Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlInputControl.cs / 1 / HtmlInputControl.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlInputControl.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System; using System.ComponentModel; using System.Web; using System.Web.UI; using Debug=System.Web.Util.Debug; using System.Security.Permissions; /* * An abstract base class representing an intrinsic INPUT tag. */ ////// [ ControlBuilderAttribute(typeof(HtmlEmptyTagControlBuilder)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] abstract public class HtmlInputControl : HtmlControl { private string _type; /* * Creates a new Input */ ////// The ///abstract class defines /// the methods, properties, and events common to all HTML input controls. /// These include controls for the <input type=text>, <input /// type=submit>, and <input type=file> elements. /// /// protected HtmlInputControl(string type) : base("input") { _type = type; // VSWhidbey 546690: Need to add the type value to the Attributes collection to match Everett behavior. Attributes["type"] = type; } /* * Name property */ ///Initializes a new instance of the ///class. /// [ WebCategory("Behavior"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public virtual string Name { get { return UniqueID; //string s = Attributes["name"]; //return ((s != null) ? s : String.Empty); } set { //Attributes["name"] = MapStringAttributeToString(value); } } // Value that gets rendered for the Name attribute internal virtual string RenderedNameAttribute { get { return Name; //string name = Name; //if (name.Length == 0) // return UniqueID; //return name; } } /* * Value property. */ ////// Gets the value of the HTML /// Name attribute that will be rendered to the browser. /// ////// [ WebCategory("Appearance"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public virtual string Value { get { string s = Attributes["value"]; return((s != null) ? s : String.Empty); } set { Attributes["value"] = MapStringAttributeToString(value); } } /* * Type of input */ ////// Gets or sets the contents of a text box. /// ////// [ WebCategory("Behavior"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Type { get { string s = Attributes["type"]; if (!string.IsNullOrEmpty(s)) { return s; } return((_type != null) ? _type : String.Empty); } } /* * Override to render unique name attribute. * The name attribute is owned by the framework. */ ////// Gets the Type attribute for a particular HTML input control. /// ////// /// protected override void RenderAttributes(HtmlTextWriter writer) { writer.WriteAttribute("name", RenderedNameAttribute); Attributes.Remove("name"); bool removedTypeAttribute = false; string type = Type; if (!String.IsNullOrEmpty(type)) { writer.WriteAttribute("type", type); Attributes.Remove("type"); removedTypeAttribute = true; } base.RenderAttributes(writer); if (removedTypeAttribute && DesignMode) { Attributes.Add("type", type); } writer.Write(" /"); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlInputControl.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System; using System.ComponentModel; using System.Web; using System.Web.UI; using Debug=System.Web.Util.Debug; using System.Security.Permissions; /* * An abstract base class representing an intrinsic INPUT tag. */ ////// [ ControlBuilderAttribute(typeof(HtmlEmptyTagControlBuilder)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] abstract public class HtmlInputControl : HtmlControl { private string _type; /* * Creates a new Input */ ////// The ///abstract class defines /// the methods, properties, and events common to all HTML input controls. /// These include controls for the <input type=text>, <input /// type=submit>, and <input type=file> elements. /// /// protected HtmlInputControl(string type) : base("input") { _type = type; // VSWhidbey 546690: Need to add the type value to the Attributes collection to match Everett behavior. Attributes["type"] = type; } /* * Name property */ ///Initializes a new instance of the ///class. /// [ WebCategory("Behavior"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public virtual string Name { get { return UniqueID; //string