Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Data / System / Data / Filter / ExpressionNode.cs / 1 / ExpressionNode.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Collections.Generic; using System.Data.Common; using System.Data.SqlTypes; internal abstract class ExpressionNode { private DataTable _table; protected ExpressionNode(DataTable table) { _table = table; } internal IFormatProvider FormatProvider { get { return ((null != _table) ? _table.FormatProvider : System.Globalization.CultureInfo.CurrentCulture); } } internal virtual bool IsSqlColumn{ get{ return false; } } protected DataTable table { get { return _table; } } protected void BindTable(DataTable table) { // when the expression is created, DataColumn may not be associated with a table yet _table = table; } internal abstract void Bind(DataTable table, Listlist); internal abstract object Eval(); internal abstract object Eval(DataRow row, DataRowVersion version); internal abstract object Eval(int[] recordNos); internal abstract bool IsConstant(); internal abstract bool IsTableConstant(); internal abstract bool HasLocalAggregate(); internal abstract bool HasRemoteAggregate(); internal abstract ExpressionNode Optimize(); internal virtual bool DependsOn(DataColumn column) { return false; } internal static bool IsInteger(StorageType type) { return(type == StorageType.Int16 || type == StorageType.Int32 || type == StorageType.Int64 || type == StorageType.UInt16 || type == StorageType.UInt32 || type == StorageType.UInt64 || type == StorageType.SByte || type == StorageType.Byte); } internal static bool IsIntegerSql(StorageType type) { return(type == StorageType.Int16 || type == StorageType.Int32 || type == StorageType.Int64 || type == StorageType.UInt16 || type == StorageType.UInt32 || type == StorageType.UInt64 || type == StorageType.SByte || type == StorageType.Byte || type == StorageType.SqlInt64 || type == StorageType.SqlInt32 || type == StorageType.SqlInt16 || type == StorageType.SqlByte); } internal static bool IsSigned(StorageType type) { return(type == StorageType.Int16 || type == StorageType.Int32 || type == StorageType.Int64 || type == StorageType.SByte || IsFloat(type)); } internal static bool IsSignedSql(StorageType type) { return(type == StorageType.Int16 || // IsSigned(type) type == StorageType.Int32 || type == StorageType.Int64 || type == StorageType.SByte || type == StorageType.SqlInt64 || type == StorageType.SqlInt32 || type == StorageType.SqlInt16 || IsFloatSql(type)); } internal static bool IsUnsigned(StorageType type) { return(type == StorageType.UInt16 || type == StorageType.UInt32 || type == StorageType.UInt64 || type == StorageType.Byte); } internal static bool IsUnsignedSql(StorageType type) { return(type == StorageType.UInt16 || type == StorageType.UInt32 || type == StorageType.UInt64 || type == StorageType.SqlByte ||// SqlByte represents an 8-bit unsigned integer, in the range of 0 through 255, type == StorageType.Byte); } internal static bool IsNumeric(StorageType type) { return(IsFloat(type) || IsInteger(type)); } internal static bool IsNumericSql(StorageType type) { return(IsFloatSql(type) || IsIntegerSql(type)); } internal static bool IsFloat(StorageType type) { return(type == StorageType.Single || type == StorageType.Double || type == StorageType.Decimal); } internal static bool IsFloatSql(StorageType type) { return(type == StorageType.Single || type == StorageType.Double || type == StorageType.Decimal || type == StorageType.SqlDouble || type == StorageType.SqlDecimal || // I expect decimal to be Integer! type == StorageType.SqlMoney || // if decimal is here, this should be definitely here! type == StorageType.SqlSingle); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
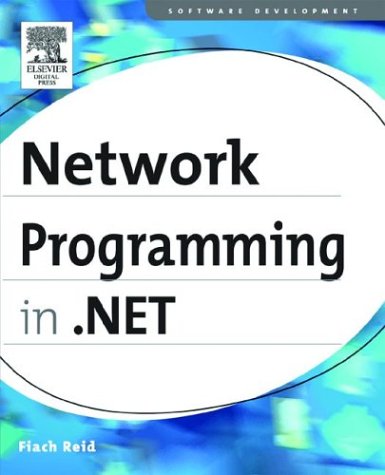
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLDateTime.cs
- MinimizableAttributeTypeConverter.cs
- IPipelineRuntime.cs
- DateTimeConverter.cs
- PathSegment.cs
- RunInstallerAttribute.cs
- ResourceContainerWrapper.cs
- GeneralTransformGroup.cs
- XmlArrayItemAttributes.cs
- HttpConfigurationSystem.cs
- EUCJPEncoding.cs
- SqlUserDefinedTypeAttribute.cs
- ComAwareEventInfo.cs
- BlurBitmapEffect.cs
- PathData.cs
- CodeGroup.cs
- GridViewDeletedEventArgs.cs
- ListBindingConverter.cs
- SqlMethodAttribute.cs
- ResourceReferenceExpression.cs
- SignerInfo.cs
- WebUtil.cs
- WindowsTooltip.cs
- ObjectPersistData.cs
- ThicknessAnimationBase.cs
- shaperfactory.cs
- IsolatedStorageFilePermission.cs
- LineInfo.cs
- ListViewContainer.cs
- LogConverter.cs
- FolderBrowserDialogDesigner.cs
- HtmlTableCellCollection.cs
- ChineseLunisolarCalendar.cs
- OperationCanceledException.cs
- SR.cs
- TextElement.cs
- Annotation.cs
- FormViewPageEventArgs.cs
- LineServices.cs
- Typeface.cs
- PersianCalendar.cs
- DropSource.cs
- ExistsInCollection.cs
- XMLSchema.cs
- TextPattern.cs
- RelationshipConstraintValidator.cs
- SimpleLine.cs
- ScriptRef.cs
- DBCSCodePageEncoding.cs
- ColumnMapProcessor.cs
- VirtualDirectoryMapping.cs
- XamlSerializerUtil.cs
- HtmlTableCell.cs
- Enum.cs
- DataGridColumn.cs
- SafeRightsManagementSessionHandle.cs
- TagNameToTypeMapper.cs
- SiteMapDataSourceView.cs
- FacetValueContainer.cs
- BitmapEffectState.cs
- XamlPathDataSerializer.cs
- ComponentEditorForm.cs
- SQLSingleStorage.cs
- Point3DAnimationBase.cs
- TrackingServices.cs
- DataPager.cs
- DataGridViewCellLinkedList.cs
- SerTrace.cs
- Transform.cs
- NonSerializedAttribute.cs
- BaseCollection.cs
- Debug.cs
- StringOutput.cs
- RuleConditionDialog.Designer.cs
- XamlClipboardData.cs
- DBProviderConfigurationHandler.cs
- Section.cs
- SmtpReplyReaderFactory.cs
- RadioButton.cs
- SafeCryptHandles.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- Types.cs
- GenericsNotImplementedException.cs
- TcpHostedTransportConfiguration.cs
- TabItemAutomationPeer.cs
- MarkupExtensionParser.cs
- HGlobalSafeHandle.cs
- XamlParser.cs
- NavigatorInput.cs
- IgnoreFlushAndCloseStream.cs
- PolicyChain.cs
- ListenerAdapterBase.cs
- DoubleAnimationUsingPath.cs
- CommandField.cs
- ValueOfAction.cs
- _ListenerRequestStream.cs
- HelpProvider.cs
- XmlAtomicValue.cs
- ResourceAttributes.cs
- MergeLocalizationDirectives.cs