Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / WebControls / ListControls / BaseDataListPage.cs / 1 / BaseDataListPage.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.WebControls.ListControls { using System.Design; using System; using System.CodeDom; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Data; using System.Drawing; using System.Web.UI.WebControls; using System.Windows.Forms; using System.Windows.Forms.Design; ////// /// The base class for all DataGrid and DataList component editor pages. /// ///[System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] internal abstract class BaseDataListPage : ComponentEditorPage { private bool dataGridMode; protected abstract string HelpKeyword { get; } protected bool IsDataGridMode { get { return dataGridMode; } } protected BaseDataList GetBaseControl() { IComponent selectedComponent = GetSelectedComponent(); Debug.Assert(selectedComponent is BaseDataList, "Unexpected component type for BaseDataListPage: " + selectedComponent.GetType().FullName); return (BaseDataList)selectedComponent; } protected BaseDataListDesigner GetBaseDesigner() { BaseDataListDesigner controlDesigner = null; IComponent selectedComponent = GetSelectedComponent(); ISite componentSite = selectedComponent.Site; Debug.Assert(componentSite != null, "Expected the component to be sited."); IDesignerHost designerHost = (IDesignerHost)componentSite.GetService(typeof(IDesignerHost)); Debug.Assert(designerHost != null, "Expected a designer host."); if (designerHost != null) { object designer = designerHost.GetDesigner(selectedComponent); Debug.Assert(designer != null, "Expected a designer for the selected component"); Debug.Assert(designer is BaseDataListDesigner, "Expected a designer that derives from BaseDataListDesigner"); controlDesigner = (BaseDataListDesigner)designer; } return controlDesigner; } /// /// /// Sets the component that is to be edited in the page. /// public override void SetComponent(IComponent component) { base.SetComponent(component); dataGridMode = (GetBaseControl() is System.Web.UI.WebControls.DataGrid); string rtlText = SR.GetString(SR.RTL); if (!String.Equals(rtlText, "RTL_False", StringComparison.Ordinal)) { RightToLeft = RightToLeft.Yes; } } ///public override void ShowHelp() { IComponent selectedComponent = GetSelectedComponent(); ISite componentSite = selectedComponent.Site; Debug.Assert(componentSite != null, "Expected the component to be sited."); IHelpService helpService = (IHelpService)componentSite.GetService(typeof(IHelpService)); if (helpService != null) { helpService.ShowHelpFromKeyword(HelpKeyword); } } /// public override bool SupportsHelp() { return true; } /// /// /// This contains information about a datasource and is used to populate /// the datasource combo. This is used in the General page for a DataList /// and the Data page for a DataGrid. /// protected class DataSourceItem { private IEnumerable runtimeDataSource; private string dataSourceName; private PropertyDescriptorCollection dataFields; public DataSourceItem(string dataSourceName, IEnumerable runtimeDataSource) { Debug.Assert(dataSourceName != null, "invalid name for datasource"); this.runtimeDataSource = runtimeDataSource; this.dataSourceName = dataSourceName; } public PropertyDescriptorCollection Fields { get { if (dataFields == null) { IEnumerable ds = RuntimeDataSource; if (ds != null) { dataFields = DesignTimeData.GetDataFields(ds); } } if (dataFields == null) { dataFields = new PropertyDescriptorCollection(null); } return dataFields; } } public virtual bool HasDataMembers { get { return false; } } public string Name { get { return