Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / State / ISessionStateStore.cs / 1 / ISessionStateStore.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * SessionStateStoreProviderBase * */ namespace System.Web.SessionState { using System.Xml; using System.Security.Permissions; using System.Configuration.Provider; using System.Collections.Specialized; [FlagsAttribute()] internal enum SessionStateItemFlags : int { None = 0x00000000, Uninitialized = 0x00000001, IgnoreCacheItemRemoved = 0x00000002 } [FlagsAttribute()] public enum SessionStateActions : int { None = 0x00000000, InitializeItem = 0x00000001 } // This interface is used by SessionStateModule to read/write the session state data [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class SessionStateStoreProviderBase : ProviderBase { public abstract void Dispose(); // Called by SessionStateModule to notify the provider that Session_End is defined // in global.asax, and so when an item expires, it should call the expireCallback // If the provider does not support session expiry, it should return false. public abstract bool SetItemExpireCallback(SessionStateItemExpireCallback expireCallback); // Called at the beginning of the AcquireRequestState event public abstract void InitializeRequest(HttpContext context); // Get and return a SessionStateStoreData. // Please note that we are implementing a reader/writer lock mechanism. // // If successful: // - returns the item // // If not found: // - set 'locked' to false // - returns null // // If the item is already locked by another request: // - set 'locked' to true // - set 'lockAge' to how long has the item been locked // - set 'lockId' to the context of the lock // - returns null public abstract SessionStateStoreData GetItem(HttpContext context, String id, out bool locked, out TimeSpan lockAge, out object lockId, out SessionStateActions actions); // Get and lock a SessionStateStoreData. // Please note that we are implementing a reader/writer lock mechanism. // // If successful: // - set 'lockId' to the context of the lock // - returns the item // // If not found: // - set 'locked' to false // - returns null // // If the item is already locked by another request: // - set 'locked' to true // - set 'lockAge' to how long has the item been locked // - set 'lockId' to the context of the lock // - returns null public abstract SessionStateStoreData GetItemExclusive(HttpContext context, String id, out bool locked, out TimeSpan lockAge, out object lockId, out SessionStateActions actions); // Unlock an item locked by GetExclusive // 'lockId' is the lock context returned by previous call to GetExclusive public abstract void ReleaseItemExclusive(HttpContext context, String id, object lockId); // Write an item. // Note: The item is originally obtained by GetExclusive // Because SessionStateModule will release (by ReleaseExclusive) am item if // it has been locked for too long, so it is possible that the request calling // Set() may have lost the lock to someone else already. This can be // discovered by comparing the supplied lockId with the lockId value // stored with the state item. public abstract void SetAndReleaseItemExclusive(HttpContext context, String id, SessionStateStoreData item, object lockId, bool newItem); // Remove an item. See the note in Set. public abstract void RemoveItem(HttpContext context, String id, object lockId, SessionStateStoreData item); // Reset the expire time of an item based on its timeout value public abstract void ResetItemTimeout(HttpContext context, String id); // Create a brand new SessionStateStoreData. The created SessionStateStoreData must have // a non-null ISessionStateItemCollection. public abstract SessionStateStoreData CreateNewStoreData(HttpContext context, int timeout); public abstract void CreateUninitializedItem(HttpContext context, String id, int timeout); // Called during EndRequest event public abstract void EndRequest(HttpContext