Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / ResourceLoader.cs / 1 / ResourceLoader.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.IO; using System.Net; using System.Speech.Synthesis; #if SPEECHSERVER || PROMPT_ENGINE using System.Speech.Synthesis.TtsEngine; #endif namespace System.Speech.Internal { //******************************************************************* // // Public Types // //******************************************************************* internal class ResourceLoader { //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods ////// Load a file either from a local network or from the Internet. /// /// /// /// /// internal Stream LoadFile (Uri uri, out string mimeType, out Uri baseUri, out string localPath) { localPath = null; #if SPEECHSERVER || PROMPT_ENGINE if (_resourceLoader != null) { localPath = _resourceLoader.GetLocalCopy (uri, out mimeType, out baseUri); return new FileStream (localPath, FileMode.Open, FileAccess.Read, FileShare.Read); } else #endif { Stream stream = null; // Check for a local file if (!uri.IsAbsoluteUri || uri.IsFile) { // Local file string file = uri.IsAbsoluteUri ? uri.LocalPath : uri.OriginalString; try { stream = new FileStream (file, FileMode.Open, FileAccess.Read, FileShare.Read); } catch { if (Directory.Exists (file)) { throw new InvalidOperationException (SR.Get (SRID.CannotReadFromDirectory, file)); } throw; } baseUri = null; } else { try { // http:// Load the data from the web stream = DownloadData (uri, out baseUri); } catch (WebException e) { throw new IOException (e.Message, e); } } mimeType = null; return stream; } } ////// Release a file from a cache if any /// /// internal void UnloadFile (string localPath) { #if SPEECHSERVER || PROMPT_ENGINE if (_resourceLoader != null) { _resourceLoader.ReleaseLocalCopy (localPath); } #endif } internal Stream LoadFile (Uri uri, out string localPath, out Uri redirectedUri) { string mediaTypeUnused; return LoadFile (uri, out mediaTypeUnused, out redirectedUri, out localPath); } #if SPEECHSERVER || PROMPT_ENGINE ////// Only one instance of the resource loader /// /// internal void SetResourceLoader (ISpeechResourceLoader resourceLoader) { // Changing the ResourceLoader if (_resourceLoader != null && resourceLoader != _resourceLoader) { throw new InvalidOperationException (); } _resourceLoader = resourceLoader; } #endif #endregion //******************************************************************** // // Private Methods // //******************************************************************** #region Private Methods ////// Dowload data from the web. /// Set the redirectUri as the location of the file could be redirected in ASP pages. /// /// /// ///private static Stream DownloadData (Uri uri, out Uri redirectedUri) { // Create a request for the URL. WebRequest request = WebRequest.Create (uri); // If required by the server, set the credentials. request.Credentials = CredentialCache.DefaultCredentials; // Get the response. using (HttpWebResponse response = (HttpWebResponse) request.GetResponse ()) { // Get the stream containing content returned by the server. using (Stream dataStream = response.GetResponseStream ()) { redirectedUri = response.ResponseUri; // http:// Load the data from the web using (WebClient client = new WebClient ()) { client.UseDefaultCredentials = true; return new MemoryStream (client.DownloadData (redirectedUri)); } } } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields #if SPEECHSERVER || PROMPT_ENGINE ISpeechResourceLoader _resourceLoader; #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.IO; using System.Net; using System.Speech.Synthesis; #if SPEECHSERVER || PROMPT_ENGINE using System.Speech.Synthesis.TtsEngine; #endif namespace System.Speech.Internal { //******************************************************************* // // Public Types // //******************************************************************* internal class ResourceLoader { //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods ////// Load a file either from a local network or from the Internet. /// /// /// /// /// internal Stream LoadFile (Uri uri, out string mimeType, out Uri baseUri, out string localPath) { localPath = null; #if SPEECHSERVER || PROMPT_ENGINE if (_resourceLoader != null) { localPath = _resourceLoader.GetLocalCopy (uri, out mimeType, out baseUri); return new FileStream (localPath, FileMode.Open, FileAccess.Read, FileShare.Read); } else #endif { Stream stream = null; // Check for a local file if (!uri.IsAbsoluteUri || uri.IsFile) { // Local file string file = uri.IsAbsoluteUri ? uri.LocalPath : uri.OriginalString; try { stream = new FileStream (file, FileMode.Open, FileAccess.Read, FileShare.Read); } catch { if (Directory.Exists (file)) { throw