Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / AxParameterData.cs / 1 / AxParameterData.cs
namespace System.Windows.Forms.Design { using System.Diagnostics; using System; using System.Reflection; using Microsoft.Win32; using System.CodeDom; using System.Globalization; ////// /// ///[To be supplied.] ///public class AxParameterData { private string name; private string typeName; private Type type; private bool isByRef = false; private bool isOut = false; private bool isIn = false; private bool isOptional = false; private ParameterInfo paramInfo; /// /// /// public AxParameterData(string inname, string typeName) { Name = inname; this.typeName = typeName; } ///[To be supplied.] ////// /// public AxParameterData(string inname, Type type) { Name = inname; this.type = type; this.typeName = AxWrapperGen.MapTypeName(type); } ///[To be supplied.] ////// /// public AxParameterData(ParameterInfo info) : this(info, false) { } ///[To be supplied.] ////// /// public AxParameterData(ParameterInfo info, bool ignoreByRefs) { paramInfo = info; Name = info.Name; this.type = info.ParameterType; this.typeName = AxWrapperGen.MapTypeName(info.ParameterType); this.isByRef = info.ParameterType.IsByRef && !ignoreByRefs; this.isIn = info.IsIn && !ignoreByRefs; this.isOut = info.IsOut && !this.isIn && !ignoreByRefs; this.isOptional = info.IsOptional; } ///[To be supplied.] ////// /// public FieldDirection Direction { get { if (IsOut) return FieldDirection.Out; else if (IsByRef) return FieldDirection.Ref; else return FieldDirection.In; } } ///[To be supplied.] ////// /// public bool IsByRef { get { return isByRef; } } ///[To be supplied.] ////// /// public bool IsIn { get { return isIn; } } ///[To be supplied.] ////// /// public bool IsOut { get { return isOut; } } ///[To be supplied.] ////// /// public bool IsOptional { get { return isOptional; } } ///[To be supplied.] ////// /// public string Name { get { return name; } set { if (value == null) { name = null; } else { if (value != null && value.Length > 0 && Char.IsUpper(value[0])) { char[] chars = value.ToCharArray(); if (chars.Length > 0) chars[0] = Char.ToLower(chars[0], CultureInfo.InvariantCulture); name = new String(chars); } else { name = value; } } } } ///[To be supplied.] ////// /// public Type ParameterType { get { return type; } } // For types that are ref or out, we get a munged ByRef type name like "System.Int32&", // so we've got ot convert that into a type we recognize so we can compute default values, // and check if it's a primitive, etc. // internal static Type GetByRefBaseType(Type t) { if (t.IsByRef) { if (t.FullName.EndsWith("&")) { // strip the & and reload the type without it from the assembly that // is listed as owning it. // Type baseType = t.Assembly.GetType(t.FullName.Substring(0, t.FullName.Length - 1), false); if (baseType != null) { t = baseType; } } } return t; } internal ParameterInfo ParameterInfo { get { return paramInfo; } } internal Type ParameterBaseType { get { return GetByRefBaseType(ParameterType); } } ///[To be supplied.] ////// /// public string TypeName { get { if (typeName == null) { typeName = ParameterBaseType.FullName; } else if(typeName.EndsWith("&")) { typeName = typeName.TrimEnd(new