Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / SortAction.cs / 1 / SortAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Globalization; using System.Xml; using System.Xml.XPath; internal class SortAction : CompiledAction { private int selectKey = Compiler.InvalidQueryKey; private Avt langAvt; private Avt dataTypeAvt; private Avt orderAvt; private Avt caseOrderAvt; // Compile time precalculated AVTs private string lang; private XmlDataType dataType = XmlDataType.Text; private XmlSortOrder order = XmlSortOrder.Ascending; private XmlCaseOrder caseOrder = XmlCaseOrder.None; private Sort sort; //When we not have AVTs at all we can do this. null otherwise. private bool forwardCompatibility; private InputScopeManager manager; private string ParseLang(string value) { if(value == null) { // Avt is not constant, or attribute wasn't defined return null; } // XmlComplianceUtil.IsValidLanguageID uses the outdated RFC 1766. It would be // better to remove this method completely and not call it here, but that may // change exception types for some stylesheets. if (! XmlComplianceUtil.IsValidLanguageID(value.ToCharArray(), 0, value.Length) && (value.Length == 0 || CultureInfo.GetCultureInfo(value) == null) ) { if (this.forwardCompatibility) { return null; } throw XsltException.Create(Res.Xslt_InvalidAttrValue, Keywords.s_Lang, value); } return value; } private XmlDataType ParseDataType(string value, InputScopeManager manager) { if(value == null) { // Avt is not constant, or attribute wasn't defined return XmlDataType.Text; } if (value == Keywords.s_Text) { return XmlDataType.Text; } if (value == Keywords.s_Number) { return XmlDataType.Number; } String prefix, localname; PrefixQName.ParseQualifiedName(value, out prefix, out localname); manager.ResolveXmlNamespace(prefix); if (prefix.Length == 0 && ! this.forwardCompatibility) { throw XsltException.Create(Res.Xslt_InvalidAttrValue, Keywords.s_DataType, value); } return XmlDataType.Text; } private XmlSortOrder ParseOrder(string value) { if(value == null) { // Avt is not constant, or attribute wasn't defined return XmlSortOrder.Ascending; } if (value == Keywords.s_Ascending) { return XmlSortOrder.Ascending; } if (value == Keywords.s_Descending) { return XmlSortOrder.Descending; } if (this.forwardCompatibility) { return XmlSortOrder.Ascending; } throw XsltException.Create(Res.Xslt_InvalidAttrValue, Keywords.s_Order, value); } private XmlCaseOrder ParseCaseOrder(string value) { if(value == null) { // Avt is not constant, or attribute wasn't defined return XmlCaseOrder.None; } if (value == Keywords.s_UpperFirst) { return XmlCaseOrder.UpperFirst; } if (value == Keywords.s_LowerFirst) { return XmlCaseOrder.LowerFirst; } if (this.forwardCompatibility) { return XmlCaseOrder.None; } throw XsltException.Create(Res.Xslt_InvalidAttrValue, Keywords.s_CaseOrder, value); } internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckEmpty(compiler); if (selectKey == Compiler.InvalidQueryKey) { selectKey = compiler.AddQuery("."); } this.forwardCompatibility = compiler.ForwardCompatibility; this.manager = compiler.CloneScopeManager(); this.lang = ParseLang( PrecalculateAvt(ref this.langAvt )); this.dataType = ParseDataType( PrecalculateAvt(ref this.dataTypeAvt ), manager); this.order = ParseOrder( PrecalculateAvt(ref this.orderAvt )); this.caseOrder = ParseCaseOrder(PrecalculateAvt(ref this.caseOrderAvt)); if(this.langAvt == null && this.dataTypeAvt == null && this.orderAvt == null && this.caseOrderAvt == null) { this.sort = new Sort(this.selectKey, this.lang, this.dataType, this.order, this.caseOrder); } } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Select)) { this.selectKey = compiler.AddQuery(value); } else if (Keywords.Equals(name, compiler.Atoms.Lang)) { this.langAvt = Avt.CompileAvt(compiler, value); } else if (Keywords.Equals(name, compiler.Atoms.DataType)) { this.dataTypeAvt = Avt.CompileAvt(compiler, value); } else if (Keywords.Equals(name, compiler.Atoms.Order)) { this.orderAvt = Avt.CompileAvt(compiler, value); } else