Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Xml / System / Xml / XPath / Internal / FunctionQuery.cs / 1 / FunctionQuery.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.Xsl; using System.Xml.XPath; using System.Diagnostics; using System.Globalization; using System.Collections.Generic; internal sealed class FunctionQuery : ExtensionQuery { private IListargs; private IXsltContextFunction function; public FunctionQuery(string prefix, string name, List args) : base(prefix, name) { this.args = args; } private FunctionQuery(FunctionQuery other) : base(other) { this.function = other.function; Query[] tmp = new Query[other.args.Count]; { for (int i = 0; i < tmp.Length; i ++) { tmp[i] = Clone(other.args[i]); } args = tmp; } this.args = tmp; } public override void SetXsltContext(XsltContext context) { if (context == null) { throw XPathException.Create(Res.Xp_NoContext); } if (this.xsltContext != context) { xsltContext = context; foreach (Query argument in args) { argument.SetXsltContext(context); } XPathResultType[] argTypes = new XPathResultType[args.Count]; for(int i = 0; i < args.Count; i ++) { argTypes[i] = args[i].StaticType; } function = xsltContext.ResolveFunction(prefix, name, argTypes); // KB article allows to return null, see http://support.microsoft.com/?kbid=324462#6 if (function == null) { throw XPathException.Create(Res.Xp_UndefFunc, QName); } } } public override object Evaluate(XPathNodeIterator nodeIterator) { if (xsltContext == null) { throw XPathException.Create(Res.Xp_NoContext); } // calculate arguments: object[] argVals = new object[args.Count]; for (int i = 0; i < args.Count; i ++) { argVals[i] = args[i].Evaluate(nodeIterator); if (argVals[i] is XPathNodeIterator) {// ForBack Compat. To protect our queries from users. bug#372077 & 20006123 argVals[i] = new XPathSelectionIterator(nodeIterator.Current, args[i]); } } try { return ProcessResult(function.Invoke(xsltContext, argVals, nodeIterator.Current)); } catch(Exception ex) { throw XPathException.Create(Res.Xp_FunctionFailed, QName, ex); } } public override XPathNavigator MatchNode(XPathNavigator navigator) { if (name != "key" && prefix.Length != 0) { throw XPathException.Create(Res.Xp_InvalidPattern); } this.Evaluate(new XPathSingletonIterator(navigator, /*moved:*/true)); XPathNavigator nav = null; while ((nav = this.Advance()) != null) { if (nav.IsSamePosition(navigator)) { return nav; } } return nav; } public override XPathResultType StaticType { get { XPathResultType result = function != null ? function.ReturnType : XPathResultType.Any; if (result == XPathResultType.Error) { // In v.1 we confused Error & Any so now for backward compatibility we should allow users to return any of them. result = XPathResultType.Any; } return result; } } public override XPathNodeIterator Clone() { return new FunctionQuery(this); } public override void PrintQuery(XmlWriter w) { w.WriteStartElement(this.GetType().Name); w.WriteAttributeString("name", prefix.Length != 0 ? prefix + ':' + name : name); foreach(Query arg in this.args) { arg.PrintQuery(w); } w.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
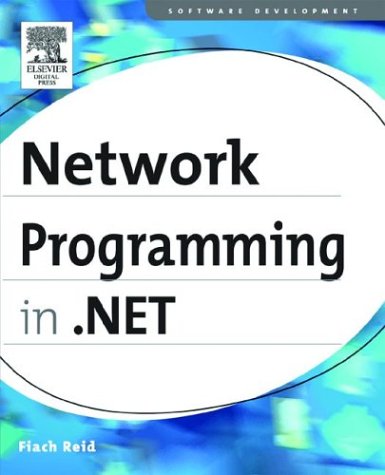
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _NegoState.cs
- ClientScriptManagerWrapper.cs
- CodeAttachEventStatement.cs
- NativeRightsManagementAPIsStructures.cs
- DebuggerAttributes.cs
- QilScopedVisitor.cs
- MenuItemStyle.cs
- TypeReference.cs
- WebBaseEventKeyComparer.cs
- Transactions.cs
- SmiContextFactory.cs
- Dump.cs
- DataGridViewCheckBoxColumn.cs
- NullableLongAverageAggregationOperator.cs
- WsatServiceAddress.cs
- While.cs
- WindowCollection.cs
- HwndSourceKeyboardInputSite.cs
- RootDesignerSerializerAttribute.cs
- Transaction.cs
- BaseCollection.cs
- ContractAdapter.cs
- ContextQuery.cs
- TagMapCollection.cs
- Label.cs
- Tracking.cs
- CreateSequence.cs
- WasHostedComPlusFactory.cs
- XXXInfos.cs
- ForeignKeyConstraint.cs
- RadioButtonList.cs
- SecurityUtils.cs
- _RequestCacheProtocol.cs
- SqlInternalConnection.cs
- Parameter.cs
- InitializerFacet.cs
- WindowsIdentity.cs
- LocalizableResourceBuilder.cs
- RegionInfo.cs
- HttpCacheVary.cs
- PreviewPageInfo.cs
- ProxyFragment.cs
- SearchForVirtualItemEventArgs.cs
- HTTPNotFoundHandler.cs
- X509CertificateStore.cs
- TranslateTransform3D.cs
- ColumnCollection.cs
- DesignerTextBoxAdapter.cs
- BamlVersionHeader.cs
- TextEditorMouse.cs
- CompModSwitches.cs
- FocusManager.cs
- DbProviderConfigurationHandler.cs
- ValidationVisibilityAttribute.cs
- FakeModelItemImpl.cs
- HttpsHostedTransportConfiguration.cs
- EntitySqlQueryState.cs
- TemplatedMailWebEventProvider.cs
- PackageRelationshipCollection.cs
- CompilerCollection.cs
- DataGridViewRowPostPaintEventArgs.cs
- XmlSchemaSubstitutionGroup.cs
- ValidatedControlConverter.cs
- TPLETWProvider.cs
- ObjectQuery.cs
- VariantWrapper.cs
- WindowsRebar.cs
- MorphHelper.cs
- TCPClient.cs
- Normalization.cs
- NullRuntimeConfig.cs
- HttpRequestContext.cs
- SmtpAuthenticationManager.cs
- MenuCommand.cs
- XomlSerializationHelpers.cs
- _SslStream.cs
- ISAPIWorkerRequest.cs
- BaseCodeDomTreeGenerator.cs
- CompilerTypeWithParams.cs
- MemberAssignmentAnalysis.cs
- OptimalBreakSession.cs
- GridItem.cs
- ImageMap.cs
- CopyOnWriteList.cs
- ObjectDataSourceSelectingEventArgs.cs
- FontStyleConverter.cs
- NativeObjectSecurity.cs
- AdornerHitTestResult.cs
- WorkflowTransactionService.cs
- NetworkStream.cs
- EngineSite.cs
- InputProviderSite.cs
- BypassElement.cs
- TextTrailingWordEllipsis.cs
- Delegate.cs
- Pair.cs
- HttpModulesSection.cs
- NativeMethodsOther.cs
- XmlAnyAttributeAttribute.cs
- XPathNodeInfoAtom.cs