context); internal virtual void Initialize(string name, NameValueCollection config, IPartitionResolver partitionResolver) { } } [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class SessionStateStoreData { ISessionStateItemCollection _sessionItems; HttpStaticObjectsCollection _staticObjects; int _timeout; public SessionStateStoreData(ISessionStateItemCollection sessionItems, HttpStaticObjectsCollection staticObjects, int timeout) { _sessionItems = sessionItems; _staticObjects = staticObjects; _timeout = timeout; } virtual public ISessionStateItemCollection Items { get { return _sessionItems; } } virtual public HttpStaticObjectsCollection StaticObjects { get { return _staticObjects; } } virtual public int Timeout { get { return _timeout; } set { _timeout = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * SessionStateStoreProviderBase * */ namespace System.Web.SessionState { using System.Xml; using System.Security.Permissions; using System.Configuration.Provider; using System.Collections.Specialized; [FlagsAttribute()] internal enum SessionStateItemFlags : int { None = 0x00000000, Uninitialized = 0x00000001, IgnoreCacheItemRemoved = 0x00000002 } [FlagsAttribute()] public enum SessionStateActions : int { None = 0x00000000, InitializeItem = 0x00000001 } // This interface is used by SessionStateModule to read/write the session state data [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class SessionStateStoreProviderBase : ProviderBase { public abstract void Dispose(); // Called by SessionStateModule to notify the provider that Session_End is defined // in global.asax, and so when an item expires, it should call the expireCallback // If the provider does not support session expiry, it should return false. public abstract bool SetItemExpireCallback(SessionStateItemExpireCallback expireCallback); // Called at the beginning of the AcquireRequestState event public abstract void InitializeRequest(HttpContext context); // Get and return a SessionStateStoreData. // Please note that we are implementing a reader/writer lock mechanism. // // If successful: // - returns the item // // If not found: // - set 'locked' to false // - returns null // // If the item is already locked by another request: // - set 'locked' to true // - set 'lockAge' to how long has the item been locked // - set 'lockId' to the context of the lock // - returns null public abstract SessionStateStoreData GetItem(HttpContext context, String id, out bool locked, out TimeSpan lockAge, out object lockId, out SessionStateActions actions); // Get and lock a SessionStateStoreData. // Please note that we are implementing a reader/writer lock mechanism. // // If successful: // - set 'lockId' to the context of the lock // - returns the item // // If not found: // - set 'locked' to false // - returns null // // If the item is already locked by another request: // - set 'locked' to true // - set 'lockAge' to how long has the item been locked // - set 'lockId' to the context of the lock // - returns null public abstract SessionStateStoreData GetItemExclusive(HttpContext context, String id, out bool locked, out TimeSpan lockAge, out object lockId, out SessionStateActions actions); // Unlock an item locked by GetExclusive // 'lockId' is the lock context returned by previous call to GetExclusive public abstract void ReleaseItemExclusive(HttpContext context, String id, object lockId); // Write an item. // Note: The item is originally obtained by GetExclusive // Because SessionStateModule will release (by ReleaseExclusive) am item if // it has been locked for too long, so it is possible that the request calling // Set() may have lost the lock to someone else already. This can be // discovered by comparing the supplied lockId with the lockId value // stored with the state item. public abstract void SetAndReleaseItemExclusive(HttpContext context, String id, SessionStateStoreData item, object lockId, bool newItem); // Remove an item. See