Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / VerticalAlignConverter.cs / 1 / VerticalAlignConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- // namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Globalization; internal class VerticalAlignConverter : EnumConverter { static string[] stringValues = new String[(int) VerticalAlign.Bottom + 1]; static VerticalAlignConverter () { stringValues[(int) VerticalAlign.NotSet] = "NotSet"; stringValues[(int) VerticalAlign.Top] = "Top"; stringValues[(int) VerticalAlign.Middle] = "Middle"; stringValues[(int) VerticalAlign.Bottom] = "Bottom"; } // this constructor needs to be public despite the fact that it's in an internal // class so it can be created by Activator.CreateInstance. public VerticalAlignConverter () : base(typeof(VerticalAlign)) {} public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } else { return base.CanConvertFrom(context, sourceType); } } public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) return null; if (value is string) { string textValue = ((string)value).Trim(); if (textValue.Length == 0) return VerticalAlign.NotSet; switch (textValue) { case "NotSet": return VerticalAlign.NotSet; case "Top": return VerticalAlign.Top; case "Middle": return VerticalAlign.Middle; case "Bottom": return VerticalAlign.Bottom; } } return base.ConvertFrom(context, culture, value); } public override bool CanConvertTo(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertTo(context, sourceType); } public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(string) && ((int) value <= (int)VerticalAlign.Bottom)) { return stringValues[(int) value]; } return base.ConvertTo(context, culture, value, destinationType); } } }
Link Menu
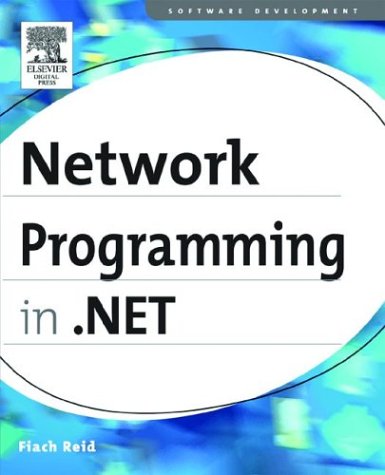
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SyndicationSerializer.cs
- NonParentingControl.cs
- DelegateOutArgument.cs
- ValuePattern.cs
- Help.cs
- DataGridViewColumnCollectionEditor.cs
- mda.cs
- Speller.cs
- HiddenField.cs
- DefaultAsyncDataDispatcher.cs
- ComPlusTypeLoader.cs
- CustomCategoryAttribute.cs
- DefaultEventAttribute.cs
- DispatcherHookEventArgs.cs
- QilPatternVisitor.cs
- ServerReliableChannelBinder.cs
- DashStyles.cs
- RotateTransform3D.cs
- ApplicationFileCodeDomTreeGenerator.cs
- LineGeometry.cs
- XmlName.cs
- MsmqIntegrationElement.cs
- ObjectDataSourceEventArgs.cs
- SafeBuffer.cs
- DataControlButton.cs
- JobStaple.cs
- TextRenderer.cs
- BufferModesCollection.cs
- StyleModeStack.cs
- SettingsAttributeDictionary.cs
- AsymmetricSecurityProtocolFactory.cs
- EmptyImpersonationContext.cs
- GridViewSortEventArgs.cs
- PingOptions.cs
- TabItemAutomationPeer.cs
- ResolveMatchesCD1.cs
- DiscoveryEndpointElement.cs
- DefaultExpression.cs
- TransformCollection.cs
- ComboBox.cs
- MtomMessageEncodingBindingElement.cs
- PeerNameResolver.cs
- PackUriHelper.cs
- VisualStyleElement.cs
- XmlSchemaCollection.cs
- ToolTipAutomationPeer.cs
- validationstate.cs
- KernelTypeValidation.cs
- ColumnBinding.cs
- XslNumber.cs
- AppSettingsSection.cs
- TransformerTypeCollection.cs
- AccessDataSourceDesigner.cs
- Table.cs
- DataGridViewCell.cs
- ListControl.cs
- UriTemplateHelpers.cs
- RegexParser.cs
- SaveLedgerEntryRequest.cs
- IsolatedStorageFile.cs
- PrintDialog.cs
- DoWorkEventArgs.cs
- TimeIntervalCollection.cs
- X509IssuerSerialKeyIdentifierClause.cs
- SqlAliaser.cs
- ManifestResourceInfo.cs
- MetadataItemCollectionFactory.cs
- PropertyIDSet.cs
- DefaultValueTypeConverter.cs
- DPTypeDescriptorContext.cs
- HotCommands.cs
- ComplexObject.cs
- DefaultPrintController.cs
- DataError.cs
- ZipFileInfo.cs
- XPathPatternBuilder.cs
- IItemContainerGenerator.cs
- ObjectDisposedException.cs
- ObjectListFieldsPage.cs
- BevelBitmapEffect.cs
- FixedDocumentPaginator.cs
- FigureParaClient.cs
- RelationshipEndMember.cs
- TableChangeProcessor.cs
- CheckBox.cs
- WinEventTracker.cs
- ArgumentOutOfRangeException.cs
- XmlValueConverter.cs
- IdleTimeoutMonitor.cs
- RemotingException.cs
- UdpConstants.cs
- CombinedHttpChannel.cs
- CodeIterationStatement.cs
- PropertyNames.cs
- Matrix3DValueSerializer.cs
- SchemaRegistration.cs
- Variant.cs
- XPathAncestorIterator.cs
- HttpListenerRequest.cs
- FixedSOMContainer.cs