Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / FlatButtonAppearance.cs / 1 / FlatButtonAppearance.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Globalization; using System.ComponentModel; using System.Windows.Forms.Layout; ////// /// [TypeConverter(typeof(FlatButtonAppearanceConverter))] public class FlatButtonAppearance { private ButtonBase owner; private int borderSize = 1; private Color borderColor = Color.Empty; private Color checkedBackColor = Color.Empty; private Color mouseDownBackColor = Color.Empty; private Color mouseOverBackColor = Color.Empty; internal FlatButtonAppearance(ButtonBase owner) { this.owner = owner; } ////// /// For buttons whose FlatStyle is FlatStyle.Flat, this property specifies the size, in pixels of the border around the button. /// [ Browsable(true), ApplicableToButton(), NotifyParentProperty(true), SRCategory(SR.CatAppearance), SRDescription(SR.ButtonBorderSizeDescr), EditorBrowsable(EditorBrowsableState.Always), DefaultValue(1), ] public int BorderSize { get { return borderSize; } set { if (value < 0) throw new ArgumentOutOfRangeException("BorderSize", value, SR.GetString(SR.InvalidLowBoundArgumentEx, "BorderSize", value.ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); if (borderSize != value) { borderSize = value; if (owner != null && owner.ParentInternal != null) { LayoutTransaction.DoLayoutIf(owner.AutoSize, owner.ParentInternal, owner, PropertyNames.FlatAppearanceBorderSize); } owner.Invalidate(); } } } ////// /// For buttons whose FlatStyle is FlatStyle.Flat, this property specifies the color of the border around the button. /// [ Browsable(true), ApplicableToButton(), NotifyParentProperty(true), SRCategory(SR.CatAppearance), SRDescription(SR.ButtonBorderColorDescr), EditorBrowsable(EditorBrowsableState.Always), DefaultValue(typeof(Color), ""), ] public Color BorderColor { get { return borderColor; } set { if (value.Equals(Color.Transparent)) { throw new NotSupportedException(SR.GetString(SR.ButtonFlatAppearanceInvalidBorderColor)); } if (borderColor != value) { borderColor = value; owner.Invalidate(); } } } ////// /// For buttons whose FlatStyle is FlatStyle.Flat, this property specifies the color of the client area /// of the button when the button state is checked and the mouse cursor is NOT within the bounds of the control. /// [ Browsable(true), NotifyParentProperty(true), SRCategory(SR.CatAppearance), SRDescription(SR.ButtonCheckedBackColorDescr), EditorBrowsable(EditorBrowsableState.Always), DefaultValue(typeof(Color), ""), ] public Color CheckedBackColor { get { return checkedBackColor; } set { if (checkedBackColor != value) { checkedBackColor = value; owner.Invalidate(); } } } ////// /// For buttons whose FlatStyle is FlatStyle.Flat, this property specifies the color of the client area /// of the button when the mouse cursor is within the bounds of the control and the left button is pressed. /// [ Browsable(true), ApplicableToButton(), NotifyParentProperty(true), SRCategory(SR.CatAppearance), SRDescription(SR.ButtonMouseDownBackColorDescr), EditorBrowsable(EditorBrowsableState.Always), DefaultValue(typeof(Color), ""), ] public Color MouseDownBackColor { get { return mouseDownBackColor; } set { if (mouseDownBackColor != value) { mouseDownBackColor = value; owner.Invalidate(); } } } ////// /// For buttons whose FlatStyle is FlatStyle.Flat, this property specifies the color of the client /// area of the button when the mouse cursor is within the bounds of the control. /// [ Browsable(true), ApplicableToButton(), NotifyParentProperty(true), SRCategory(SR.CatAppearance), SRDescription(SR.ButtonMouseOverBackColorDescr), EditorBrowsable(EditorBrowsableState.Always), DefaultValue(typeof(Color), ""), ] public