the note in Set. public abstract void RemoveItem(HttpContext context, String id, object lockId, SessionStateStoreData item); // Reset the expire time of an item based on its timeout value public abstract void ResetItemTimeout(HttpContext context, String id); // Create a brand new SessionStateStoreData. The created SessionStateStoreData must have // a non-null ISessionStateItemCollection. public abstract SessionStateStoreData CreateNewStoreData(HttpContext context, int timeout); public abstract void CreateUninitializedItem(HttpContext context, String id, int timeout); // Called during EndRequest event public abstract void EndRequest(HttpContext context); internal virtual void Initialize(string name, NameValueCollection config, IPartitionResolver partitionResolver) { } } [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class SessionStateStoreData { ISessionStateItemCollection _sessionItems; HttpStaticObjectsCollection _staticObjects; int _timeout; public SessionStateStoreData(ISessionStateItemCollection sessionItems, HttpStaticObjectsCollection staticObjects, int timeout) { _sessionItems = sessionItems; _staticObjects = staticObjects; _timeout = timeout; } virtual public ISessionStateItemCollection Items { get { return _sessionItems; } } virtual public HttpStaticObjectsCollection StaticObjects { get { return _staticObjects; } } virtual public int Timeout { get { return _timeout; } set { _timeout = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
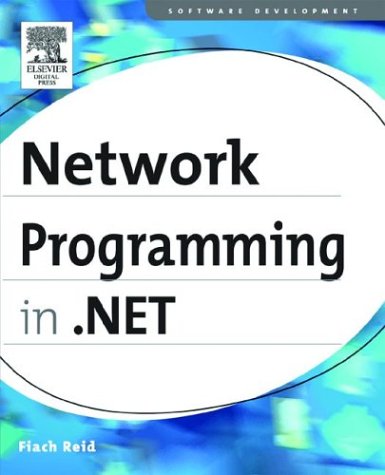
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AlignmentYValidation.cs
- PersonalizationStateQuery.cs
- FieldAccessException.cs
- Page.cs
- DtcInterfaces.cs
- XmlNullResolver.cs
- XmlSchemaAny.cs
- WorkflowInstanceProvider.cs
- ResourcesChangeInfo.cs
- BrushValueSerializer.cs
- WorkflowStateRollbackService.cs
- EmptyElement.cs
- CompareInfo.cs
- QilBinary.cs
- ObjectItemAttributeAssemblyLoader.cs
- ToolboxItemLoader.cs
- EditorPartCollection.cs
- ToolStripDropDownItem.cs
- SystemWebSectionGroup.cs
- IOException.cs
- PathGradientBrush.cs
- WindowsRichEditRange.cs
- StylusPointPropertyUnit.cs
- webeventbuffer.cs
- Convert.cs
- XmlTypeMapping.cs
- KnownTypeHelper.cs
- ExpressionBindings.cs
- RightNameExpirationInfoPair.cs
- DataGridViewEditingControlShowingEventArgs.cs
- CompilerTypeWithParams.cs
- PkcsUtils.cs
- ISessionStateStore.cs
- SimpleApplicationHost.cs
- ApplicationServicesHostFactory.cs
- TextParentUndoUnit.cs
- InvalidOperationException.cs
- HwndSource.cs
- BasicViewGenerator.cs
- DecimalConstantAttribute.cs
- DataGridViewColumnEventArgs.cs
- CapabilitiesPattern.cs
- SystemPens.cs
- WebPartDeleteVerb.cs
- DebugView.cs
- TextProviderWrapper.cs
- EventWaitHandle.cs
- AsnEncodedData.cs
- Point3DAnimation.cs
- DataGridViewButtonColumn.cs
- TimerElapsedEvenArgs.cs
- WebPartExportVerb.cs
- MenuItemBindingCollection.cs
- HuffmanTree.cs
- ZipArchive.cs
- AtomServiceDocumentSerializer.cs
- BufferedGraphicsContext.cs
- DodSequenceMerge.cs
- Transform.cs
- CompiledQuery.cs
- TCEAdapterGenerator.cs
- XmlDomTextWriter.cs
- LogFlushAsyncResult.cs
- DbConnectionClosed.cs
- _ConnectOverlappedAsyncResult.cs
- EventMappingSettings.cs
- BasicHttpBinding.cs
- FunctionGenerator.cs
- TraceUtility.cs
- SystemInformation.cs
- FixUp.cs
- ManagedFilter.cs
- ObjectHelper.cs
- BinaryNode.cs
- _TimerThread.cs
- BigInt.cs
- WebPartRestoreVerb.cs
- ChannelFactory.cs
- TextTreeTextNode.cs
- HtmlElementEventArgs.cs
- TextCollapsingProperties.cs
- DataAdapter.cs
- ParenthesizePropertyNameAttribute.cs
- Types.cs
- DragDrop.cs
- EventMappingSettingsCollection.cs
- RegionIterator.cs
- XmlDomTextWriter.cs
- PackageController.cs
- HtmlInputFile.cs
- ObjectMaterializedEventArgs.cs
- CustomError.cs
- FixedSOMContainer.cs
- SettingsBindableAttribute.cs
- DataPagerCommandEventArgs.cs
- KeyedHashAlgorithm.cs
- XmlDesignerDataSourceView.cs
- DataSourceHelper.cs
- MsmqIntegrationProcessProtocolHandler.cs
- BulletedListDesigner.cs