Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / Xml / System / Xml / Dom / XmlAttributeCollection.cs / 1 / XmlAttributeCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System; using System.Collections; using System.Diagnostics; // Represents a collection of attributes that can be accessed by name or index. public sealed class XmlAttributeCollection: XmlNamedNodeMap, ICollection { internal XmlAttributeCollection( XmlNode parent ): base( parent ) { } // Gets the attribute with the specified index. [System.Runtime.CompilerServices.IndexerName ("ItemOf")] public XmlAttribute this[ int i ] { get { try { return(XmlAttribute) Nodes[i]; } catch ( ArgumentOutOfRangeException ) { throw new IndexOutOfRangeException(Res.GetString(Res.Xdom_IndexOutOfRange)); } } } // Gets the attribute with the specified name. [System.Runtime.CompilerServices.IndexerName ("ItemOf")] public XmlAttribute this[ string name ] { get { ArrayList nodes = this.Nodes; int hash = XmlName.GetHashCode(name); for (int i = 0; i < nodes.Count; i++) { XmlAttribute node = (XmlAttribute) nodes[i]; if (hash == node.LocalNameHash && name == node.Name ) { return node; } } return null; } } // Gets the attribute with the specified LocalName and NamespaceUri. [System.Runtime.CompilerServices.IndexerName ("ItemOf")] public XmlAttribute this[ string localName, string namespaceURI ] { get { ArrayList nodes = this.Nodes; int hash = XmlName.GetHashCode(localName); for (int i = 0; i < nodes.Count; i++) { XmlAttribute node = (XmlAttribute) nodes[i]; if (hash == node.LocalNameHash && localName == node.LocalName && namespaceURI == node.NamespaceURI) { return node; } } return null; } } internal int FindNodeOffset( XmlAttribute node ) { ArrayList nodes = this.Nodes; for (int i = 0; i < nodes.Count; i++) { XmlAttribute tmp = (XmlAttribute) nodes[i]; if (tmp.LocalNameHash == node.LocalNameHash && tmp.Name == node.Name && tmp.NamespaceURI == node.NamespaceURI ) { return i; } } return -1; } internal int FindNodeOffsetNS(XmlAttribute node) { ArrayList nodes = Nodes; for (int i = 0; i < nodes.Count; i++) { XmlAttribute tmp = (XmlAttribute) nodes[i]; if (tmp.LocalNameHash == node.LocalNameHash && tmp.LocalName == node.LocalName && tmp.NamespaceURI == node.NamespaceURI) { return i; } } return -1; } // Adds a XmlNode using its Name property public override XmlNode SetNamedItem(XmlNode node) { if (node != null && !(node is XmlAttribute)) throw new ArgumentException(Res.GetString(Res.Xdom_AttrCol_Object)); int offset = FindNodeOffset( node.LocalName, node.NamespaceURI ); if (offset == -1) { return InternalAppendAttribute( (XmlAttribute) node ); } else { XmlNode oldNode = base.RemoveNodeAt( offset ); InsertNodeAt( offset, node ); return oldNode; } } // Inserts the specified node as the first node in the collection. public XmlAttribute Prepend( XmlAttribute node ) { if (node.OwnerDocument != null && node.OwnerDocument != parent.OwnerDocument) throw new ArgumentException(Res.GetString(Res.Xdom_NamedNode_Context)); if (node.OwnerElement != null) Detach( node ); RemoveDuplicateAttribute( node ); InsertNodeAt( 0, node ); return node; } // Inserts the specified node as the last node in the collection. public XmlAttribute Append(XmlAttribute node) { XmlDocument doc = node.OwnerDocument; if (doc == null || doc.IsLoading == false) { if (doc != null && doc != parent.OwnerDocument) { throw new ArgumentException(Res.GetString(Res.Xdom_NamedNode_Context)); } if (node.OwnerElement != null) { Detach(node); } AddNode(node); } else { base.AddNodeForLoad(node, doc); InsertParentIntoElementIdAttrMap(node); } return node; } // Inserts the specified attribute immediately before the specified reference attribute. public XmlAttribute InsertBefore( XmlAttribute newNode, XmlAttribute refNode ) { if ( newNode == refNode ) return newNode; if (refNode == null) return Append(newNode); if (refNode.OwnerElement != parent) throw new ArgumentException(Res.GetString(Res.Xdom_AttrCol_Insert)); if (newNode.OwnerDocument != null && newNode.OwnerDocument != parent.OwnerDocument) throw new ArgumentException(Res.GetString(Res.Xdom_NamedNode_Context)); if (newNode.OwnerElement != null) Detach( newNode ); int offset = FindNodeOffset( refNode.LocalName, refNode.NamespaceURI ); Debug.Assert( offset != -1 ); // the if statement above guarantees that the ref node is in the collection int dupoff = RemoveDuplicateAttribute( newNode ); if ( dupoff >= 0 && dupoff < offset ) offset--; InsertNodeAt( offset, newNode ); return newNode; } // Inserts the specified attribute immediately after the specified reference attribute. public XmlAttribute InsertAfter( XmlAttribute newNode, XmlAttribute refNode ) { if ( newNode == refNode ) return newNode; if (refNode == null) return Prepend(newNode); if (refNode.OwnerElement != parent) throw new ArgumentException(Res.GetString(Res.Xdom_AttrCol_Insert)); if (newNode.OwnerDocument != null && newNode.OwnerDocument != parent.OwnerDocument) throw new ArgumentException(Res.GetString(Res.Xdom_NamedNode_Context)); if (newNode.OwnerElement != null) Detach( newNode ); int offset = FindNodeOffset( refNode.LocalName, refNode.NamespaceURI ); Debug.Assert( offset != -1 ); // the if statement above guarantees that the ref node is in the collection int dupoff = RemoveDuplicateAttribute( newNode ); if ( dupoff >= 0 && dupoff < offset ) offset--; InsertNodeAt( offset+1, newNode ); return newNode; } // Removes the specified attribute node from the map. public XmlAttribute Remove( XmlAttribute node ) { if (nodes != null) { int cNodes = nodes.Count; for (int offset = 0; offset < cNodes; offset++) { if (nodes[offset] == node) { RemoveNodeAt( offset ); return node; } } } return null; } // Removes the attribute node with the specified index from the map. public XmlAttribute RemoveAt( int i ) { if (i < 0 || i >= Count || nodes == null) return null; return(XmlAttribute) RemoveNodeAt( i ); } // Removes all attributes from the map. public void RemoveAll() { int n = Count; while (n > 0) { n--; RemoveAt( n ); } } void ICollection.CopyTo(Array array, int index) { for (int i=0, max=Nodes.Count; i
Link Menu
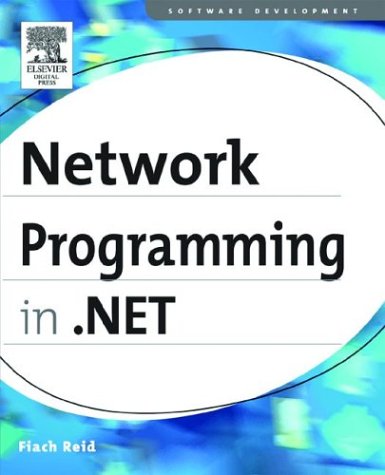
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RenderData.cs
- ShaderRenderModeValidation.cs
- WorkflowCreationContext.cs
- ProjectionPlan.cs
- HttpGetProtocolReflector.cs
- SQLRoleProvider.cs
- WinEventQueueItem.cs
- Substitution.cs
- ContainerUtilities.cs
- JpegBitmapEncoder.cs
- EventSinkHelperWriter.cs
- BehaviorEditorPart.cs
- WorkflowItemPresenter.cs
- WebBrowser.cs
- HostedTransportConfigurationBase.cs
- XmlTextEncoder.cs
- DebuggerAttributes.cs
- Native.cs
- InteropAutomationProvider.cs
- HtmlInputCheckBox.cs
- CommonObjectSecurity.cs
- xmlNames.cs
- TagElement.cs
- SessionSwitchEventArgs.cs
- BufferedGraphics.cs
- TreeNodeClickEventArgs.cs
- DebugView.cs
- WindowsAltTab.cs
- SwitchAttribute.cs
- PageTheme.cs
- DataSourceControlBuilder.cs
- CollectionBase.cs
- Size.cs
- DecryptRequest.cs
- DataView.cs
- StatusBarPanelClickEvent.cs
- OleDbConnectionInternal.cs
- HiddenField.cs
- InvalidComObjectException.cs
- BuildProvidersCompiler.cs
- SqlServer2KCompatibilityAnnotation.cs
- ByteAnimationBase.cs
- documentsequencetextview.cs
- IsolatedStoragePermission.cs
- WindowsClaimSet.cs
- PolicyManager.cs
- AesCryptoServiceProvider.cs
- CellLabel.cs
- Int32CollectionConverter.cs
- ExpressionBindings.cs
- Span.cs
- RichTextBox.cs
- RangeValueProviderWrapper.cs
- BitmapEffectDrawingContent.cs
- Validator.cs
- PenThreadWorker.cs
- QuadraticBezierSegment.cs
- NameValuePair.cs
- ActiveDocumentEvent.cs
- Type.cs
- Trace.cs
- EventHandlersStore.cs
- SiteMembershipCondition.cs
- SafeMarshalContext.cs
- BaseTemplateBuildProvider.cs
- RuntimeConfigurationRecord.cs
- LineGeometry.cs
- FindProgressChangedEventArgs.cs
- OdbcParameter.cs
- RootAction.cs
- XmlDataImplementation.cs
- EntityDataSourceDesignerHelper.cs
- WmlTextBoxAdapter.cs
- QueryStringHandler.cs
- DataServices.cs
- MetadataUtilsSmi.cs
- WindowPattern.cs
- TextComposition.cs
- ScrollBar.cs
- EdmTypeAttribute.cs
- Matrix.cs
- RadialGradientBrush.cs
- arabicshape.cs
- RunWorkerCompletedEventArgs.cs
- SQLInt64.cs
- PixelShader.cs
- ToolboxCategoryItems.cs
- ProtectedConfigurationSection.cs
- DataTableReaderListener.cs
- TimeSpanFormat.cs
- BlockExpression.cs
- XmlQualifiedNameTest.cs
- OpenTypeLayoutCache.cs
- ListControl.cs
- GPRECT.cs
- TransformerInfo.cs
- EnumCodeDomSerializer.cs
- IconHelper.cs
- SubclassTypeValidator.cs
- EntityDataSourceChangingEventArgs.cs