Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / Advanced / MetafileHeader.cs / 1 / MetafileHeader.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /*************************************************************************\ * * Copyright (c) 1998-1999, Microsoft Corp. All Rights Reserved. * * Module Name: * * MetafileHeader.cs * * Abstract: * * Native GDI+ MetafileHeader structure. * * Revision History: * * 10/21/1999 [....] * Created it. * \**************************************************************************/ namespace System.Drawing.Imaging { using System.Configuration.Assemblies; using System.Diagnostics; using System; using System.Drawing; using System.Runtime.InteropServices; using System.Drawing.Internal; ////// /// Contains attributes of an /// associated [StructLayout(LayoutKind.Sequential)] public sealed class MetafileHeader { // determine which to use by nullity internal MetafileHeaderWmf wmf; internal MetafileHeaderEmf emf; internal MetafileHeader() { } ///. /// /// /// Gets the type of the associated public MetafileType Type { get { return IsWmf() ? wmf.type : emf.type; } } ///. /// /// /// public int MetafileSize { get { return IsWmf() ? wmf.size : emf.size; } } ////// Gets the size, in bytes, of the associated /// ///. /// /// /// Gets the version number of the associated /// public int Version { get { return IsWmf() ? wmf.version : emf.version; } } /* FxCop rule 'AvoidBuildingNonCallableCode' - Left here in case it is needed in the future. private EmfPlusFlags EmfPlusFlags { get { return IsWmf() ? wmf.emfPlusFlags : emf.emfPlusFlags; } } */ ///. /// /// /// Gets the horizontal resolution, in /// dots-per-inch, of the associated public float DpiX { get { return IsWmf() ? wmf.dpiX : emf.dpiX; } } ///. /// /// /// Gets the vertical resolution, in /// dots-per-inch, of the associated public float DpiY { get { return IsWmf() ? wmf.dpiY : emf.dpiY; } } ///. /// /// /// Gets a public Rectangle Bounds { get { return IsWmf() ? new Rectangle(wmf.X, wmf.Y, wmf.Width, wmf.Height) : new Rectangle(emf.X, emf.Y, emf.Width, emf.Height); } } ///that bounds the associated /// . /// /// /// Returns a value indicating whether the /// associated public bool IsWmf() { if ((wmf == null) && (emf == null)) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); if ((wmf != null) && ((wmf.type == MetafileType.Wmf) || (wmf.type == MetafileType.WmfPlaceable))) return true; else return false; } ///is in the Windows metafile /// format. /// /// /// public bool IsWmfPlaceable() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((wmf != null) && (wmf.type == MetafileType.WmfPlaceable)); } ////// Returns a value indicating whether the /// associated ///is in the Windows Placeable metafile /// format. /// /// /// Returns a value indicating whether the /// associated public bool IsEmf() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type == MetafileType.Emf)); } ///is in the Windows enhanced metafile /// format. /// /// /// Returns a value indicating whether the /// associated public bool IsEmfOrEmfPlus() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type >= MetafileType.Emf)); } ///is in the Windows enhanced metafile /// format or the Windows enhanced metafile plus. /// /// /// Returns a value indicating whether the /// associated public bool IsEmfPlus() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type >= MetafileType.EmfPlusOnly)); } ///is in the Windows enhanced metafile /// plus format. /// /// /// Returns a value indicating whether the /// associated public bool IsEmfPlusDual() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type == MetafileType.EmfPlusDual)); } ///is in the Dual enhanced /// metafile format. This format supports both the enhanced and the enhanced /// plus format. /// /// /// public bool IsEmfPlusOnly() { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return ((emf != null) && (emf.type == MetafileType.EmfPlusOnly)); } ////// Returns a value indicating whether the associated ///supports only the Windows /// enhanced metafile plus format. /// /// /// public