Color MouseOverBackColor { get { return mouseOverBackColor; } set { if (mouseOverBackColor != value) { mouseOverBackColor = value; owner.Invalidate(); } } } } internal sealed class ApplicableToButtonAttribute : Attribute { public ApplicableToButtonAttribute() { } } internal class FlatButtonAppearanceConverter : ExpandableObjectConverter { // Don't let the property grid display the full type name in the value cell public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(string)) { return ""; } return base.ConvertTo(context, culture, value, destinationType); } // Don't let the property grid display the CheckedBackColor property for Button controls public override PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object value, Attribute[] attributes) { if (context != null && context.Instance is Button) { Attribute[] attributes2 = new Attribute[attributes.Length + 1]; attributes.CopyTo(attributes2, 0); attributes2[attributes.Length] = new ApplicableToButtonAttribute(); attributes = attributes2; } return TypeDescriptor.GetProperties(value, attributes); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
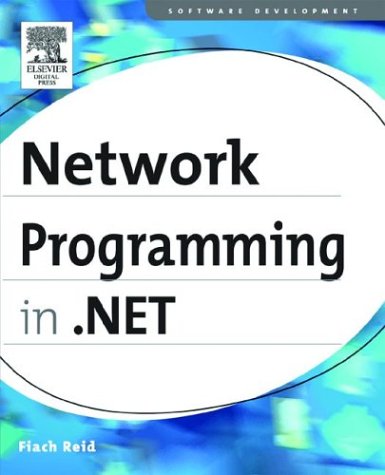
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TimelineClockCollection.cs
- CellTreeNodeVisitors.cs
- ColumnClickEvent.cs
- GlyphRun.cs
- AVElementHelper.cs
- MinMaxParagraphWidth.cs
- Parser.cs
- PageBuildProvider.cs
- EntityDataSourceValidationException.cs
- SqlClientWrapperSmiStreamChars.cs
- DataGridViewComboBoxCell.cs
- MessageBox.cs
- MenuCommand.cs
- PathSegmentCollection.cs
- autovalidator.cs
- CompositeDataBoundControl.cs
- SplayTreeNode.cs
- ChannelProtectionRequirements.cs
- XmlCharType.cs
- unsafeIndexingFilterStream.cs
- OleDbException.cs
- LocalizedNameDescriptionPair.cs
- Renderer.cs
- ItemAutomationPeer.cs
- StoreAnnotationsMap.cs
- BindableTemplateBuilder.cs
- RadioButtonRenderer.cs
- TraceSection.cs
- DocobjHost.cs
- Logging.cs
- RegexMatchCollection.cs
- ConnectionProviderAttribute.cs
- BindToObject.cs
- TransformValueSerializer.cs
- SafeBitVector32.cs
- httpapplicationstate.cs
- XmlEntity.cs
- ClickablePoint.cs
- ReferentialConstraintRoleElement.cs
- MergeFilterQuery.cs
- XdrBuilder.cs
- ScaleTransform3D.cs
- Processor.cs
- WebPartDisplayModeCollection.cs
- SafeRightsManagementHandle.cs
- StylusPointPropertyUnit.cs
- ConstNode.cs
- Calendar.cs
- TextElementEditingBehaviorAttribute.cs
- UnmanagedMemoryStream.cs
- TreeNodeClickEventArgs.cs
- UserPreferenceChangingEventArgs.cs
- InfoCardTrace.cs
- X509Utils.cs
- SimpleWebHandlerParser.cs
- TransformDescriptor.cs
- CodeStatement.cs
- FileLoadException.cs
- ErrorStyle.cs
- CollectionBase.cs
- SiteMembershipCondition.cs
- Events.cs
- EncoderBestFitFallback.cs
- ToolZone.cs
- DropShadowBitmapEffect.cs
- EntityDataSourceWrapper.cs
- AsyncParams.cs
- BindingCompleteEventArgs.cs
- Selection.cs
- DefaultHttpHandler.cs
- SmiContextFactory.cs
- EntityCollection.cs
- PersianCalendar.cs
- UidManager.cs
- KeyedCollection.cs
- SafeNativeMethods.cs
- DeclarativeCatalogPartDesigner.cs
- OneWayElement.cs
- Vector3dCollection.cs
- CallbackBehaviorAttribute.cs
- MatrixStack.cs
- Size3D.cs
- UInt64Converter.cs
- RoleManagerEventArgs.cs
- ReflectionTypeLoadException.cs
- ReliableMessagingVersion.cs
- ColorBuilder.cs
- EntityParameter.cs
- TimeManager.cs
- ToolBar.cs
- SamlAuthorizationDecisionClaimResource.cs
- UriExt.cs
- ModuleBuilderData.cs
- HopperCache.cs
- MultiDataTrigger.cs
- InvokeBinder.cs
- ContentOperations.cs
- COAUTHINFO.cs
- WindowsScroll.cs
- RemoteCryptoTokenProvider.cs