if (Keywords.Equals(name, compiler.Atoms.CaseOrder)) { this.caseOrderAvt = Avt.CompileAvt(compiler, value); } else { return false; } return true; } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); Debug.Assert(frame.State == Initialized); processor.AddSort(this.sort != null ? this.sort : new Sort( this.selectKey, this.langAvt == null ? this.lang : ParseLang( this.langAvt .Evaluate(processor, frame)), this.dataTypeAvt == null ? this.dataType : ParseDataType( this.dataTypeAvt .Evaluate(processor, frame), manager), this.orderAvt == null ? this.order : ParseOrder( this.orderAvt .Evaluate(processor, frame)), this.caseOrderAvt == null ? this.caseOrder : ParseCaseOrder(this.caseOrderAvt.Evaluate(processor, frame)) ) ); frame.Finished(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Globalization; using System.Xml; using System.Xml.XPath; internal class SortAction : CompiledAction { private int selectKey = Compiler.InvalidQueryKey; private Avt langAvt; private Avt dataTypeAvt; private Avt orderAvt; private Avt caseOrderAvt; // Compile time precalculated AVTs private string lang; private XmlDataType dataType = XmlDataType.Text; private XmlSortOrder order = XmlSortOrder.Ascending; private XmlCaseOrder caseOrder = XmlCaseOrder.None; private Sort sort; //When we not have AVTs at all we can do this. null otherwise. private bool forwardCompatibility; private InputScopeManager manager; private string ParseLang(string value) { if(value == null) { // Avt is not constant, or attribute wasn't defined return null; } // XmlComplianceUtil.IsValidLanguageID uses the outdated RFC 1766. It would be // better to remove this method completely and not call it here, but that may // change exception types for some stylesheets. if (! XmlComplianceUtil.IsValidLanguageID(value.ToCharArray(), 0, value.Length) && (value.Length == 0 || CultureInfo.GetCultureInfo(value) == null) ) { if (this.forwardCompatibility) { return null; } throw XsltException.Create(Res.Xslt_InvalidAttrValue, Keywords.s_Lang, value); } return value; } private XmlDataType ParseDataType(string value, InputScopeManager manager) { if(value == null) { // Avt is not constant, or attribute wasn't defined return XmlDataType.Text; } if (value == Keywords.s_Text) { return XmlDataType.Text; } if (value == Keywords.s_Number) { return XmlDataType.Number; } String prefix, localname; PrefixQName.ParseQualifiedName(value, out prefix, out localname); manager.ResolveXmlNamespace(prefix); if (prefix.Length == 0 && ! this.forwardCompatibility) { throw XsltException.Create(Res.Xslt_InvalidAttrValue, Keywords.s_DataType, value); } return XmlDataType.Text; } private XmlSortOrder ParseOrder(string value) { if(value == null) { // Avt is not constant, or attribute wasn't defined return XmlSortOrder.Ascending; } if (value == Keywords.s_Ascending) { return XmlSortOrder.Ascending; } if (value == Keywords.s_Descending) { return XmlSortOrder.Descending; } if (this.forwardCompatibility) { return XmlSortOrder.Ascending; } throw XsltException.Create(Res.Xslt_InvalidAttrValue, Keywords.s_Order, value); } private XmlCaseOrder ParseCaseOrder(string value) { if(value == null) { // Avt is not constant, or attribute wasn't defined return XmlCaseOrder.None; } if (value == Keywords.s_UpperFirst) { return XmlCaseOrder.UpperFirst; } if (value == Keywords.s_LowerFirst) { return XmlCaseOrder.LowerFirst; } if (this.forwardCompatibility) { return XmlCaseOrder.None; } throw XsltException.Create(Res.Xslt_InvalidAttrValue, Keywords.s_CaseOrder, value); } internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckEmpty(compiler); if (selectKey == Compiler.InvalidQueryKey) { selectKey = compiler.AddQuery("."); } this.forwardCompatibility = compiler.ForwardCompatibility; this.manager = compiler.CloneScopeManager(); this.lang = ParseLang( PrecalculateAvt(ref this.langAvt )); this.dataType = ParseDataType( PrecalculateAvt(ref this.dataTypeAvt ), manager); this.order = ParseOrder( PrecalculateAvt(ref this.orderAvt )); this.caseOrder = ParseCaseOrder(PrecalculateAvt(ref this.caseOrderAvt)); if(this.langAvt == null && this.dataTypeAvt == null && this.orderAvt == null && this.caseOrderAvt == null) { this.sort = new Sort(this.selectKey, this.lang, this.dataType, this.order, this.caseOrder); } } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Select)) { this.selectKey = compiler.AddQuery(value); } else if (Keywords.Equals(name, compiler.Atoms.Lang)) { this.langAvt = Avt.CompileAvt(compiler, value); } else if (Keywords.Equals(name, compiler.Atoms.DataType)) { this.dataTypeAvt = Avt.CompileAvt(compiler, value); } else if (Keywords.Equals(name, compiler.Atoms.Order)) { this.orderAvt = Avt.CompileAvt(compiler, value); } else if (Keywords.Equals(name, compiler.Atoms.CaseOrder)) { this.caseOrderAvt = Avt.CompileAvt(compiler, value); } else { return false; } return true; } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); Debug.Assert(frame.State == Initialized); processor.AddSort(this.sort != null ? this.sort : new Sort( this.selectKey, this.langAvt == null ? this.lang : ParseLang( this.langAvt .Evaluate(processor, frame)), this.dataTypeAvt == null ? this.dataType : ParseDataType( this.dataTypeAvt .Evaluate(processor, frame), manager), this.orderAvt == null ? this.order : ParseOrder( this.orderAvt .Evaluate(processor, frame)), this.caseOrderAvt == null ? this.caseOrder : ParseCaseOrder(this.caseOrderAvt.Evaluate(processor, frame)) ) ); frame.Finished(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
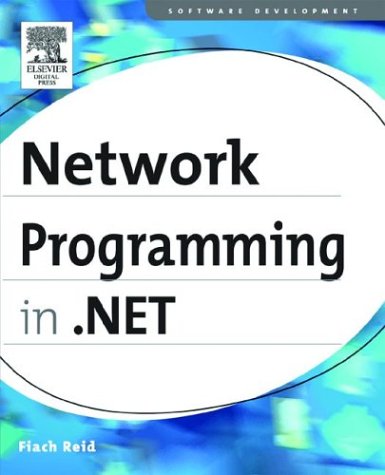
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RolePrincipal.cs
- EntityCommandExecutionException.cs
- TypeToken.cs
- ForeignKeyConstraint.cs
- GeometryDrawing.cs
- FixedTextContainer.cs
- GridViewRowPresenter.cs
- ObjectStateEntryDbDataRecord.cs
- CacheEntry.cs
- DllNotFoundException.cs
- HostingPreferredMapPath.cs
- CapabilitiesAssignment.cs
- WindowsAltTab.cs
- XmlNodeChangedEventManager.cs
- ConstantCheck.cs
- XmlSchemaImporter.cs
- GuidConverter.cs
- TransactionContextValidator.cs
- MailWebEventProvider.cs
- DeviceContext.cs
- UTF32Encoding.cs
- GACIdentityPermission.cs
- NativeMethods.cs
- InheritedPropertyChangedEventArgs.cs
- CompareValidator.cs
- XmlFormatWriterGenerator.cs
- TextDecorations.cs
- HttpCookiesSection.cs
- ResolveResponse.cs
- KeyedByTypeCollection.cs
- XmlNamespaceDeclarationsAttribute.cs
- SourceChangedEventArgs.cs
- ProcessHostMapPath.cs
- UriSection.cs
- SQLStringStorage.cs
- ClearCollection.cs
- DotExpr.cs
- XmlSerializerNamespaces.cs
- Privilege.cs
- ClientRolePrincipal.cs
- ExpressionBuilder.cs
- AstTree.cs
- UserPreferenceChangedEventArgs.cs
- ContentElement.cs
- FormViewPagerRow.cs
- DropShadowBitmapEffect.cs
- HideDisabledControlAdapter.cs
- PKCS1MaskGenerationMethod.cs
- RbTree.cs
- GridItemPattern.cs
- BrushValueSerializer.cs
- BinaryCommonClasses.cs
- SqlFunctionAttribute.cs
- ChildTable.cs
- VisualTreeHelper.cs
- XPathDocumentBuilder.cs
- ChtmlCalendarAdapter.cs
- ArrayItemReference.cs
- DoubleLinkListEnumerator.cs
- ContourSegment.cs
- LineServicesCallbacks.cs
- RoleService.cs
- RequestCache.cs
- WebFormsRootDesigner.cs
- AppDomainFactory.cs
- RouteParametersHelper.cs
- Style.cs
- GlobalizationAssembly.cs
- OleDbFactory.cs
- DigestComparer.cs
- XmlCustomFormatter.cs
- UnmanagedMemoryStreamWrapper.cs
- XmlReaderDelegator.cs
- DateTimeOffsetAdapter.cs
- IncrementalReadDecoders.cs
- MsmqProcessProtocolHandler.cs
- ImageField.cs
- GrammarBuilderRuleRef.cs
- ProfileSettingsCollection.cs
- HttpListenerRequest.cs
- ComPlusSynchronizationContext.cs
- SocketElement.cs
- X509Certificate2.cs
- Token.cs
- StrongNameMembershipCondition.cs
- ItemsControlAutomationPeer.cs
- ValidatingReaderNodeData.cs
- CommandEventArgs.cs
- ColorConvertedBitmap.cs
- DataGridViewCellStyle.cs
- QueryInterceptorAttribute.cs
- unsafeIndexingFilterStream.cs
- GeneralTransform.cs
- MutexSecurity.cs
- CryptoApi.cs
- Rotation3DAnimation.cs
- RestClientProxyHandler.cs
- FlowPanelDesigner.cs
- PrinterResolution.cs
- SslStreamSecurityUpgradeProvider.cs