dataSourceName; } } protected virtual object RuntimeComponent { get { return runtimeDataSource; } } protected virtual IEnumerable RuntimeDataSource { get { return runtimeDataSource; } } protected void ClearFields() { dataFields = null; } public override string ToString() { return this.Name; } } ////// /// protected class ListSourceDataSourceItem : DataSourceItem { private IListSource runtimeListSource; private string currentDataMember; public ListSourceDataSourceItem(string dataSourceName, IListSource runtimeListSource) : base(dataSourceName, null) { Debug.Assert(runtimeListSource != null); this.runtimeListSource = runtimeListSource; } public string CurrentDataMember { get { return currentDataMember; } set { currentDataMember = value; ClearFields(); } } public override bool HasDataMembers { get { return runtimeListSource.ContainsListCollection; } } protected override object RuntimeComponent { get { return runtimeListSource; } } protected override IEnumerable RuntimeDataSource { get { if (HasDataMembers) { return DesignTimeData.GetDataMember(runtimeListSource, currentDataMember); } else { return (IEnumerable)runtimeListSource.GetList(); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
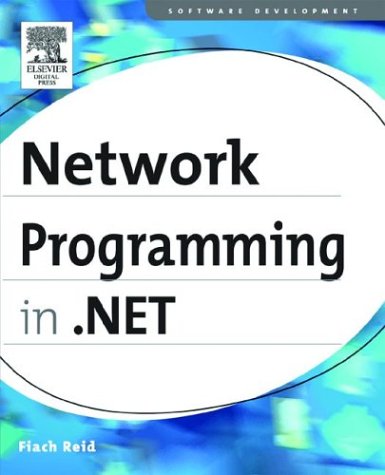
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbCommandDefinition.cs
- XsltException.cs
- FormsAuthenticationEventArgs.cs
- DataTableClearEvent.cs
- ContainerUIElement3D.cs
- XmlSchemaNotation.cs
- DesignerObject.cs
- XmlWriterTraceListener.cs
- ModelTreeEnumerator.cs
- WhitespaceRuleReader.cs
- DependencySource.cs
- SpeechRecognitionEngine.cs
- DeclarativeConditionsCollection.cs
- Executor.cs
- VirtualPathUtility.cs
- ContextProperty.cs
- ITreeGenerator.cs
- WebPartDisplayMode.cs
- _Win32.cs
- ValuePatternIdentifiers.cs
- MemberHolder.cs
- TimeSpanSecondsConverter.cs
- FontFamilyValueSerializer.cs
- DataGridViewHitTestInfo.cs
- XmlToDatasetMap.cs
- IdentifierService.cs
- MobileControl.cs
- basecomparevalidator.cs
- QueryOperator.cs
- DateBoldEvent.cs
- Hex.cs
- ConstructorArgumentAttribute.cs
- GridViewRowCollection.cs
- SlotInfo.cs
- PerfCounters.cs
- FormViewAutoFormat.cs
- DbXmlEnabledProviderManifest.cs
- ObjectDataSourceMethodEventArgs.cs
- recordstate.cs
- Compilation.cs
- SoapDocumentMethodAttribute.cs
- CollectionChangeEventArgs.cs
- ColumnMap.cs
- HtmlWindowCollection.cs
- QuaternionAnimationBase.cs
- ModelPerspective.cs
- StaticResourceExtension.cs
- StringKeyFrameCollection.cs
- ControlBuilderAttribute.cs
- XPathSingletonIterator.cs
- FragmentQueryProcessor.cs
- XmlSchemaExporter.cs
- StdValidatorsAndConverters.cs
- EventSinkHelperWriter.cs
- TreeViewItemAutomationPeer.cs
- RegexCharClass.cs
- ControlCollection.cs
- ObjectStorage.cs
- SessionParameter.cs
- SupportingTokenListenerFactory.cs
- ExtensionFile.cs
- TextContainerHelper.cs
- PTProvider.cs
- MethodToken.cs
- ContextBase.cs
- LoginView.cs
- MimeTypePropertyAttribute.cs
- XmlILAnnotation.cs
- Exceptions.cs
- FormViewPagerRow.cs
- ExeContext.cs
- CommandDevice.cs
- PtsContext.cs
- ObjectQueryExecutionPlan.cs
- CrossAppDomainChannel.cs
- FileChangeNotifier.cs
- Baml2006KnownTypes.cs
- ThaiBuddhistCalendar.cs
- AsyncStreamReader.cs
- PageTextBox.cs
- DesignOnlyAttribute.cs
- EventToken.cs
- ModelToObjectValueConverter.cs
- ServiceNotStartedException.cs
- FeatureAttribute.cs
- Point3D.cs
- TypeViewSchema.cs
- DataGridColumnCollection.cs
- BlurEffect.cs
- ConstructorBuilder.cs
- DbConnectionStringBuilder.cs
- PerformanceCounter.cs
- hresults.cs
- Stream.cs
- FixedSOMTextRun.cs
- WorkflowClientDeliverMessageWrapper.cs
- LinkedList.cs
- DataGridViewButtonColumn.cs
- DesignerWidgets.cs
- OdbcTransaction.cs