new InvalidOperationException (SR.Get (SRID.CannotReadFromDirectory, file)); } throw; } baseUri = null; } else { try { // http:// Load the data from the web stream = DownloadData (uri, out baseUri); } catch (WebException e) { throw new IOException (e.Message, e); } } mimeType = null; return stream; } } ////// Release a file from a cache if any /// /// internal void UnloadFile (string localPath) { #if SPEECHSERVER || PROMPT_ENGINE if (_resourceLoader != null) { _resourceLoader.ReleaseLocalCopy (localPath); } #endif } internal Stream LoadFile (Uri uri, out string localPath, out Uri redirectedUri) { string mediaTypeUnused; return LoadFile (uri, out mediaTypeUnused, out redirectedUri, out localPath); } #if SPEECHSERVER || PROMPT_ENGINE ////// Only one instance of the resource loader /// /// internal void SetResourceLoader (ISpeechResourceLoader resourceLoader) { // Changing the ResourceLoader if (_resourceLoader != null && resourceLoader != _resourceLoader) { throw new InvalidOperationException (); } _resourceLoader = resourceLoader; } #endif #endregion //******************************************************************** // // Private Methods // //******************************************************************** #region Private Methods ////// Dowload data from the web. /// Set the redirectUri as the location of the file could be redirected in ASP pages. /// /// /// ///private static Stream DownloadData (Uri uri, out Uri redirectedUri) { // Create a request for the URL. WebRequest request = WebRequest.Create (uri); // If required by the server, set the credentials. request.Credentials = CredentialCache.DefaultCredentials; // Get the response. using (HttpWebResponse response = (HttpWebResponse) request.GetResponse ()) { // Get the stream containing content returned by the server. using (Stream dataStream = response.GetResponseStream ()) { redirectedUri = response.ResponseUri; // http:// Load the data from the web using (WebClient client = new WebClient ()) { client.UseDefaultCredentials = true; return new MemoryStream (client.DownloadData (redirectedUri)); } } } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields #if SPEECHSERVER || PROMPT_ENGINE ISpeechResourceLoader _resourceLoader; #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
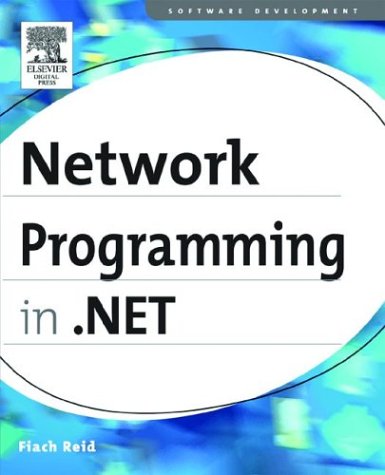
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GacUtil.cs
- FontSource.cs
- SignerInfo.cs
- TrackPointCollection.cs
- RawKeyboardInputReport.cs
- DocumentPageView.cs
- BindingList.cs
- CodeParameterDeclarationExpression.cs
- ExpressionCopier.cs
- PostBackOptions.cs
- HandlerMappingMemo.cs
- OleDbReferenceCollection.cs
- BindingExpression.cs
- ZoomPercentageConverter.cs
- TripleDES.cs
- ObjectStateEntry.cs
- SchemaAttDef.cs
- WsdlInspector.cs
- PageBuildProvider.cs
- Preprocessor.cs
- XmlDictionary.cs
- XmlArrayAttribute.cs
- RawAppCommandInputReport.cs
- TimeSpanStorage.cs
- AsmxEndpointPickerExtension.cs
- ApplicationTrust.cs
- WebBaseEventKeyComparer.cs
- FreeFormDragDropManager.cs
- exports.cs
- TextBoxBase.cs
- SqlCachedBuffer.cs
- StreamReader.cs
- Button.cs
- WebServiceMethodData.cs
- COM2EnumConverter.cs
- Literal.cs
- DeflateEmulationStream.cs
- DynamicEndpoint.cs
- ClientEventManager.cs
- DataGridViewCellMouseEventArgs.cs
- CodeCommentStatement.cs
- ExpressionPrinter.cs
- SortExpressionBuilder.cs
- Section.cs
- FilteredReadOnlyMetadataCollection.cs
- basecomparevalidator.cs
- NotFiniteNumberException.cs
- EditableRegion.cs
- Hash.cs
- EntityTemplateFactory.cs
- Marshal.cs
- XmlSchemaRedefine.cs
- ServiceInstanceProvider.cs
- TypeUsageBuilder.cs
- InternalsVisibleToAttribute.cs
- ToolStripArrowRenderEventArgs.cs
- ToolStripSettings.cs
- Console.cs
- Freezable.cs
- OleDbWrapper.cs
- HtmlInputImage.cs
- StylusButtonEventArgs.cs
- RightsManagementSuppressedStream.cs
- WebContext.cs
- UIElementPropertyUndoUnit.cs
- objectquery_tresulttype.cs
- Validator.cs
- TextRenderer.cs
- COM2IDispatchConverter.cs
- PreviewKeyDownEventArgs.cs
- MimeTextImporter.cs
- PrefixHandle.cs
- StaticDataManager.cs
- UnsafeNativeMethods.cs
- ServiceModelDictionary.cs
- InkCanvasInnerCanvas.cs
- CategoryState.cs
- BamlLocalizableResource.cs
- UInt32Converter.cs
- KeyedCollection.cs
- GetImportFileNameRequest.cs
- DbConnectionPoolGroupProviderInfo.cs
- DataGridItem.cs
- ComEventsHelper.cs
- TextSelectionProcessor.cs
- SizeIndependentAnimationStorage.cs
- TextTreeDeleteContentUndoUnit.cs
- BamlTreeNode.cs
- StreamInfo.cs
- AllMembershipCondition.cs
- CopyAttributesAction.cs
- NullExtension.cs
- DataListItemEventArgs.cs
- _NTAuthentication.cs
- InheritanceContextHelper.cs
- WizardPanelChangingEventArgs.cs
- ContentTypeSettingDispatchMessageFormatter.cs
- SQLResource.cs
- InkCanvasSelectionAdorner.cs
- SymbolEqualComparer.cs