Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / CodeDOM / Compiler / CompilerResults.cs / 1 / CompilerResults.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System; using System.CodeDom; using System.Reflection; using System.Collections; using System.Collections.Specialized; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters.Binary; using System.IO; ////// [Serializable()] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] public class CompilerResults { private CompilerErrorCollection errors = new CompilerErrorCollection(); private StringCollection output = new StringCollection(); private Assembly compiledAssembly; private string pathToAssembly; private int nativeCompilerReturnValue; private TempFileCollection tempFiles; private Evidence evidence; ////// Represents the results /// of compilation from the compiler. /// ////// [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] public CompilerResults(TempFileCollection tempFiles) { this.tempFiles = tempFiles; } ////// Initializes a new instance of ////// that uses the specified /// temporary files. /// /// public TempFileCollection TempFiles { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { return tempFiles; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { tempFiles = value; } } ////// Gets or sets the temporary files to use. /// ////// public Evidence Evidence { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { Evidence e = null; if (evidence != null) e = CloneEvidence(evidence); return e; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [SecurityPermissionAttribute( SecurityAction.Demand, ControlEvidence = true )] set { if (value != null) evidence = CloneEvidence(value); else evidence = null; } } ////// Set the evidence for partially trusted scenarios. /// ////// public Assembly CompiledAssembly { [SecurityPermissionAttribute(SecurityAction.Assert, Flags=SecurityPermissionFlag.ControlEvidence)] get { if (compiledAssembly == null && pathToAssembly != null) { AssemblyName assemName = new AssemblyName(); assemName.CodeBase = pathToAssembly; compiledAssembly = Assembly.Load(assemName,evidence); } return compiledAssembly; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { compiledAssembly = value; } } ////// The compiled assembly. /// ////// public CompilerErrorCollection Errors { get { return errors; } } ////// Gets or sets the collection of compiler errors. /// ////// public StringCollection Output { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { return output; } } ////// Gets or sets the compiler output messages. /// ////// public string PathToAssembly { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { return pathToAssembly; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { pathToAssembly = value; } } ////// Gets or sets the path to the assembly. /// ////// public int NativeCompilerReturnValue { get { return nativeCompilerReturnValue; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { nativeCompilerReturnValue = value; } } internal static Evidence CloneEvidence(Evidence ev) { new PermissionSet( PermissionState.Unrestricted ).Assert(); MemoryStream stream = new MemoryStream(); BinaryFormatter formatter = new BinaryFormatter(); formatter.Serialize( stream, ev ); stream.Position = 0; return (Evidence)formatter.Deserialize( stream ); } } }/// Gets or sets the compiler's return value. /// ///
Link Menu
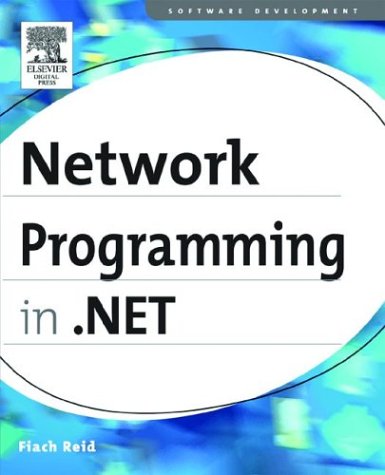
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- JoinElimination.cs
- WebPartDeleteVerb.cs
- ServiceTimeoutsElement.cs
- DataSourceViewSchemaConverter.cs
- DataColumnChangeEvent.cs
- MyContact.cs
- AesCryptoServiceProvider.cs
- SafeLibraryHandle.cs
- SystemWebCachingSectionGroup.cs
- ServiceDebugElement.cs
- DocumentOutline.cs
- RIPEMD160Managed.cs
- EntityWrapper.cs
- DrawingContextDrawingContextWalker.cs
- SQLMoney.cs
- SerializerWriterEventHandlers.cs
- MembershipValidatePasswordEventArgs.cs
- HyperLink.cs
- IntellisenseTextBox.cs
- PropertyValueChangedEvent.cs
- StorageComplexPropertyMapping.cs
- UIElementHelper.cs
- Overlapped.cs
- DiscreteKeyFrames.cs
- TreeNodeBinding.cs
- CanonicalizationDriver.cs
- COM2PropertyDescriptor.cs
- SystemColorTracker.cs
- XmlSchemaSearchPattern.cs
- ConnectionString.cs
- XPathSingletonIterator.cs
- CodeDomComponentSerializationService.cs
- BuildManager.cs
- GeneralTransform3D.cs
- SweepDirectionValidation.cs
- XmlSubtreeReader.cs
- GuidTagList.cs
- XmlAttributeOverrides.cs
- ThreadTrace.cs
- SelectionRange.cs
- URI.cs
- baseaxisquery.cs
- DataSysAttribute.cs
- FileVersionInfo.cs
- LocalizationCodeDomSerializer.cs
- ActivationArguments.cs
- ProfileBuildProvider.cs
- PeerCollaboration.cs
- DbMetaDataColumnNames.cs
- EpmSourceTree.cs
- AppSettingsExpressionBuilder.cs
- NumberFormatter.cs
- IndicCharClassifier.cs
- ProfileGroupSettings.cs
- AuthorizationSection.cs
- ApplyImportsAction.cs
- BaseCodeDomTreeGenerator.cs
- ElapsedEventArgs.cs
- NameValueCollection.cs
- SeverityFilter.cs
- SubMenuStyleCollection.cs
- BatchParser.cs
- TextEditor.cs
- LineMetrics.cs
- SqlComparer.cs
- TdsParser.cs
- CompositeDataBoundControl.cs
- LocatorGroup.cs
- PropertyMapper.cs
- ProfessionalColorTable.cs
- EventLogTraceListener.cs
- XmlNavigatorFilter.cs
- AlternateView.cs
- OutputCacheSettingsSection.cs
- Message.cs
- DialogResultConverter.cs
- ResetableIterator.cs
- DataGridViewRowPostPaintEventArgs.cs
- control.ime.cs
- ValidationPropertyAttribute.cs
- DateTimeOffsetStorage.cs
- TiffBitmapDecoder.cs
- CharEntityEncoderFallback.cs
- Shape.cs
- ColumnResizeAdorner.cs
- WebPartVerbCollection.cs
- ObjectViewFactory.cs
- TransformerInfoCollection.cs
- LinearQuaternionKeyFrame.cs
- Encoding.cs
- ContractSearchPattern.cs
- DbConnectionPoolGroupProviderInfo.cs
- OleTxTransactionInfo.cs
- ConfigXmlWhitespace.cs
- MethodCallTranslator.cs
- AddInContractAttribute.cs
- DurableInstanceManager.cs
- EditingContext.cs
- Line.cs
- SqlProviderServices.cs