bool IsDisplay() { return IsEmfPlus() && ((((int)emf.emfPlusFlags) & ((int)EmfPlusFlags.Display)) != 0); } ////// Returns a value indicating whether the associated ///is device-dependent. /// /// /// Gets the WMF header file for the associated /// public MetaHeader WmfHeader { get { if (wmf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return wmf.WmfHeader; } } ///. /// /// /// /* FxCop rule 'AvoidBuildingNonCallableCode' - Left here in case it is needed in the future. internal SafeNativeMethods.ENHMETAHEADER EmfHeader { get { if (emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return emf.EmfHeader; } } */ ////// Gets the WMF header file for the associated ///. /// /// /// Gets the size, in bytes, of the /// enhanced metafile plus header file. /// public int EmfPlusHeaderSize { get { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return IsWmf() ? wmf.EmfPlusHeaderSize : emf.EmfPlusHeaderSize; } } ////// /// public int LogicalDpiX { get { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return IsWmf() ? wmf.LogicalDpiX : emf.LogicalDpiX; } } ////// Gets the logical horizontal resolution, in /// dots-per-inch, of the associated ///. /// /// /// Gets the logical vertical resolution, in /// dots-per-inch, of the associated public int LogicalDpiY { get { if (wmf == null && emf == null) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); return IsWmf() ? wmf.LogicalDpiY : emf.LogicalDpiX; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.. ///
Link Menu
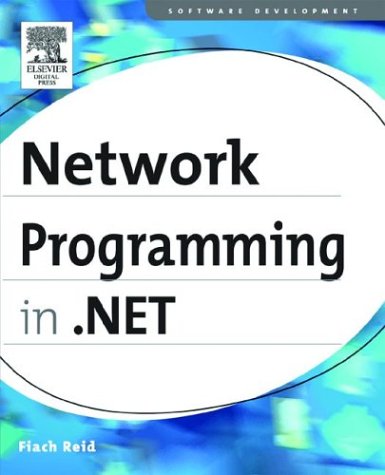
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScriptingJsonSerializationSection.cs
- TraceSource.cs
- WebConfigurationFileMap.cs
- NonValidatingSecurityTokenAuthenticator.cs
- MILUtilities.cs
- DebugHandleTracker.cs
- WinFormsSecurity.cs
- TypeSemantics.cs
- DebugView.cs
- GridViewColumnHeader.cs
- StrokeRenderer.cs
- JsonDataContract.cs
- MessageHeaderDescriptionCollection.cs
- SelectionProcessor.cs
- MenuItemAutomationPeer.cs
- AppManager.cs
- MimePart.cs
- entityreference_tresulttype.cs
- ReadOnlyDataSourceView.cs
- EnterpriseServicesHelper.cs
- PolyLineSegment.cs
- XmlWellformedWriter.cs
- HtmlInputText.cs
- StreamInfo.cs
- CompositeCollection.cs
- TreeViewImageKeyConverter.cs
- ContentAlignmentEditor.cs
- BlobPersonalizationState.cs
- ObjectMemberMapping.cs
- TypeInfo.cs
- FilteredAttributeCollection.cs
- ListViewInsertedEventArgs.cs
- ListBindableAttribute.cs
- StaticFileHandler.cs
- FormsAuthenticationConfiguration.cs
- XmlSchemaRedefine.cs
- StyleHelper.cs
- DataGridItemEventArgs.cs
- ExcCanonicalXml.cs
- TemplateControl.cs
- CellRelation.cs
- FixedSOMContainer.cs
- XmlNodeList.cs
- NamespaceExpr.cs
- WindowPattern.cs
- XPathPatternBuilder.cs
- OdbcConnectionFactory.cs
- SafeFileMappingHandle.cs
- AggregateNode.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- Parser.cs
- UIElement3D.cs
- LayoutTableCell.cs
- SpecialNameAttribute.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- WindowsGraphicsCacheManager.cs
- DataGridViewTextBoxCell.cs
- HttpVersion.cs
- ModelUIElement3D.cs
- ColumnResizeAdorner.cs
- CellLabel.cs
- DataTemplateKey.cs
- TimeoutValidationAttribute.cs
- RelationshipSet.cs
- LogExtent.cs
- HyperLinkField.cs
- HttpWebResponse.cs
- AssertUtility.cs
- CompatibleComparer.cs
- ByteFacetDescriptionElement.cs
- EntityDataSourceStatementEditor.cs
- MappingException.cs
- WebPartsSection.cs
- SamlAdvice.cs
- TextDecorations.cs
- ProcessHost.cs
- DataGridViewCellValueEventArgs.cs
- Int32Rect.cs
- _CommandStream.cs
- ReadOnlyMetadataCollection.cs
- Message.cs
- SerializationObjectManager.cs
- PersonalizationProviderHelper.cs
- UnsignedPublishLicense.cs
- WindowsServiceElement.cs
- ProcessStartInfo.cs
- IListConverters.cs
- Line.cs
- DesignerActionVerbList.cs
- BuildDependencySet.cs
- DirectoryNotFoundException.cs
- MethodCallTranslator.cs
- MachinePropertyVariants.cs
- keycontainerpermission.cs
- NetworkCredential.cs
- SerializableReadOnlyDictionary.cs
- ParseChildrenAsPropertiesAttribute.cs
- SafeFileMapViewHandle.cs
- FixUpCollection.cs
- RecordBuilder.cs