.cs
- WebBrowser.cs
- ListViewHitTestInfo.cs
- WrappedIUnknown.cs
- nulltextcontainer.cs
- UserControlParser.cs