char[] {'&'}); } return typeName; } } ///[To be supplied.] ////// /// public static AxParameterData[] Convert(ParameterInfo[] infos) { return AxParameterData.Convert(infos, false); } ///[To be supplied.] ////// /// public static AxParameterData[] Convert(ParameterInfo[] infos, bool ignoreByRefs) { if (infos == null) return new AxParameterData[0]; int noname = 0; AxParameterData[] parameters = new AxParameterData[infos.Length]; for (int i = 0; i < infos.Length; ++i) { parameters[i] = new AxParameterData(infos[i], ignoreByRefs); if (parameters[i].Name == null || parameters[i].Name == "") { parameters[i].Name = "param" + (noname++); } } return parameters; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
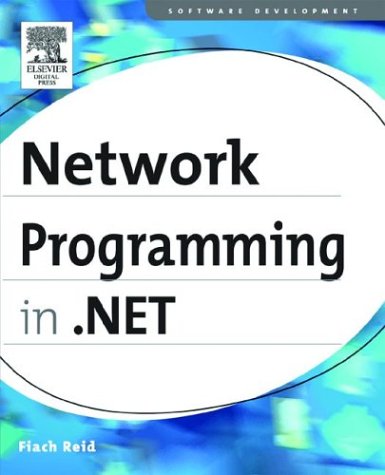
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VerificationAttribute.cs
- ByteArrayHelperWithString.cs
- ThreadStartException.cs
- GenericTextProperties.cs
- TypeInformation.cs
- RectConverter.cs
- DataConnectionHelper.cs
- Config.cs
- Transform3DGroup.cs
- Filter.cs
- recordstate.cs
- MasterPage.cs
- XPathScanner.cs
- DataTableMappingCollection.cs
- TextTrailingWordEllipsis.cs
- DeriveBytes.cs
- SQLSingleStorage.cs
- FtpRequestCacheValidator.cs
- ArgumentNullException.cs
- PrintDialogException.cs
- ListViewInsertEventArgs.cs
- XmlProcessingInstruction.cs
- UserValidatedEventArgs.cs
- WindowsListView.cs
- ToolStripContainer.cs
- RotateTransform.cs
- FontStyleConverter.cs
- UInt64Converter.cs
- HttpHostedTransportConfiguration.cs
- SQLDecimalStorage.cs
- MessageSecurityOverMsmqElement.cs
- RijndaelManaged.cs
- ReadOnlyHierarchicalDataSourceView.cs
- DataGridViewTopRowAccessibleObject.cs
- Size3DValueSerializer.cs
- EventWaitHandle.cs
- sqlpipe.cs
- SendActivityDesigner.cs
- AsymmetricKeyExchangeDeformatter.cs
- IxmlLineInfo.cs
- ThreadExceptionEvent.cs
- RegexParser.cs
- PropertySourceInfo.cs
- MDIWindowDialog.cs
- Mapping.cs
- CheckBoxField.cs
- ObjectPersistData.cs
- LayoutInformation.cs
- MemberPathMap.cs
- SignatureDescription.cs
- XmlSchemaSimpleType.cs
- PageContent.cs
- ContentPlaceHolder.cs
- _PooledStream.cs
- OleDbPropertySetGuid.cs
- BooleanFunctions.cs
- CodeCommentStatement.cs
- ChainedAsyncResult.cs
- FileChangesMonitor.cs
- ConditionValidator.cs
- MenuItemBindingCollection.cs
- WebPartCollection.cs
- ProxyManager.cs
- XmlSchemaAny.cs
- Internal.cs
- InkCanvasFeedbackAdorner.cs
- elementinformation.cs
- TextTreeTextBlock.cs
- VectorAnimationUsingKeyFrames.cs
- StrokeNodeOperations.cs
- DecimalKeyFrameCollection.cs
- EncoderFallback.cs
- TemplateControlParser.cs
- WebPartsPersonalization.cs
- ConfigurationException.cs
- NullableBoolConverter.cs
- ThreadBehavior.cs
- GroupBoxAutomationPeer.cs
- SoapProtocolReflector.cs
- CacheForPrimitiveTypes.cs
- DockPatternIdentifiers.cs
- IdentitySection.cs
- WebPartZone.cs
- DataServiceRequestOfT.cs
- TypeRefElement.cs
- DataObjectPastingEventArgs.cs
- UrlMappingsModule.cs
- NameValueCollection.cs
- AnnotationResourceCollection.cs
- RegistryKey.cs
- ISFClipboardData.cs
- SerializationException.cs
- WeakEventManager.cs
- TextEditorSelection.cs
- QilIterator.cs
- LockCookie.cs
- EncoderReplacementFallback.cs
- EmbeddedMailObjectsCollection.cs
- rsa.cs
- UserControl.cs