s = Attributes["name"]; //return ((s != null) ? s : String.Empty); } set { //Attributes["name"] = MapStringAttributeToString(value); } } // Value that gets rendered for the Name attribute internal virtual string RenderedNameAttribute { get { return Name; //string name = Name; //if (name.Length == 0) // return UniqueID; //return name; } } /* * Value property. */ ////// Gets the value of the HTML /// Name attribute that will be rendered to the browser. /// ////// [ WebCategory("Appearance"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public virtual string Value { get { string s = Attributes["value"]; return((s != null) ? s : String.Empty); } set { Attributes["value"] = MapStringAttributeToString(value); } } /* * Type of input */ ////// Gets or sets the contents of a text box. /// ////// [ WebCategory("Behavior"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Type { get { string s = Attributes["type"]; if (!string.IsNullOrEmpty(s)) { return s; } return((_type != null) ? _type : String.Empty); } } /* * Override to render unique name attribute. * The name attribute is owned by the framework. */ ////// Gets the Type attribute for a particular HTML input control. /// ////// /// protected override void RenderAttributes(HtmlTextWriter writer) { writer.WriteAttribute("name", RenderedNameAttribute); Attributes.Remove("name"); bool removedTypeAttribute = false; string type = Type; if (!String.IsNullOrEmpty(type)) { writer.WriteAttribute("type", type); Attributes.Remove("type"); removedTypeAttribute = true; } base.RenderAttributes(writer); if (removedTypeAttribute && DesignMode) { Attributes.Add("type", type); } writer.Write(" /"); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
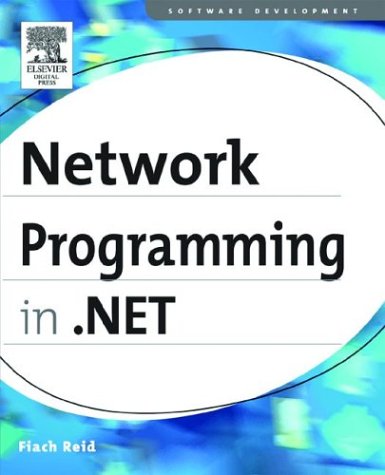
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OpenTypeCommon.cs
- ListViewItemEventArgs.cs
- DbDataSourceEnumerator.cs
- WebPartUserCapability.cs
- NativeMethods.cs
- ProcessStartInfo.cs
- TrackBarDesigner.cs
- StateWorkerRequest.cs
- contentDescriptor.cs
- PartBasedPackageProperties.cs
- IdentityHolder.cs
- FormViewPagerRow.cs
- MetadataArtifactLoaderCompositeResource.cs
- WebPartEditVerb.cs
- PrintPreviewControl.cs
- XPathNodeInfoAtom.cs
- AlignmentXValidation.cs
- NameValueConfigurationCollection.cs
- contentDescriptor.cs
- SchemaImporterExtensionsSection.cs
- JavaScriptObjectDeserializer.cs
- ScrollChangedEventArgs.cs
- Misc.cs
- Encoder.cs
- WebServiceClientProxyGenerator.cs
- CodeIdentifier.cs
- DataTableTypeConverter.cs
- XmlSchemas.cs
- ObjectListDesigner.cs
- MultiPropertyDescriptorGridEntry.cs
- RoleServiceManager.cs
- SafeNativeMethods.cs
- ArrayList.cs
- HttpValueCollection.cs
- ScrollBar.cs
- DataGridViewToolTip.cs
- VSWCFServiceContractGenerator.cs
- MetaModel.cs
- xmlsaver.cs
- CommentAction.cs
- DocumentPageHost.cs
- HttpProtocolReflector.cs
- FontStretchConverter.cs
- BlobPersonalizationState.cs
- MdiWindowListItemConverter.cs
- CloudCollection.cs
- GetLedgerRequest.cs
- HttpHeaderCollection.cs
- PerformanceCounterLib.cs
- Vector3D.cs
- UdpTransportSettings.cs
- ProfileServiceManager.cs
- DataRow.cs
- CellQuery.cs
- ProviderConnectionPoint.cs
- DataColumnCollection.cs
- ParallelRangeManager.cs
- DataControlPagerLinkButton.cs
- ProcessHostMapPath.cs
- Decoder.cs
- PkcsMisc.cs
- PipelineModuleStepContainer.cs
- CharUnicodeInfo.cs
- DesignerHelpers.cs
- NegationPusher.cs
- Pointer.cs
- MimeMapping.cs
- DataGridParentRows.cs
- FileController.cs
- LingerOption.cs
- CustomValidator.cs
- UdpDiscoveryEndpointElement.cs
- HtmlEmptyTagControlBuilder.cs
- MailDefinition.cs
- DataTableCollection.cs
- ServiceThrottle.cs
- ArglessEventHandlerProxy.cs
- HtmlInputControl.cs
- COM2PictureConverter.cs
- Array.cs
- DocComment.cs
- State.cs
- IdlingCommunicationPool.cs
- SrgsRulesCollection.cs
- TypeHelper.cs
- ReachPrintTicketSerializerAsync.cs
- COM2IProvidePropertyBuilderHandler.cs
- ImageSource.cs
- controlskin.cs
- SafeReversePInvokeHandle.cs
- ParagraphVisual.cs
- EntityConnection.cs
- Rotation3DAnimation.cs
- Currency.cs
- QuotedPrintableStream.cs
- SelectionRange.cs
- Rect3DValueSerializer.cs
- Scalars.cs
- TypeDescriptionProvider.cs
- Directory.cs