Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ImageIndexEditor.cs / 1 / ImageIndexEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Design.ImageIndexEditor..ctor()")] namespace System.Windows.Forms.Design { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.Windows.Forms; using System.Drawing; using System.Drawing.Design; using System.ComponentModel.Design; using Microsoft.Win32; using System.Windows.Forms.Design; using System.Windows.Forms.ComponentModel; ////// /// internal class ImageIndexEditor : UITypeEditor { protected ImageList currentImageList; protected PropertyDescriptor currentImageListProp; protected object currentInstance; protected UITypeEditor imageEditor; protected string parentImageListProperty = "Parent"; protected string imageListPropertyName = null; ///Provides an editor for visually picking an image index. ////// /// public ImageIndexEditor() { // Get the type editor for images. We use the properties on // this to determine if we support value painting, etc. // imageEditor = (UITypeEditor)TypeDescriptor.GetEditor(typeof(Image), typeof(UITypeEditor)); } internal UITypeEditor ImageEditor { get { return imageEditor; } } internal string ParentImageListProperty { get { return parentImageListProperty; } } ///Initializes a new instance of the ///class. /// /// Retrieves an image for the current context at current index. /// protected virtual Image GetImage(ITypeDescriptorContext context, int index, string key, bool useIntIndex) { Image image = null; object instance = context.Instance; if(instance is object[]) { // we would not know what to do in this case anyway (i.e. multiple selection of objects) return null; } // If the instances are different, then we need to re-aquire our image list. // if ((index >= 0) || (key != null)) { if (currentImageList == null || instance != currentInstance || (currentImageListProp != null && (ImageList)currentImageListProp.GetValue(currentInstance) != currentImageList)) { currentInstance = instance; // first look for an attribute PropertyDescriptor imageListProp = ImageListUtils.GetImageListProperty(context.PropertyDescriptor, ref instance); // not found as an attribute, do the old behavior while(instance != null && imageListProp == null) { PropertyDescriptorCollection props = TypeDescriptor.GetProperties(instance); foreach (PropertyDescriptor prop in props) { if (typeof(ImageList).IsAssignableFrom(prop.PropertyType)) { imageListProp = prop; break; } } if (imageListProp == null) { // We didn't find the image list in this component. See if the // component has a "parent" property. If so, walk the tree... // PropertyDescriptor parentProp = props[ParentImageListProperty]; if (parentProp != null) { instance = parentProp.GetValue(instance); } else { // Stick a fork in us, we're done. // instance = null; } } } if (imageListProp != null) { currentImageList = (ImageList)imageListProp.GetValue(instance); currentImageListProp = imageListProp; currentInstance = instance; } } if (currentImageList != null) { if (useIntIndex) { if (currentImageList != null && index < currentImageList.Images.Count) { index = (index > 0) ? index : 0; image = currentImageList.Images[index]; } } else { image = currentImageList.Images[key]; } } else { // no image list, no image image = null; } } return image; } ////// /// public override bool GetPaintValueSupported(ITypeDescriptorContext