Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / Util / SymbolEqualComparer.cs / 1 / SymbolEqualComparer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Util { using System.Collections; using System.Globalization; ////// internal class SymbolEqualComparer: IComparer { ////// For internal use only. This implements a comparison that only /// checks for equalilty, so this should only be used in un-sorted data /// structures like Hastable and ListDictionary. This is a little faster /// than using CaseInsensitiveComparer because it does a strict character by /// character equality chech rather than a sorted comparison. /// ////// internal static readonly IComparer Default = new SymbolEqualComparer(); internal SymbolEqualComparer() { } int IComparer.Compare(object keyLeft, object keyRight) { string sLeft = keyLeft as string; string sRight = keyRight as string; if (sLeft == null) { throw new ArgumentNullException("keyLeft"); } if (sRight == null) { throw new ArgumentNullException("keyRight"); } int lLeft = sLeft.Length; int lRight = sRight.Length; if (lLeft != lRight) { return 1; } for (int i = 0; i < lLeft; i++) { char charLeft = sLeft[i]; char charRight = sRight[i]; if (charLeft == charRight) { continue; } UnicodeCategory catLeft = Char.GetUnicodeCategory(charLeft); UnicodeCategory catRight = Char.GetUnicodeCategory(charRight); if (catLeft == UnicodeCategory.UppercaseLetter && catRight == UnicodeCategory.LowercaseLetter) { if (Char.ToLower(charLeft, CultureInfo.InvariantCulture) == charRight) { continue; } } else if (catRight == UnicodeCategory.UppercaseLetter && catLeft == UnicodeCategory.LowercaseLetter){ if (Char.ToLower(charRight, CultureInfo.InvariantCulture) == charLeft) { continue; } } return 1; } return 0; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Util { using System.Collections; using System.Globalization; ////// internal class SymbolEqualComparer: IComparer { ////// For internal use only. This implements a comparison that only /// checks for equalilty, so this should only be used in un-sorted data /// structures like Hastable and ListDictionary. This is a little faster /// than using CaseInsensitiveComparer because it does a strict character by /// character equality chech rather than a sorted comparison. /// ////// internal static readonly IComparer Default = new SymbolEqualComparer(); internal SymbolEqualComparer() { } int IComparer.Compare(object keyLeft, object keyRight) { string sLeft = keyLeft as string; string sRight = keyRight as string; if (sLeft == null) { throw new ArgumentNullException("keyLeft"); } if (sRight == null) { throw new ArgumentNullException("keyRight"); } int lLeft = sLeft.Length; int lRight = sRight.Length; if (lLeft != lRight) { return 1; } for (int i = 0; i < lLeft; i++) { char charLeft = sLeft[i]; char charRight = sRight[i]; if (charLeft == charRight) { continue; } UnicodeCategory catLeft = Char.GetUnicodeCategory(charLeft); UnicodeCategory catRight = Char.GetUnicodeCategory(charRight); if (catLeft == UnicodeCategory.UppercaseLetter && catRight == UnicodeCategory.LowercaseLetter) { if (Char.ToLower(charLeft, CultureInfo.InvariantCulture) == charRight) { continue; } } else if (catRight == UnicodeCategory.UppercaseLetter && catLeft == UnicodeCategory.LowercaseLetter){ if (Char.ToLower(charRight, CultureInfo.InvariantCulture) == charLeft) { continue; } } return 1; } return 0; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
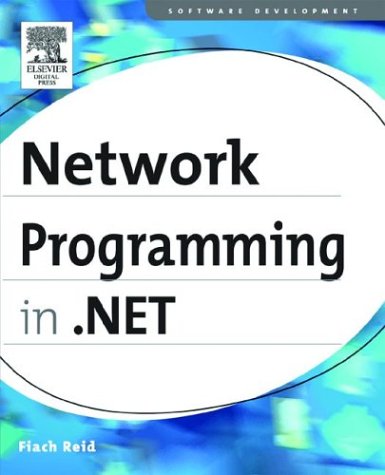
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- basecomparevalidator.cs
- ItemAutomationPeer.cs
- EtwTrace.cs
- CollectionConverter.cs
- ServiceModelExtensionCollectionElement.cs
- ThaiBuddhistCalendar.cs
- WebEventTraceProvider.cs
- OpenTypeCommon.cs
- FileInfo.cs
- InheritanceContextChangedEventManager.cs
- CatalogZone.cs
- DesignerRegion.cs
- SystemNetHelpers.cs
- MouseEvent.cs
- ParenthesizePropertyNameAttribute.cs
- FixedHyperLink.cs
- DescendantOverDescendantQuery.cs
- LinearGradientBrush.cs
- LZCodec.cs
- datacache.cs
- DbConvert.cs
- ArithmeticException.cs
- TableSectionStyle.cs
- DecimalStorage.cs
- EventDescriptorCollection.cs
- SubclassTypeValidatorAttribute.cs
- Evidence.cs
- SQLInt64.cs
- Set.cs
- AppDomainShutdownMonitor.cs
- SqlInfoMessageEvent.cs
- DataControlExtensions.cs
- GeometryDrawing.cs
- TrustManagerPromptUI.cs
- ListControlDesigner.cs
- DefaultTypeArgumentAttribute.cs
- ObjectToIdCache.cs
- FileRecordSequenceHelper.cs
- OleServicesContext.cs
- DataGridColumnFloatingHeader.cs
- FilteredDataSetHelper.cs
- ConversionContext.cs
- CngAlgorithmGroup.cs
- GetPolicyDetailsRequest.cs
- MailSettingsSection.cs
- SmiEventSink.cs
- EnvelopedPkcs7.cs
- RangeValidator.cs
- ReadOnlyHierarchicalDataSource.cs
- Win32Exception.cs
- NativeMethods.cs
- CreateUserErrorEventArgs.cs
- UIElementParaClient.cs
- RectAnimation.cs
- EntityViewContainer.cs
- EntityDataSourceUtil.cs
- IdleTimeoutMonitor.cs
- BitVec.cs
- SystemThemeKey.cs
- StackOverflowException.cs
- UserControlParser.cs
- SqlDataSourceCache.cs
- GetWinFXPath.cs
- RadioButton.cs
- IntegerFacetDescriptionElement.cs
- OletxTransactionManager.cs
- ModuleBuilder.cs
- State.cs
- SplitContainer.cs
- FrameAutomationPeer.cs
- HttpServerVarsCollection.cs
- ListManagerBindingsCollection.cs
- Panel.cs
- MenuItemCollection.cs
- WorkflowInstanceExtensionManager.cs
- SessionStateItemCollection.cs
- PublisherIdentityPermission.cs
- DataSourceHelper.cs
- Hashtable.cs
- ValidatedControlConverter.cs
- ReaderWriterLockWrapper.cs
- GlyphRunDrawing.cs
- recordstate.cs
- Scheduler.cs
- SchemaElementDecl.cs
- SymDocumentType.cs
- XsltException.cs
- FormViewUpdateEventArgs.cs
- OlePropertyStructs.cs
- StylusPointCollection.cs
- DoubleIndependentAnimationStorage.cs
- APCustomTypeDescriptor.cs
- DataServiceRequestException.cs
- DataGridViewEditingControlShowingEventArgs.cs
- WpfKnownMemberInvoker.cs
- ToolStripDropDownItem.cs
- TextTreeUndoUnit.cs
- DataStorage.cs
- TextParentUndoUnit.cs
- PeerApplication.cs