context) { if (imageEditor != null) { return imageEditor.GetPaintValueSupported(context); } return false; } ///Gets a value indicating whether this editor supports the painting of a representation /// of an object's value. ////// /// public override void PaintValue(PaintValueEventArgs e) { if (ImageEditor != null){ Image image = null; if (e.Value is int) { image = GetImage(e.Context, (int)e.Value, null, true); } else if (e.Value is string) { image = GetImage(e.Context, -1, (string)e.Value, false); } if (image != null) { ImageEditor.PaintValue(new PaintValueEventArgs(e.Context, image, e.Graphics, e.Bounds)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Paints a representative value of the given object to the provided /// canvas. Painting should be done within the boundaries of the /// provided rectangle. /// ///
Link Menu
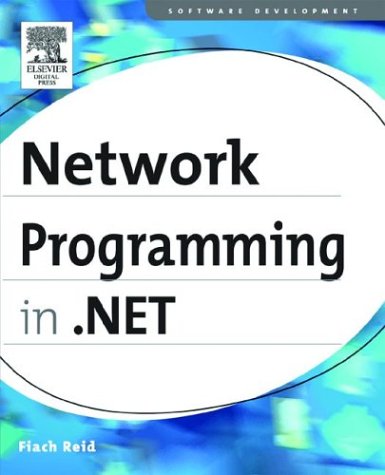
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThreadAbortException.cs
- DbConnectionPoolCounters.cs
- ProcessHostMapPath.cs
- WebServicesSection.cs
- MethodToken.cs
- RIPEMD160.cs
- CodeDirectiveCollection.cs
- NotifyParentPropertyAttribute.cs
- CatalogPartChrome.cs
- XPathItem.cs
- CollectionViewGroup.cs
- DoubleCollection.cs
- SHA1CryptoServiceProvider.cs
- Drawing.cs
- SafeWaitHandle.cs
- SafeTokenHandle.cs
- ScalarOps.cs
- PreProcessInputEventArgs.cs
- StaticTextPointer.cs
- FormViewAutoFormat.cs
- SynchronizedInputAdaptor.cs
- DoubleAnimationUsingKeyFrames.cs
- HttpModulesSection.cs
- BaseTemplateParser.cs
- AutoResetEvent.cs
- AdornerLayer.cs
- ServiceObjectContainer.cs
- NullableLongAverageAggregationOperator.cs
- ActivityCodeDomSerializer.cs
- ListViewItemSelectionChangedEvent.cs
- SamlDelegatingWriter.cs
- DependencyPropertyHelper.cs
- DesignerSerializationOptionsAttribute.cs
- WorkflowOperationBehavior.cs
- FormsAuthenticationUserCollection.cs
- COM2ICategorizePropertiesHandler.cs
- BaseValidatorDesigner.cs
- GridViewRowCollection.cs
- Dispatcher.cs
- OrthographicCamera.cs
- SqlFacetAttribute.cs
- TokenBasedSet.cs
- AssemblyResourceLoader.cs
- XmlAttributeAttribute.cs
- ColorTranslator.cs
- SchemaComplexType.cs
- RadioButton.cs
- UniqueSet.cs
- ToolStripControlHost.cs
- RoutedEventArgs.cs
- ButtonStandardAdapter.cs
- FactoryGenerator.cs
- XmlWellformedWriter.cs
- __ConsoleStream.cs
- ToolBarTray.cs
- ServicePointManager.cs
- cryptoapiTransform.cs
- RevocationPoint.cs
- ItemsPanelTemplate.cs
- HostSecurityManager.cs
- SystemIPGlobalProperties.cs
- WindowsRichEdit.cs
- HwndSourceKeyboardInputSite.cs
- ApplicationHost.cs
- XmlSchemaSet.cs
- SmtpMail.cs
- AddressHeader.cs
- IndexedEnumerable.cs
- DataGridViewColumnEventArgs.cs
- SmiMetaDataProperty.cs
- SecurityUtils.cs
- FieldToken.cs
- EntityContainer.cs
- LateBoundChannelParameterCollection.cs
- ManagedCodeMarkers.cs
- SchemaTypeEmitter.cs
- NetMsmqSecurity.cs
- TextBoxBaseDesigner.cs
- ServicesUtilities.cs
- BinaryParser.cs
- ScriptMethodAttribute.cs
- WebBrowsableAttribute.cs
- XmlEnumAttribute.cs
- RegisteredDisposeScript.cs
- XmlAttributeHolder.cs
- ToolStripSplitButton.cs
- ErrorTableItemStyle.cs
- XmlDataDocument.cs
- D3DImage.cs
- CommonObjectSecurity.cs
- Relationship.cs
- ProxyHelper.cs
- CellTreeNodeVisitors.cs
- QueryOperatorEnumerator.cs
- Resources.Designer.cs
- Utils.cs
- TdsParserStateObject.cs
- RoleManagerEventArgs.cs
- DbConnectionFactory.cs
- ImageCodecInfoPrivate.cs